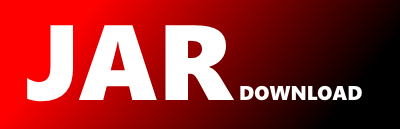
software.amazon.awssdk.services.iotfleetwise.DefaultIoTFleetWiseClient Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotfleetwise;
import java.util.Collections;
import java.util.List;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsSyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.client.handler.SyncClientHandler;
import software.amazon.awssdk.core.exception.SdkClientException;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.services.iotfleetwise.model.AccessDeniedException;
import software.amazon.awssdk.services.iotfleetwise.model.AssociateVehicleFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.AssociateVehicleFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.BatchCreateVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.BatchCreateVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.BatchUpdateVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.BatchUpdateVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ConflictException;
import software.amazon.awssdk.services.iotfleetwise.model.CreateCampaignRequest;
import software.amazon.awssdk.services.iotfleetwise.model.CreateCampaignResponse;
import software.amazon.awssdk.services.iotfleetwise.model.CreateDecoderManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.CreateDecoderManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.CreateFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.CreateFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.CreateModelManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.CreateModelManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.CreateSignalCatalogRequest;
import software.amazon.awssdk.services.iotfleetwise.model.CreateSignalCatalogResponse;
import software.amazon.awssdk.services.iotfleetwise.model.CreateVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.CreateVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DecoderManifestValidationException;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteCampaignRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteCampaignResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteDecoderManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteDecoderManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteModelManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteModelManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteSignalCatalogRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteSignalCatalogResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DeleteVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.DisassociateVehicleFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.DisassociateVehicleFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetCampaignRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetCampaignResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetDecoderManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetDecoderManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetLoggingOptionsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetLoggingOptionsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetModelManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetModelManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetRegisterAccountStatusRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetRegisterAccountStatusResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetSignalCatalogRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetSignalCatalogResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.GetVehicleStatusRequest;
import software.amazon.awssdk.services.iotfleetwise.model.GetVehicleStatusResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ImportDecoderManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ImportDecoderManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ImportSignalCatalogRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ImportSignalCatalogResponse;
import software.amazon.awssdk.services.iotfleetwise.model.InternalServerException;
import software.amazon.awssdk.services.iotfleetwise.model.InvalidNodeException;
import software.amazon.awssdk.services.iotfleetwise.model.InvalidSignalsException;
import software.amazon.awssdk.services.iotfleetwise.model.IoTFleetWiseException;
import software.amazon.awssdk.services.iotfleetwise.model.LimitExceededException;
import software.amazon.awssdk.services.iotfleetwise.model.ListCampaignsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListCampaignsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListDecoderManifestNetworkInterfacesRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListDecoderManifestNetworkInterfacesResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListDecoderManifestSignalsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListDecoderManifestSignalsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListDecoderManifestsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListDecoderManifestsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListFleetsForVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListFleetsForVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListFleetsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListFleetsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListModelManifestNodesRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListModelManifestNodesResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListModelManifestsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListModelManifestsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListSignalCatalogNodesRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListSignalCatalogNodesResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListSignalCatalogsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListSignalCatalogsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListVehiclesInFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListVehiclesInFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ListVehiclesRequest;
import software.amazon.awssdk.services.iotfleetwise.model.ListVehiclesResponse;
import software.amazon.awssdk.services.iotfleetwise.model.PutLoggingOptionsRequest;
import software.amazon.awssdk.services.iotfleetwise.model.PutLoggingOptionsResponse;
import software.amazon.awssdk.services.iotfleetwise.model.RegisterAccountRequest;
import software.amazon.awssdk.services.iotfleetwise.model.RegisterAccountResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ResourceNotFoundException;
import software.amazon.awssdk.services.iotfleetwise.model.TagResourceRequest;
import software.amazon.awssdk.services.iotfleetwise.model.TagResourceResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ThrottlingException;
import software.amazon.awssdk.services.iotfleetwise.model.UntagResourceRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UntagResourceResponse;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateCampaignRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateCampaignResponse;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateDecoderManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateDecoderManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateFleetRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateFleetResponse;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateModelManifestRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateModelManifestResponse;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateSignalCatalogRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateSignalCatalogResponse;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateVehicleRequest;
import software.amazon.awssdk.services.iotfleetwise.model.UpdateVehicleResponse;
import software.amazon.awssdk.services.iotfleetwise.model.ValidationException;
import software.amazon.awssdk.services.iotfleetwise.transform.AssociateVehicleFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.BatchCreateVehicleRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.BatchUpdateVehicleRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.CreateCampaignRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.CreateDecoderManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.CreateFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.CreateModelManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.CreateSignalCatalogRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.CreateVehicleRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DeleteCampaignRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DeleteDecoderManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DeleteFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DeleteModelManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DeleteSignalCatalogRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DeleteVehicleRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.DisassociateVehicleFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetCampaignRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetDecoderManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetLoggingOptionsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetModelManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetRegisterAccountStatusRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetSignalCatalogRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetVehicleRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.GetVehicleStatusRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ImportDecoderManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ImportSignalCatalogRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListCampaignsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListDecoderManifestNetworkInterfacesRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListDecoderManifestSignalsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListDecoderManifestsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListFleetsForVehicleRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListFleetsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListModelManifestNodesRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListModelManifestsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListSignalCatalogNodesRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListSignalCatalogsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListVehiclesInFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.ListVehiclesRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.PutLoggingOptionsRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.RegisterAccountRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UpdateCampaignRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UpdateDecoderManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UpdateFleetRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UpdateModelManifestRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UpdateSignalCatalogRequestMarshaller;
import software.amazon.awssdk.services.iotfleetwise.transform.UpdateVehicleRequestMarshaller;
import software.amazon.awssdk.utils.Logger;
/**
* Internal implementation of {@link IoTFleetWiseClient}.
*
* @see IoTFleetWiseClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultIoTFleetWiseClient implements IoTFleetWiseClient {
private static final Logger log = Logger.loggerFor(DefaultIoTFleetWiseClient.class);
private final SyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
private final IoTFleetWiseServiceClientConfiguration serviceClientConfiguration;
protected DefaultIoTFleetWiseClient(IoTFleetWiseServiceClientConfiguration serviceClientConfiguration,
SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsSyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration;
this.serviceClientConfiguration = serviceClientConfiguration;
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Adds, or associates, a vehicle with a fleet.
*
*
* @param associateVehicleFleetRequest
* @return Result of the AssociateVehicleFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.AssociateVehicleFleet
* @see AWS API Documentation
*/
@Override
public AssociateVehicleFleetResponse associateVehicleFleet(AssociateVehicleFleetRequest associateVehicleFleetRequest)
throws InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, AssociateVehicleFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, associateVehicleFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "AssociateVehicleFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("AssociateVehicleFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(associateVehicleFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new AssociateVehicleFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a group, or batch, of vehicles.
*
*
*
* You must specify a decoder manifest and a vehicle model (model manifest) for each vehicle.
*
*
*
* For more information, see Create multiple
* vehicles (AWS CLI) in the Amazon Web Services IoT FleetWise Developer Guide.
*
*
* @param batchCreateVehicleRequest
* @return Result of the BatchCreateVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.BatchCreateVehicle
* @see AWS API Documentation
*/
@Override
public BatchCreateVehicleResponse batchCreateVehicle(BatchCreateVehicleRequest batchCreateVehicleRequest)
throws InternalServerException, LimitExceededException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchCreateVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchCreateVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchCreateVehicle");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchCreateVehicle").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(batchCreateVehicleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchCreateVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a group, or batch, of vehicles.
*
*
*
* You must specify a decoder manifest and a vehicle model (model manifest) for each vehicle.
*
*
*
* For more information, see Update multiple
* vehicles (AWS CLI) in the Amazon Web Services IoT FleetWise Developer Guide.
*
*
* @param batchUpdateVehicleRequest
* @return Result of the BatchUpdateVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.BatchUpdateVehicle
* @see AWS API Documentation
*/
@Override
public BatchUpdateVehicleResponse batchUpdateVehicle(BatchUpdateVehicleRequest batchUpdateVehicleRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, BatchUpdateVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, batchUpdateVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "BatchUpdateVehicle");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("BatchUpdateVehicle").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(batchUpdateVehicleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new BatchUpdateVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates an orchestration of data collection rules. The Amazon Web Services IoT FleetWise Edge Agent software
* running in vehicles uses campaigns to decide how to collect and transfer data to the cloud. You create campaigns
* in the cloud. After you or your team approve campaigns, Amazon Web Services IoT FleetWise automatically deploys
* them to vehicles.
*
*
* For more information, see Collect and transfer data
* with campaigns in the Amazon Web Services IoT FleetWise Developer Guide.
*
*
* @param createCampaignRequest
* @return Result of the CreateCampaign operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.CreateCampaign
* @see AWS
* API Documentation
*/
@Override
public CreateCampaignResponse createCampaign(CreateCampaignRequest createCampaignRequest) throws ResourceNotFoundException,
InternalServerException, ConflictException, LimitExceededException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createCampaignRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateCampaign");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateCampaign").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createCampaignRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateCampaignRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates the decoder manifest associated with a model manifest. To create a decoder manifest, the following must
* be true:
*
*
* -
*
* Every signal decoder has a unique name.
*
*
* -
*
* Each signal decoder is associated with a network interface.
*
*
* -
*
* Each network interface has a unique ID.
*
*
* -
*
* The signal decoders are specified in the model manifest.
*
*
*
*
* @param createDecoderManifestRequest
* @return Result of the CreateDecoderManifest operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws DecoderManifestValidationException
* The request couldn't be completed because it contains signal decoders with one or more validation errors.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.CreateDecoderManifest
* @see AWS API Documentation
*/
@Override
public CreateDecoderManifestResponse createDecoderManifest(CreateDecoderManifestRequest createDecoderManifestRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, LimitExceededException,
DecoderManifestValidationException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateDecoderManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createDecoderManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateDecoderManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateDecoderManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createDecoderManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateDecoderManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a fleet that represents a group of vehicles.
*
*
*
* You must create both a signal catalog and vehicles before you can create a fleet.
*
*
*
* For more information, see Fleets in the Amazon
* Web Services IoT FleetWise Developer Guide.
*
*
* @param createFleetRequest
* @return Result of the CreateFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.CreateFleet
* @see AWS API
* Documentation
*/
@Override
public CreateFleetResponse createFleet(CreateFleetRequest createFleetRequest) throws InternalServerException,
ResourceNotFoundException, ConflictException, LimitExceededException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a vehicle model (model manifest) that specifies signals (attributes, branches, sensors, and actuators).
*
*
* For more information, see Vehicle models in
* the Amazon Web Services IoT FleetWise Developer Guide.
*
*
* @param createModelManifestRequest
* @return Result of the CreateModelManifest operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InvalidSignalsException
* The request couldn't be completed because it contains signals that aren't valid.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.CreateModelManifest
* @see AWS API Documentation
*/
@Override
public CreateModelManifestResponse createModelManifest(CreateModelManifestRequest createModelManifestRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, LimitExceededException,
ThrottlingException, ValidationException, InvalidSignalsException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateModelManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createModelManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateModelManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateModelManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createModelManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateModelManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a collection of standardized signals that can be reused to create vehicle models.
*
*
* @param createSignalCatalogRequest
* @return Result of the CreateSignalCatalog operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws InvalidNodeException
* The specified node type doesn't match the expected node type for a node. You can specify the node type as
* branch, sensor, actuator, or attribute.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InvalidSignalsException
* The request couldn't be completed because it contains signals that aren't valid.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.CreateSignalCatalog
* @see AWS API Documentation
*/
@Override
public CreateSignalCatalogResponse createSignalCatalog(CreateSignalCatalogRequest createSignalCatalogRequest)
throws InternalServerException, ConflictException, LimitExceededException, InvalidNodeException, ThrottlingException,
ValidationException, InvalidSignalsException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateSignalCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createSignalCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateSignalCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateSignalCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createSignalCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateSignalCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a vehicle, which is an instance of a vehicle model (model manifest). Vehicles created from the same
* vehicle model consist of the same signals inherited from the vehicle model.
*
*
*
* If you have an existing Amazon Web Services IoT thing, you can use Amazon Web Services IoT FleetWise to create a
* vehicle and collect data from your thing.
*
*
*
* For more information, see Create a vehicle
* (AWS CLI) in the Amazon Web Services IoT FleetWise Developer Guide.
*
*
* @param createVehicleRequest
* @return Result of the CreateVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.CreateVehicle
* @see AWS
* API Documentation
*/
@Override
public CreateVehicleResponse createVehicle(CreateVehicleRequest createVehicleRequest) throws InternalServerException,
ResourceNotFoundException, ConflictException, LimitExceededException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateVehicle");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("CreateVehicle").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(createVehicleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new CreateVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a data collection campaign. Deleting a campaign suspends all data collection and removes it from any
* vehicles.
*
*
* @param deleteCampaignRequest
* @return Result of the DeleteCampaign operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DeleteCampaign
* @see AWS
* API Documentation
*/
@Override
public DeleteCampaignResponse deleteCampaign(DeleteCampaignRequest deleteCampaignRequest) throws ResourceNotFoundException,
InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteCampaignRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteCampaign");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteCampaign").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteCampaignRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteCampaignRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a decoder manifest. You can't delete a decoder manifest if it has vehicles associated with it.
*
*
*
* If the decoder manifest is successfully deleted, Amazon Web Services IoT FleetWise sends back an HTTP 200
* response with an empty body.
*
*
*
* @param deleteDecoderManifestRequest
* @return Result of the DeleteDecoderManifest operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DeleteDecoderManifest
* @see AWS API Documentation
*/
@Override
public DeleteDecoderManifestResponse deleteDecoderManifest(DeleteDecoderManifestRequest deleteDecoderManifestRequest)
throws InternalServerException, ConflictException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteDecoderManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteDecoderManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteDecoderManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteDecoderManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteDecoderManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteDecoderManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a fleet. Before you delete a fleet, all vehicles must be dissociated from the fleet. For more
* information, see Delete a fleet (AWS
* CLI) in the Amazon Web Services IoT FleetWise Developer Guide.
*
*
*
* If the fleet is successfully deleted, Amazon Web Services IoT FleetWise sends back an HTTP 200 response with an
* empty body.
*
*
*
* @param deleteFleetRequest
* @return Result of the DeleteFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DeleteFleet
* @see AWS API
* Documentation
*/
@Override
public DeleteFleetResponse deleteFleet(DeleteFleetRequest deleteFleetRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a vehicle model (model manifest).
*
*
*
* If the vehicle model is successfully deleted, Amazon Web Services IoT FleetWise sends back an HTTP 200 response
* with an empty body.
*
*
*
* @param deleteModelManifestRequest
* @return Result of the DeleteModelManifest operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DeleteModelManifest
* @see AWS API Documentation
*/
@Override
public DeleteModelManifestResponse deleteModelManifest(DeleteModelManifestRequest deleteModelManifestRequest)
throws InternalServerException, ConflictException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteModelManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteModelManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteModelManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteModelManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteModelManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteModelManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a signal catalog.
*
*
*
* If the signal catalog is successfully deleted, Amazon Web Services IoT FleetWise sends back an HTTP 200 response
* with an empty body.
*
*
*
* @param deleteSignalCatalogRequest
* @return Result of the DeleteSignalCatalog operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DeleteSignalCatalog
* @see AWS API Documentation
*/
@Override
public DeleteSignalCatalogResponse deleteSignalCatalog(DeleteSignalCatalogRequest deleteSignalCatalogRequest)
throws InternalServerException, ConflictException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteSignalCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteSignalCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteSignalCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteSignalCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteSignalCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteSignalCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Deletes a vehicle and removes it from any campaigns.
*
*
*
* If the vehicle is successfully deleted, Amazon Web Services IoT FleetWise sends back an HTTP 200 response with an
* empty body.
*
*
*
* @param deleteVehicleRequest
* @return Result of the DeleteVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DeleteVehicle
* @see AWS
* API Documentation
*/
@Override
public DeleteVehicleResponse deleteVehicle(DeleteVehicleRequest deleteVehicleRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteVehicle");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("DeleteVehicle").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(deleteVehicleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DeleteVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes, or disassociates, a vehicle from a fleet. Disassociating a vehicle from a fleet doesn't delete the
* vehicle.
*
*
*
* If the vehicle is successfully dissociated from a fleet, Amazon Web Services IoT FleetWise sends back an HTTP 200
* response with an empty body.
*
*
*
* @param disassociateVehicleFleetRequest
* @return Result of the DisassociateVehicleFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.DisassociateVehicleFleet
* @see AWS API Documentation
*/
@Override
public DisassociateVehicleFleetResponse disassociateVehicleFleet(
DisassociateVehicleFleetRequest disassociateVehicleFleetRequest) throws InternalServerException,
ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisassociateVehicleFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disassociateVehicleFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisassociateVehicleFleet");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisassociateVehicleFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(disassociateVehicleFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new DisassociateVehicleFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a campaign.
*
*
* @param getCampaignRequest
* @return Result of the GetCampaign operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetCampaign
* @see AWS API
* Documentation
*/
@Override
public GetCampaignResponse getCampaign(GetCampaignRequest getCampaignRequest) throws ResourceNotFoundException,
InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getCampaignRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetCampaign");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetCampaign").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getCampaignRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetCampaignRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a created decoder manifest.
*
*
* @param getDecoderManifestRequest
* @return Result of the GetDecoderManifest operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetDecoderManifest
* @see AWS API Documentation
*/
@Override
public GetDecoderManifestResponse getDecoderManifest(GetDecoderManifestRequest getDecoderManifestRequest)
throws ResourceNotFoundException, InternalServerException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetDecoderManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getDecoderManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetDecoderManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetDecoderManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getDecoderManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetDecoderManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a fleet.
*
*
* @param getFleetRequest
* @return Result of the GetFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetFleet
* @see AWS API
* Documentation
*/
@Override
public GetFleetResponse getFleet(GetFleetRequest getFleetRequest) throws InternalServerException, ResourceNotFoundException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getFleetRequest)
.withMetricCollector(apiCallMetricCollector).withMarshaller(new GetFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves the logging options.
*
*
* @param getLoggingOptionsRequest
* @return Result of the GetLoggingOptions operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetLoggingOptions
* @see AWS API Documentation
*/
@Override
public GetLoggingOptionsResponse getLoggingOptions(GetLoggingOptionsRequest getLoggingOptionsRequest)
throws InternalServerException, ThrottlingException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetLoggingOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getLoggingOptionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetLoggingOptions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetLoggingOptions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getLoggingOptionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetLoggingOptionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a vehicle model (model manifest).
*
*
* @param getModelManifestRequest
* @return Result of the GetModelManifest operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetModelManifest
* @see AWS
* API Documentation
*/
@Override
public GetModelManifestResponse getModelManifest(GetModelManifestRequest getModelManifestRequest)
throws ResourceNotFoundException, InternalServerException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetModelManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getModelManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetModelManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetModelManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getModelManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetModelManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about the status of registering your Amazon Web Services account, IAM, and Amazon
* Timestream resources so that Amazon Web Services IoT FleetWise can transfer your vehicle data to the Amazon Web
* Services Cloud.
*
*
* For more information, including step-by-step procedures, see Setting up Amazon Web
* Services IoT FleetWise.
*
*
*
* This API operation doesn't require input parameters.
*
*
*
* @param getRegisterAccountStatusRequest
* @return Result of the GetRegisterAccountStatus operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetRegisterAccountStatus
* @see AWS API Documentation
*/
@Override
public GetRegisterAccountStatusResponse getRegisterAccountStatus(
GetRegisterAccountStatusRequest getRegisterAccountStatusRequest) throws ResourceNotFoundException,
InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetRegisterAccountStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRegisterAccountStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRegisterAccountStatus");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetRegisterAccountStatus").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getRegisterAccountStatusRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetRegisterAccountStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a signal catalog.
*
*
* @param getSignalCatalogRequest
* @return Result of the GetSignalCatalog operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetSignalCatalog
* @see AWS
* API Documentation
*/
@Override
public GetSignalCatalogResponse getSignalCatalog(GetSignalCatalogRequest getSignalCatalogRequest)
throws ResourceNotFoundException, InternalServerException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetSignalCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getSignalCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetSignalCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetSignalCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getSignalCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetSignalCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about a vehicle.
*
*
* @param getVehicleRequest
* @return Result of the GetVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetVehicle
* @see AWS API
* Documentation
*/
@Override
public GetVehicleResponse getVehicle(GetVehicleRequest getVehicleRequest) throws InternalServerException,
ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetVehicle");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("GetVehicle")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(getVehicleRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information about the status of a vehicle with any associated campaigns.
*
*
* @param getVehicleStatusRequest
* @return Result of the GetVehicleStatus operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.GetVehicleStatus
* @see AWS
* API Documentation
*/
@Override
public GetVehicleStatusResponse getVehicleStatus(GetVehicleStatusRequest getVehicleStatusRequest)
throws ResourceNotFoundException, InternalServerException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetVehicleStatusResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getVehicleStatusRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetVehicleStatus");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("GetVehicleStatus").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(getVehicleStatusRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new GetVehicleStatusRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a decoder manifest using your existing CAN DBC file from your local device.
*
*
* @param importDecoderManifestRequest
* @return Result of the ImportDecoderManifest operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws DecoderManifestValidationException
* The request couldn't be completed because it contains signal decoders with one or more validation errors.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InvalidSignalsException
* The request couldn't be completed because it contains signals that aren't valid.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ImportDecoderManifest
* @see AWS API Documentation
*/
@Override
public ImportDecoderManifestResponse importDecoderManifest(ImportDecoderManifestRequest importDecoderManifestRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, DecoderManifestValidationException,
ThrottlingException, ValidationException, InvalidSignalsException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportDecoderManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importDecoderManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportDecoderManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ImportDecoderManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(importDecoderManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ImportDecoderManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates a signal catalog using your existing VSS formatted content from your local device.
*
*
* @param importSignalCatalogRequest
* @return Result of the ImportSignalCatalog operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InvalidSignalsException
* The request couldn't be completed because it contains signals that aren't valid.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ImportSignalCatalog
* @see AWS API Documentation
*/
@Override
public ImportSignalCatalogResponse importSignalCatalog(ImportSignalCatalogRequest importSignalCatalogRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, LimitExceededException,
ThrottlingException, ValidationException, InvalidSignalsException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ImportSignalCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, importSignalCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ImportSignalCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ImportSignalCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(importSignalCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ImportSignalCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists information about created campaigns.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listCampaignsRequest
* @return Result of the ListCampaigns operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListCampaigns
* @see AWS
* API Documentation
*/
@Override
public ListCampaignsResponse listCampaigns(ListCampaignsRequest listCampaignsRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListCampaignsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listCampaignsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListCampaigns");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListCampaigns").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listCampaignsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListCampaignsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the network interfaces specified in a decoder manifest.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listDecoderManifestNetworkInterfacesRequest
* @return Result of the ListDecoderManifestNetworkInterfaces operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListDecoderManifestNetworkInterfaces
* @see AWS API Documentation
*/
@Override
public ListDecoderManifestNetworkInterfacesResponse listDecoderManifestNetworkInterfaces(
ListDecoderManifestNetworkInterfacesRequest listDecoderManifestNetworkInterfacesRequest)
throws ResourceNotFoundException, InternalServerException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory
.createResponseHandler(operationMetadata, ListDecoderManifestNetworkInterfacesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration,
listDecoderManifestNetworkInterfacesRequest.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDecoderManifestNetworkInterfaces");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDecoderManifestNetworkInterfaces").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler)
.withInput(listDecoderManifestNetworkInterfacesRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDecoderManifestNetworkInterfacesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* A list of information about signal decoders specified in a decoder manifest.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listDecoderManifestSignalsRequest
* @return Result of the ListDecoderManifestSignals operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListDecoderManifestSignals
* @see AWS API Documentation
*/
@Override
public ListDecoderManifestSignalsResponse listDecoderManifestSignals(
ListDecoderManifestSignalsRequest listDecoderManifestSignalsRequest) throws ResourceNotFoundException,
InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDecoderManifestSignalsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDecoderManifestSignalsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDecoderManifestSignals");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListDecoderManifestSignals").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listDecoderManifestSignalsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDecoderManifestSignalsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists decoder manifests.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listDecoderManifestsRequest
* @return Result of the ListDecoderManifests operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListDecoderManifests
* @see AWS API Documentation
*/
@Override
public ListDecoderManifestsResponse listDecoderManifests(ListDecoderManifestsRequest listDecoderManifestsRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListDecoderManifestsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listDecoderManifestsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListDecoderManifests");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListDecoderManifests").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listDecoderManifestsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListDecoderManifestsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves information for each created fleet in an Amazon Web Services account.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listFleetsRequest
* @return Result of the ListFleets operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListFleets
* @see AWS API
* Documentation
*/
@Override
public ListFleetsResponse listFleets(ListFleetsRequest listFleetsRequest) throws InternalServerException,
ResourceNotFoundException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListFleetsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listFleetsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListFleets");
return clientHandler
.execute(new ClientExecutionParams().withOperationName("ListFleets")
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withInput(listFleetsRequest).withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListFleetsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of IDs for all fleets that the vehicle is associated with.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listFleetsForVehicleRequest
* @return Result of the ListFleetsForVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListFleetsForVehicle
* @see AWS API Documentation
*/
@Override
public ListFleetsForVehicleResponse listFleetsForVehicle(ListFleetsForVehicleRequest listFleetsForVehicleRequest)
throws InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListFleetsForVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listFleetsForVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListFleetsForVehicle");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListFleetsForVehicle").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listFleetsForVehicleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListFleetsForVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists information about nodes specified in a vehicle model (model manifest).
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listModelManifestNodesRequest
* @return Result of the ListModelManifestNodes operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListModelManifestNodes
* @see AWS API Documentation
*/
@Override
public ListModelManifestNodesResponse listModelManifestNodes(ListModelManifestNodesRequest listModelManifestNodesRequest)
throws InternalServerException, ResourceNotFoundException, LimitExceededException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListModelManifestNodesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listModelManifestNodesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListModelManifestNodes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListModelManifestNodes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listModelManifestNodesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListModelManifestNodesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of vehicle models (model manifests).
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listModelManifestsRequest
* @return Result of the ListModelManifests operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListModelManifests
* @see AWS API Documentation
*/
@Override
public ListModelManifestsResponse listModelManifests(ListModelManifestsRequest listModelManifestsRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListModelManifestsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listModelManifestsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListModelManifests");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListModelManifests").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listModelManifestsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListModelManifestsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists of information about the signals (nodes) specified in a signal catalog.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listSignalCatalogNodesRequest
* @return Result of the ListSignalCatalogNodes operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListSignalCatalogNodes
* @see AWS API Documentation
*/
@Override
public ListSignalCatalogNodesResponse listSignalCatalogNodes(ListSignalCatalogNodesRequest listSignalCatalogNodesRequest)
throws InternalServerException, ResourceNotFoundException, LimitExceededException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSignalCatalogNodesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSignalCatalogNodesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSignalCatalogNodes");
return clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListSignalCatalogNodes").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listSignalCatalogNodesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSignalCatalogNodesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists all the created signal catalogs in an Amazon Web Services account.
*
*
* You can use to list information about each signal (node) specified in a signal catalog.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listSignalCatalogsRequest
* @return Result of the ListSignalCatalogs operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListSignalCatalogs
* @see AWS API Documentation
*/
@Override
public ListSignalCatalogsResponse listSignalCatalogs(ListSignalCatalogsRequest listSignalCatalogsRequest)
throws InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListSignalCatalogsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listSignalCatalogsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListSignalCatalogs");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListSignalCatalogs").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listSignalCatalogsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListSignalCatalogsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Lists the tags (metadata) you have assigned to the resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResponse listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest)
throws ResourceNotFoundException, InternalServerException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listTagsForResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of summaries of created vehicles.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listVehiclesRequest
* @return Result of the ListVehicles operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListVehicles
* @see AWS API
* Documentation
*/
@Override
public ListVehiclesResponse listVehicles(ListVehiclesRequest listVehiclesRequest) throws InternalServerException,
ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListVehiclesResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listVehiclesRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListVehicles");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListVehicles").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listVehiclesRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListVehiclesRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Retrieves a list of summaries of all vehicles associated with a fleet.
*
*
*
* This API operation uses pagination. Specify the nextToken
parameter in the request to return more
* results.
*
*
*
* @param listVehiclesInFleetRequest
* @return Result of the ListVehiclesInFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.ListVehiclesInFleet
* @see AWS API Documentation
*/
@Override
public ListVehiclesInFleetResponse listVehiclesInFleet(ListVehiclesInFleetRequest listVehiclesInFleetRequest)
throws InternalServerException, ResourceNotFoundException, ThrottlingException, ValidationException,
AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListVehiclesInFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listVehiclesInFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListVehiclesInFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("ListVehiclesInFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(listVehiclesInFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new ListVehiclesInFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Creates or updates the logging option.
*
*
* @param putLoggingOptionsRequest
* @return Result of the PutLoggingOptions operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.PutLoggingOptions
* @see AWS API Documentation
*/
@Override
public PutLoggingOptionsResponse putLoggingOptions(PutLoggingOptionsRequest putLoggingOptionsRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
PutLoggingOptionsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, putLoggingOptionsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "PutLoggingOptions");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("PutLoggingOptions").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(putLoggingOptionsRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new PutLoggingOptionsRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
*
* This API operation contains deprecated parameters. Register your account again without the Timestream resources
* parameter so that Amazon Web Services IoT FleetWise can remove the Timestream metadata stored. You should then
* pass the data destination into the CreateCampaign
* API operation.
*
*
* You must delete any existing campaigns that include an empty data destination before you register your account
* again. For more information, see the DeleteCampaign
* API operation.
*
*
* If you want to delete the Timestream inline policy from the service-linked role, such as to mitigate an overly
* permissive policy, you must first delete any existing campaigns. Then delete the service-linked role and register
* your account again to enable CloudWatch metrics. For more information, see DeleteServiceLinkedRole in the Identity and Access Management API Reference.
*
*
*
*
* <p>Registers your Amazon Web Services account, IAM, and Amazon Timestream resources so Amazon Web Services IoT FleetWise can transfer your vehicle data to the Amazon Web Services Cloud. For more information, including step-by-step procedures, see <a href="https://docs.aws.amazon.com/iot-fleetwise/latest/developerguide/setting-up.html">Setting up Amazon Web Services IoT FleetWise</a>. </p> <note> <p>An Amazon Web Services account is <b>not</b> the same thing as a "user." An <a href="https://docs.aws.amazon.com/IAM/latest/UserGuide/introduction_identity-management.html#intro-identity-users">Amazon Web Services user</a> is an identity that you create using Identity and Access Management (IAM) and takes the form of either an <a href="https://docs.aws.amazon.com/IAM/latest/UserGuide/id_users.html">IAM user</a> or an <a href="https://docs.aws.amazon.com/IAM/latest/UserGuide/id_roles.html">IAM role, both with credentials</a>. A single Amazon Web Services account can, and typically does, contain many users and roles.</p> </note>
*
*
* @param registerAccountRequest
* @return Result of the RegisterAccount operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.RegisterAccount
* @see AWS
* API Documentation
*/
@Override
public RegisterAccountResponse registerAccount(RegisterAccountRequest registerAccountRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, ThrottlingException,
ValidationException, AccessDeniedException, AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
RegisterAccountResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, registerAccountRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "RegisterAccount");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("RegisterAccount").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(registerAccountRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new RegisterAccountRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Adds to or modifies the tags of the given resource. Tags are metadata which can be used to manage a resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public TagResourceResponse tagResource(TagResourceRequest tagResourceRequest) throws ResourceNotFoundException,
InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("TagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(tagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Removes the given tags (metadata) from the resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResponse untagResource(UntagResourceRequest untagResourceRequest) throws ResourceNotFoundException,
InternalServerException, ThrottlingException, ValidationException, AccessDeniedException, AwsServiceException,
SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(untagResourceRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a campaign.
*
*
* @param updateCampaignRequest
* @return Result of the UpdateCampaign operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UpdateCampaign
* @see AWS
* API Documentation
*/
@Override
public UpdateCampaignResponse updateCampaign(UpdateCampaignRequest updateCampaignRequest) throws ResourceNotFoundException,
InternalServerException, ConflictException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateCampaignResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateCampaignRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateCampaign");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateCampaign").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateCampaignRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateCampaignRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a decoder manifest.
*
*
* A decoder manifest can only be updated when the status is DRAFT
. Only ACTIVE
decoder
* manifests can be associated with vehicles.
*
*
* @param updateDecoderManifestRequest
* @return Result of the UpdateDecoderManifest operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws DecoderManifestValidationException
* The request couldn't be completed because it contains signal decoders with one or more validation errors.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UpdateDecoderManifest
* @see AWS API Documentation
*/
@Override
public UpdateDecoderManifestResponse updateDecoderManifest(UpdateDecoderManifestRequest updateDecoderManifestRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, LimitExceededException,
DecoderManifestValidationException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateDecoderManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateDecoderManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateDecoderManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateDecoderManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateDecoderManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateDecoderManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates the description of an existing fleet.
*
*
*
* If the fleet is successfully updated, Amazon Web Services IoT FleetWise sends back an HTTP 200 response with an
* empty HTTP body.
*
*
*
* @param updateFleetRequest
* @return Result of the UpdateFleet operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UpdateFleet
* @see AWS API
* Documentation
*/
@Override
public UpdateFleetResponse updateFleet(UpdateFleetRequest updateFleetRequest) throws InternalServerException,
ResourceNotFoundException, ConflictException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateFleetResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateFleetRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateFleet");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateFleet").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateFleetRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateFleetRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a vehicle model (model manifest). If created vehicles are associated with a vehicle model, it can't be
* updated.
*
*
* @param updateModelManifestRequest
* @return Result of the UpdateModelManifest operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InvalidSignalsException
* The request couldn't be completed because it contains signals that aren't valid.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UpdateModelManifest
* @see AWS API Documentation
*/
@Override
public UpdateModelManifestResponse updateModelManifest(UpdateModelManifestRequest updateModelManifestRequest)
throws InternalServerException, ResourceNotFoundException, ConflictException, ThrottlingException,
ValidationException, InvalidSignalsException, AccessDeniedException, AwsServiceException, SdkClientException,
IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateModelManifestResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateModelManifestRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateModelManifest");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateModelManifest").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateModelManifestRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateModelManifestRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a signal catalog.
*
*
* @param updateSignalCatalogRequest
* @return Result of the UpdateSignalCatalog operation returned by the service.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws LimitExceededException
* A service quota was exceeded.
* @throws InvalidNodeException
* The specified node type doesn't match the expected node type for a node. You can specify the node type as
* branch, sensor, actuator, or attribute.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws InvalidSignalsException
* The request couldn't be completed because it contains signals that aren't valid.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UpdateSignalCatalog
* @see AWS API Documentation
*/
@Override
public UpdateSignalCatalogResponse updateSignalCatalog(UpdateSignalCatalogRequest updateSignalCatalogRequest)
throws ResourceNotFoundException, InternalServerException, ConflictException, LimitExceededException,
InvalidNodeException, ThrottlingException, ValidationException, InvalidSignalsException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateSignalCatalogResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateSignalCatalogRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateSignalCatalog");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateSignalCatalog").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateSignalCatalogRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateSignalCatalogRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
/**
*
* Updates a vehicle.
*
*
* @param updateVehicleRequest
* @return Result of the UpdateVehicle operation returned by the service.
* @throws InternalServerException
* The request couldn't be completed because the server temporarily failed.
* @throws ResourceNotFoundException
* The resource wasn't found.
* @throws ConflictException
* The request has conflicting operations. This can occur if you're trying to perform more than one
* operation on the same resource at the same time.
* @throws ThrottlingException
* The request couldn't be completed due to throttling.
* @throws ValidationException
* The input fails to satisfy the constraints specified by an Amazon Web Services service.
* @throws AccessDeniedException
* You don't have sufficient permission to perform this action.
* @throws SdkException
* Base class for all exceptions that can be thrown by the SDK (both service and client). Can be used for
* catch all scenarios.
* @throws SdkClientException
* If any client side error occurs such as an IO related failure, failure to get credentials, etc.
* @throws IoTFleetWiseException
* Base class for all service exceptions. Unknown exceptions will be thrown as an instance of this type.
* @sample IoTFleetWiseClient.UpdateVehicle
* @see AWS
* API Documentation
*/
@Override
public UpdateVehicleResponse updateVehicle(UpdateVehicleRequest updateVehicleRequest) throws InternalServerException,
ResourceNotFoundException, ConflictException, ThrottlingException, ValidationException, AccessDeniedException,
AwsServiceException, SdkClientException, IoTFleetWiseException {
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateVehicleResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateVehicleRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "IoTFleetWise");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateVehicle");
return clientHandler.execute(new ClientExecutionParams()
.withOperationName("UpdateVehicle").withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withInput(updateVehicleRequest)
.withMetricCollector(apiCallMetricCollector)
.withMarshaller(new UpdateVehicleRequestMarshaller(protocolFactory)));
} finally {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
}
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(IoTFleetWiseException::builder)
.protocol(AwsJsonProtocol.AWS_JSON)
.protocolVersion("1.0")
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("DecoderManifestValidationException")
.exceptionBuilderSupplier(DecoderManifestValidationException::builder).httpStatusCode(400)
.build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidSignalsException")
.exceptionBuilderSupplier(InvalidSignalsException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("LimitExceededException")
.exceptionBuilderSupplier(LimitExceededException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InvalidNodeException")
.exceptionBuilderSupplier(InvalidNodeException::builder).httpStatusCode(400).build());
}
@Override
public final IoTFleetWiseServiceClientConfiguration serviceClientConfiguration() {
return this.serviceClientConfiguration;
}
@Override
public void close() {
clientHandler.close();
}
}