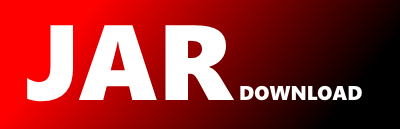
software.amazon.awssdk.services.iot.model.CertificateDescription Maven / Gradle / Ivy
Show all versions of iot Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iot.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes a certificate.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CertificateDescription implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CERTIFICATE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("certificateArn").getter(getter(CertificateDescription::certificateArn))
.setter(setter(Builder::certificateArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificateArn").build()).build();
private static final SdkField CERTIFICATE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("certificateId").getter(getter(CertificateDescription::certificateId))
.setter(setter(Builder::certificateId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificateId").build()).build();
private static final SdkField CA_CERTIFICATE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("caCertificateId").getter(getter(CertificateDescription::caCertificateId))
.setter(setter(Builder::caCertificateId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("caCertificateId").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(CertificateDescription::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField CERTIFICATE_PEM_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("certificatePem").getter(getter(CertificateDescription::certificatePem))
.setter(setter(Builder::certificatePem))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificatePem").build()).build();
private static final SdkField OWNED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ownedBy").getter(getter(CertificateDescription::ownedBy)).setter(setter(Builder::ownedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ownedBy").build()).build();
private static final SdkField PREVIOUS_OWNED_BY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("previousOwnedBy").getter(getter(CertificateDescription::previousOwnedBy))
.setter(setter(Builder::previousOwnedBy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("previousOwnedBy").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("creationDate").getter(getter(CertificateDescription::creationDate))
.setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationDate").build()).build();
private static final SdkField LAST_MODIFIED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastModifiedDate").getter(getter(CertificateDescription::lastModifiedDate))
.setter(setter(Builder::lastModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastModifiedDate").build()).build();
private static final SdkField CUSTOMER_VERSION_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("customerVersion").getter(getter(CertificateDescription::customerVersion))
.setter(setter(Builder::customerVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("customerVersion").build()).build();
private static final SdkField TRANSFER_DATA_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("transferData").getter(getter(CertificateDescription::transferData))
.setter(setter(Builder::transferData)).constructor(TransferData::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("transferData").build()).build();
private static final SdkField GENERATION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("generationId").getter(getter(CertificateDescription::generationId))
.setter(setter(Builder::generationId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("generationId").build()).build();
private static final SdkField VALIDITY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("validity")
.getter(getter(CertificateDescription::validity)).setter(setter(Builder::validity))
.constructor(CertificateValidity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("validity").build()).build();
private static final SdkField CERTIFICATE_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("certificateMode").getter(getter(CertificateDescription::certificateModeAsString))
.setter(setter(Builder::certificateMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("certificateMode").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CERTIFICATE_ARN_FIELD,
CERTIFICATE_ID_FIELD, CA_CERTIFICATE_ID_FIELD, STATUS_FIELD, CERTIFICATE_PEM_FIELD, OWNED_BY_FIELD,
PREVIOUS_OWNED_BY_FIELD, CREATION_DATE_FIELD, LAST_MODIFIED_DATE_FIELD, CUSTOMER_VERSION_FIELD, TRANSFER_DATA_FIELD,
GENERATION_ID_FIELD, VALIDITY_FIELD, CERTIFICATE_MODE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String certificateArn;
private final String certificateId;
private final String caCertificateId;
private final String status;
private final String certificatePem;
private final String ownedBy;
private final String previousOwnedBy;
private final Instant creationDate;
private final Instant lastModifiedDate;
private final Integer customerVersion;
private final TransferData transferData;
private final String generationId;
private final CertificateValidity validity;
private final String certificateMode;
private CertificateDescription(BuilderImpl builder) {
this.certificateArn = builder.certificateArn;
this.certificateId = builder.certificateId;
this.caCertificateId = builder.caCertificateId;
this.status = builder.status;
this.certificatePem = builder.certificatePem;
this.ownedBy = builder.ownedBy;
this.previousOwnedBy = builder.previousOwnedBy;
this.creationDate = builder.creationDate;
this.lastModifiedDate = builder.lastModifiedDate;
this.customerVersion = builder.customerVersion;
this.transferData = builder.transferData;
this.generationId = builder.generationId;
this.validity = builder.validity;
this.certificateMode = builder.certificateMode;
}
/**
*
* The ARN of the certificate.
*
*
* @return The ARN of the certificate.
*/
public final String certificateArn() {
return certificateArn;
}
/**
*
* The ID of the certificate.
*
*
* @return The ID of the certificate.
*/
public final String certificateId() {
return certificateId;
}
/**
*
* The certificate ID of the CA certificate used to sign this certificate.
*
*
* @return The certificate ID of the CA certificate used to sign this certificate.
*/
public final String caCertificateId() {
return caCertificateId;
}
/**
*
* The status of the certificate.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link CertificateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the certificate.
* @see CertificateStatus
*/
public final CertificateStatus status() {
return CertificateStatus.fromValue(status);
}
/**
*
* The status of the certificate.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link CertificateStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the certificate.
* @see CertificateStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The certificate data, in PEM format.
*
*
* @return The certificate data, in PEM format.
*/
public final String certificatePem() {
return certificatePem;
}
/**
*
* The ID of the Amazon Web Services account that owns the certificate.
*
*
* @return The ID of the Amazon Web Services account that owns the certificate.
*/
public final String ownedBy() {
return ownedBy;
}
/**
*
* The ID of the Amazon Web Services account of the previous owner of the certificate.
*
*
* @return The ID of the Amazon Web Services account of the previous owner of the certificate.
*/
public final String previousOwnedBy() {
return previousOwnedBy;
}
/**
*
* The date and time the certificate was created.
*
*
* @return The date and time the certificate was created.
*/
public final Instant creationDate() {
return creationDate;
}
/**
*
* The date and time the certificate was last modified.
*
*
* @return The date and time the certificate was last modified.
*/
public final Instant lastModifiedDate() {
return lastModifiedDate;
}
/**
*
* The customer version of the certificate.
*
*
* @return The customer version of the certificate.
*/
public final Integer customerVersion() {
return customerVersion;
}
/**
*
* The transfer data.
*
*
* @return The transfer data.
*/
public final TransferData transferData() {
return transferData;
}
/**
*
* The generation ID of the certificate.
*
*
* @return The generation ID of the certificate.
*/
public final String generationId() {
return generationId;
}
/**
*
* When the certificate is valid.
*
*
* @return When the certificate is valid.
*/
public final CertificateValidity validity() {
return validity;
}
/**
*
* The mode of the certificate.
*
*
* DEFAULT
: A certificate in DEFAULT
mode is either generated by Amazon Web Services IoT
* Core or registered with an issuer certificate authority (CA) in DEFAULT
mode. Devices with
* certificates in DEFAULT
mode aren't required to send the Server Name Indication (SNI) extension when
* connecting to Amazon Web Services IoT Core. However, to use features such as custom domains and VPC endpoints, we
* recommend that you use the SNI extension when connecting to Amazon Web Services IoT Core.
*
*
* SNI_ONLY
: A certificate in SNI_ONLY
mode is registered without an issuer CA. Devices
* with certificates in SNI_ONLY
mode must send the SNI extension when connecting to Amazon Web
* Services IoT Core.
*
*
* For more information about the value for SNI extension, see Transport security in
* IoT.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #certificateMode}
* will return {@link CertificateMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #certificateModeAsString}.
*
*
* @return The mode of the certificate.
*
* DEFAULT
: A certificate in DEFAULT
mode is either generated by Amazon Web
* Services IoT Core or registered with an issuer certificate authority (CA) in DEFAULT
mode.
* Devices with certificates in DEFAULT
mode aren't required to send the Server Name Indication
* (SNI) extension when connecting to Amazon Web Services IoT Core. However, to use features such as custom
* domains and VPC endpoints, we recommend that you use the SNI extension when connecting to Amazon Web
* Services IoT Core.
*
*
* SNI_ONLY
: A certificate in SNI_ONLY
mode is registered without an issuer CA.
* Devices with certificates in SNI_ONLY
mode must send the SNI extension when connecting to
* Amazon Web Services IoT Core.
*
*
* For more information about the value for SNI extension, see Transport security
* in IoT.
* @see CertificateMode
*/
public final CertificateMode certificateMode() {
return CertificateMode.fromValue(certificateMode);
}
/**
*
* The mode of the certificate.
*
*
* DEFAULT
: A certificate in DEFAULT
mode is either generated by Amazon Web Services IoT
* Core or registered with an issuer certificate authority (CA) in DEFAULT
mode. Devices with
* certificates in DEFAULT
mode aren't required to send the Server Name Indication (SNI) extension when
* connecting to Amazon Web Services IoT Core. However, to use features such as custom domains and VPC endpoints, we
* recommend that you use the SNI extension when connecting to Amazon Web Services IoT Core.
*
*
* SNI_ONLY
: A certificate in SNI_ONLY
mode is registered without an issuer CA. Devices
* with certificates in SNI_ONLY
mode must send the SNI extension when connecting to Amazon Web
* Services IoT Core.
*
*
* For more information about the value for SNI extension, see Transport security in
* IoT.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #certificateMode}
* will return {@link CertificateMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #certificateModeAsString}.
*
*
* @return The mode of the certificate.
*
* DEFAULT
: A certificate in DEFAULT
mode is either generated by Amazon Web
* Services IoT Core or registered with an issuer certificate authority (CA) in DEFAULT
mode.
* Devices with certificates in DEFAULT
mode aren't required to send the Server Name Indication
* (SNI) extension when connecting to Amazon Web Services IoT Core. However, to use features such as custom
* domains and VPC endpoints, we recommend that you use the SNI extension when connecting to Amazon Web
* Services IoT Core.
*
*
* SNI_ONLY
: A certificate in SNI_ONLY
mode is registered without an issuer CA.
* Devices with certificates in SNI_ONLY
mode must send the SNI extension when connecting to
* Amazon Web Services IoT Core.
*
*
* For more information about the value for SNI extension, see Transport security
* in IoT.
* @see CertificateMode
*/
public final String certificateModeAsString() {
return certificateMode;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(certificateArn());
hashCode = 31 * hashCode + Objects.hashCode(certificateId());
hashCode = 31 * hashCode + Objects.hashCode(caCertificateId());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(certificatePem());
hashCode = 31 * hashCode + Objects.hashCode(ownedBy());
hashCode = 31 * hashCode + Objects.hashCode(previousOwnedBy());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedDate());
hashCode = 31 * hashCode + Objects.hashCode(customerVersion());
hashCode = 31 * hashCode + Objects.hashCode(transferData());
hashCode = 31 * hashCode + Objects.hashCode(generationId());
hashCode = 31 * hashCode + Objects.hashCode(validity());
hashCode = 31 * hashCode + Objects.hashCode(certificateModeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CertificateDescription)) {
return false;
}
CertificateDescription other = (CertificateDescription) obj;
return Objects.equals(certificateArn(), other.certificateArn()) && Objects.equals(certificateId(), other.certificateId())
&& Objects.equals(caCertificateId(), other.caCertificateId())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(certificatePem(), other.certificatePem()) && Objects.equals(ownedBy(), other.ownedBy())
&& Objects.equals(previousOwnedBy(), other.previousOwnedBy())
&& Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(lastModifiedDate(), other.lastModifiedDate())
&& Objects.equals(customerVersion(), other.customerVersion())
&& Objects.equals(transferData(), other.transferData()) && Objects.equals(generationId(), other.generationId())
&& Objects.equals(validity(), other.validity())
&& Objects.equals(certificateModeAsString(), other.certificateModeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CertificateDescription").add("CertificateArn", certificateArn())
.add("CertificateId", certificateId()).add("CaCertificateId", caCertificateId()).add("Status", statusAsString())
.add("CertificatePem", certificatePem()).add("OwnedBy", ownedBy()).add("PreviousOwnedBy", previousOwnedBy())
.add("CreationDate", creationDate()).add("LastModifiedDate", lastModifiedDate())
.add("CustomerVersion", customerVersion()).add("TransferData", transferData())
.add("GenerationId", generationId()).add("Validity", validity())
.add("CertificateMode", certificateModeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "certificateArn":
return Optional.ofNullable(clazz.cast(certificateArn()));
case "certificateId":
return Optional.ofNullable(clazz.cast(certificateId()));
case "caCertificateId":
return Optional.ofNullable(clazz.cast(caCertificateId()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "certificatePem":
return Optional.ofNullable(clazz.cast(certificatePem()));
case "ownedBy":
return Optional.ofNullable(clazz.cast(ownedBy()));
case "previousOwnedBy":
return Optional.ofNullable(clazz.cast(previousOwnedBy()));
case "creationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "lastModifiedDate":
return Optional.ofNullable(clazz.cast(lastModifiedDate()));
case "customerVersion":
return Optional.ofNullable(clazz.cast(customerVersion()));
case "transferData":
return Optional.ofNullable(clazz.cast(transferData()));
case "generationId":
return Optional.ofNullable(clazz.cast(generationId()));
case "validity":
return Optional.ofNullable(clazz.cast(validity()));
case "certificateMode":
return Optional.ofNullable(clazz.cast(certificateModeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("certificateArn", CERTIFICATE_ARN_FIELD);
map.put("certificateId", CERTIFICATE_ID_FIELD);
map.put("caCertificateId", CA_CERTIFICATE_ID_FIELD);
map.put("status", STATUS_FIELD);
map.put("certificatePem", CERTIFICATE_PEM_FIELD);
map.put("ownedBy", OWNED_BY_FIELD);
map.put("previousOwnedBy", PREVIOUS_OWNED_BY_FIELD);
map.put("creationDate", CREATION_DATE_FIELD);
map.put("lastModifiedDate", LAST_MODIFIED_DATE_FIELD);
map.put("customerVersion", CUSTOMER_VERSION_FIELD);
map.put("transferData", TRANSFER_DATA_FIELD);
map.put("generationId", GENERATION_ID_FIELD);
map.put("validity", VALIDITY_FIELD);
map.put("certificateMode", CERTIFICATE_MODE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function