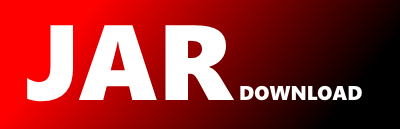
software.amazon.awssdk.services.iotsitewise.model.CreateAssetModelCompositeModelRequest Maven / Gradle / Ivy
Show all versions of iotsitewise Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotsitewise.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.DefaultValueTrait;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateAssetModelCompositeModelRequest extends IoTSiteWiseRequest implements
ToCopyableBuilder {
private static final SdkField ASSET_MODEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("assetModelId").getter(getter(CreateAssetModelCompositeModelRequest::assetModelId))
.setter(setter(Builder::assetModelId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("assetModelId").build()).build();
private static final SdkField ASSET_MODEL_COMPOSITE_MODEL_EXTERNAL_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("assetModelCompositeModelExternalId")
.getter(getter(CreateAssetModelCompositeModelRequest::assetModelCompositeModelExternalId))
.setter(setter(Builder::assetModelCompositeModelExternalId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assetModelCompositeModelExternalId")
.build()).build();
private static final SdkField PARENT_ASSET_MODEL_COMPOSITE_MODEL_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("parentAssetModelCompositeModelId")
.getter(getter(CreateAssetModelCompositeModelRequest::parentAssetModelCompositeModelId))
.setter(setter(Builder::parentAssetModelCompositeModelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("parentAssetModelCompositeModelId")
.build()).build();
private static final SdkField ASSET_MODEL_COMPOSITE_MODEL_ID_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("assetModelCompositeModelId")
.getter(getter(CreateAssetModelCompositeModelRequest::assetModelCompositeModelId))
.setter(setter(Builder::assetModelCompositeModelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assetModelCompositeModelId").build())
.build();
private static final SdkField ASSET_MODEL_COMPOSITE_MODEL_DESCRIPTION_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("assetModelCompositeModelDescription")
.getter(getter(CreateAssetModelCompositeModelRequest::assetModelCompositeModelDescription))
.setter(setter(Builder::assetModelCompositeModelDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("assetModelCompositeModelDescription").build()).build();
private static final SdkField ASSET_MODEL_COMPOSITE_MODEL_NAME_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("assetModelCompositeModelName")
.getter(getter(CreateAssetModelCompositeModelRequest::assetModelCompositeModelName))
.setter(setter(Builder::assetModelCompositeModelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assetModelCompositeModelName")
.build()).build();
private static final SdkField ASSET_MODEL_COMPOSITE_MODEL_TYPE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("assetModelCompositeModelType")
.getter(getter(CreateAssetModelCompositeModelRequest::assetModelCompositeModelType))
.setter(setter(Builder::assetModelCompositeModelType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assetModelCompositeModelType")
.build()).build();
private static final SdkField CLIENT_TOKEN_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("clientToken")
.getter(getter(CreateAssetModelCompositeModelRequest::clientToken))
.setter(setter(Builder::clientToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientToken").build(),
DefaultValueTrait.idempotencyToken()).build();
private static final SdkField COMPOSED_ASSET_MODEL_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("composedAssetModelId").getter(getter(CreateAssetModelCompositeModelRequest::composedAssetModelId))
.setter(setter(Builder::composedAssetModelId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("composedAssetModelId").build())
.build();
private static final SdkField> ASSET_MODEL_COMPOSITE_MODEL_PROPERTIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("assetModelCompositeModelProperties")
.getter(getter(CreateAssetModelCompositeModelRequest::assetModelCompositeModelProperties))
.setter(setter(Builder::assetModelCompositeModelProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assetModelCompositeModelProperties")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(AssetModelPropertyDefinition::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField IF_MATCH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ifMatch").getter(getter(CreateAssetModelCompositeModelRequest::ifMatch))
.setter(setter(Builder::ifMatch))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("If-Match").build()).build();
private static final SdkField IF_NONE_MATCH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ifNoneMatch").getter(getter(CreateAssetModelCompositeModelRequest::ifNoneMatch))
.setter(setter(Builder::ifNoneMatch))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("If-None-Match").build()).build();
private static final SdkField MATCH_FOR_VERSION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("matchForVersionType").getter(getter(CreateAssetModelCompositeModelRequest::matchForVersionTypeAsString))
.setter(setter(Builder::matchForVersionType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Match-For-Version-Type").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ASSET_MODEL_ID_FIELD,
ASSET_MODEL_COMPOSITE_MODEL_EXTERNAL_ID_FIELD, PARENT_ASSET_MODEL_COMPOSITE_MODEL_ID_FIELD,
ASSET_MODEL_COMPOSITE_MODEL_ID_FIELD, ASSET_MODEL_COMPOSITE_MODEL_DESCRIPTION_FIELD,
ASSET_MODEL_COMPOSITE_MODEL_NAME_FIELD, ASSET_MODEL_COMPOSITE_MODEL_TYPE_FIELD, CLIENT_TOKEN_FIELD,
COMPOSED_ASSET_MODEL_ID_FIELD, ASSET_MODEL_COMPOSITE_MODEL_PROPERTIES_FIELD, IF_MATCH_FIELD, IF_NONE_MATCH_FIELD,
MATCH_FOR_VERSION_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("assetModelId", ASSET_MODEL_ID_FIELD);
put("assetModelCompositeModelExternalId", ASSET_MODEL_COMPOSITE_MODEL_EXTERNAL_ID_FIELD);
put("parentAssetModelCompositeModelId", PARENT_ASSET_MODEL_COMPOSITE_MODEL_ID_FIELD);
put("assetModelCompositeModelId", ASSET_MODEL_COMPOSITE_MODEL_ID_FIELD);
put("assetModelCompositeModelDescription", ASSET_MODEL_COMPOSITE_MODEL_DESCRIPTION_FIELD);
put("assetModelCompositeModelName", ASSET_MODEL_COMPOSITE_MODEL_NAME_FIELD);
put("assetModelCompositeModelType", ASSET_MODEL_COMPOSITE_MODEL_TYPE_FIELD);
put("clientToken", CLIENT_TOKEN_FIELD);
put("composedAssetModelId", COMPOSED_ASSET_MODEL_ID_FIELD);
put("assetModelCompositeModelProperties", ASSET_MODEL_COMPOSITE_MODEL_PROPERTIES_FIELD);
put("If-Match", IF_MATCH_FIELD);
put("If-None-Match", IF_NONE_MATCH_FIELD);
put("Match-For-Version-Type", MATCH_FOR_VERSION_TYPE_FIELD);
}
});
private final String assetModelId;
private final String assetModelCompositeModelExternalId;
private final String parentAssetModelCompositeModelId;
private final String assetModelCompositeModelId;
private final String assetModelCompositeModelDescription;
private final String assetModelCompositeModelName;
private final String assetModelCompositeModelType;
private final String clientToken;
private final String composedAssetModelId;
private final List assetModelCompositeModelProperties;
private final String ifMatch;
private final String ifNoneMatch;
private final String matchForVersionType;
private CreateAssetModelCompositeModelRequest(BuilderImpl builder) {
super(builder);
this.assetModelId = builder.assetModelId;
this.assetModelCompositeModelExternalId = builder.assetModelCompositeModelExternalId;
this.parentAssetModelCompositeModelId = builder.parentAssetModelCompositeModelId;
this.assetModelCompositeModelId = builder.assetModelCompositeModelId;
this.assetModelCompositeModelDescription = builder.assetModelCompositeModelDescription;
this.assetModelCompositeModelName = builder.assetModelCompositeModelName;
this.assetModelCompositeModelType = builder.assetModelCompositeModelType;
this.clientToken = builder.clientToken;
this.composedAssetModelId = builder.composedAssetModelId;
this.assetModelCompositeModelProperties = builder.assetModelCompositeModelProperties;
this.ifMatch = builder.ifMatch;
this.ifNoneMatch = builder.ifNoneMatch;
this.matchForVersionType = builder.matchForVersionType;
}
/**
*
* The ID of the asset model this composite model is a part of.
*
*
* @return The ID of the asset model this composite model is a part of.
*/
public final String assetModelId() {
return assetModelId;
}
/**
*
* An external ID to assign to the composite model.
*
*
* If the composite model is a derived composite model, or one nested inside a component model, you can only set the
* external ID using UpdateAssetModelCompositeModel
and specifying the derived ID of the model or
* property from the created model it's a part of.
*
*
* @return An external ID to assign to the composite model.
*
* If the composite model is a derived composite model, or one nested inside a component model, you can only
* set the external ID using UpdateAssetModelCompositeModel
and specifying the derived ID of
* the model or property from the created model it's a part of.
*/
public final String assetModelCompositeModelExternalId() {
return assetModelCompositeModelExternalId;
}
/**
*
* The ID of the parent composite model in this asset model relationship.
*
*
* @return The ID of the parent composite model in this asset model relationship.
*/
public final String parentAssetModelCompositeModelId() {
return parentAssetModelCompositeModelId;
}
/**
*
* The ID of the composite model. IoT SiteWise automatically generates a unique ID for you, so this parameter is
* never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format. If
* you specify your own ID, it must be globally unique.
*
*
* @return The ID of the composite model. IoT SiteWise automatically generates a unique ID for you, so this
* parameter is never required. However, if you prefer to supply your own ID instead, you can specify it
* here in UUID format. If you specify your own ID, it must be globally unique.
*/
public final String assetModelCompositeModelId() {
return assetModelCompositeModelId;
}
/**
*
* A description for the composite model.
*
*
* @return A description for the composite model.
*/
public final String assetModelCompositeModelDescription() {
return assetModelCompositeModelDescription;
}
/**
*
* A unique name for the composite model.
*
*
* @return A unique name for the composite model.
*/
public final String assetModelCompositeModelName() {
return assetModelCompositeModelName;
}
/**
*
* The composite model type. Valid values are AWS/ALARM
, CUSTOM
, or
* AWS/L4E_ANOMALY
.
*
*
* @return The composite model type. Valid values are AWS/ALARM
, CUSTOM
, or
* AWS/L4E_ANOMALY
.
*/
public final String assetModelCompositeModelType() {
return assetModelCompositeModelType;
}
/**
*
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't reuse
* this client token if a new idempotent request is required.
*
*
* @return A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't
* reuse this client token if a new idempotent request is required.
*/
public final String clientToken() {
return clientToken;
}
/**
*
* The ID of a component model which is reused to create this composite model.
*
*
* @return The ID of a component model which is reused to create this composite model.
*/
public final String composedAssetModelId() {
return composedAssetModelId;
}
/**
* For responses, this returns true if the service returned a value for the AssetModelCompositeModelProperties
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasAssetModelCompositeModelProperties() {
return assetModelCompositeModelProperties != null
&& !(assetModelCompositeModelProperties instanceof SdkAutoConstructList);
}
/**
*
* The property definitions of the composite model. For more information, see
* Inline custom composite models in the IoT SiteWise User Guide.
*
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT SiteWise
* User Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasAssetModelCompositeModelProperties}
* method.
*
*
* @return The property definitions of the composite model. For more information, see Inline custom composite models in the IoT SiteWise User Guide.
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT
* SiteWise User Guide.
*/
public final List assetModelCompositeModelProperties() {
return assetModelCompositeModelProperties;
}
/**
*
* The expected current entity tag (ETag) for the asset model’s latest or active version (specified using
* matchForVersionType
). The create request is rejected if the tag does not match the latest or active
* version's current entity tag. See Optimistic locking
* for asset model writes in the IoT SiteWise User Guide.
*
*
* @return The expected current entity tag (ETag) for the asset model’s latest or active version (specified using
* matchForVersionType
). The create request is rejected if the tag does not match the latest or
* active version's current entity tag. See Optimistic
* locking for asset model writes in the IoT SiteWise User Guide.
*/
public final String ifMatch() {
return ifMatch;
}
/**
*
* Accepts * to reject the create request if an active version (specified using
* matchForVersionType
as ACTIVE
) already exists for the asset model.
*
*
* @return Accepts * to reject the create request if an active version (specified using
* matchForVersionType
as ACTIVE
) already exists for the asset model.
*/
public final String ifNoneMatch() {
return ifNoneMatch;
}
/**
*
* Specifies the asset model version type (LATEST
or ACTIVE
) used in conjunction with
* If-Match
or If-None-Match
headers to determine the target ETag for the create
* operation.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #matchForVersionType} will return {@link AssetModelVersionType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #matchForVersionTypeAsString}.
*
*
* @return Specifies the asset model version type (LATEST
or ACTIVE
) used in conjunction
* with If-Match
or If-None-Match
headers to determine the target ETag for the
* create operation.
* @see AssetModelVersionType
*/
public final AssetModelVersionType matchForVersionType() {
return AssetModelVersionType.fromValue(matchForVersionType);
}
/**
*
* Specifies the asset model version type (LATEST
or ACTIVE
) used in conjunction with
* If-Match
or If-None-Match
headers to determine the target ETag for the create
* operation.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #matchForVersionType} will return {@link AssetModelVersionType#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #matchForVersionTypeAsString}.
*
*
* @return Specifies the asset model version type (LATEST
or ACTIVE
) used in conjunction
* with If-Match
or If-None-Match
headers to determine the target ETag for the
* create operation.
* @see AssetModelVersionType
*/
public final String matchForVersionTypeAsString() {
return matchForVersionType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(assetModelId());
hashCode = 31 * hashCode + Objects.hashCode(assetModelCompositeModelExternalId());
hashCode = 31 * hashCode + Objects.hashCode(parentAssetModelCompositeModelId());
hashCode = 31 * hashCode + Objects.hashCode(assetModelCompositeModelId());
hashCode = 31 * hashCode + Objects.hashCode(assetModelCompositeModelDescription());
hashCode = 31 * hashCode + Objects.hashCode(assetModelCompositeModelName());
hashCode = 31 * hashCode + Objects.hashCode(assetModelCompositeModelType());
hashCode = 31 * hashCode + Objects.hashCode(clientToken());
hashCode = 31 * hashCode + Objects.hashCode(composedAssetModelId());
hashCode = 31 * hashCode
+ Objects.hashCode(hasAssetModelCompositeModelProperties() ? assetModelCompositeModelProperties() : null);
hashCode = 31 * hashCode + Objects.hashCode(ifMatch());
hashCode = 31 * hashCode + Objects.hashCode(ifNoneMatch());
hashCode = 31 * hashCode + Objects.hashCode(matchForVersionTypeAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateAssetModelCompositeModelRequest)) {
return false;
}
CreateAssetModelCompositeModelRequest other = (CreateAssetModelCompositeModelRequest) obj;
return Objects.equals(assetModelId(), other.assetModelId())
&& Objects.equals(assetModelCompositeModelExternalId(), other.assetModelCompositeModelExternalId())
&& Objects.equals(parentAssetModelCompositeModelId(), other.parentAssetModelCompositeModelId())
&& Objects.equals(assetModelCompositeModelId(), other.assetModelCompositeModelId())
&& Objects.equals(assetModelCompositeModelDescription(), other.assetModelCompositeModelDescription())
&& Objects.equals(assetModelCompositeModelName(), other.assetModelCompositeModelName())
&& Objects.equals(assetModelCompositeModelType(), other.assetModelCompositeModelType())
&& Objects.equals(clientToken(), other.clientToken())
&& Objects.equals(composedAssetModelId(), other.composedAssetModelId())
&& hasAssetModelCompositeModelProperties() == other.hasAssetModelCompositeModelProperties()
&& Objects.equals(assetModelCompositeModelProperties(), other.assetModelCompositeModelProperties())
&& Objects.equals(ifMatch(), other.ifMatch()) && Objects.equals(ifNoneMatch(), other.ifNoneMatch())
&& Objects.equals(matchForVersionTypeAsString(), other.matchForVersionTypeAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("CreateAssetModelCompositeModelRequest")
.add("AssetModelId", assetModelId())
.add("AssetModelCompositeModelExternalId", assetModelCompositeModelExternalId())
.add("ParentAssetModelCompositeModelId", parentAssetModelCompositeModelId())
.add("AssetModelCompositeModelId", assetModelCompositeModelId())
.add("AssetModelCompositeModelDescription", assetModelCompositeModelDescription())
.add("AssetModelCompositeModelName", assetModelCompositeModelName())
.add("AssetModelCompositeModelType", assetModelCompositeModelType())
.add("ClientToken", clientToken())
.add("ComposedAssetModelId", composedAssetModelId())
.add("AssetModelCompositeModelProperties",
hasAssetModelCompositeModelProperties() ? assetModelCompositeModelProperties() : null)
.add("IfMatch", ifMatch()).add("IfNoneMatch", ifNoneMatch())
.add("MatchForVersionType", matchForVersionTypeAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "assetModelId":
return Optional.ofNullable(clazz.cast(assetModelId()));
case "assetModelCompositeModelExternalId":
return Optional.ofNullable(clazz.cast(assetModelCompositeModelExternalId()));
case "parentAssetModelCompositeModelId":
return Optional.ofNullable(clazz.cast(parentAssetModelCompositeModelId()));
case "assetModelCompositeModelId":
return Optional.ofNullable(clazz.cast(assetModelCompositeModelId()));
case "assetModelCompositeModelDescription":
return Optional.ofNullable(clazz.cast(assetModelCompositeModelDescription()));
case "assetModelCompositeModelName":
return Optional.ofNullable(clazz.cast(assetModelCompositeModelName()));
case "assetModelCompositeModelType":
return Optional.ofNullable(clazz.cast(assetModelCompositeModelType()));
case "clientToken":
return Optional.ofNullable(clazz.cast(clientToken()));
case "composedAssetModelId":
return Optional.ofNullable(clazz.cast(composedAssetModelId()));
case "assetModelCompositeModelProperties":
return Optional.ofNullable(clazz.cast(assetModelCompositeModelProperties()));
case "ifMatch":
return Optional.ofNullable(clazz.cast(ifMatch()));
case "ifNoneMatch":
return Optional.ofNullable(clazz.cast(ifNoneMatch()));
case "matchForVersionType":
return Optional.ofNullable(clazz.cast(matchForVersionTypeAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* If the composite model is a derived composite model, or one nested inside a component model, you can
* only set the external ID using UpdateAssetModelCompositeModel
and specifying the derived
* ID of the model or property from the created model it's a part of.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelExternalId(String assetModelCompositeModelExternalId);
/**
*
* The ID of the parent composite model in this asset model relationship.
*
*
* @param parentAssetModelCompositeModelId
* The ID of the parent composite model in this asset model relationship.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder parentAssetModelCompositeModelId(String parentAssetModelCompositeModelId);
/**
*
* The ID of the composite model. IoT SiteWise automatically generates a unique ID for you, so this parameter is
* never required. However, if you prefer to supply your own ID instead, you can specify it here in UUID format.
* If you specify your own ID, it must be globally unique.
*
*
* @param assetModelCompositeModelId
* The ID of the composite model. IoT SiteWise automatically generates a unique ID for you, so this
* parameter is never required. However, if you prefer to supply your own ID instead, you can specify it
* here in UUID format. If you specify your own ID, it must be globally unique.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelId(String assetModelCompositeModelId);
/**
*
* A description for the composite model.
*
*
* @param assetModelCompositeModelDescription
* A description for the composite model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelDescription(String assetModelCompositeModelDescription);
/**
*
* A unique name for the composite model.
*
*
* @param assetModelCompositeModelName
* A unique name for the composite model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelName(String assetModelCompositeModelName);
/**
*
* The composite model type. Valid values are AWS/ALARM
, CUSTOM
, or
* AWS/L4E_ANOMALY
.
*
*
* @param assetModelCompositeModelType
* The composite model type. Valid values are AWS/ALARM
, CUSTOM
, or
* AWS/L4E_ANOMALY
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelType(String assetModelCompositeModelType);
/**
*
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request. Don't reuse
* this client token if a new idempotent request is required.
*
*
* @param clientToken
* A unique case-sensitive identifier that you can provide to ensure the idempotency of the request.
* Don't reuse this client token if a new idempotent request is required.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder clientToken(String clientToken);
/**
*
* The ID of a component model which is reused to create this composite model.
*
*
* @param composedAssetModelId
* The ID of a component model which is reused to create this composite model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder composedAssetModelId(String composedAssetModelId);
/**
*
* The property definitions of the composite model. For more information, see Inline custom composite models in the IoT SiteWise User Guide.
*
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT
* SiteWise User Guide.
*
*
* @param assetModelCompositeModelProperties
* The property definitions of the composite model. For more information, see Inline custom composite models in the IoT SiteWise User Guide.
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT
* SiteWise User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelProperties(Collection assetModelCompositeModelProperties);
/**
*
* The property definitions of the composite model. For more information, see Inline custom composite models in the IoT SiteWise User Guide.
*
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT
* SiteWise User Guide.
*
*
* @param assetModelCompositeModelProperties
* The property definitions of the composite model. For more information, see Inline custom composite models in the IoT SiteWise User Guide.
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT
* SiteWise User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder assetModelCompositeModelProperties(AssetModelPropertyDefinition... assetModelCompositeModelProperties);
/**
*
* The property definitions of the composite model. For more information, see Inline custom composite models in the IoT SiteWise User Guide.
*
*
* You can specify up to 200 properties per composite model. For more information, see Quotas in the IoT
* SiteWise User Guide.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.iotsitewise.model.AssetModelPropertyDefinition.Builder} avoiding the
* need to create one manually via
* {@link software.amazon.awssdk.services.iotsitewise.model.AssetModelPropertyDefinition#builder()}.
*
*
* When the {@link Consumer} completes,
* {@link software.amazon.awssdk.services.iotsitewise.model.AssetModelPropertyDefinition.Builder#build()} is
* called immediately and its result is passed to {@link
* #assetModelCompositeModelProperties(List)}.
*
* @param assetModelCompositeModelProperties
* a consumer that will call methods on
* {@link software.amazon.awssdk.services.iotsitewise.model.AssetModelPropertyDefinition.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #assetModelCompositeModelProperties(java.util.Collection)
*/
Builder assetModelCompositeModelProperties(
Consumer... assetModelCompositeModelProperties);
/**
*
* The expected current entity tag (ETag) for the asset model’s latest or active version (specified using
* matchForVersionType
). The create request is rejected if the tag does not match the latest or
* active version's current entity tag. See Optimistic
* locking for asset model writes in the IoT SiteWise User Guide.
*
*
* @param ifMatch
* The expected current entity tag (ETag) for the asset model’s latest or active version (specified using
* matchForVersionType
). The create request is rejected if the tag does not match the latest
* or active version's current entity tag. See Optimistic
* locking for asset model writes in the IoT SiteWise User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder ifMatch(String ifMatch);
/**
*
* Accepts * to reject the create request if an active version (specified using
* matchForVersionType
as ACTIVE
) already exists for the asset model.
*
*
* @param ifNoneMatch
* Accepts * to reject the create request if an active version (specified using
* matchForVersionType
as ACTIVE
) already exists for the asset model.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder ifNoneMatch(String ifNoneMatch);
/**
*
* Specifies the asset model version type (LATEST
or ACTIVE
) used in conjunction with
* If-Match
or If-None-Match
headers to determine the target ETag for the create
* operation.
*
*
* @param matchForVersionType
* Specifies the asset model version type (LATEST
or ACTIVE
) used in
* conjunction with If-Match
or If-None-Match
headers to determine the target
* ETag for the create operation.
* @see AssetModelVersionType
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetModelVersionType
*/
Builder matchForVersionType(String matchForVersionType);
/**
*
* Specifies the asset model version type (LATEST
or ACTIVE
) used in conjunction with
* If-Match
or If-None-Match
headers to determine the target ETag for the create
* operation.
*
*
* @param matchForVersionType
* Specifies the asset model version type (LATEST
or ACTIVE
) used in
* conjunction with If-Match
or If-None-Match
headers to determine the target
* ETag for the create operation.
* @see AssetModelVersionType
* @return Returns a reference to this object so that method calls can be chained together.
* @see AssetModelVersionType
*/
Builder matchForVersionType(AssetModelVersionType matchForVersionType);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends IoTSiteWiseRequest.BuilderImpl implements Builder {
private String assetModelId;
private String assetModelCompositeModelExternalId;
private String parentAssetModelCompositeModelId;
private String assetModelCompositeModelId;
private String assetModelCompositeModelDescription;
private String assetModelCompositeModelName;
private String assetModelCompositeModelType;
private String clientToken;
private String composedAssetModelId;
private List assetModelCompositeModelProperties = DefaultSdkAutoConstructList.getInstance();
private String ifMatch;
private String ifNoneMatch;
private String matchForVersionType;
private BuilderImpl() {
}
private BuilderImpl(CreateAssetModelCompositeModelRequest model) {
super(model);
assetModelId(model.assetModelId);
assetModelCompositeModelExternalId(model.assetModelCompositeModelExternalId);
parentAssetModelCompositeModelId(model.parentAssetModelCompositeModelId);
assetModelCompositeModelId(model.assetModelCompositeModelId);
assetModelCompositeModelDescription(model.assetModelCompositeModelDescription);
assetModelCompositeModelName(model.assetModelCompositeModelName);
assetModelCompositeModelType(model.assetModelCompositeModelType);
clientToken(model.clientToken);
composedAssetModelId(model.composedAssetModelId);
assetModelCompositeModelProperties(model.assetModelCompositeModelProperties);
ifMatch(model.ifMatch);
ifNoneMatch(model.ifNoneMatch);
matchForVersionType(model.matchForVersionType);
}
public final String getAssetModelId() {
return assetModelId;
}
public final void setAssetModelId(String assetModelId) {
this.assetModelId = assetModelId;
}
@Override
public final Builder assetModelId(String assetModelId) {
this.assetModelId = assetModelId;
return this;
}
public final String getAssetModelCompositeModelExternalId() {
return assetModelCompositeModelExternalId;
}
public final void setAssetModelCompositeModelExternalId(String assetModelCompositeModelExternalId) {
this.assetModelCompositeModelExternalId = assetModelCompositeModelExternalId;
}
@Override
public final Builder assetModelCompositeModelExternalId(String assetModelCompositeModelExternalId) {
this.assetModelCompositeModelExternalId = assetModelCompositeModelExternalId;
return this;
}
public final String getParentAssetModelCompositeModelId() {
return parentAssetModelCompositeModelId;
}
public final void setParentAssetModelCompositeModelId(String parentAssetModelCompositeModelId) {
this.parentAssetModelCompositeModelId = parentAssetModelCompositeModelId;
}
@Override
public final Builder parentAssetModelCompositeModelId(String parentAssetModelCompositeModelId) {
this.parentAssetModelCompositeModelId = parentAssetModelCompositeModelId;
return this;
}
public final String getAssetModelCompositeModelId() {
return assetModelCompositeModelId;
}
public final void setAssetModelCompositeModelId(String assetModelCompositeModelId) {
this.assetModelCompositeModelId = assetModelCompositeModelId;
}
@Override
public final Builder assetModelCompositeModelId(String assetModelCompositeModelId) {
this.assetModelCompositeModelId = assetModelCompositeModelId;
return this;
}
public final String getAssetModelCompositeModelDescription() {
return assetModelCompositeModelDescription;
}
public final void setAssetModelCompositeModelDescription(String assetModelCompositeModelDescription) {
this.assetModelCompositeModelDescription = assetModelCompositeModelDescription;
}
@Override
public final Builder assetModelCompositeModelDescription(String assetModelCompositeModelDescription) {
this.assetModelCompositeModelDescription = assetModelCompositeModelDescription;
return this;
}
public final String getAssetModelCompositeModelName() {
return assetModelCompositeModelName;
}
public final void setAssetModelCompositeModelName(String assetModelCompositeModelName) {
this.assetModelCompositeModelName = assetModelCompositeModelName;
}
@Override
public final Builder assetModelCompositeModelName(String assetModelCompositeModelName) {
this.assetModelCompositeModelName = assetModelCompositeModelName;
return this;
}
public final String getAssetModelCompositeModelType() {
return assetModelCompositeModelType;
}
public final void setAssetModelCompositeModelType(String assetModelCompositeModelType) {
this.assetModelCompositeModelType = assetModelCompositeModelType;
}
@Override
public final Builder assetModelCompositeModelType(String assetModelCompositeModelType) {
this.assetModelCompositeModelType = assetModelCompositeModelType;
return this;
}
public final String getClientToken() {
return clientToken;
}
public final void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
@Override
public final Builder clientToken(String clientToken) {
this.clientToken = clientToken;
return this;
}
public final String getComposedAssetModelId() {
return composedAssetModelId;
}
public final void setComposedAssetModelId(String composedAssetModelId) {
this.composedAssetModelId = composedAssetModelId;
}
@Override
public final Builder composedAssetModelId(String composedAssetModelId) {
this.composedAssetModelId = composedAssetModelId;
return this;
}
public final List getAssetModelCompositeModelProperties() {
List result = AssetModelPropertyDefinitionsCopier
.copyToBuilder(this.assetModelCompositeModelProperties);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setAssetModelCompositeModelProperties(
Collection assetModelCompositeModelProperties) {
this.assetModelCompositeModelProperties = AssetModelPropertyDefinitionsCopier
.copyFromBuilder(assetModelCompositeModelProperties);
}
@Override
public final Builder assetModelCompositeModelProperties(
Collection assetModelCompositeModelProperties) {
this.assetModelCompositeModelProperties = AssetModelPropertyDefinitionsCopier
.copy(assetModelCompositeModelProperties);
return this;
}
@Override
@SafeVarargs
public final Builder assetModelCompositeModelProperties(
AssetModelPropertyDefinition... assetModelCompositeModelProperties) {
assetModelCompositeModelProperties(Arrays.asList(assetModelCompositeModelProperties));
return this;
}
@Override
@SafeVarargs
public final Builder assetModelCompositeModelProperties(
Consumer... assetModelCompositeModelProperties) {
assetModelCompositeModelProperties(Stream.of(assetModelCompositeModelProperties)
.map(c -> AssetModelPropertyDefinition.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final String getIfMatch() {
return ifMatch;
}
public final void setIfMatch(String ifMatch) {
this.ifMatch = ifMatch;
}
@Override
public final Builder ifMatch(String ifMatch) {
this.ifMatch = ifMatch;
return this;
}
public final String getIfNoneMatch() {
return ifNoneMatch;
}
public final void setIfNoneMatch(String ifNoneMatch) {
this.ifNoneMatch = ifNoneMatch;
}
@Override
public final Builder ifNoneMatch(String ifNoneMatch) {
this.ifNoneMatch = ifNoneMatch;
return this;
}
public final String getMatchForVersionType() {
return matchForVersionType;
}
public final void setMatchForVersionType(String matchForVersionType) {
this.matchForVersionType = matchForVersionType;
}
@Override
public final Builder matchForVersionType(String matchForVersionType) {
this.matchForVersionType = matchForVersionType;
return this;
}
@Override
public final Builder matchForVersionType(AssetModelVersionType matchForVersionType) {
this.matchForVersionType(matchForVersionType == null ? null : matchForVersionType.toString());
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateAssetModelCompositeModelRequest build() {
return new CreateAssetModelCompositeModelRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}