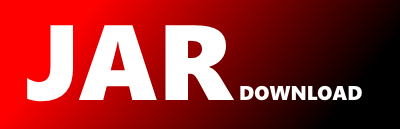
software.amazon.awssdk.services.iot.model.ThingIndexingConfiguration Maven / Gradle / Ivy
Show all versions of iot Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iot.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The thing indexing configuration. For more information, see Managing Thing Indexing.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ThingIndexingConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField THING_INDEXING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("thingIndexingMode").getter(getter(ThingIndexingConfiguration::thingIndexingModeAsString))
.setter(setter(Builder::thingIndexingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("thingIndexingMode").build()).build();
private static final SdkField THING_CONNECTIVITY_INDEXING_MODE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("thingConnectivityIndexingMode")
.getter(getter(ThingIndexingConfiguration::thingConnectivityIndexingModeAsString))
.setter(setter(Builder::thingConnectivityIndexingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("thingConnectivityIndexingMode")
.build()).build();
private static final SdkField DEVICE_DEFENDER_INDEXING_MODE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("deviceDefenderIndexingMode")
.getter(getter(ThingIndexingConfiguration::deviceDefenderIndexingModeAsString))
.setter(setter(Builder::deviceDefenderIndexingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("deviceDefenderIndexingMode").build())
.build();
private static final SdkField NAMED_SHADOW_INDEXING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("namedShadowIndexingMode").getter(getter(ThingIndexingConfiguration::namedShadowIndexingModeAsString))
.setter(setter(Builder::namedShadowIndexingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("namedShadowIndexingMode").build())
.build();
private static final SdkField> MANAGED_FIELDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("managedFields")
.getter(getter(ThingIndexingConfiguration::managedFields))
.setter(setter(Builder::managedFields))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("managedFields").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Field::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CUSTOM_FIELDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("customFields")
.getter(getter(ThingIndexingConfiguration::customFields))
.setter(setter(Builder::customFields))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("customFields").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Field::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField FILTER_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("filter").getter(getter(ThingIndexingConfiguration::filter)).setter(setter(Builder::filter))
.constructor(IndexingFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("filter").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(THING_INDEXING_MODE_FIELD,
THING_CONNECTIVITY_INDEXING_MODE_FIELD, DEVICE_DEFENDER_INDEXING_MODE_FIELD, NAMED_SHADOW_INDEXING_MODE_FIELD,
MANAGED_FIELDS_FIELD, CUSTOM_FIELDS_FIELD, FILTER_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String thingIndexingMode;
private final String thingConnectivityIndexingMode;
private final String deviceDefenderIndexingMode;
private final String namedShadowIndexingMode;
private final List managedFields;
private final List customFields;
private final IndexingFilter filter;
private ThingIndexingConfiguration(BuilderImpl builder) {
this.thingIndexingMode = builder.thingIndexingMode;
this.thingConnectivityIndexingMode = builder.thingConnectivityIndexingMode;
this.deviceDefenderIndexingMode = builder.deviceDefenderIndexingMode;
this.namedShadowIndexingMode = builder.namedShadowIndexingMode;
this.managedFields = builder.managedFields;
this.customFields = builder.customFields;
this.filter = builder.filter;
}
/**
*
* Thing indexing mode. Valid values are:
*
*
* -
*
* REGISTRY – Your thing index contains registry data only.
*
*
* -
*
* REGISTRY_AND_SHADOW - Your thing index contains registry and shadow data.
*
*
* -
*
* OFF - Thing indexing is disabled.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #thingIndexingMode}
* will return {@link ThingIndexingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #thingIndexingModeAsString}.
*
*
* @return Thing indexing mode. Valid values are:
*
* -
*
* REGISTRY – Your thing index contains registry data only.
*
*
* -
*
* REGISTRY_AND_SHADOW - Your thing index contains registry and shadow data.
*
*
* -
*
* OFF - Thing indexing is disabled.
*
*
* @see ThingIndexingMode
*/
public final ThingIndexingMode thingIndexingMode() {
return ThingIndexingMode.fromValue(thingIndexingMode);
}
/**
*
* Thing indexing mode. Valid values are:
*
*
* -
*
* REGISTRY – Your thing index contains registry data only.
*
*
* -
*
* REGISTRY_AND_SHADOW - Your thing index contains registry and shadow data.
*
*
* -
*
* OFF - Thing indexing is disabled.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #thingIndexingMode}
* will return {@link ThingIndexingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #thingIndexingModeAsString}.
*
*
* @return Thing indexing mode. Valid values are:
*
* -
*
* REGISTRY – Your thing index contains registry data only.
*
*
* -
*
* REGISTRY_AND_SHADOW - Your thing index contains registry and shadow data.
*
*
* -
*
* OFF - Thing indexing is disabled.
*
*
* @see ThingIndexingMode
*/
public final String thingIndexingModeAsString() {
return thingIndexingMode;
}
/**
*
* Thing connectivity indexing mode. Valid values are:
*
*
* -
*
* STATUS – Your thing index contains connectivity status. To enable thing connectivity indexing,
* thingIndexMode must not be set to OFF.
*
*
* -
*
* OFF - Thing connectivity status indexing is disabled.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #thingConnectivityIndexingMode} will return {@link ThingConnectivityIndexingMode#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #thingConnectivityIndexingModeAsString}.
*
*
* @return Thing connectivity indexing mode. Valid values are:
*
* -
*
* STATUS – Your thing index contains connectivity status. To enable thing connectivity indexing,
* thingIndexMode must not be set to OFF.
*
*
* -
*
* OFF - Thing connectivity status indexing is disabled.
*
*
* @see ThingConnectivityIndexingMode
*/
public final ThingConnectivityIndexingMode thingConnectivityIndexingMode() {
return ThingConnectivityIndexingMode.fromValue(thingConnectivityIndexingMode);
}
/**
*
* Thing connectivity indexing mode. Valid values are:
*
*
* -
*
* STATUS – Your thing index contains connectivity status. To enable thing connectivity indexing,
* thingIndexMode must not be set to OFF.
*
*
* -
*
* OFF - Thing connectivity status indexing is disabled.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #thingConnectivityIndexingMode} will return {@link ThingConnectivityIndexingMode#UNKNOWN_TO_SDK_VERSION}.
* The raw value returned by the service is available from {@link #thingConnectivityIndexingModeAsString}.
*
*
* @return Thing connectivity indexing mode. Valid values are:
*
* -
*
* STATUS – Your thing index contains connectivity status. To enable thing connectivity indexing,
* thingIndexMode must not be set to OFF.
*
*
* -
*
* OFF - Thing connectivity status indexing is disabled.
*
*
* @see ThingConnectivityIndexingMode
*/
public final String thingConnectivityIndexingModeAsString() {
return thingConnectivityIndexingMode;
}
/**
*
* Device Defender indexing mode. Valid values are:
*
*
* -
*
* VIOLATIONS – Your thing index contains Device Defender violations. To enable Device Defender indexing,
* deviceDefenderIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Device Defender indexing is disabled.
*
*
*
*
* For more information about Device Defender violations, see Device Defender
* Detect.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #deviceDefenderIndexingMode} will return {@link DeviceDefenderIndexingMode#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #deviceDefenderIndexingModeAsString}.
*
*
* @return Device Defender indexing mode. Valid values are:
*
* -
*
* VIOLATIONS – Your thing index contains Device Defender violations. To enable Device Defender indexing,
* deviceDefenderIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Device Defender indexing is disabled.
*
*
*
*
* For more information about Device Defender violations, see Device Defender
* Detect.
* @see DeviceDefenderIndexingMode
*/
public final DeviceDefenderIndexingMode deviceDefenderIndexingMode() {
return DeviceDefenderIndexingMode.fromValue(deviceDefenderIndexingMode);
}
/**
*
* Device Defender indexing mode. Valid values are:
*
*
* -
*
* VIOLATIONS – Your thing index contains Device Defender violations. To enable Device Defender indexing,
* deviceDefenderIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Device Defender indexing is disabled.
*
*
*
*
* For more information about Device Defender violations, see Device Defender
* Detect.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #deviceDefenderIndexingMode} will return {@link DeviceDefenderIndexingMode#UNKNOWN_TO_SDK_VERSION}. The
* raw value returned by the service is available from {@link #deviceDefenderIndexingModeAsString}.
*
*
* @return Device Defender indexing mode. Valid values are:
*
* -
*
* VIOLATIONS – Your thing index contains Device Defender violations. To enable Device Defender indexing,
* deviceDefenderIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Device Defender indexing is disabled.
*
*
*
*
* For more information about Device Defender violations, see Device Defender
* Detect.
* @see DeviceDefenderIndexingMode
*/
public final String deviceDefenderIndexingModeAsString() {
return deviceDefenderIndexingMode;
}
/**
*
* Named shadow indexing mode. Valid values are:
*
*
* -
*
* ON – Your thing index contains named shadow. To enable thing named shadow indexing,
* namedShadowIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Named shadow indexing is disabled.
*
*
*
*
* For more information about Shadows, see IoT Device Shadow
* service.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #namedShadowIndexingMode} will return {@link NamedShadowIndexingMode#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #namedShadowIndexingModeAsString}.
*
*
* @return Named shadow indexing mode. Valid values are:
*
* -
*
* ON – Your thing index contains named shadow. To enable thing named shadow indexing,
* namedShadowIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Named shadow indexing is disabled.
*
*
*
*
* For more information about Shadows, see IoT Device Shadow
* service.
* @see NamedShadowIndexingMode
*/
public final NamedShadowIndexingMode namedShadowIndexingMode() {
return NamedShadowIndexingMode.fromValue(namedShadowIndexingMode);
}
/**
*
* Named shadow indexing mode. Valid values are:
*
*
* -
*
* ON – Your thing index contains named shadow. To enable thing named shadow indexing,
* namedShadowIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Named shadow indexing is disabled.
*
*
*
*
* For more information about Shadows, see IoT Device Shadow
* service.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #namedShadowIndexingMode} will return {@link NamedShadowIndexingMode#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #namedShadowIndexingModeAsString}.
*
*
* @return Named shadow indexing mode. Valid values are:
*
* -
*
* ON – Your thing index contains named shadow. To enable thing named shadow indexing,
* namedShadowIndexingMode must not be set to OFF.
*
*
* -
*
* OFF - Named shadow indexing is disabled.
*
*
*
*
* For more information about Shadows, see IoT Device Shadow
* service.
* @see NamedShadowIndexingMode
*/
public final String namedShadowIndexingModeAsString() {
return namedShadowIndexingMode;
}
/**
* For responses, this returns true if the service returned a value for the ManagedFields property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasManagedFields() {
return managedFields != null && !(managedFields instanceof SdkAutoConstructList);
}
/**
*
* Contains fields that are indexed and whose types are already known by the Fleet Indexing service. This is an
* optional field. For more information, see Managed
* fields in the Amazon Web Services IoT Core Developer Guide.
*
*
*
* You can't modify managed fields by updating fleet indexing configuration.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasManagedFields} method.
*
*
* @return Contains fields that are indexed and whose types are already known by the Fleet Indexing service. This is
* an optional field. For more information, see Managed fields in the Amazon Web Services IoT Core Developer Guide.
*
* You can't modify managed fields by updating fleet indexing configuration.
*
*/
public final List managedFields() {
return managedFields;
}
/**
* For responses, this returns true if the service returned a value for the CustomFields property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasCustomFields() {
return customFields != null && !(customFields instanceof SdkAutoConstructList);
}
/**
*
* Contains custom field names and their data type.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCustomFields} method.
*
*
* @return Contains custom field names and their data type.
*/
public final List customFields() {
return customFields;
}
/**
*
* Provides additional selections for named shadows and geolocation data.
*
*
* To add named shadows to your fleet indexing configuration, set namedShadowIndexingMode
to be ON and
* specify your shadow names in namedShadowNames
filter.
*
*
* To add geolocation data to your fleet indexing configuration:
*
*
* -
*
* If you store geolocation data in a class/unnamed shadow, set thingIndexingMode
to be
* REGISTRY_AND_SHADOW
and specify your geolocation data in geoLocations
filter.
*
*
* -
*
* If you store geolocation data in a named shadow, set namedShadowIndexingMode
to be ON
,
* add the shadow name in namedShadowNames
filter, and specify your geolocation data in
* geoLocations
filter. For more information, see Managing fleet
* indexing.
*
*
*
*
* @return Provides additional selections for named shadows and geolocation data.
*
* To add named shadows to your fleet indexing configuration, set namedShadowIndexingMode
to be
* ON and specify your shadow names in namedShadowNames
filter.
*
*
* To add geolocation data to your fleet indexing configuration:
*
*
* -
*
* If you store geolocation data in a class/unnamed shadow, set thingIndexingMode
to be
* REGISTRY_AND_SHADOW
and specify your geolocation data in geoLocations
filter.
*
*
* -
*
* If you store geolocation data in a named shadow, set namedShadowIndexingMode
to be
* ON
, add the shadow name in namedShadowNames
filter, and specify your
* geolocation data in geoLocations
filter. For more information, see Managing fleet
* indexing.
*
*
*/
public final IndexingFilter filter() {
return filter;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(thingIndexingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(thingConnectivityIndexingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(deviceDefenderIndexingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(namedShadowIndexingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasManagedFields() ? managedFields() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasCustomFields() ? customFields() : null);
hashCode = 31 * hashCode + Objects.hashCode(filter());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ThingIndexingConfiguration)) {
return false;
}
ThingIndexingConfiguration other = (ThingIndexingConfiguration) obj;
return Objects.equals(thingIndexingModeAsString(), other.thingIndexingModeAsString())
&& Objects.equals(thingConnectivityIndexingModeAsString(), other.thingConnectivityIndexingModeAsString())
&& Objects.equals(deviceDefenderIndexingModeAsString(), other.deviceDefenderIndexingModeAsString())
&& Objects.equals(namedShadowIndexingModeAsString(), other.namedShadowIndexingModeAsString())
&& hasManagedFields() == other.hasManagedFields() && Objects.equals(managedFields(), other.managedFields())
&& hasCustomFields() == other.hasCustomFields() && Objects.equals(customFields(), other.customFields())
&& Objects.equals(filter(), other.filter());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ThingIndexingConfiguration").add("ThingIndexingMode", thingIndexingModeAsString())
.add("ThingConnectivityIndexingMode", thingConnectivityIndexingModeAsString())
.add("DeviceDefenderIndexingMode", deviceDefenderIndexingModeAsString())
.add("NamedShadowIndexingMode", namedShadowIndexingModeAsString())
.add("ManagedFields", hasManagedFields() ? managedFields() : null)
.add("CustomFields", hasCustomFields() ? customFields() : null).add("Filter", filter()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "thingIndexingMode":
return Optional.ofNullable(clazz.cast(thingIndexingModeAsString()));
case "thingConnectivityIndexingMode":
return Optional.ofNullable(clazz.cast(thingConnectivityIndexingModeAsString()));
case "deviceDefenderIndexingMode":
return Optional.ofNullable(clazz.cast(deviceDefenderIndexingModeAsString()));
case "namedShadowIndexingMode":
return Optional.ofNullable(clazz.cast(namedShadowIndexingModeAsString()));
case "managedFields":
return Optional.ofNullable(clazz.cast(managedFields()));
case "customFields":
return Optional.ofNullable(clazz.cast(customFields()));
case "filter":
return Optional.ofNullable(clazz.cast(filter()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("thingIndexingMode", THING_INDEXING_MODE_FIELD);
map.put("thingConnectivityIndexingMode", THING_CONNECTIVITY_INDEXING_MODE_FIELD);
map.put("deviceDefenderIndexingMode", DEVICE_DEFENDER_INDEXING_MODE_FIELD);
map.put("namedShadowIndexingMode", NAMED_SHADOW_INDEXING_MODE_FIELD);
map.put("managedFields", MANAGED_FIELDS_FIELD);
map.put("customFields", CUSTOM_FIELDS_FIELD);
map.put("filter", FILTER_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function