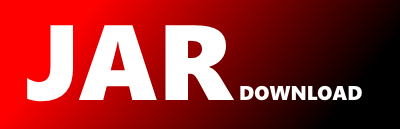
software.amazon.awssdk.services.iotfleetwise.model.NodeCounts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iotfleetwise Show documentation
Show all versions of iotfleetwise Show documentation
The AWS Java SDK for Io T Fleet Wise module holds the client classes that are used for
communicating with Io T Fleet Wise.
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotfleetwise.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Information about the number of nodes and node types in a vehicle network.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class NodeCounts implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField TOTAL_NODES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalNodes").getter(getter(NodeCounts::totalNodes)).setter(setter(Builder::totalNodes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalNodes").build()).build();
private static final SdkField TOTAL_BRANCHES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalBranches").getter(getter(NodeCounts::totalBranches)).setter(setter(Builder::totalBranches))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalBranches").build()).build();
private static final SdkField TOTAL_SENSORS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalSensors").getter(getter(NodeCounts::totalSensors)).setter(setter(Builder::totalSensors))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalSensors").build()).build();
private static final SdkField TOTAL_ATTRIBUTES_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalAttributes").getter(getter(NodeCounts::totalAttributes)).setter(setter(Builder::totalAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalAttributes").build()).build();
private static final SdkField TOTAL_ACTUATORS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalActuators").getter(getter(NodeCounts::totalActuators)).setter(setter(Builder::totalActuators))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalActuators").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TOTAL_NODES_FIELD,
TOTAL_BRANCHES_FIELD, TOTAL_SENSORS_FIELD, TOTAL_ATTRIBUTES_FIELD, TOTAL_ACTUATORS_FIELD));
private static final long serialVersionUID = 1L;
private final Integer totalNodes;
private final Integer totalBranches;
private final Integer totalSensors;
private final Integer totalAttributes;
private final Integer totalActuators;
private NodeCounts(BuilderImpl builder) {
this.totalNodes = builder.totalNodes;
this.totalBranches = builder.totalBranches;
this.totalSensors = builder.totalSensors;
this.totalAttributes = builder.totalAttributes;
this.totalActuators = builder.totalActuators;
}
/**
*
* The total number of nodes in a vehicle network.
*
*
* @return The total number of nodes in a vehicle network.
*/
public final Integer totalNodes() {
return totalNodes;
}
/**
*
* The total number of nodes in a vehicle network that represent branches.
*
*
* @return The total number of nodes in a vehicle network that represent branches.
*/
public final Integer totalBranches() {
return totalBranches;
}
/**
*
* The total number of nodes in a vehicle network that represent sensors.
*
*
* @return The total number of nodes in a vehicle network that represent sensors.
*/
public final Integer totalSensors() {
return totalSensors;
}
/**
*
* The total number of nodes in a vehicle network that represent attributes.
*
*
* @return The total number of nodes in a vehicle network that represent attributes.
*/
public final Integer totalAttributes() {
return totalAttributes;
}
/**
*
* The total number of nodes in a vehicle network that represent actuators.
*
*
* @return The total number of nodes in a vehicle network that represent actuators.
*/
public final Integer totalActuators() {
return totalActuators;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(totalNodes());
hashCode = 31 * hashCode + Objects.hashCode(totalBranches());
hashCode = 31 * hashCode + Objects.hashCode(totalSensors());
hashCode = 31 * hashCode + Objects.hashCode(totalAttributes());
hashCode = 31 * hashCode + Objects.hashCode(totalActuators());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof NodeCounts)) {
return false;
}
NodeCounts other = (NodeCounts) obj;
return Objects.equals(totalNodes(), other.totalNodes()) && Objects.equals(totalBranches(), other.totalBranches())
&& Objects.equals(totalSensors(), other.totalSensors())
&& Objects.equals(totalAttributes(), other.totalAttributes())
&& Objects.equals(totalActuators(), other.totalActuators());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("NodeCounts").add("TotalNodes", totalNodes()).add("TotalBranches", totalBranches())
.add("TotalSensors", totalSensors()).add("TotalAttributes", totalAttributes())
.add("TotalActuators", totalActuators()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "totalNodes":
return Optional.ofNullable(clazz.cast(totalNodes()));
case "totalBranches":
return Optional.ofNullable(clazz.cast(totalBranches()));
case "totalSensors":
return Optional.ofNullable(clazz.cast(totalSensors()));
case "totalAttributes":
return Optional.ofNullable(clazz.cast(totalAttributes()));
case "totalActuators":
return Optional.ofNullable(clazz.cast(totalActuators()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy