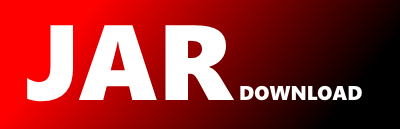
software.amazon.awssdk.services.iot.model.DetectMitigationActionsTaskSummary Maven / Gradle / Ivy
Show all versions of iot Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iot.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The summary of the mitigation action tasks.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class DetectMitigationActionsTaskSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TASK_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("taskId")
.getter(getter(DetectMitigationActionsTaskSummary::taskId)).setter(setter(Builder::taskId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskId").build()).build();
private static final SdkField TASK_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("taskStatus").getter(getter(DetectMitigationActionsTaskSummary::taskStatusAsString))
.setter(setter(Builder::taskStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskStatus").build()).build();
private static final SdkField TASK_START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("taskStartTime").getter(getter(DetectMitigationActionsTaskSummary::taskStartTime))
.setter(setter(Builder::taskStartTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskStartTime").build()).build();
private static final SdkField TASK_END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("taskEndTime").getter(getter(DetectMitigationActionsTaskSummary::taskEndTime))
.setter(setter(Builder::taskEndTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskEndTime").build()).build();
private static final SdkField TARGET_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("target")
.getter(getter(DetectMitigationActionsTaskSummary::target)).setter(setter(Builder::target))
.constructor(DetectMitigationActionsTaskTarget::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("target").build()).build();
private static final SdkField VIOLATION_EVENT_OCCURRENCE_RANGE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("violationEventOccurrenceRange")
.getter(getter(DetectMitigationActionsTaskSummary::violationEventOccurrenceRange))
.setter(setter(Builder::violationEventOccurrenceRange))
.constructor(ViolationEventOccurrenceRange::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("violationEventOccurrenceRange")
.build()).build();
private static final SdkField ONLY_ACTIVE_VIOLATIONS_INCLUDED_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("onlyActiveViolationsIncluded")
.getter(getter(DetectMitigationActionsTaskSummary::onlyActiveViolationsIncluded))
.setter(setter(Builder::onlyActiveViolationsIncluded))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("onlyActiveViolationsIncluded")
.build()).build();
private static final SdkField SUPPRESSED_ALERTS_INCLUDED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("suppressedAlertsIncluded").getter(getter(DetectMitigationActionsTaskSummary::suppressedAlertsIncluded))
.setter(setter(Builder::suppressedAlertsIncluded))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("suppressedAlertsIncluded").build())
.build();
private static final SdkField> ACTIONS_DEFINITION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("actionsDefinition")
.getter(getter(DetectMitigationActionsTaskSummary::actionsDefinition))
.setter(setter(Builder::actionsDefinition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("actionsDefinition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(MitigationAction::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField TASK_STATISTICS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("taskStatistics")
.getter(getter(DetectMitigationActionsTaskSummary::taskStatistics)).setter(setter(Builder::taskStatistics))
.constructor(DetectMitigationActionsTaskStatistics::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("taskStatistics").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TASK_ID_FIELD,
TASK_STATUS_FIELD, TASK_START_TIME_FIELD, TASK_END_TIME_FIELD, TARGET_FIELD, VIOLATION_EVENT_OCCURRENCE_RANGE_FIELD,
ONLY_ACTIVE_VIOLATIONS_INCLUDED_FIELD, SUPPRESSED_ALERTS_INCLUDED_FIELD, ACTIONS_DEFINITION_FIELD,
TASK_STATISTICS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String taskId;
private final String taskStatus;
private final Instant taskStartTime;
private final Instant taskEndTime;
private final DetectMitigationActionsTaskTarget target;
private final ViolationEventOccurrenceRange violationEventOccurrenceRange;
private final Boolean onlyActiveViolationsIncluded;
private final Boolean suppressedAlertsIncluded;
private final List actionsDefinition;
private final DetectMitigationActionsTaskStatistics taskStatistics;
private DetectMitigationActionsTaskSummary(BuilderImpl builder) {
this.taskId = builder.taskId;
this.taskStatus = builder.taskStatus;
this.taskStartTime = builder.taskStartTime;
this.taskEndTime = builder.taskEndTime;
this.target = builder.target;
this.violationEventOccurrenceRange = builder.violationEventOccurrenceRange;
this.onlyActiveViolationsIncluded = builder.onlyActiveViolationsIncluded;
this.suppressedAlertsIncluded = builder.suppressedAlertsIncluded;
this.actionsDefinition = builder.actionsDefinition;
this.taskStatistics = builder.taskStatistics;
}
/**
*
* The unique identifier of the task.
*
*
* @return The unique identifier of the task.
*/
public final String taskId() {
return taskId;
}
/**
*
* The status of the task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #taskStatus} will
* return {@link DetectMitigationActionsTaskStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #taskStatusAsString}.
*
*
* @return The status of the task.
* @see DetectMitigationActionsTaskStatus
*/
public final DetectMitigationActionsTaskStatus taskStatus() {
return DetectMitigationActionsTaskStatus.fromValue(taskStatus);
}
/**
*
* The status of the task.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #taskStatus} will
* return {@link DetectMitigationActionsTaskStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #taskStatusAsString}.
*
*
* @return The status of the task.
* @see DetectMitigationActionsTaskStatus
*/
public final String taskStatusAsString() {
return taskStatus;
}
/**
*
* The date the task started.
*
*
* @return The date the task started.
*/
public final Instant taskStartTime() {
return taskStartTime;
}
/**
*
* The date the task ended.
*
*
* @return The date the task ended.
*/
public final Instant taskEndTime() {
return taskEndTime;
}
/**
*
* Specifies the ML Detect findings to which the mitigation actions are applied.
*
*
* @return Specifies the ML Detect findings to which the mitigation actions are applied.
*/
public final DetectMitigationActionsTaskTarget target() {
return target;
}
/**
*
* Specifies the time period of which violation events occurred between.
*
*
* @return Specifies the time period of which violation events occurred between.
*/
public final ViolationEventOccurrenceRange violationEventOccurrenceRange() {
return violationEventOccurrenceRange;
}
/**
*
* Includes only active violations.
*
*
* @return Includes only active violations.
*/
public final Boolean onlyActiveViolationsIncluded() {
return onlyActiveViolationsIncluded;
}
/**
*
* Includes suppressed alerts.
*
*
* @return Includes suppressed alerts.
*/
public final Boolean suppressedAlertsIncluded() {
return suppressedAlertsIncluded;
}
/**
* For responses, this returns true if the service returned a value for the ActionsDefinition property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasActionsDefinition() {
return actionsDefinition != null && !(actionsDefinition instanceof SdkAutoConstructList);
}
/**
*
* The definition of the actions.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasActionsDefinition} method.
*
*
* @return The definition of the actions.
*/
public final List actionsDefinition() {
return actionsDefinition;
}
/**
*
* The statistics of a mitigation action task.
*
*
* @return The statistics of a mitigation action task.
*/
public final DetectMitigationActionsTaskStatistics taskStatistics() {
return taskStatistics;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(taskId());
hashCode = 31 * hashCode + Objects.hashCode(taskStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(taskStartTime());
hashCode = 31 * hashCode + Objects.hashCode(taskEndTime());
hashCode = 31 * hashCode + Objects.hashCode(target());
hashCode = 31 * hashCode + Objects.hashCode(violationEventOccurrenceRange());
hashCode = 31 * hashCode + Objects.hashCode(onlyActiveViolationsIncluded());
hashCode = 31 * hashCode + Objects.hashCode(suppressedAlertsIncluded());
hashCode = 31 * hashCode + Objects.hashCode(hasActionsDefinition() ? actionsDefinition() : null);
hashCode = 31 * hashCode + Objects.hashCode(taskStatistics());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DetectMitigationActionsTaskSummary)) {
return false;
}
DetectMitigationActionsTaskSummary other = (DetectMitigationActionsTaskSummary) obj;
return Objects.equals(taskId(), other.taskId()) && Objects.equals(taskStatusAsString(), other.taskStatusAsString())
&& Objects.equals(taskStartTime(), other.taskStartTime()) && Objects.equals(taskEndTime(), other.taskEndTime())
&& Objects.equals(target(), other.target())
&& Objects.equals(violationEventOccurrenceRange(), other.violationEventOccurrenceRange())
&& Objects.equals(onlyActiveViolationsIncluded(), other.onlyActiveViolationsIncluded())
&& Objects.equals(suppressedAlertsIncluded(), other.suppressedAlertsIncluded())
&& hasActionsDefinition() == other.hasActionsDefinition()
&& Objects.equals(actionsDefinition(), other.actionsDefinition())
&& Objects.equals(taskStatistics(), other.taskStatistics());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DetectMitigationActionsTaskSummary").add("TaskId", taskId())
.add("TaskStatus", taskStatusAsString()).add("TaskStartTime", taskStartTime()).add("TaskEndTime", taskEndTime())
.add("Target", target()).add("ViolationEventOccurrenceRange", violationEventOccurrenceRange())
.add("OnlyActiveViolationsIncluded", onlyActiveViolationsIncluded())
.add("SuppressedAlertsIncluded", suppressedAlertsIncluded())
.add("ActionsDefinition", hasActionsDefinition() ? actionsDefinition() : null)
.add("TaskStatistics", taskStatistics()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "taskId":
return Optional.ofNullable(clazz.cast(taskId()));
case "taskStatus":
return Optional.ofNullable(clazz.cast(taskStatusAsString()));
case "taskStartTime":
return Optional.ofNullable(clazz.cast(taskStartTime()));
case "taskEndTime":
return Optional.ofNullable(clazz.cast(taskEndTime()));
case "target":
return Optional.ofNullable(clazz.cast(target()));
case "violationEventOccurrenceRange":
return Optional.ofNullable(clazz.cast(violationEventOccurrenceRange()));
case "onlyActiveViolationsIncluded":
return Optional.ofNullable(clazz.cast(onlyActiveViolationsIncluded()));
case "suppressedAlertsIncluded":
return Optional.ofNullable(clazz.cast(suppressedAlertsIncluded()));
case "actionsDefinition":
return Optional.ofNullable(clazz.cast(actionsDefinition()));
case "taskStatistics":
return Optional.ofNullable(clazz.cast(taskStatistics()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("taskId", TASK_ID_FIELD);
map.put("taskStatus", TASK_STATUS_FIELD);
map.put("taskStartTime", TASK_START_TIME_FIELD);
map.put("taskEndTime", TASK_END_TIME_FIELD);
map.put("target", TARGET_FIELD);
map.put("violationEventOccurrenceRange", VIOLATION_EVENT_OCCURRENCE_RANGE_FIELD);
map.put("onlyActiveViolationsIncluded", ONLY_ACTIVE_VIOLATIONS_INCLUDED_FIELD);
map.put("suppressedAlertsIncluded", SUPPRESSED_ALERTS_INCLUDED_FIELD);
map.put("actionsDefinition", ACTIONS_DEFINITION_FIELD);
map.put("taskStatistics", TASK_STATISTICS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function