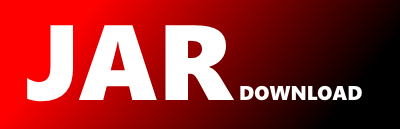
software.amazon.awssdk.services.iotdeviceadvisor.IotDeviceAdvisorAsyncClient Maven / Gradle / Ivy
Show all versions of iotdeviceadvisor Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotdeviceadvisor;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.iotdeviceadvisor.model.CreateSuiteDefinitionRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.CreateSuiteDefinitionResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.DeleteSuiteDefinitionRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.DeleteSuiteDefinitionResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetEndpointRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetEndpointResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteDefinitionRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteDefinitionResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteRunReportRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteRunReportResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteRunRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteRunResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.StartSuiteRunRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.StartSuiteRunResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.StopSuiteRunRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.StopSuiteRunResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.TagResourceRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.TagResourceResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.UntagResourceRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.UntagResourceResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.model.UpdateSuiteDefinitionRequest;
import software.amazon.awssdk.services.iotdeviceadvisor.model.UpdateSuiteDefinitionResponse;
import software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteDefinitionsPublisher;
import software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteRunsPublisher;
/**
* Service client for accessing AWSIoTDeviceAdvisor asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* Amazon Web Services IoT Core Device Advisor is a cloud-based, fully managed test capability for validating IoT
* devices during device software development. Device Advisor provides pre-built tests that you can use to validate IoT
* devices for reliable and secure connectivity with Amazon Web Services IoT Core before deploying devices to
* production. By using Device Advisor, you can confirm that your devices can connect to Amazon Web Services IoT Core,
* follow security best practices and, if applicable, receive software updates from IoT Device Management. You can also
* download signed qualification reports to submit to the Amazon Web Services Partner Network to get your device
* qualified for the Amazon Web Services Partner Device Catalog without the need to send your device in and wait for it
* to be tested.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface IotDeviceAdvisorAsyncClient extends AwsClient {
String SERVICE_NAME = "iotdeviceadvisor";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "api.iotdeviceadvisor";
/**
*
* Creates a Device Advisor test suite.
*
*
* Requires permission to access the CreateSuiteDefinition action.
*
*
* @param createSuiteDefinitionRequest
* @return A Java Future containing the result of the CreateSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.CreateSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture createSuiteDefinition(
CreateSuiteDefinitionRequest createSuiteDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a Device Advisor test suite.
*
*
* Requires permission to access the CreateSuiteDefinition action.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSuiteDefinitionRequest.Builder} avoiding the
* need to create one manually via {@link CreateSuiteDefinitionRequest#builder()}
*
*
* @param createSuiteDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.CreateSuiteDefinitionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the CreateSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.CreateSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture createSuiteDefinition(
Consumer createSuiteDefinitionRequest) {
return createSuiteDefinition(CreateSuiteDefinitionRequest.builder().applyMutation(createSuiteDefinitionRequest).build());
}
/**
*
* Deletes a Device Advisor test suite.
*
*
* Requires permission to access the DeleteSuiteDefinition action.
*
*
* @param deleteSuiteDefinitionRequest
* @return A Java Future containing the result of the DeleteSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.DeleteSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture deleteSuiteDefinition(
DeleteSuiteDefinitionRequest deleteSuiteDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a Device Advisor test suite.
*
*
* Requires permission to access the DeleteSuiteDefinition action.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSuiteDefinitionRequest.Builder} avoiding the
* need to create one manually via {@link DeleteSuiteDefinitionRequest#builder()}
*
*
* @param deleteSuiteDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.DeleteSuiteDefinitionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the DeleteSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.DeleteSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture deleteSuiteDefinition(
Consumer deleteSuiteDefinitionRequest) {
return deleteSuiteDefinition(DeleteSuiteDefinitionRequest.builder().applyMutation(deleteSuiteDefinitionRequest).build());
}
/**
*
* Gets information about an Device Advisor endpoint.
*
*
* @param getEndpointRequest
* @return A Java Future containing the result of the GetEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetEndpoint
* @see AWS
* API Documentation
*/
default CompletableFuture getEndpoint(GetEndpointRequest getEndpointRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about an Device Advisor endpoint.
*
*
*
* This is a convenience which creates an instance of the {@link GetEndpointRequest.Builder} avoiding the need to
* create one manually via {@link GetEndpointRequest#builder()}
*
*
* @param getEndpointRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.GetEndpointRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetEndpoint operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetEndpoint
* @see AWS
* API Documentation
*/
default CompletableFuture getEndpoint(Consumer getEndpointRequest) {
return getEndpoint(GetEndpointRequest.builder().applyMutation(getEndpointRequest).build());
}
/**
*
* Gets information about a Device Advisor test suite.
*
*
* Requires permission to access the GetSuiteDefinition action.
*
*
* @param getSuiteDefinitionRequest
* @return A Java Future containing the result of the GetSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture getSuiteDefinition(GetSuiteDefinitionRequest getSuiteDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a Device Advisor test suite.
*
*
* Requires permission to access the GetSuiteDefinition action.
*
*
*
* This is a convenience which creates an instance of the {@link GetSuiteDefinitionRequest.Builder} avoiding the
* need to create one manually via {@link GetSuiteDefinitionRequest#builder()}
*
*
* @param getSuiteDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteDefinitionRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture getSuiteDefinition(
Consumer getSuiteDefinitionRequest) {
return getSuiteDefinition(GetSuiteDefinitionRequest.builder().applyMutation(getSuiteDefinitionRequest).build());
}
/**
*
* Gets information about a Device Advisor test suite run.
*
*
* Requires permission to access the GetSuiteRun action.
*
*
* @param getSuiteRunRequest
* @return A Java Future containing the result of the GetSuiteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetSuiteRun
* @see AWS
* API Documentation
*/
default CompletableFuture getSuiteRun(GetSuiteRunRequest getSuiteRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about a Device Advisor test suite run.
*
*
* Requires permission to access the GetSuiteRun action.
*
*
*
* This is a convenience which creates an instance of the {@link GetSuiteRunRequest.Builder} avoiding the need to
* create one manually via {@link GetSuiteRunRequest#builder()}
*
*
* @param getSuiteRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteRunRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetSuiteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetSuiteRun
* @see AWS
* API Documentation
*/
default CompletableFuture getSuiteRun(Consumer getSuiteRunRequest) {
return getSuiteRun(GetSuiteRunRequest.builder().applyMutation(getSuiteRunRequest).build());
}
/**
*
* Gets a report download link for a successful Device Advisor qualifying test suite run.
*
*
* Requires permission to access the GetSuiteRunReport action.
*
*
* @param getSuiteRunReportRequest
* @return A Java Future containing the result of the GetSuiteRunReport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetSuiteRunReport
* @see AWS API Documentation
*/
default CompletableFuture getSuiteRunReport(GetSuiteRunReportRequest getSuiteRunReportRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a report download link for a successful Device Advisor qualifying test suite run.
*
*
* Requires permission to access the GetSuiteRunReport action.
*
*
*
* This is a convenience which creates an instance of the {@link GetSuiteRunReportRequest.Builder} avoiding the need
* to create one manually via {@link GetSuiteRunReportRequest#builder()}
*
*
* @param getSuiteRunReportRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.GetSuiteRunReportRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the GetSuiteRunReport operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.GetSuiteRunReport
* @see AWS API Documentation
*/
default CompletableFuture getSuiteRunReport(
Consumer getSuiteRunReportRequest) {
return getSuiteRunReport(GetSuiteRunReportRequest.builder().applyMutation(getSuiteRunReportRequest).build());
}
/**
*
* Lists the Device Advisor test suites you have created.
*
*
* Requires permission to access the ListSuiteDefinitions action.
*
*
* @param listSuiteDefinitionsRequest
* @return A Java Future containing the result of the ListSuiteDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteDefinitions
* @see AWS API Documentation
*/
default CompletableFuture listSuiteDefinitions(
ListSuiteDefinitionsRequest listSuiteDefinitionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the Device Advisor test suites you have created.
*
*
* Requires permission to access the ListSuiteDefinitions action.
*
*
*
* This is a convenience which creates an instance of the {@link ListSuiteDefinitionsRequest.Builder} avoiding the
* need to create one manually via {@link ListSuiteDefinitionsRequest#builder()}
*
*
* @param listSuiteDefinitionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListSuiteDefinitions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteDefinitions
* @see AWS API Documentation
*/
default CompletableFuture listSuiteDefinitions(
Consumer listSuiteDefinitionsRequest) {
return listSuiteDefinitions(ListSuiteDefinitionsRequest.builder().applyMutation(listSuiteDefinitionsRequest).build());
}
/**
*
* This is a variant of
* {@link #listSuiteDefinitions(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteDefinitionsPublisher publisher = client.listSuiteDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteDefinitionsPublisher publisher = client.listSuiteDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSuiteDefinitions(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest)}
* operation.
*
*
* @param listSuiteDefinitionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteDefinitions
* @see AWS API Documentation
*/
default ListSuiteDefinitionsPublisher listSuiteDefinitionsPaginator(ListSuiteDefinitionsRequest listSuiteDefinitionsRequest) {
return new ListSuiteDefinitionsPublisher(this, listSuiteDefinitionsRequest);
}
/**
*
* This is a variant of
* {@link #listSuiteDefinitions(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteDefinitionsPublisher publisher = client.listSuiteDefinitionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteDefinitionsPublisher publisher = client.listSuiteDefinitionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSuiteDefinitions(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSuiteDefinitionsRequest.Builder} avoiding the
* need to create one manually via {@link ListSuiteDefinitionsRequest#builder()}
*
*
* @param listSuiteDefinitionsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteDefinitionsRequest.Builder} to
* create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteDefinitions
* @see AWS API Documentation
*/
default ListSuiteDefinitionsPublisher listSuiteDefinitionsPaginator(
Consumer listSuiteDefinitionsRequest) {
return listSuiteDefinitionsPaginator(ListSuiteDefinitionsRequest.builder().applyMutation(listSuiteDefinitionsRequest)
.build());
}
/**
*
* Lists runs of the specified Device Advisor test suite. You can list all runs of the test suite, or the runs of a
* specific version of the test suite.
*
*
* Requires permission to access the ListSuiteRuns action.
*
*
* @param listSuiteRunsRequest
* @return A Java Future containing the result of the ListSuiteRuns operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteRuns
* @see AWS API Documentation
*/
default CompletableFuture listSuiteRuns(ListSuiteRunsRequest listSuiteRunsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists runs of the specified Device Advisor test suite. You can list all runs of the test suite, or the runs of a
* specific version of the test suite.
*
*
* Requires permission to access the ListSuiteRuns action.
*
*
*
* This is a convenience which creates an instance of the {@link ListSuiteRunsRequest.Builder} avoiding the need to
* create one manually via {@link ListSuiteRunsRequest#builder()}
*
*
* @param listSuiteRunsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListSuiteRuns operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteRuns
* @see AWS API Documentation
*/
default CompletableFuture listSuiteRuns(Consumer listSuiteRunsRequest) {
return listSuiteRuns(ListSuiteRunsRequest.builder().applyMutation(listSuiteRunsRequest).build());
}
/**
*
* This is a variant of
* {@link #listSuiteRuns(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteRunsPublisher publisher = client.listSuiteRunsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteRunsPublisher publisher = client.listSuiteRunsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSuiteRuns(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest)}
* operation.
*
*
* @param listSuiteRunsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteRuns
* @see AWS API Documentation
*/
default ListSuiteRunsPublisher listSuiteRunsPaginator(ListSuiteRunsRequest listSuiteRunsRequest) {
return new ListSuiteRunsPublisher(this, listSuiteRunsRequest);
}
/**
*
* This is a variant of
* {@link #listSuiteRuns(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteRunsPublisher publisher = client.listSuiteRunsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iotdeviceadvisor.paginators.ListSuiteRunsPublisher publisher = client.listSuiteRunsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listSuiteRuns(software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListSuiteRunsRequest.Builder} avoiding the need to
* create one manually via {@link ListSuiteRunsRequest#builder()}
*
*
* @param listSuiteRunsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.ListSuiteRunsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListSuiteRuns
* @see AWS API Documentation
*/
default ListSuiteRunsPublisher listSuiteRunsPaginator(Consumer listSuiteRunsRequest) {
return listSuiteRunsPaginator(ListSuiteRunsRequest.builder().applyMutation(listSuiteRunsRequest).build());
}
/**
*
* Lists the tags attached to an IoT Device Advisor resource.
*
*
* Requires permission to access the ListTagsForResource action.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException Sends an Internal Failure exception.
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists the tags attached to an IoT Device Advisor resource.
*
*
* Requires permission to access the ListTagsForResource action.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.ListTagsForResourceRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException Sends an Internal Failure exception.
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Starts a Device Advisor test suite run.
*
*
* Requires permission to access the StartSuiteRun action.
*
*
* @param startSuiteRunRequest
* @return A Java Future containing the result of the StartSuiteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ConflictException Sends a Conflict Exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.StartSuiteRun
* @see AWS API Documentation
*/
default CompletableFuture startSuiteRun(StartSuiteRunRequest startSuiteRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Starts a Device Advisor test suite run.
*
*
* Requires permission to access the StartSuiteRun action.
*
*
*
* This is a convenience which creates an instance of the {@link StartSuiteRunRequest.Builder} avoiding the need to
* create one manually via {@link StartSuiteRunRequest#builder()}
*
*
* @param startSuiteRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.StartSuiteRunRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StartSuiteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - ConflictException Sends a Conflict Exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.StartSuiteRun
* @see AWS API Documentation
*/
default CompletableFuture startSuiteRun(Consumer startSuiteRunRequest) {
return startSuiteRun(StartSuiteRunRequest.builder().applyMutation(startSuiteRunRequest).build());
}
/**
*
* Stops a Device Advisor test suite run that is currently running.
*
*
* Requires permission to access the StopSuiteRun action.
*
*
* @param stopSuiteRunRequest
* @return A Java Future containing the result of the StopSuiteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.StopSuiteRun
* @see AWS
* API Documentation
*/
default CompletableFuture stopSuiteRun(StopSuiteRunRequest stopSuiteRunRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Stops a Device Advisor test suite run that is currently running.
*
*
* Requires permission to access the StopSuiteRun action.
*
*
*
* This is a convenience which creates an instance of the {@link StopSuiteRunRequest.Builder} avoiding the need to
* create one manually via {@link StopSuiteRunRequest#builder()}
*
*
* @param stopSuiteRunRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.StopSuiteRunRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the StopSuiteRun operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.StopSuiteRun
* @see AWS
* API Documentation
*/
default CompletableFuture stopSuiteRun(Consumer stopSuiteRunRequest) {
return stopSuiteRun(StopSuiteRunRequest.builder().applyMutation(stopSuiteRunRequest).build());
}
/**
*
* Adds to and modifies existing tags of an IoT Device Advisor resource.
*
*
* Requires permission to access the TagResource action.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException Sends an Internal Failure exception.
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds to and modifies existing tags of an IoT Device Advisor resource.
*
*
* Requires permission to access the TagResource action.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.TagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException Sends an Internal Failure exception.
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.TagResource
* @see AWS
* API Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from an IoT Device Advisor resource.
*
*
* Requires permission to access the UntagResource action.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException Sends an Internal Failure exception.
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from an IoT Device Advisor resource.
*
*
* Requires permission to access the UntagResource action.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.UntagResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - InternalServerException Sends an Internal Failure exception.
* - ValidationException Sends a validation exception.
* - ResourceNotFoundException Sends a Resource Not Found exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.UntagResource
* @see AWS API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a Device Advisor test suite.
*
*
* Requires permission to access the UpdateSuiteDefinition action.
*
*
* @param updateSuiteDefinitionRequest
* @return A Java Future containing the result of the UpdateSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.UpdateSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture updateSuiteDefinition(
UpdateSuiteDefinitionRequest updateSuiteDefinitionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a Device Advisor test suite.
*
*
* Requires permission to access the UpdateSuiteDefinition action.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSuiteDefinitionRequest.Builder} avoiding the
* need to create one manually via {@link UpdateSuiteDefinitionRequest#builder()}
*
*
* @param updateSuiteDefinitionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.iotdeviceadvisor.model.UpdateSuiteDefinitionRequest.Builder} to
* create a request.
* @return A Java Future containing the result of the UpdateSuiteDefinition operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ValidationException Sends a validation exception.
* - InternalServerException Sends an Internal Failure exception.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IotDeviceAdvisorException Base class for all service exceptions. Unknown exceptions will be thrown as
* an instance of this type.
*
* @sample IotDeviceAdvisorAsyncClient.UpdateSuiteDefinition
* @see AWS API Documentation
*/
default CompletableFuture updateSuiteDefinition(
Consumer updateSuiteDefinitionRequest) {
return updateSuiteDefinition(UpdateSuiteDefinitionRequest.builder().applyMutation(updateSuiteDefinitionRequest).build());
}
@Override
default IotDeviceAdvisorServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link IotDeviceAdvisorAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static IotDeviceAdvisorAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link IotDeviceAdvisorAsyncClient}.
*/
static IotDeviceAdvisorAsyncClientBuilder builder() {
return new DefaultIotDeviceAdvisorAsyncClientBuilder();
}
}