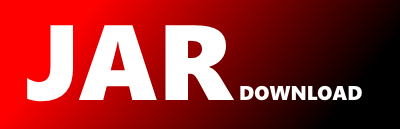
software.amazon.awssdk.services.iotfleetwise.model.SignalDecoder Maven / Gradle / Ivy
Show all versions of iotfleetwise Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotfleetwise.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about a signal decoder.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class SignalDecoder implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField FULLY_QUALIFIED_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("fullyQualifiedName").getter(getter(SignalDecoder::fullyQualifiedName))
.setter(setter(Builder::fullyQualifiedName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fullyQualifiedName").build())
.build();
private static final SdkField TYPE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("type")
.getter(getter(SignalDecoder::typeAsString)).setter(setter(Builder::type))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("type").build()).build();
private static final SdkField INTERFACE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("interfaceId").getter(getter(SignalDecoder::interfaceId)).setter(setter(Builder::interfaceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interfaceId").build()).build();
private static final SdkField CAN_SIGNAL_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("canSignal").getter(getter(SignalDecoder::canSignal)).setter(setter(Builder::canSignal))
.constructor(CanSignal::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("canSignal").build()).build();
private static final SdkField OBD_SIGNAL_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("obdSignal").getter(getter(SignalDecoder::obdSignal)).setter(setter(Builder::obdSignal))
.constructor(ObdSignal::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("obdSignal").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FULLY_QUALIFIED_NAME_FIELD,
TYPE_FIELD, INTERFACE_ID_FIELD, CAN_SIGNAL_FIELD, OBD_SIGNAL_FIELD));
private static final long serialVersionUID = 1L;
private final String fullyQualifiedName;
private final String type;
private final String interfaceId;
private final CanSignal canSignal;
private final ObdSignal obdSignal;
private SignalDecoder(BuilderImpl builder) {
this.fullyQualifiedName = builder.fullyQualifiedName;
this.type = builder.type;
this.interfaceId = builder.interfaceId;
this.canSignal = builder.canSignal;
this.obdSignal = builder.obdSignal;
}
/**
*
* The fully qualified name of a signal decoder as defined in a vehicle model.
*
*
* @return The fully qualified name of a signal decoder as defined in a vehicle model.
*/
public final String fullyQualifiedName() {
return fullyQualifiedName;
}
/**
*
* The network protocol for the vehicle. For example, CAN_SIGNAL
specifies a protocol that defines how
* data is communicated between electronic control units (ECUs). OBD_SIGNAL
specifies a protocol that
* defines how self-diagnostic data is communicated between ECUs.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SignalDecoderType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The network protocol for the vehicle. For example, CAN_SIGNAL
specifies a protocol that
* defines how data is communicated between electronic control units (ECUs). OBD_SIGNAL
* specifies a protocol that defines how self-diagnostic data is communicated between ECUs.
* @see SignalDecoderType
*/
public final SignalDecoderType type() {
return SignalDecoderType.fromValue(type);
}
/**
*
* The network protocol for the vehicle. For example, CAN_SIGNAL
specifies a protocol that defines how
* data is communicated between electronic control units (ECUs). OBD_SIGNAL
specifies a protocol that
* defines how self-diagnostic data is communicated between ECUs.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #type} will return
* {@link SignalDecoderType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #typeAsString}.
*
*
* @return The network protocol for the vehicle. For example, CAN_SIGNAL
specifies a protocol that
* defines how data is communicated between electronic control units (ECUs). OBD_SIGNAL
* specifies a protocol that defines how self-diagnostic data is communicated between ECUs.
* @see SignalDecoderType
*/
public final String typeAsString() {
return type;
}
/**
*
* The ID of a network interface that specifies what network protocol a vehicle follows.
*
*
* @return The ID of a network interface that specifies what network protocol a vehicle follows.
*/
public final String interfaceId() {
return interfaceId;
}
/**
*
* Information about signal decoder using the Controller Area Network (CAN) protocol.
*
*
* @return Information about signal decoder using the Controller Area Network (CAN) protocol.
*/
public final CanSignal canSignal() {
return canSignal;
}
/**
*
* Information about signal decoder using the On-board diagnostic (OBD) II protocol.
*
*
* @return Information about signal decoder using the On-board diagnostic (OBD) II protocol.
*/
public final ObdSignal obdSignal() {
return obdSignal;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(fullyQualifiedName());
hashCode = 31 * hashCode + Objects.hashCode(typeAsString());
hashCode = 31 * hashCode + Objects.hashCode(interfaceId());
hashCode = 31 * hashCode + Objects.hashCode(canSignal());
hashCode = 31 * hashCode + Objects.hashCode(obdSignal());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SignalDecoder)) {
return false;
}
SignalDecoder other = (SignalDecoder) obj;
return Objects.equals(fullyQualifiedName(), other.fullyQualifiedName())
&& Objects.equals(typeAsString(), other.typeAsString()) && Objects.equals(interfaceId(), other.interfaceId())
&& Objects.equals(canSignal(), other.canSignal()) && Objects.equals(obdSignal(), other.obdSignal());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("SignalDecoder").add("FullyQualifiedName", fullyQualifiedName()).add("Type", typeAsString())
.add("InterfaceId", interfaceId()).add("CanSignal", canSignal()).add("ObdSignal", obdSignal()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "fullyQualifiedName":
return Optional.ofNullable(clazz.cast(fullyQualifiedName()));
case "type":
return Optional.ofNullable(clazz.cast(typeAsString()));
case "interfaceId":
return Optional.ofNullable(clazz.cast(interfaceId()));
case "canSignal":
return Optional.ofNullable(clazz.cast(canSignal()));
case "obdSignal":
return Optional.ofNullable(clazz.cast(obdSignal()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function