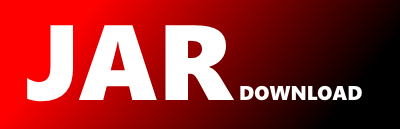
software.amazon.awssdk.services.iotdeviceadvisor.model.SuiteDefinitionConfiguration Maven / Gradle / Ivy
Show all versions of iotdeviceadvisor Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotdeviceadvisor.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Gets the suite definition configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SuiteDefinitionConfiguration implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField SUITE_DEFINITION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("suiteDefinitionName").getter(getter(SuiteDefinitionConfiguration::suiteDefinitionName))
.setter(setter(Builder::suiteDefinitionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("suiteDefinitionName").build())
.build();
private static final SdkField> DEVICES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("devices")
.getter(getter(SuiteDefinitionConfiguration::devices))
.setter(setter(Builder::devices))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("devices").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(DeviceUnderTest::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INTENDED_FOR_QUALIFICATION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("intendedForQualification").getter(getter(SuiteDefinitionConfiguration::intendedForQualification))
.setter(setter(Builder::intendedForQualification))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("intendedForQualification").build())
.build();
private static final SdkField IS_LONG_DURATION_TEST_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("isLongDurationTest").getter(getter(SuiteDefinitionConfiguration::isLongDurationTest))
.setter(setter(Builder::isLongDurationTest))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("isLongDurationTest").build())
.build();
private static final SdkField ROOT_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("rootGroup").getter(getter(SuiteDefinitionConfiguration::rootGroup)).setter(setter(Builder::rootGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rootGroup").build()).build();
private static final SdkField DEVICE_PERMISSION_ROLE_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("devicePermissionRoleArn").getter(getter(SuiteDefinitionConfiguration::devicePermissionRoleArn))
.setter(setter(Builder::devicePermissionRoleArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("devicePermissionRoleArn").build())
.build();
private static final SdkField PROTOCOL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("protocol").getter(getter(SuiteDefinitionConfiguration::protocolAsString))
.setter(setter(Builder::protocol))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("protocol").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(SUITE_DEFINITION_NAME_FIELD,
DEVICES_FIELD, INTENDED_FOR_QUALIFICATION_FIELD, IS_LONG_DURATION_TEST_FIELD, ROOT_GROUP_FIELD,
DEVICE_PERMISSION_ROLE_ARN_FIELD, PROTOCOL_FIELD));
private static final long serialVersionUID = 1L;
private final String suiteDefinitionName;
private final List devices;
private final Boolean intendedForQualification;
private final Boolean isLongDurationTest;
private final String rootGroup;
private final String devicePermissionRoleArn;
private final String protocol;
private SuiteDefinitionConfiguration(BuilderImpl builder) {
this.suiteDefinitionName = builder.suiteDefinitionName;
this.devices = builder.devices;
this.intendedForQualification = builder.intendedForQualification;
this.isLongDurationTest = builder.isLongDurationTest;
this.rootGroup = builder.rootGroup;
this.devicePermissionRoleArn = builder.devicePermissionRoleArn;
this.protocol = builder.protocol;
}
/**
*
* Gets the suite definition name. This is a required parameter.
*
*
* @return Gets the suite definition name. This is a required parameter.
*/
public final String suiteDefinitionName() {
return suiteDefinitionName;
}
/**
* For responses, this returns true if the service returned a value for the Devices property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasDevices() {
return devices != null && !(devices instanceof SdkAutoConstructList);
}
/**
*
* Gets the devices configured.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDevices} method.
*
*
* @return Gets the devices configured.
*/
public final List devices() {
return devices;
}
/**
*
* Gets the tests intended for qualification in a suite.
*
*
* @return Gets the tests intended for qualification in a suite.
*/
public final Boolean intendedForQualification() {
return intendedForQualification;
}
/**
*
* Verifies if the test suite is a long duration test.
*
*
* @return Verifies if the test suite is a long duration test.
*/
public final Boolean isLongDurationTest() {
return isLongDurationTest;
}
/**
*
* Gets the test suite root group. This is a required parameter. For updating or creating the latest qualification
* suite, if intendedForQualification
is set to true, rootGroup
can be an empty string. If
* intendedForQualification
is false, rootGroup
cannot be an empty string. If
* rootGroup
is empty, and intendedForQualification
is set to true, all the qualification
* tests are included, and the configuration is default.
*
*
* For a qualification suite, the minimum length is 0, and the maximum is 2048. For a non-qualification suite, the
* minimum length is 1, and the maximum is 2048.
*
*
* @return Gets the test suite root group. This is a required parameter. For updating or creating the latest
* qualification suite, if intendedForQualification
is set to true, rootGroup
can
* be an empty string. If intendedForQualification
is false, rootGroup
cannot be
* an empty string. If rootGroup
is empty, and intendedForQualification
is set to
* true, all the qualification tests are included, and the configuration is default.
*
* For a qualification suite, the minimum length is 0, and the maximum is 2048. For a non-qualification
* suite, the minimum length is 1, and the maximum is 2048.
*/
public final String rootGroup() {
return rootGroup;
}
/**
*
* Gets the device permission ARN. This is a required parameter.
*
*
* @return Gets the device permission ARN. This is a required parameter.
*/
public final String devicePermissionRoleArn() {
return devicePermissionRoleArn;
}
/**
*
* Sets the MQTT protocol that is configured in the suite definition.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link Protocol#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return Sets the MQTT protocol that is configured in the suite definition.
* @see Protocol
*/
public final Protocol protocol() {
return Protocol.fromValue(protocol);
}
/**
*
* Sets the MQTT protocol that is configured in the suite definition.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #protocol} will
* return {@link Protocol#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #protocolAsString}.
*
*
* @return Sets the MQTT protocol that is configured in the suite definition.
* @see Protocol
*/
public final String protocolAsString() {
return protocol;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(suiteDefinitionName());
hashCode = 31 * hashCode + Objects.hashCode(hasDevices() ? devices() : null);
hashCode = 31 * hashCode + Objects.hashCode(intendedForQualification());
hashCode = 31 * hashCode + Objects.hashCode(isLongDurationTest());
hashCode = 31 * hashCode + Objects.hashCode(rootGroup());
hashCode = 31 * hashCode + Objects.hashCode(devicePermissionRoleArn());
hashCode = 31 * hashCode + Objects.hashCode(protocolAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SuiteDefinitionConfiguration)) {
return false;
}
SuiteDefinitionConfiguration other = (SuiteDefinitionConfiguration) obj;
return Objects.equals(suiteDefinitionName(), other.suiteDefinitionName()) && hasDevices() == other.hasDevices()
&& Objects.equals(devices(), other.devices())
&& Objects.equals(intendedForQualification(), other.intendedForQualification())
&& Objects.equals(isLongDurationTest(), other.isLongDurationTest())
&& Objects.equals(rootGroup(), other.rootGroup())
&& Objects.equals(devicePermissionRoleArn(), other.devicePermissionRoleArn())
&& Objects.equals(protocolAsString(), other.protocolAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SuiteDefinitionConfiguration").add("SuiteDefinitionName", suiteDefinitionName())
.add("Devices", hasDevices() ? devices() : null).add("IntendedForQualification", intendedForQualification())
.add("IsLongDurationTest", isLongDurationTest()).add("RootGroup", rootGroup())
.add("DevicePermissionRoleArn", devicePermissionRoleArn()).add("Protocol", protocolAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "suiteDefinitionName":
return Optional.ofNullable(clazz.cast(suiteDefinitionName()));
case "devices":
return Optional.ofNullable(clazz.cast(devices()));
case "intendedForQualification":
return Optional.ofNullable(clazz.cast(intendedForQualification()));
case "isLongDurationTest":
return Optional.ofNullable(clazz.cast(isLongDurationTest()));
case "rootGroup":
return Optional.ofNullable(clazz.cast(rootGroup()));
case "devicePermissionRoleArn":
return Optional.ofNullable(clazz.cast(devicePermissionRoleArn()));
case "protocol":
return Optional.ofNullable(clazz.cast(protocolAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function