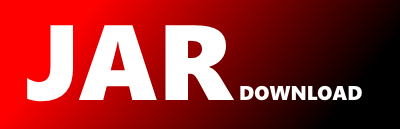
software.amazon.awssdk.services.iotsitewise.model.DescribeTimeSeriesResponse Maven / Gradle / Ivy
Show all versions of iotsitewise Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iotsitewise.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class DescribeTimeSeriesResponse extends IoTSiteWiseResponse implements
ToCopyableBuilder {
private static final SdkField ASSET_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("assetId").getter(getter(DescribeTimeSeriesResponse::assetId)).setter(setter(Builder::assetId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("assetId").build()).build();
private static final SdkField PROPERTY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("propertyId").getter(getter(DescribeTimeSeriesResponse::propertyId)).setter(setter(Builder::propertyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propertyId").build()).build();
private static final SdkField ALIAS_FIELD = SdkField. builder(MarshallingType.STRING).memberName("alias")
.getter(getter(DescribeTimeSeriesResponse::alias)).setter(setter(Builder::alias))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("alias").build()).build();
private static final SdkField TIME_SERIES_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("timeSeriesId").getter(getter(DescribeTimeSeriesResponse::timeSeriesId))
.setter(setter(Builder::timeSeriesId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeSeriesId").build()).build();
private static final SdkField DATA_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataType").getter(getter(DescribeTimeSeriesResponse::dataTypeAsString))
.setter(setter(Builder::dataType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataType").build()).build();
private static final SdkField DATA_TYPE_SPEC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dataTypeSpec").getter(getter(DescribeTimeSeriesResponse::dataTypeSpec))
.setter(setter(Builder::dataTypeSpec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dataTypeSpec").build()).build();
private static final SdkField TIME_SERIES_CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("timeSeriesCreationDate").getter(getter(DescribeTimeSeriesResponse::timeSeriesCreationDate))
.setter(setter(Builder::timeSeriesCreationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeSeriesCreationDate").build())
.build();
private static final SdkField TIME_SERIES_LAST_UPDATE_DATE_FIELD = SdkField
. builder(MarshallingType.INSTANT).memberName("timeSeriesLastUpdateDate")
.getter(getter(DescribeTimeSeriesResponse::timeSeriesLastUpdateDate))
.setter(setter(Builder::timeSeriesLastUpdateDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeSeriesLastUpdateDate").build())
.build();
private static final SdkField TIME_SERIES_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("timeSeriesArn").getter(getter(DescribeTimeSeriesResponse::timeSeriesArn))
.setter(setter(Builder::timeSeriesArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("timeSeriesArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(ASSET_ID_FIELD,
PROPERTY_ID_FIELD, ALIAS_FIELD, TIME_SERIES_ID_FIELD, DATA_TYPE_FIELD, DATA_TYPE_SPEC_FIELD,
TIME_SERIES_CREATION_DATE_FIELD, TIME_SERIES_LAST_UPDATE_DATE_FIELD, TIME_SERIES_ARN_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("assetId", ASSET_ID_FIELD);
put("propertyId", PROPERTY_ID_FIELD);
put("alias", ALIAS_FIELD);
put("timeSeriesId", TIME_SERIES_ID_FIELD);
put("dataType", DATA_TYPE_FIELD);
put("dataTypeSpec", DATA_TYPE_SPEC_FIELD);
put("timeSeriesCreationDate", TIME_SERIES_CREATION_DATE_FIELD);
put("timeSeriesLastUpdateDate", TIME_SERIES_LAST_UPDATE_DATE_FIELD);
put("timeSeriesArn", TIME_SERIES_ARN_FIELD);
}
});
private final String assetId;
private final String propertyId;
private final String alias;
private final String timeSeriesId;
private final String dataType;
private final String dataTypeSpec;
private final Instant timeSeriesCreationDate;
private final Instant timeSeriesLastUpdateDate;
private final String timeSeriesArn;
private DescribeTimeSeriesResponse(BuilderImpl builder) {
super(builder);
this.assetId = builder.assetId;
this.propertyId = builder.propertyId;
this.alias = builder.alias;
this.timeSeriesId = builder.timeSeriesId;
this.dataType = builder.dataType;
this.dataTypeSpec = builder.dataTypeSpec;
this.timeSeriesCreationDate = builder.timeSeriesCreationDate;
this.timeSeriesLastUpdateDate = builder.timeSeriesLastUpdateDate;
this.timeSeriesArn = builder.timeSeriesArn;
}
/**
*
* The ID of the asset in which the asset property was created.
*
*
* @return The ID of the asset in which the asset property was created.
*/
public final String assetId() {
return assetId;
}
/**
*
* The ID of the asset property, in UUID format.
*
*
* @return The ID of the asset property, in UUID format.
*/
public final String propertyId() {
return propertyId;
}
/**
*
* The alias that identifies the time series.
*
*
* @return The alias that identifies the time series.
*/
public final String alias() {
return alias;
}
/**
*
* The ID of the time series.
*
*
* @return The ID of the time series.
*/
public final String timeSeriesId() {
return timeSeriesId;
}
/**
*
* The data type of the time series.
*
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the type of the
* structure for this time series.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataType} will
* return {@link PropertyDataType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTypeAsString}.
*
*
* @return The data type of the time series.
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the type
* of the structure for this time series.
* @see PropertyDataType
*/
public final PropertyDataType dataType() {
return PropertyDataType.fromValue(dataType);
}
/**
*
* The data type of the time series.
*
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the type of the
* structure for this time series.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dataType} will
* return {@link PropertyDataType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dataTypeAsString}.
*
*
* @return The data type of the time series.
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the type
* of the structure for this time series.
* @see PropertyDataType
*/
public final String dataTypeAsString() {
return dataType;
}
/**
*
* The data type of the structure for this time series. This parameter is required for time series that have the
* STRUCT
data type.
*
*
* The options for this parameter depend on the type of the composite model in which you created the asset property
* that is associated with your time series. Use AWS/ALARM_STATE
for alarm state in alarm composite
* models.
*
*
* @return The data type of the structure for this time series. This parameter is required for time series that have
* the STRUCT
data type.
*
* The options for this parameter depend on the type of the composite model in which you created the asset
* property that is associated with your time series. Use AWS/ALARM_STATE
for alarm state in
* alarm composite models.
*/
public final String dataTypeSpec() {
return dataTypeSpec;
}
/**
*
* The date that the time series was created, in Unix epoch time.
*
*
* @return The date that the time series was created, in Unix epoch time.
*/
public final Instant timeSeriesCreationDate() {
return timeSeriesCreationDate;
}
/**
*
* The date that the time series was last updated, in Unix epoch time.
*
*
* @return The date that the time series was last updated, in Unix epoch time.
*/
public final Instant timeSeriesLastUpdateDate() {
return timeSeriesLastUpdateDate;
}
/**
*
* The ARN of the time
* series, which has the following format.
*
*
* arn:${Partition}:iotsitewise:${Region}:${Account}:time-series/${TimeSeriesId}
*
*
* @return The ARN of the
* time series, which has the following format.
*
* arn:${Partition}:iotsitewise:${Region}:${Account}:time-series/${TimeSeriesId}
*/
public final String timeSeriesArn() {
return timeSeriesArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(assetId());
hashCode = 31 * hashCode + Objects.hashCode(propertyId());
hashCode = 31 * hashCode + Objects.hashCode(alias());
hashCode = 31 * hashCode + Objects.hashCode(timeSeriesId());
hashCode = 31 * hashCode + Objects.hashCode(dataTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(dataTypeSpec());
hashCode = 31 * hashCode + Objects.hashCode(timeSeriesCreationDate());
hashCode = 31 * hashCode + Objects.hashCode(timeSeriesLastUpdateDate());
hashCode = 31 * hashCode + Objects.hashCode(timeSeriesArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DescribeTimeSeriesResponse)) {
return false;
}
DescribeTimeSeriesResponse other = (DescribeTimeSeriesResponse) obj;
return Objects.equals(assetId(), other.assetId()) && Objects.equals(propertyId(), other.propertyId())
&& Objects.equals(alias(), other.alias()) && Objects.equals(timeSeriesId(), other.timeSeriesId())
&& Objects.equals(dataTypeAsString(), other.dataTypeAsString())
&& Objects.equals(dataTypeSpec(), other.dataTypeSpec())
&& Objects.equals(timeSeriesCreationDate(), other.timeSeriesCreationDate())
&& Objects.equals(timeSeriesLastUpdateDate(), other.timeSeriesLastUpdateDate())
&& Objects.equals(timeSeriesArn(), other.timeSeriesArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("DescribeTimeSeriesResponse").add("AssetId", assetId()).add("PropertyId", propertyId())
.add("Alias", alias()).add("TimeSeriesId", timeSeriesId()).add("DataType", dataTypeAsString())
.add("DataTypeSpec", dataTypeSpec()).add("TimeSeriesCreationDate", timeSeriesCreationDate())
.add("TimeSeriesLastUpdateDate", timeSeriesLastUpdateDate()).add("TimeSeriesArn", timeSeriesArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "assetId":
return Optional.ofNullable(clazz.cast(assetId()));
case "propertyId":
return Optional.ofNullable(clazz.cast(propertyId()));
case "alias":
return Optional.ofNullable(clazz.cast(alias()));
case "timeSeriesId":
return Optional.ofNullable(clazz.cast(timeSeriesId()));
case "dataType":
return Optional.ofNullable(clazz.cast(dataTypeAsString()));
case "dataTypeSpec":
return Optional.ofNullable(clazz.cast(dataTypeSpec()));
case "timeSeriesCreationDate":
return Optional.ofNullable(clazz.cast(timeSeriesCreationDate()));
case "timeSeriesLastUpdateDate":
return Optional.ofNullable(clazz.cast(timeSeriesLastUpdateDate()));
case "timeSeriesArn":
return Optional.ofNullable(clazz.cast(timeSeriesArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the
* type of the structure for this time series.
* @see PropertyDataType
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropertyDataType
*/
Builder dataType(String dataType);
/**
*
* The data type of the time series.
*
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the type of
* the structure for this time series.
*
*
* @param dataType
* The data type of the time series.
*
* If you specify STRUCT
, you must also specify dataTypeSpec
to identify the
* type of the structure for this time series.
* @see PropertyDataType
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropertyDataType
*/
Builder dataType(PropertyDataType dataType);
/**
*
* The data type of the structure for this time series. This parameter is required for time series that have the
* STRUCT
data type.
*
*
* The options for this parameter depend on the type of the composite model in which you created the asset
* property that is associated with your time series. Use AWS/ALARM_STATE
for alarm state in alarm
* composite models.
*
*
* @param dataTypeSpec
* The data type of the structure for this time series. This parameter is required for time series that
* have the STRUCT
data type.
*
* The options for this parameter depend on the type of the composite model in which you created the
* asset property that is associated with your time series. Use AWS/ALARM_STATE
for alarm
* state in alarm composite models.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dataTypeSpec(String dataTypeSpec);
/**
*
* The date that the time series was created, in Unix epoch time.
*
*
* @param timeSeriesCreationDate
* The date that the time series was created, in Unix epoch time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeSeriesCreationDate(Instant timeSeriesCreationDate);
/**
*
* The date that the time series was last updated, in Unix epoch time.
*
*
* @param timeSeriesLastUpdateDate
* The date that the time series was last updated, in Unix epoch time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeSeriesLastUpdateDate(Instant timeSeriesLastUpdateDate);
/**
*
* The ARN of the time
* series, which has the following format.
*
*
* arn:${Partition}:iotsitewise:${Region}:${Account}:time-series/${TimeSeriesId}
*
*
* @param timeSeriesArn
* The ARN of
* the time series, which has the following format.
*
* arn:${Partition}:iotsitewise:${Region}:${Account}:time-series/${TimeSeriesId}
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder timeSeriesArn(String timeSeriesArn);
}
static final class BuilderImpl extends IoTSiteWiseResponse.BuilderImpl implements Builder {
private String assetId;
private String propertyId;
private String alias;
private String timeSeriesId;
private String dataType;
private String dataTypeSpec;
private Instant timeSeriesCreationDate;
private Instant timeSeriesLastUpdateDate;
private String timeSeriesArn;
private BuilderImpl() {
}
private BuilderImpl(DescribeTimeSeriesResponse model) {
super(model);
assetId(model.assetId);
propertyId(model.propertyId);
alias(model.alias);
timeSeriesId(model.timeSeriesId);
dataType(model.dataType);
dataTypeSpec(model.dataTypeSpec);
timeSeriesCreationDate(model.timeSeriesCreationDate);
timeSeriesLastUpdateDate(model.timeSeriesLastUpdateDate);
timeSeriesArn(model.timeSeriesArn);
}
public final String getAssetId() {
return assetId;
}
public final void setAssetId(String assetId) {
this.assetId = assetId;
}
@Override
public final Builder assetId(String assetId) {
this.assetId = assetId;
return this;
}
public final String getPropertyId() {
return propertyId;
}
public final void setPropertyId(String propertyId) {
this.propertyId = propertyId;
}
@Override
public final Builder propertyId(String propertyId) {
this.propertyId = propertyId;
return this;
}
public final String getAlias() {
return alias;
}
public final void setAlias(String alias) {
this.alias = alias;
}
@Override
public final Builder alias(String alias) {
this.alias = alias;
return this;
}
public final String getTimeSeriesId() {
return timeSeriesId;
}
public final void setTimeSeriesId(String timeSeriesId) {
this.timeSeriesId = timeSeriesId;
}
@Override
public final Builder timeSeriesId(String timeSeriesId) {
this.timeSeriesId = timeSeriesId;
return this;
}
public final String getDataType() {
return dataType;
}
public final void setDataType(String dataType) {
this.dataType = dataType;
}
@Override
public final Builder dataType(String dataType) {
this.dataType = dataType;
return this;
}
@Override
public final Builder dataType(PropertyDataType dataType) {
this.dataType(dataType == null ? null : dataType.toString());
return this;
}
public final String getDataTypeSpec() {
return dataTypeSpec;
}
public final void setDataTypeSpec(String dataTypeSpec) {
this.dataTypeSpec = dataTypeSpec;
}
@Override
public final Builder dataTypeSpec(String dataTypeSpec) {
this.dataTypeSpec = dataTypeSpec;
return this;
}
public final Instant getTimeSeriesCreationDate() {
return timeSeriesCreationDate;
}
public final void setTimeSeriesCreationDate(Instant timeSeriesCreationDate) {
this.timeSeriesCreationDate = timeSeriesCreationDate;
}
@Override
public final Builder timeSeriesCreationDate(Instant timeSeriesCreationDate) {
this.timeSeriesCreationDate = timeSeriesCreationDate;
return this;
}
public final Instant getTimeSeriesLastUpdateDate() {
return timeSeriesLastUpdateDate;
}
public final void setTimeSeriesLastUpdateDate(Instant timeSeriesLastUpdateDate) {
this.timeSeriesLastUpdateDate = timeSeriesLastUpdateDate;
}
@Override
public final Builder timeSeriesLastUpdateDate(Instant timeSeriesLastUpdateDate) {
this.timeSeriesLastUpdateDate = timeSeriesLastUpdateDate;
return this;
}
public final String getTimeSeriesArn() {
return timeSeriesArn;
}
public final void setTimeSeriesArn(String timeSeriesArn) {
this.timeSeriesArn = timeSeriesArn;
}
@Override
public final Builder timeSeriesArn(String timeSeriesArn) {
this.timeSeriesArn = timeSeriesArn;
return this;
}
@Override
public DescribeTimeSeriesResponse build() {
return new DescribeTimeSeriesResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}