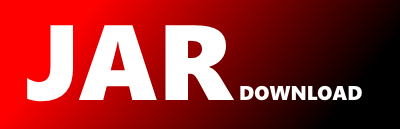
software.amazon.awssdk.services.iotdeviceadvisor.model.TestCaseRun Maven / Gradle / Ivy
Show all versions of iotdeviceadvisor Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotdeviceadvisor.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Provides the test case run.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class TestCaseRun implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TEST_CASE_RUN_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("testCaseRunId").getter(getter(TestCaseRun::testCaseRunId)).setter(setter(Builder::testCaseRunId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testCaseRunId").build()).build();
private static final SdkField TEST_CASE_DEFINITION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("testCaseDefinitionId").getter(getter(TestCaseRun::testCaseDefinitionId))
.setter(setter(Builder::testCaseDefinitionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testCaseDefinitionId").build())
.build();
private static final SdkField TEST_CASE_DEFINITION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("testCaseDefinitionName").getter(getter(TestCaseRun::testCaseDefinitionName))
.setter(setter(Builder::testCaseDefinitionName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testCaseDefinitionName").build())
.build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(TestCaseRun::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startTime").getter(getter(TestCaseRun::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("endTime").getter(getter(TestCaseRun::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final SdkField LOG_URL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("logUrl")
.getter(getter(TestCaseRun::logUrl)).setter(setter(Builder::logUrl))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("logUrl").build()).build();
private static final SdkField WARNINGS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("warnings").getter(getter(TestCaseRun::warnings)).setter(setter(Builder::warnings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("warnings").build()).build();
private static final SdkField FAILURE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("failure")
.getter(getter(TestCaseRun::failure)).setter(setter(Builder::failure))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failure").build()).build();
private static final SdkField> TEST_SCENARIOS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("testScenarios")
.getter(getter(TestCaseRun::testScenarios))
.setter(setter(Builder::testScenarios))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("testScenarios").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(TestCaseScenario::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TEST_CASE_RUN_ID_FIELD,
TEST_CASE_DEFINITION_ID_FIELD, TEST_CASE_DEFINITION_NAME_FIELD, STATUS_FIELD, START_TIME_FIELD, END_TIME_FIELD,
LOG_URL_FIELD, WARNINGS_FIELD, FAILURE_FIELD, TEST_SCENARIOS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private static final long serialVersionUID = 1L;
private final String testCaseRunId;
private final String testCaseDefinitionId;
private final String testCaseDefinitionName;
private final String status;
private final Instant startTime;
private final Instant endTime;
private final String logUrl;
private final String warnings;
private final String failure;
private final List testScenarios;
private TestCaseRun(BuilderImpl builder) {
this.testCaseRunId = builder.testCaseRunId;
this.testCaseDefinitionId = builder.testCaseDefinitionId;
this.testCaseDefinitionName = builder.testCaseDefinitionName;
this.status = builder.status;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.logUrl = builder.logUrl;
this.warnings = builder.warnings;
this.failure = builder.failure;
this.testScenarios = builder.testScenarios;
}
/**
*
* Provides the test case run ID.
*
*
* @return Provides the test case run ID.
*/
public final String testCaseRunId() {
return testCaseRunId;
}
/**
*
* Provides the test case run definition ID.
*
*
* @return Provides the test case run definition ID.
*/
public final String testCaseDefinitionId() {
return testCaseDefinitionId;
}
/**
*
* Provides the test case run definition name.
*
*
* @return Provides the test case run definition name.
*/
public final String testCaseDefinitionName() {
return testCaseDefinitionName;
}
/**
*
* Provides the test case run status. Status is one of the following:
*
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Provides the test case run status. Status is one of the following:
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a
* suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
* @see Status
*/
public final Status status() {
return Status.fromValue(status);
}
/**
*
* Provides the test case run status. Status is one of the following:
*
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return Provides the test case run status. Status is one of the following:
*
* -
*
* PASS
: Test passed.
*
*
* -
*
* FAIL
: Test failed.
*
*
* -
*
* PENDING
: Test has not started running but is scheduled.
*
*
* -
*
* RUNNING
: Test is running.
*
*
* -
*
* STOPPING
: Test is performing cleanup steps. You will see this status only if you stop a
* suite run.
*
*
* -
*
* STOPPED
Test is stopped. You will see this status only if you stop a suite run.
*
*
* -
*
* PASS_WITH_WARNINGS
: Test passed with warnings.
*
*
* -
*
* ERORR
: Test faced an error when running due to an internal issue.
*
*
* @see Status
*/
public final String statusAsString() {
return status;
}
/**
*
* Provides test case run start time.
*
*
* @return Provides test case run start time.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* Provides test case run end time.
*
*
* @return Provides test case run end time.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* Provides test case run log URL.
*
*
* @return Provides test case run log URL.
*/
public final String logUrl() {
return logUrl;
}
/**
*
* Provides test case run warnings.
*
*
* @return Provides test case run warnings.
*/
public final String warnings() {
return warnings;
}
/**
*
* Provides test case run failure result.
*
*
* @return Provides test case run failure result.
*/
public final String failure() {
return failure;
}
/**
* For responses, this returns true if the service returned a value for the TestScenarios property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTestScenarios() {
return testScenarios != null && !(testScenarios instanceof SdkAutoConstructList);
}
/**
*
* Provides the test scenarios for the test case run.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTestScenarios} method.
*
*
* @return Provides the test scenarios for the test case run.
*/
public final List testScenarios() {
return testScenarios;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(testCaseRunId());
hashCode = 31 * hashCode + Objects.hashCode(testCaseDefinitionId());
hashCode = 31 * hashCode + Objects.hashCode(testCaseDefinitionName());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(logUrl());
hashCode = 31 * hashCode + Objects.hashCode(warnings());
hashCode = 31 * hashCode + Objects.hashCode(failure());
hashCode = 31 * hashCode + Objects.hashCode(hasTestScenarios() ? testScenarios() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof TestCaseRun)) {
return false;
}
TestCaseRun other = (TestCaseRun) obj;
return Objects.equals(testCaseRunId(), other.testCaseRunId())
&& Objects.equals(testCaseDefinitionId(), other.testCaseDefinitionId())
&& Objects.equals(testCaseDefinitionName(), other.testCaseDefinitionName())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(logUrl(), other.logUrl())
&& Objects.equals(warnings(), other.warnings()) && Objects.equals(failure(), other.failure())
&& hasTestScenarios() == other.hasTestScenarios() && Objects.equals(testScenarios(), other.testScenarios());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("TestCaseRun").add("TestCaseRunId", testCaseRunId())
.add("TestCaseDefinitionId", testCaseDefinitionId()).add("TestCaseDefinitionName", testCaseDefinitionName())
.add("Status", statusAsString()).add("StartTime", startTime()).add("EndTime", endTime()).add("LogUrl", logUrl())
.add("Warnings", warnings()).add("Failure", failure())
.add("TestScenarios", hasTestScenarios() ? testScenarios() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "testCaseRunId":
return Optional.ofNullable(clazz.cast(testCaseRunId()));
case "testCaseDefinitionId":
return Optional.ofNullable(clazz.cast(testCaseDefinitionId()));
case "testCaseDefinitionName":
return Optional.ofNullable(clazz.cast(testCaseDefinitionName()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "logUrl":
return Optional.ofNullable(clazz.cast(logUrl()));
case "warnings":
return Optional.ofNullable(clazz.cast(warnings()));
case "failure":
return Optional.ofNullable(clazz.cast(failure()));
case "testScenarios":
return Optional.ofNullable(clazz.cast(testScenarios()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("testCaseRunId", TEST_CASE_RUN_ID_FIELD);
map.put("testCaseDefinitionId", TEST_CASE_DEFINITION_ID_FIELD);
map.put("testCaseDefinitionName", TEST_CASE_DEFINITION_NAME_FIELD);
map.put("status", STATUS_FIELD);
map.put("startTime", START_TIME_FIELD);
map.put("endTime", END_TIME_FIELD);
map.put("logUrl", LOG_URL_FIELD);
map.put("warnings", WARNINGS_FIELD);
map.put("failure", FAILURE_FIELD);
map.put("testScenarios", TEST_SCENARIOS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function