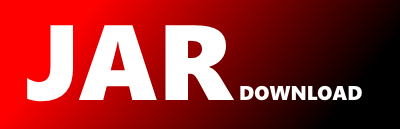
software.amazon.awssdk.services.iotfleetwise.model.GetCampaignResponse Maven / Gradle / Ivy
Show all versions of iotfleetwise Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotfleetwise.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetCampaignResponse extends IoTFleetWiseResponse implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(GetCampaignResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("arn")
.getter(getter(GetCampaignResponse::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("arn").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("description").getter(getter(GetCampaignResponse::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField SIGNAL_CATALOG_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("signalCatalogArn").getter(getter(GetCampaignResponse::signalCatalogArn))
.setter(setter(Builder::signalCatalogArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("signalCatalogArn").build()).build();
private static final SdkField TARGET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("targetArn").getter(getter(GetCampaignResponse::targetArn)).setter(setter(Builder::targetArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("targetArn").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("status")
.getter(getter(GetCampaignResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startTime").getter(getter(GetCampaignResponse::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField EXPIRY_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("expiryTime").getter(getter(GetCampaignResponse::expiryTime)).setter(setter(Builder::expiryTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("expiryTime").build()).build();
private static final SdkField POST_TRIGGER_COLLECTION_DURATION_FIELD = SdkField
. builder(MarshallingType.LONG)
.memberName("postTriggerCollectionDuration")
.getter(getter(GetCampaignResponse::postTriggerCollectionDuration))
.setter(setter(Builder::postTriggerCollectionDuration))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("postTriggerCollectionDuration")
.build()).build();
private static final SdkField DIAGNOSTICS_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("diagnosticsMode").getter(getter(GetCampaignResponse::diagnosticsModeAsString))
.setter(setter(Builder::diagnosticsMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("diagnosticsMode").build()).build();
private static final SdkField SPOOLING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("spoolingMode").getter(getter(GetCampaignResponse::spoolingModeAsString))
.setter(setter(Builder::spoolingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("spoolingMode").build()).build();
private static final SdkField COMPRESSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("compression").getter(getter(GetCampaignResponse::compressionAsString))
.setter(setter(Builder::compression))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("compression").build()).build();
private static final SdkField PRIORITY_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("priority").getter(getter(GetCampaignResponse::priority)).setter(setter(Builder::priority))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("priority").build()).build();
private static final SdkField> SIGNALS_TO_COLLECT_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("signalsToCollect")
.getter(getter(GetCampaignResponse::signalsToCollect))
.setter(setter(Builder::signalsToCollect))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("signalsToCollect").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(SignalInformation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField COLLECTION_SCHEME_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("collectionScheme")
.getter(getter(GetCampaignResponse::collectionScheme)).setter(setter(Builder::collectionScheme))
.constructor(CollectionScheme::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("collectionScheme").build()).build();
private static final SdkField> DATA_EXTRA_DIMENSIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("dataExtraDimensions")
.getter(getter(GetCampaignResponse::dataExtraDimensions))
.setter(setter(Builder::dataExtraDimensions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataExtraDimensions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("creationTime").getter(getter(GetCampaignResponse::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("creationTime").build()).build();
private static final SdkField LAST_MODIFICATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("lastModificationTime").getter(getter(GetCampaignResponse::lastModificationTime))
.setter(setter(Builder::lastModificationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastModificationTime").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, ARN_FIELD,
DESCRIPTION_FIELD, SIGNAL_CATALOG_ARN_FIELD, TARGET_ARN_FIELD, STATUS_FIELD, START_TIME_FIELD, EXPIRY_TIME_FIELD,
POST_TRIGGER_COLLECTION_DURATION_FIELD, DIAGNOSTICS_MODE_FIELD, SPOOLING_MODE_FIELD, COMPRESSION_FIELD,
PRIORITY_FIELD, SIGNALS_TO_COLLECT_FIELD, COLLECTION_SCHEME_FIELD, DATA_EXTRA_DIMENSIONS_FIELD, CREATION_TIME_FIELD,
LAST_MODIFICATION_TIME_FIELD));
private final String name;
private final String arn;
private final String description;
private final String signalCatalogArn;
private final String targetArn;
private final String status;
private final Instant startTime;
private final Instant expiryTime;
private final Long postTriggerCollectionDuration;
private final String diagnosticsMode;
private final String spoolingMode;
private final String compression;
private final Integer priority;
private final List signalsToCollect;
private final CollectionScheme collectionScheme;
private final List dataExtraDimensions;
private final Instant creationTime;
private final Instant lastModificationTime;
private GetCampaignResponse(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.arn = builder.arn;
this.description = builder.description;
this.signalCatalogArn = builder.signalCatalogArn;
this.targetArn = builder.targetArn;
this.status = builder.status;
this.startTime = builder.startTime;
this.expiryTime = builder.expiryTime;
this.postTriggerCollectionDuration = builder.postTriggerCollectionDuration;
this.diagnosticsMode = builder.diagnosticsMode;
this.spoolingMode = builder.spoolingMode;
this.compression = builder.compression;
this.priority = builder.priority;
this.signalsToCollect = builder.signalsToCollect;
this.collectionScheme = builder.collectionScheme;
this.dataExtraDimensions = builder.dataExtraDimensions;
this.creationTime = builder.creationTime;
this.lastModificationTime = builder.lastModificationTime;
}
/**
*
* The name of the campaign.
*
*
* @return The name of the campaign.
*/
public final String name() {
return name;
}
/**
*
* The Amazon Resource Name (ARN) of the campaign.
*
*
* @return The Amazon Resource Name (ARN) of the campaign.
*/
public final String arn() {
return arn;
}
/**
*
* The description of the campaign.
*
*
* @return The description of the campaign.
*/
public final String description() {
return description;
}
/**
*
* The ARN of a signal catalog.
*
*
* @return The ARN of a signal catalog.
*/
public final String signalCatalogArn() {
return signalCatalogArn;
}
/**
*
* The ARN of the vehicle or the fleet targeted by the campaign.
*
*
* @return The ARN of the vehicle or the fleet targeted by the campaign.
*/
public final String targetArn() {
return targetArn;
}
/**
*
* The state of the campaign. The status can be one of: CREATING
, WAITING_FOR_APPROVAL
,
* RUNNING
, and SUSPENDED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link CampaignStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The state of the campaign. The status can be one of: CREATING
,
* WAITING_FOR_APPROVAL
, RUNNING
, and SUSPENDED
.
* @see CampaignStatus
*/
public final CampaignStatus status() {
return CampaignStatus.fromValue(status);
}
/**
*
* The state of the campaign. The status can be one of: CREATING
, WAITING_FOR_APPROVAL
,
* RUNNING
, and SUSPENDED
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link CampaignStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The state of the campaign. The status can be one of: CREATING
,
* WAITING_FOR_APPROVAL
, RUNNING
, and SUSPENDED
.
* @see CampaignStatus
*/
public final String statusAsString() {
return status;
}
/**
*
* The time, in milliseconds, to deliver a campaign after it was approved.
*
*
* @return The time, in milliseconds, to deliver a campaign after it was approved.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* The time the campaign expires, in seconds since epoch (January 1, 1970 at midnight UTC time). Vehicle data won't
* be collected after the campaign expires.
*
*
* @return The time the campaign expires, in seconds since epoch (January 1, 1970 at midnight UTC time). Vehicle
* data won't be collected after the campaign expires.
*/
public final Instant expiryTime() {
return expiryTime;
}
/**
*
* How long (in seconds) to collect raw data after a triggering event initiates the collection.
*
*
* @return How long (in seconds) to collect raw data after a triggering event initiates the collection.
*/
public final Long postTriggerCollectionDuration() {
return postTriggerCollectionDuration;
}
/**
*
* Option for a vehicle to send diagnostic trouble codes to Amazon Web Services IoT FleetWise.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #diagnosticsMode}
* will return {@link DiagnosticsMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #diagnosticsModeAsString}.
*
*
* @return Option for a vehicle to send diagnostic trouble codes to Amazon Web Services IoT FleetWise.
* @see DiagnosticsMode
*/
public final DiagnosticsMode diagnosticsMode() {
return DiagnosticsMode.fromValue(diagnosticsMode);
}
/**
*
* Option for a vehicle to send diagnostic trouble codes to Amazon Web Services IoT FleetWise.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #diagnosticsMode}
* will return {@link DiagnosticsMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #diagnosticsModeAsString}.
*
*
* @return Option for a vehicle to send diagnostic trouble codes to Amazon Web Services IoT FleetWise.
* @see DiagnosticsMode
*/
public final String diagnosticsModeAsString() {
return diagnosticsMode;
}
/**
*
* Whether to store collected data after a vehicle lost a connection with the cloud. After a connection is
* re-established, the data is automatically forwarded to Amazon Web Services IoT FleetWise.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #spoolingMode} will
* return {@link SpoolingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #spoolingModeAsString}.
*
*
* @return Whether to store collected data after a vehicle lost a connection with the cloud. After a connection is
* re-established, the data is automatically forwarded to Amazon Web Services IoT FleetWise.
* @see SpoolingMode
*/
public final SpoolingMode spoolingMode() {
return SpoolingMode.fromValue(spoolingMode);
}
/**
*
* Whether to store collected data after a vehicle lost a connection with the cloud. After a connection is
* re-established, the data is automatically forwarded to Amazon Web Services IoT FleetWise.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #spoolingMode} will
* return {@link SpoolingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #spoolingModeAsString}.
*
*
* @return Whether to store collected data after a vehicle lost a connection with the cloud. After a connection is
* re-established, the data is automatically forwarded to Amazon Web Services IoT FleetWise.
* @see SpoolingMode
*/
public final String spoolingModeAsString() {
return spoolingMode;
}
/**
*
* Whether to compress signals before transmitting data to Amazon Web Services IoT FleetWise. If OFF
is
* specified, the signals aren't compressed. If it's not specified, SNAPPY
is used.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compression} will
* return {@link Compression#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compressionAsString}.
*
*
* @return Whether to compress signals before transmitting data to Amazon Web Services IoT FleetWise. If
* OFF
is specified, the signals aren't compressed. If it's not specified, SNAPPY
* is used.
* @see Compression
*/
public final Compression compression() {
return Compression.fromValue(compression);
}
/**
*
* Whether to compress signals before transmitting data to Amazon Web Services IoT FleetWise. If OFF
is
* specified, the signals aren't compressed. If it's not specified, SNAPPY
is used.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compression} will
* return {@link Compression#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compressionAsString}.
*
*
* @return Whether to compress signals before transmitting data to Amazon Web Services IoT FleetWise. If
* OFF
is specified, the signals aren't compressed. If it's not specified, SNAPPY
* is used.
* @see Compression
*/
public final String compressionAsString() {
return compression;
}
/**
*
* A number indicating the priority of one campaign over another campaign for a certain vehicle or fleet. A campaign
* with the lowest value is deployed to vehicles before any other campaigns.
*
*
* @return A number indicating the priority of one campaign over another campaign for a certain vehicle or fleet. A
* campaign with the lowest value is deployed to vehicles before any other campaigns.
*/
public final Integer priority() {
return priority;
}
/**
* For responses, this returns true if the service returned a value for the SignalsToCollect property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSignalsToCollect() {
return signalsToCollect != null && !(signalsToCollect instanceof SdkAutoConstructList);
}
/**
*
* Information about a list of signals to collect data on.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSignalsToCollect} method.
*
*
* @return Information about a list of signals to collect data on.
*/
public final List signalsToCollect() {
return signalsToCollect;
}
/**
*
* Information about the data collection scheme associated with the campaign.
*
*
* @return Information about the data collection scheme associated with the campaign.
*/
public final CollectionScheme collectionScheme() {
return collectionScheme;
}
/**
* For responses, this returns true if the service returned a value for the DataExtraDimensions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDataExtraDimensions() {
return dataExtraDimensions != null && !(dataExtraDimensions instanceof SdkAutoConstructList);
}
/**
*
* A list of vehicle attributes associated with the campaign.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDataExtraDimensions} method.
*
*
* @return A list of vehicle attributes associated with the campaign.
*/
public final List dataExtraDimensions() {
return dataExtraDimensions;
}
/**
*
* The time the campaign was created in seconds since epoch (January 1, 1970 at midnight UTC time).
*
*
* @return The time the campaign was created in seconds since epoch (January 1, 1970 at midnight UTC time).
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* The last time the campaign was modified.
*
*
* @return The last time the campaign was modified.
*/
public final Instant lastModificationTime() {
return lastModificationTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(signalCatalogArn());
hashCode = 31 * hashCode + Objects.hashCode(targetArn());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(expiryTime());
hashCode = 31 * hashCode + Objects.hashCode(postTriggerCollectionDuration());
hashCode = 31 * hashCode + Objects.hashCode(diagnosticsModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(spoolingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(compressionAsString());
hashCode = 31 * hashCode + Objects.hashCode(priority());
hashCode = 31 * hashCode + Objects.hashCode(hasSignalsToCollect() ? signalsToCollect() : null);
hashCode = 31 * hashCode + Objects.hashCode(collectionScheme());
hashCode = 31 * hashCode + Objects.hashCode(hasDataExtraDimensions() ? dataExtraDimensions() : null);
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(lastModificationTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetCampaignResponse)) {
return false;
}
GetCampaignResponse other = (GetCampaignResponse) obj;
return Objects.equals(name(), other.name()) && Objects.equals(arn(), other.arn())
&& Objects.equals(description(), other.description())
&& Objects.equals(signalCatalogArn(), other.signalCatalogArn()) && Objects.equals(targetArn(), other.targetArn())
&& Objects.equals(statusAsString(), other.statusAsString()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(expiryTime(), other.expiryTime())
&& Objects.equals(postTriggerCollectionDuration(), other.postTriggerCollectionDuration())
&& Objects.equals(diagnosticsModeAsString(), other.diagnosticsModeAsString())
&& Objects.equals(spoolingModeAsString(), other.spoolingModeAsString())
&& Objects.equals(compressionAsString(), other.compressionAsString())
&& Objects.equals(priority(), other.priority()) && hasSignalsToCollect() == other.hasSignalsToCollect()
&& Objects.equals(signalsToCollect(), other.signalsToCollect())
&& Objects.equals(collectionScheme(), other.collectionScheme())
&& hasDataExtraDimensions() == other.hasDataExtraDimensions()
&& Objects.equals(dataExtraDimensions(), other.dataExtraDimensions())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(lastModificationTime(), other.lastModificationTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetCampaignResponse").add("Name", name()).add("Arn", arn()).add("Description", description())
.add("SignalCatalogArn", signalCatalogArn()).add("TargetArn", targetArn()).add("Status", statusAsString())
.add("StartTime", startTime()).add("ExpiryTime", expiryTime())
.add("PostTriggerCollectionDuration", postTriggerCollectionDuration())
.add("DiagnosticsMode", diagnosticsModeAsString()).add("SpoolingMode", spoolingModeAsString())
.add("Compression", compressionAsString()).add("Priority", priority())
.add("SignalsToCollect", hasSignalsToCollect() ? signalsToCollect() : null)
.add("CollectionScheme", collectionScheme())
.add("DataExtraDimensions", hasDataExtraDimensions() ? dataExtraDimensions() : null)
.add("CreationTime", creationTime()).add("LastModificationTime", lastModificationTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "arn":
return Optional.ofNullable(clazz.cast(arn()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "signalCatalogArn":
return Optional.ofNullable(clazz.cast(signalCatalogArn()));
case "targetArn":
return Optional.ofNullable(clazz.cast(targetArn()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "expiryTime":
return Optional.ofNullable(clazz.cast(expiryTime()));
case "postTriggerCollectionDuration":
return Optional.ofNullable(clazz.cast(postTriggerCollectionDuration()));
case "diagnosticsMode":
return Optional.ofNullable(clazz.cast(diagnosticsModeAsString()));
case "spoolingMode":
return Optional.ofNullable(clazz.cast(spoolingModeAsString()));
case "compression":
return Optional.ofNullable(clazz.cast(compressionAsString()));
case "priority":
return Optional.ofNullable(clazz.cast(priority()));
case "signalsToCollect":
return Optional.ofNullable(clazz.cast(signalsToCollect()));
case "collectionScheme":
return Optional.ofNullable(clazz.cast(collectionScheme()));
case "dataExtraDimensions":
return Optional.ofNullable(clazz.cast(dataExtraDimensions()));
case "creationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "lastModificationTime":
return Optional.ofNullable(clazz.cast(lastModificationTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function