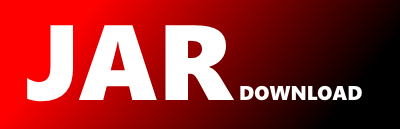
software.amazon.awssdk.services.iotdeviceadvisor.model.TestCaseScenario Maven / Gradle / Ivy
Show all versions of iotdeviceadvisor Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotdeviceadvisor.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides test case scenario.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TestCaseScenario implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TEST_CASE_SCENARIO_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("testCaseScenarioId").getter(getter(TestCaseScenario::testCaseScenarioId))
.setter(setter(Builder::testCaseScenarioId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testCaseScenarioId").build())
.build();
private static final SdkField TEST_CASE_SCENARIO_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("testCaseScenarioType").getter(getter(TestCaseScenario::testCaseScenarioTypeAsString))
.setter(setter(Builder::testCaseScenarioType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testCaseScenarioType").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(TestCaseScenario::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField FAILURE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("failure")
.getter(getter(TestCaseScenario::failure)).setter(setter(Builder::failure))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failure").build()).build();
private static final SdkField SYSTEM_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("systemMessage").getter(getter(TestCaseScenario::systemMessage)).setter(setter(Builder::systemMessage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("systemMessage").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TEST_CASE_SCENARIO_ID_FIELD,
TEST_CASE_SCENARIO_TYPE_FIELD, STATUS_FIELD, FAILURE_FIELD, SYSTEM_MESSAGE_FIELD));
private static final long serialVersionUID = 1L;
private final String testCaseScenarioId;
private final String testCaseScenarioType;
private final String status;
private final String failure;
private final String systemMessage;
private TestCaseScenario(BuilderImpl builder) {
this.testCaseScenarioId = builder.testCaseScenarioId;
this.testCaseScenarioType = builder.testCaseScenarioType;
this.status = builder.status;
this.failure = builder.failure;
this.systemMessage = builder.systemMessage;
}
/**
*
* Provides test case scenario ID.
*
*
* @return Provides test case scenario ID.
*/
public final String testCaseScenarioId() {
return testCaseScenarioId;
}
/**
*
* Provides test case scenario type. Type is one of the following:
*
*
* -
*
* Advanced
*
*
* -
*
* Basic
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #testCaseScenarioType} will return {@link TestCaseScenarioType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #testCaseScenarioTypeAsString}.
*
*
* @return Provides test case scenario type. Type is one of the following:
*
* -
*
* Advanced
*
*
* -
*
* Basic
*
*
* @see TestCaseScenarioType
*/
public final TestCaseScenarioType testCaseScenarioType() {
return TestCaseScenarioType.fromValue(testCaseScenarioType);
}
/**
*
* Provides test case scenario type. Type is one of the following:
*
*
* -
*
* Advanced
*
*
* -
*
* Basic
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #testCaseScenarioType} will return {@link TestCaseScenarioType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #testCaseScenarioTypeAsString}.
*
*
* @return Provides test case scenario type. Type is one of the following:
*
* -
*
* Advanced
*
*
* -
*
* Basic
*
*
* @see TestCaseScenarioType
*/
public final String testCaseScenarioTypeAsString() {
return testCaseScenarioType;
}
/**
*
* Provides the test case scenario status. Status is one of the following:
*
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TestCaseScenarioStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return Provides the test case scenario status. Status is one of the following:
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a
* suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
* @see TestCaseScenarioStatus
*/
public final TestCaseScenarioStatus status() {
return TestCaseScenarioStatus.fromValue(status);
}
/**
*
* Provides the test case scenario status. Status is one of the following:
*
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link TestCaseScenarioStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #statusAsString}.
*
*
* @return Provides the test case scenario status. Status is one of the following:
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a
* suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
* @see TestCaseScenarioStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* Provides test case scenario failure result.
*
*
* @return Provides test case scenario failure result.
*/
public final String failure() {
return failure;
}
/**
*
* Provides test case scenario system messages if any.
*
*
* @return Provides test case scenario system messages if any.
*/
public final String systemMessage() {
return systemMessage;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(testCaseScenarioId());
hashCode = 31 * hashCode + Objects.hashCode(testCaseScenarioTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failure());
hashCode = 31 * hashCode + Objects.hashCode(systemMessage());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TestCaseScenario)) {
return false;
}
TestCaseScenario other = (TestCaseScenario) obj;
return Objects.equals(testCaseScenarioId(), other.testCaseScenarioId())
&& Objects.equals(testCaseScenarioTypeAsString(), other.testCaseScenarioTypeAsString())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(failure(), other.failure())
&& Objects.equals(systemMessage(), other.systemMessage());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TestCaseScenario").add("TestCaseScenarioId", testCaseScenarioId())
.add("TestCaseScenarioType", testCaseScenarioTypeAsString()).add("Status", statusAsString())
.add("Failure", failure()).add("SystemMessage", systemMessage()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "testCaseScenarioId":
return Optional.ofNullable(clazz.cast(testCaseScenarioId()));
case "testCaseScenarioType":
return Optional.ofNullable(clazz.cast(testCaseScenarioTypeAsString()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "failure":
return Optional.ofNullable(clazz.cast(failure()));
case "systemMessage":
return Optional.ofNullable(clazz.cast(systemMessage()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function