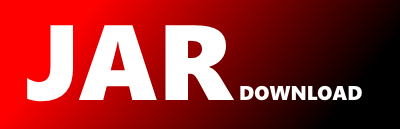
software.amazon.awssdk.services.iot.model.DescribeProvisioningTemplateResponse Maven / Gradle / Ivy
Show all versions of iot Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iot.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeProvisioningTemplateResponse extends IotResponse implements
ToCopyableBuilder {
private static final SdkField TEMPLATE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("templateArn").getter(getter(DescribeProvisioningTemplateResponse::templateArn))
.setter(setter(Builder::templateArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("templateArn").build()).build();
private static final SdkField TEMPLATE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("templateName").getter(getter(DescribeProvisioningTemplateResponse::templateName))
.setter(setter(Builder::templateName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("templateName").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(DescribeProvisioningTemplateResponse::description))
.setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("creationDate").getter(getter(DescribeProvisioningTemplateResponse::creationDate))
.setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationDate").build()).build();
private static final SdkField LAST_MODIFIED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastModifiedDate").getter(getter(DescribeProvisioningTemplateResponse::lastModifiedDate))
.setter(setter(Builder::lastModifiedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastModifiedDate").build()).build();
private static final SdkField DEFAULT_VERSION_ID_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("defaultVersionId").getter(getter(DescribeProvisioningTemplateResponse::defaultVersionId))
.setter(setter(Builder::defaultVersionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("defaultVersionId").build()).build();
private static final SdkField TEMPLATE_BODY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("templateBody").getter(getter(DescribeProvisioningTemplateResponse::templateBody))
.setter(setter(Builder::templateBody))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("templateBody").build()).build();
private static final SdkField ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("enabled").getter(getter(DescribeProvisioningTemplateResponse::enabled)).setter(setter(Builder::enabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("enabled").build()).build();
private static final SdkField PROVISIONING_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("provisioningRoleArn").getter(getter(DescribeProvisioningTemplateResponse::provisioningRoleArn))
.setter(setter(Builder::provisioningRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("provisioningRoleArn").build())
.build();
private static final SdkField PRE_PROVISIONING_HOOK_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("preProvisioningHook")
.getter(getter(DescribeProvisioningTemplateResponse::preProvisioningHook))
.setter(setter(Builder::preProvisioningHook)).constructor(ProvisioningHook::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("preProvisioningHook").build())
.build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(DescribeProvisioningTemplateResponse::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TEMPLATE_ARN_FIELD,
TEMPLATE_NAME_FIELD, DESCRIPTION_FIELD, CREATION_DATE_FIELD, LAST_MODIFIED_DATE_FIELD, DEFAULT_VERSION_ID_FIELD,
TEMPLATE_BODY_FIELD, ENABLED_FIELD, PROVISIONING_ROLE_ARN_FIELD, PRE_PROVISIONING_HOOK_FIELD, TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String templateArn;
private final String templateName;
private final String description;
private final Instant creationDate;
private final Instant lastModifiedDate;
private final Integer defaultVersionId;
private final String templateBody;
private final Boolean enabled;
private final String provisioningRoleArn;
private final ProvisioningHook preProvisioningHook;
private final String type;
private DescribeProvisioningTemplateResponse(BuilderImpl builder) {
super(builder);
this.templateArn = builder.templateArn;
this.templateName = builder.templateName;
this.description = builder.description;
this.creationDate = builder.creationDate;
this.lastModifiedDate = builder.lastModifiedDate;
this.defaultVersionId = builder.defaultVersionId;
this.templateBody = builder.templateBody;
this.enabled = builder.enabled;
this.provisioningRoleArn = builder.provisioningRoleArn;
this.preProvisioningHook = builder.preProvisioningHook;
this.type = builder.type;
}
/**
*
* The ARN of the provisioning template.
*
*
* @return The ARN of the provisioning template.
*/
public final String templateArn() {
return templateArn;
}
/**
*
* The name of the provisioning template.
*
*
* @return The name of the provisioning template.
*/
public final String templateName() {
return templateName;
}
/**
*
* The description of the provisioning template.
*
*
* @return The description of the provisioning template.
*/
public final String description() {
return description;
}
/**
*
* The date when the provisioning template was created.
*
*
* @return The date when the provisioning template was created.
*/
public final Instant creationDate() {
return creationDate;
}
/**
*
* The date when the provisioning template was last modified.
*
*
* @return The date when the provisioning template was last modified.
*/
public final Instant lastModifiedDate() {
return lastModifiedDate;
}
/**
*
* The default fleet template version ID.
*
*
* @return The default fleet template version ID.
*/
public final Integer defaultVersionId() {
return defaultVersionId;
}
/**
*
* The JSON formatted contents of the provisioning template.
*
*
* @return The JSON formatted contents of the provisioning template.
*/
public final String templateBody() {
return templateBody;
}
/**
*
* True if the provisioning template is enabled, otherwise false.
*
*
* @return True if the provisioning template is enabled, otherwise false.
*/
public final Boolean enabled() {
return enabled;
}
/**
*
* The ARN of the role associated with the provisioning template. This IoT role grants permission to provision a
* device.
*
*
* @return The ARN of the role associated with the provisioning template. This IoT role grants permission to
* provision a device.
*/
public final String provisioningRoleArn() {
return provisioningRoleArn;
}
/**
*
* Gets information about a pre-provisioned hook.
*
*
* @return Gets information about a pre-provisioned hook.
*/
public final ProvisioningHook preProvisioningHook() {
return preProvisioningHook;
}
/**
*
* The type you define in a provisioning template. You can create a template with only one type. You can't change
* the template type after its creation. The default value is FLEET_PROVISIONING
. For more information
* about provisioning template, see: Provisioning template.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TemplateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type you define in a provisioning template. You can create a template with only one type. You can't
* change the template type after its creation. The default value is FLEET_PROVISIONING
. For
* more information about provisioning template, see: Provisioning
* template.
* @see TemplateType
*/
public final TemplateType type() {
return TemplateType.fromValue(type);
}
/**
*
* The type you define in a provisioning template. You can create a template with only one type. You can't change
* the template type after its creation. The default value is FLEET_PROVISIONING
. For more information
* about provisioning template, see: Provisioning template.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link TemplateType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The type you define in a provisioning template. You can create a template with only one type. You can't
* change the template type after its creation. The default value is FLEET_PROVISIONING
. For
* more information about provisioning template, see: Provisioning
* template.
* @see TemplateType
*/
public final String typeAsString() {
return type;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(templateArn());
hashCode = 31 * hashCode + Objects.hashCode(templateName());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(lastModifiedDate());
hashCode = 31 * hashCode + Objects.hashCode(defaultVersionId());
hashCode = 31 * hashCode + Objects.hashCode(templateBody());
hashCode = 31 * hashCode + Objects.hashCode(enabled());
hashCode = 31 * hashCode + Objects.hashCode(provisioningRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(preProvisioningHook());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeProvisioningTemplateResponse)) {
return false;
}
DescribeProvisioningTemplateResponse other = (DescribeProvisioningTemplateResponse) obj;
return Objects.equals(templateArn(), other.templateArn()) && Objects.equals(templateName(), other.templateName())
&& Objects.equals(description(), other.description()) && Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(lastModifiedDate(), other.lastModifiedDate())
&& Objects.equals(defaultVersionId(), other.defaultVersionId())
&& Objects.equals(templateBody(), other.templateBody()) && Objects.equals(enabled(), other.enabled())
&& Objects.equals(provisioningRoleArn(), other.provisioningRoleArn())
&& Objects.equals(preProvisioningHook(), other.preProvisioningHook())
&& Objects.equals(typeAsString(), other.typeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeProvisioningTemplateResponse").add("TemplateArn", templateArn())
.add("TemplateName", templateName()).add("Description", description()).add("CreationDate", creationDate())
.add("LastModifiedDate", lastModifiedDate()).add("DefaultVersionId", defaultVersionId())
.add("TemplateBody", templateBody()).add("Enabled", enabled()).add("ProvisioningRoleArn", provisioningRoleArn())
.add("PreProvisioningHook", preProvisioningHook()).add("Type", typeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "templateArn":
return Optional.ofNullable(clazz.cast(templateArn()));
case "templateName":
return Optional.ofNullable(clazz.cast(templateName()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "creationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "lastModifiedDate":
return Optional.ofNullable(clazz.cast(lastModifiedDate()));
case "defaultVersionId":
return Optional.ofNullable(clazz.cast(defaultVersionId()));
case "templateBody":
return Optional.ofNullable(clazz.cast(templateBody()));
case "enabled":
return Optional.ofNullable(clazz.cast(enabled()));
case "provisioningRoleArn":
return Optional.ofNullable(clazz.cast(provisioningRoleArn()));
case "preProvisioningHook":
return Optional.ofNullable(clazz.cast(preProvisioningHook()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("templateArn", TEMPLATE_ARN_FIELD);
map.put("templateName", TEMPLATE_NAME_FIELD);
map.put("description", DESCRIPTION_FIELD);
map.put("creationDate", CREATION_DATE_FIELD);
map.put("lastModifiedDate", LAST_MODIFIED_DATE_FIELD);
map.put("defaultVersionId", DEFAULT_VERSION_ID_FIELD);
map.put("templateBody", TEMPLATE_BODY_FIELD);
map.put("enabled", ENABLED_FIELD);
map.put("provisioningRoleArn", PROVISIONING_ROLE_ARN_FIELD);
map.put("preProvisioningHook", PRE_PROVISIONING_HOOK_FIELD);
map.put("type", TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function