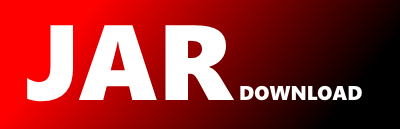
software.amazon.awssdk.services.iotfleetwise.model.S3Config Maven / Gradle / Ivy
Show all versions of iotfleetwise Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotfleetwise.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The Amazon S3 bucket where the Amazon Web Services IoT FleetWise campaign sends data. Amazon S3 is an object storage
* service that stores data as objects within buckets. For more information, see Creating, configuring, and
* working with Amazon S3 buckets in the Amazon Simple Storage Service User Guide.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class S3Config implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BUCKET_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("bucketArn").getter(getter(S3Config::bucketArn)).setter(setter(Builder::bucketArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("bucketArn").build()).build();
private static final SdkField DATA_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataFormat").getter(getter(S3Config::dataFormatAsString)).setter(setter(Builder::dataFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataFormat").build()).build();
private static final SdkField STORAGE_COMPRESSION_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("storageCompressionFormat").getter(getter(S3Config::storageCompressionFormatAsString))
.setter(setter(Builder::storageCompressionFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("storageCompressionFormat").build())
.build();
private static final SdkField PREFIX_FIELD = SdkField. builder(MarshallingType.STRING).memberName("prefix")
.getter(getter(S3Config::prefix)).setter(setter(Builder::prefix))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("prefix").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BUCKET_ARN_FIELD,
DATA_FORMAT_FIELD, STORAGE_COMPRESSION_FORMAT_FIELD, PREFIX_FIELD));
private static final long serialVersionUID = 1L;
private final String bucketArn;
private final String dataFormat;
private final String storageCompressionFormat;
private final String prefix;
private S3Config(BuilderImpl builder) {
this.bucketArn = builder.bucketArn;
this.dataFormat = builder.dataFormat;
this.storageCompressionFormat = builder.storageCompressionFormat;
this.prefix = builder.prefix;
}
/**
*
* The Amazon Resource Name (ARN) of the Amazon S3 bucket.
*
*
* @return The Amazon Resource Name (ARN) of the Amazon S3 bucket.
*/
public final String bucketArn() {
return bucketArn;
}
/**
*
* Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet or JSON
* format.
*
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and can reduce
* costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataFormat} will
* return {@link DataFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataFormatAsString}.
*
*
* @return Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet
* or JSON format.
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and
* can reduce costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
* @see DataFormat
*/
public final DataFormat dataFormat() {
return DataFormat.fromValue(dataFormat);
}
/**
*
* Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet or JSON
* format.
*
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and can reduce
* costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataFormat} will
* return {@link DataFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataFormatAsString}.
*
*
* @return Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet
* or JSON format.
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and
* can reduce costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
* @see DataFormat
*/
public final String dataFormatAsString() {
return dataFormat;
}
/**
*
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which can
* optimize the cost of data storage.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #storageCompressionFormat} will return {@link StorageCompressionFormat#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #storageCompressionFormatAsString}.
*
*
* @return By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which
* can optimize the cost of data storage.
* @see StorageCompressionFormat
*/
public final StorageCompressionFormat storageCompressionFormat() {
return StorageCompressionFormat.fromValue(storageCompressionFormat);
}
/**
*
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which can
* optimize the cost of data storage.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #storageCompressionFormat} will return {@link StorageCompressionFormat#UNKNOWN_TO_SDK_VERSION}. The raw
* value returned by the service is available from {@link #storageCompressionFormatAsString}.
*
*
* @return By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which
* can optimize the cost of data storage.
* @see StorageCompressionFormat
*/
public final String storageCompressionFormatAsString() {
return storageCompressionFormat;
}
/**
*
* (Optional) Enter an S3 bucket prefix. The prefix is the string of characters after the bucket name and before the
* object name. You can use the prefix to organize data stored in Amazon S3 buckets. For more information, see Organizing objects using
* prefixes in the Amazon Simple Storage Service User Guide.
*
*
* By default, Amazon Web Services IoT FleetWise sets the prefix
* processed-data/year=YY/month=MM/date=DD/hour=HH/
(in UTC) to data it delivers to Amazon S3. You can
* enter a prefix to append it to this default prefix. For example, if you enter the prefix vehicles
,
* the prefix will be vehicles/processed-data/year=YY/month=MM/date=DD/hour=HH/
.
*
*
* @return (Optional) Enter an S3 bucket prefix. The prefix is the string of characters after the bucket name and
* before the object name. You can use the prefix to organize data stored in Amazon S3 buckets. For more
* information, see Organizing objects using
* prefixes in the Amazon Simple Storage Service User Guide.
*
* By default, Amazon Web Services IoT FleetWise sets the prefix
* processed-data/year=YY/month=MM/date=DD/hour=HH/
(in UTC) to data it delivers to Amazon S3.
* You can enter a prefix to append it to this default prefix. For example, if you enter the prefix
* vehicles
, the prefix will be
* vehicles/processed-data/year=YY/month=MM/date=DD/hour=HH/
.
*/
public final String prefix() {
return prefix;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(bucketArn());
hashCode = 31 * hashCode + Objects.hashCode(dataFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(storageCompressionFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(prefix());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof S3Config)) {
return false;
}
S3Config other = (S3Config) obj;
return Objects.equals(bucketArn(), other.bucketArn()) && Objects.equals(dataFormatAsString(), other.dataFormatAsString())
&& Objects.equals(storageCompressionFormatAsString(), other.storageCompressionFormatAsString())
&& Objects.equals(prefix(), other.prefix());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("S3Config").add("BucketArn", bucketArn()).add("DataFormat", dataFormatAsString())
.add("StorageCompressionFormat", storageCompressionFormatAsString()).add("Prefix", prefix()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "bucketArn":
return Optional.ofNullable(clazz.cast(bucketArn()));
case "dataFormat":
return Optional.ofNullable(clazz.cast(dataFormatAsString()));
case "storageCompressionFormat":
return Optional.ofNullable(clazz.cast(storageCompressionFormatAsString()));
case "prefix":
return Optional.ofNullable(clazz.cast(prefix()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and
* can reduce costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
* @see DataFormat
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataFormat
*/
Builder dataFormat(String dataFormat);
/**
*
* Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache Parquet or
* JSON format.
*
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and can
* reduce costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
*
*
* @param dataFormat
* Specify the format that files are saved in the Amazon S3 bucket. You can save files in an Apache
* Parquet or JSON format.
*
* -
*
* Parquet - Store data in a columnar storage file format. Parquet is optimal for fast data retrieval and
* can reduce costs. This option is selected by default.
*
*
* -
*
* JSON - Store data in a standard text-based JSON file format.
*
*
* @see DataFormat
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataFormat
*/
Builder dataFormat(DataFormat dataFormat);
/**
*
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which can
* optimize the cost of data storage.
*
*
* @param storageCompressionFormat
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size,
* which can optimize the cost of data storage.
* @see StorageCompressionFormat
* @return Returns a reference to this object so that method calls can be chained together.
* @see StorageCompressionFormat
*/
Builder storageCompressionFormat(String storageCompressionFormat);
/**
*
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size, which can
* optimize the cost of data storage.
*
*
* @param storageCompressionFormat
* By default, stored data is compressed as a .gzip file. Compressed files have a reduced file size,
* which can optimize the cost of data storage.
* @see StorageCompressionFormat
* @return Returns a reference to this object so that method calls can be chained together.
* @see StorageCompressionFormat
*/
Builder storageCompressionFormat(StorageCompressionFormat storageCompressionFormat);
/**
*
* (Optional) Enter an S3 bucket prefix. The prefix is the string of characters after the bucket name and before
* the object name. You can use the prefix to organize data stored in Amazon S3 buckets. For more information,
* see Organizing objects
* using prefixes in the Amazon Simple Storage Service User Guide.
*
*
* By default, Amazon Web Services IoT FleetWise sets the prefix
* processed-data/year=YY/month=MM/date=DD/hour=HH/
(in UTC) to data it delivers to Amazon S3. You
* can enter a prefix to append it to this default prefix. For example, if you enter the prefix
* vehicles
, the prefix will be
* vehicles/processed-data/year=YY/month=MM/date=DD/hour=HH/
.
*
*
* @param prefix
* (Optional) Enter an S3 bucket prefix. The prefix is the string of characters after the bucket name and
* before the object name. You can use the prefix to organize data stored in Amazon S3 buckets. For more
* information, see Organizing objects
* using prefixes in the Amazon Simple Storage Service User Guide.
*
* By default, Amazon Web Services IoT FleetWise sets the prefix
* processed-data/year=YY/month=MM/date=DD/hour=HH/
(in UTC) to data it delivers to Amazon
* S3. You can enter a prefix to append it to this default prefix. For example, if you enter the prefix
* vehicles
, the prefix will be
* vehicles/processed-data/year=YY/month=MM/date=DD/hour=HH/
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder prefix(String prefix);
}
static final class BuilderImpl implements Builder {
private String bucketArn;
private String dataFormat;
private String storageCompressionFormat;
private String prefix;
private BuilderImpl() {
}
private BuilderImpl(S3Config model) {
bucketArn(model.bucketArn);
dataFormat(model.dataFormat);
storageCompressionFormat(model.storageCompressionFormat);
prefix(model.prefix);
}
public final String getBucketArn() {
return bucketArn;
}
public final void setBucketArn(String bucketArn) {
this.bucketArn = bucketArn;
}
@Override
public final Builder bucketArn(String bucketArn) {
this.bucketArn = bucketArn;
return this;
}
public final String getDataFormat() {
return dataFormat;
}
public final void setDataFormat(String dataFormat) {
this.dataFormat = dataFormat;
}
@Override
public final Builder dataFormat(String dataFormat) {
this.dataFormat = dataFormat;
return this;
}
@Override
public final Builder dataFormat(DataFormat dataFormat) {
this.dataFormat(dataFormat == null ? null : dataFormat.toString());
return this;
}
public final String getStorageCompressionFormat() {
return storageCompressionFormat;
}
public final void setStorageCompressionFormat(String storageCompressionFormat) {
this.storageCompressionFormat = storageCompressionFormat;
}
@Override
public final Builder storageCompressionFormat(String storageCompressionFormat) {
this.storageCompressionFormat = storageCompressionFormat;
return this;
}
@Override
public final Builder storageCompressionFormat(StorageCompressionFormat storageCompressionFormat) {
this.storageCompressionFormat(storageCompressionFormat == null ? null : storageCompressionFormat.toString());
return this;
}
public final String getPrefix() {
return prefix;
}
public final void setPrefix(String prefix) {
this.prefix = prefix;
}
@Override
public final Builder prefix(String prefix) {
this.prefix = prefix;
return this;
}
@Override
public S3Config build() {
return new S3Config(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}