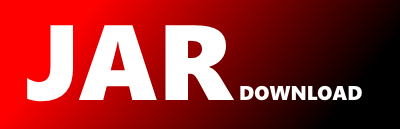
software.amazon.awssdk.services.iotdataplane.DefaultIotDataPlaneAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotdata Show documentation
Show all versions of iotdata Show documentation
The AWS Java SDK for AWS Iot Data Plane Service module holds the client classes that are used for communicating
with AWS IoT Data Plane Service
The newest version!
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotdataplane;
import java.util.concurrent.CompletableFuture;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.protocol.json.AwsJsonProtocol;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolMetadata;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.internal.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.protocol.json.JsonClientMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorResponseMetadata;
import software.amazon.awssdk.core.protocol.json.JsonErrorShapeMetadata;
import software.amazon.awssdk.core.protocol.json.JsonOperationMetadata;
import software.amazon.awssdk.services.iotdataplane.model.ConflictException;
import software.amazon.awssdk.services.iotdataplane.model.DeleteThingShadowRequest;
import software.amazon.awssdk.services.iotdataplane.model.DeleteThingShadowResponse;
import software.amazon.awssdk.services.iotdataplane.model.GetThingShadowRequest;
import software.amazon.awssdk.services.iotdataplane.model.GetThingShadowResponse;
import software.amazon.awssdk.services.iotdataplane.model.InternalFailureException;
import software.amazon.awssdk.services.iotdataplane.model.InvalidRequestException;
import software.amazon.awssdk.services.iotdataplane.model.MethodNotAllowedException;
import software.amazon.awssdk.services.iotdataplane.model.PublishRequest;
import software.amazon.awssdk.services.iotdataplane.model.PublishResponse;
import software.amazon.awssdk.services.iotdataplane.model.RequestEntityTooLargeException;
import software.amazon.awssdk.services.iotdataplane.model.ResourceNotFoundException;
import software.amazon.awssdk.services.iotdataplane.model.ServiceUnavailableException;
import software.amazon.awssdk.services.iotdataplane.model.ThrottlingException;
import software.amazon.awssdk.services.iotdataplane.model.UnauthorizedException;
import software.amazon.awssdk.services.iotdataplane.model.UnsupportedDocumentEncodingException;
import software.amazon.awssdk.services.iotdataplane.model.UpdateThingShadowRequest;
import software.amazon.awssdk.services.iotdataplane.model.UpdateThingShadowResponse;
import software.amazon.awssdk.services.iotdataplane.transform.DeleteThingShadowRequestMarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.DeleteThingShadowResponseUnmarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.GetThingShadowRequestMarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.GetThingShadowResponseUnmarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.PublishRequestMarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.PublishResponseUnmarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.UpdateThingShadowRequestMarshaller;
import software.amazon.awssdk.services.iotdataplane.transform.UpdateThingShadowResponseUnmarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link IotDataPlaneAsyncClient}.
*
* @see IotDataPlaneAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultIotDataPlaneAsyncClient implements IotDataPlaneAsyncClient {
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
protected DefaultIotDataPlaneAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.protocolFactory = init();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
/**
*
* Deletes the thing shadow for the specified thing.
*
*
* For more information, see DeleteThingShadow in
* the AWS IoT Developer Guide.
*
*
* @param deleteThingShadowRequest
* The input for the DeleteThingShadow operation.
* @return A Java Future containing the result of the DeleteThingShadow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException The specified resource does not exist.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The rate exceeds the limit.
* - UnauthorizedException You are not authorized to perform this operation.
* - ServiceUnavailableException The service is temporarily unavailable.
* - InternalFailureException An unexpected error has occurred.
* - MethodNotAllowedException The specified combination of HTTP verb and URI is not supported.
* - UnsupportedDocumentEncodingException The document encoding is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDataPlaneException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IotDataPlaneAsyncClient.DeleteThingShadow
*/
@Override
public CompletableFuture deleteThingShadow(DeleteThingShadowRequest deleteThingShadowRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(false),
new DeleteThingShadowResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new DeleteThingShadowRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteThingShadowRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the thing shadow for the specified thing.
*
*
* For more information, see GetThingShadow in the
* AWS IoT Developer Guide.
*
*
* @param getThingShadowRequest
* The input for the GetThingShadow operation.
* @return A Java Future containing the result of the GetThingShadow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InvalidRequestException The request is not valid.
* - ResourceNotFoundException The specified resource does not exist.
* - ThrottlingException The rate exceeds the limit.
* - UnauthorizedException You are not authorized to perform this operation.
* - ServiceUnavailableException The service is temporarily unavailable.
* - InternalFailureException An unexpected error has occurred.
* - MethodNotAllowedException The specified combination of HTTP verb and URI is not supported.
* - UnsupportedDocumentEncodingException The document encoding is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDataPlaneException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IotDataPlaneAsyncClient.GetThingShadow
*/
@Override
public CompletableFuture getThingShadow(GetThingShadowRequest getThingShadowRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(false),
new GetThingShadowResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new GetThingShadowRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getThingShadowRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Publishes state information.
*
*
* For more information, see HTTP
* Protocol in the AWS IoT Developer Guide.
*
*
* @param publishRequest
* The input for the Publish operation.
* @return A Java Future containing the result of the Publish operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalFailureException An unexpected error has occurred.
* - InvalidRequestException The request is not valid.
* - UnauthorizedException You are not authorized to perform this operation.
* - MethodNotAllowedException The specified combination of HTTP verb and URI is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDataPlaneException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IotDataPlaneAsyncClient.Publish
*/
@Override
public CompletableFuture publish(PublishRequest publishRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PublishResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new PublishRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(publishRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates the thing shadow for the specified thing.
*
*
* For more information, see UpdateThingShadow in
* the AWS IoT Developer Guide.
*
*
* @param updateThingShadowRequest
* The input for the UpdateThingShadow operation.
* @return A Java Future containing the result of the UpdateThingShadow operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ConflictException The specified version does not match the version of the document.
* - RequestEntityTooLargeException The payload exceeds the maximum size allowed.
* - InvalidRequestException The request is not valid.
* - ThrottlingException The rate exceeds the limit.
* - UnauthorizedException You are not authorized to perform this operation.
* - ServiceUnavailableException The service is temporarily unavailable.
* - InternalFailureException An unexpected error has occurred.
* - MethodNotAllowedException The specified combination of HTTP verb and URI is not supported.
* - UnsupportedDocumentEncodingException The document encoding is not supported.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDataPlaneException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IotDataPlaneAsyncClient.UpdateThingShadow
*/
@Override
public CompletableFuture updateThingShadow(UpdateThingShadowRequest updateThingShadowRequest) {
try {
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(false).withHasStreamingSuccessResponse(false),
new UpdateThingShadowResponseUnmarshaller());
HttpResponseHandler errorResponseHandler = createErrorResponseHandler();
return clientHandler.execute(new ClientExecutionParams()
.withMarshaller(new UpdateThingShadowRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateThingShadowRequest));
} catch (Throwable t) {
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public void close() {
clientHandler.close();
}
private software.amazon.awssdk.awscore.protocol.json.AwsJsonProtocolFactory init() {
return new AwsJsonProtocolFactory(
new JsonClientMetadata()
.withSupportsCbor(false)
.withSupportsIon(false)
.withBaseServiceExceptionClass(
software.amazon.awssdk.services.iotdataplane.model.IotDataPlaneException.class)
.withContentTypeOverride("")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnsupportedDocumentEncodingException")
.withModeledClass(UnsupportedDocumentEncodingException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("MethodNotAllowedException").withModeledClass(
MethodNotAllowedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withModeledClass(
ConflictException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withModeledClass(
ServiceUnavailableException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InternalFailureException").withModeledClass(
InternalFailureException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withModeledClass(
UnauthorizedException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("RequestEntityTooLargeException").withModeledClass(
RequestEntityTooLargeException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("InvalidRequestException").withModeledClass(
InvalidRequestException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withModeledClass(
ThrottlingException.class))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withModeledClass(
ResourceNotFoundException.class)), AwsJsonProtocolMetadata.builder()
.protocolVersion("1.1").protocol(AwsJsonProtocol.REST_JSON).build());
}
private HttpResponseHandler createErrorResponseHandler() {
return protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy