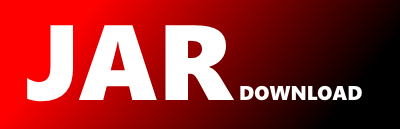
software.amazon.awssdk.services.iot.model.LocationAction Maven / Gradle / Ivy
Show all versions of iot Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iot.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The Amazon Location rule action sends device location updates from an MQTT message to an Amazon Location tracker
* resource.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LocationAction implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("roleArn").getter(getter(LocationAction::roleArn)).setter(setter(Builder::roleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("roleArn").build()).build();
private static final SdkField TRACKER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("trackerName").getter(getter(LocationAction::trackerName)).setter(setter(Builder::trackerName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("trackerName").build()).build();
private static final SdkField DEVICE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("deviceId").getter(getter(LocationAction::deviceId)).setter(setter(Builder::deviceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceId").build()).build();
private static final SdkField TIMESTAMP_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("timestamp")
.getter(getter(LocationAction::timestamp)).setter(setter(Builder::timestamp)).constructor(LocationTimestamp::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timestamp").build()).build();
private static final SdkField LATITUDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("latitude").getter(getter(LocationAction::latitude)).setter(setter(Builder::latitude))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("latitude").build()).build();
private static final SdkField LONGITUDE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("longitude").getter(getter(LocationAction::longitude)).setter(setter(Builder::longitude))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("longitude").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ROLE_ARN_FIELD,
TRACKER_NAME_FIELD, DEVICE_ID_FIELD, TIMESTAMP_FIELD, LATITUDE_FIELD, LONGITUDE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String roleArn;
private final String trackerName;
private final String deviceId;
private final LocationTimestamp timestamp;
private final String latitude;
private final String longitude;
private LocationAction(BuilderImpl builder) {
this.roleArn = builder.roleArn;
this.trackerName = builder.trackerName;
this.deviceId = builder.deviceId;
this.timestamp = builder.timestamp;
this.latitude = builder.latitude;
this.longitude = builder.longitude;
}
/**
*
* The IAM role that grants permission to write to the Amazon Location resource.
*
*
* @return The IAM role that grants permission to write to the Amazon Location resource.
*/
public final String roleArn() {
return roleArn;
}
/**
*
* The name of the tracker resource in Amazon Location in which the location is updated.
*
*
* @return The name of the tracker resource in Amazon Location in which the location is updated.
*/
public final String trackerName() {
return trackerName;
}
/**
*
* The unique ID of the device providing the location data.
*
*
* @return The unique ID of the device providing the location data.
*/
public final String deviceId() {
return deviceId;
}
/**
*
* The time that the location data was sampled. The default value is the time the MQTT message was processed.
*
*
* @return The time that the location data was sampled. The default value is the time the MQTT message was
* processed.
*/
public final LocationTimestamp timestamp() {
return timestamp;
}
/**
*
* A string that evaluates to a double value that represents the latitude of the device's location.
*
*
* @return A string that evaluates to a double value that represents the latitude of the device's location.
*/
public final String latitude() {
return latitude;
}
/**
*
* A string that evaluates to a double value that represents the longitude of the device's location.
*
*
* @return A string that evaluates to a double value that represents the longitude of the device's location.
*/
public final String longitude() {
return longitude;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(roleArn());
hashCode = 31 * hashCode + Objects.hashCode(trackerName());
hashCode = 31 * hashCode + Objects.hashCode(deviceId());
hashCode = 31 * hashCode + Objects.hashCode(timestamp());
hashCode = 31 * hashCode + Objects.hashCode(latitude());
hashCode = 31 * hashCode + Objects.hashCode(longitude());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LocationAction)) {
return false;
}
LocationAction other = (LocationAction) obj;
return Objects.equals(roleArn(), other.roleArn()) && Objects.equals(trackerName(), other.trackerName())
&& Objects.equals(deviceId(), other.deviceId()) && Objects.equals(timestamp(), other.timestamp())
&& Objects.equals(latitude(), other.latitude()) && Objects.equals(longitude(), other.longitude());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LocationAction").add("RoleArn", roleArn()).add("TrackerName", trackerName())
.add("DeviceId", deviceId()).add("Timestamp", timestamp()).add("Latitude", latitude())
.add("Longitude", longitude()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "roleArn":
return Optional.ofNullable(clazz.cast(roleArn()));
case "trackerName":
return Optional.ofNullable(clazz.cast(trackerName()));
case "deviceId":
return Optional.ofNullable(clazz.cast(deviceId()));
case "timestamp":
return Optional.ofNullable(clazz.cast(timestamp()));
case "latitude":
return Optional.ofNullable(clazz.cast(latitude()));
case "longitude":
return Optional.ofNullable(clazz.cast(longitude()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("roleArn", ROLE_ARN_FIELD);
map.put("trackerName", TRACKER_NAME_FIELD);
map.put("deviceId", DEVICE_ID_FIELD);
map.put("timestamp", TIMESTAMP_FIELD);
map.put("latitude", LATITUDE_FIELD);
map.put("longitude", LONGITUDE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function