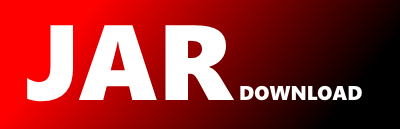
software.amazon.awssdk.services.iottwinmaker.IoTTwinMakerAsyncClient Maven / Gradle / Ivy
Show all versions of iottwinmaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iottwinmaker;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.iottwinmaker.model.BatchPutPropertyValuesRequest;
import software.amazon.awssdk.services.iottwinmaker.model.BatchPutPropertyValuesResponse;
import software.amazon.awssdk.services.iottwinmaker.model.CreateComponentTypeRequest;
import software.amazon.awssdk.services.iottwinmaker.model.CreateComponentTypeResponse;
import software.amazon.awssdk.services.iottwinmaker.model.CreateEntityRequest;
import software.amazon.awssdk.services.iottwinmaker.model.CreateEntityResponse;
import software.amazon.awssdk.services.iottwinmaker.model.CreateSceneRequest;
import software.amazon.awssdk.services.iottwinmaker.model.CreateSceneResponse;
import software.amazon.awssdk.services.iottwinmaker.model.CreateWorkspaceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.CreateWorkspaceResponse;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteComponentTypeRequest;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteComponentTypeResponse;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteEntityRequest;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteEntityResponse;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteSceneRequest;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteSceneResponse;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteWorkspaceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.DeleteWorkspaceResponse;
import software.amazon.awssdk.services.iottwinmaker.model.GetComponentTypeRequest;
import software.amazon.awssdk.services.iottwinmaker.model.GetComponentTypeResponse;
import software.amazon.awssdk.services.iottwinmaker.model.GetEntityRequest;
import software.amazon.awssdk.services.iottwinmaker.model.GetEntityResponse;
import software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryRequest;
import software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryResponse;
import software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueRequest;
import software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueResponse;
import software.amazon.awssdk.services.iottwinmaker.model.GetSceneRequest;
import software.amazon.awssdk.services.iottwinmaker.model.GetSceneResponse;
import software.amazon.awssdk.services.iottwinmaker.model.GetWorkspaceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.GetWorkspaceResponse;
import software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesRequest;
import software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesResponse;
import software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesRequest;
import software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesResponse;
import software.amazon.awssdk.services.iottwinmaker.model.ListScenesRequest;
import software.amazon.awssdk.services.iottwinmaker.model.ListScenesResponse;
import software.amazon.awssdk.services.iottwinmaker.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesRequest;
import software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesResponse;
import software.amazon.awssdk.services.iottwinmaker.model.TagResourceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.TagResourceResponse;
import software.amazon.awssdk.services.iottwinmaker.model.UntagResourceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.UntagResourceResponse;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateComponentTypeRequest;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateComponentTypeResponse;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateEntityRequest;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateEntityResponse;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateSceneRequest;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateSceneResponse;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateWorkspaceRequest;
import software.amazon.awssdk.services.iottwinmaker.model.UpdateWorkspaceResponse;
import software.amazon.awssdk.services.iottwinmaker.paginators.GetPropertyValueHistoryPublisher;
import software.amazon.awssdk.services.iottwinmaker.paginators.ListComponentTypesPublisher;
import software.amazon.awssdk.services.iottwinmaker.paginators.ListEntitiesPublisher;
import software.amazon.awssdk.services.iottwinmaker.paginators.ListScenesPublisher;
import software.amazon.awssdk.services.iottwinmaker.paginators.ListWorkspacesPublisher;
/**
* Service client for accessing AWS IoT TwinMaker asynchronously. This can be created using the static
* {@link #builder()} method.
*
*
* IoT TwinMaker is a service that enables you to build operational digital twins of physical systems. IoT TwinMaker
* overlays measurements and analysis from real-world sensors, cameras, and enterprise applications so you can create
* data visualizations to monitor your physical factory, building, or industrial plant. You can use this real-world data
* to monitor operations and diagnose and repair errors.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface IoTTwinMakerAsyncClient extends SdkClient {
String SERVICE_NAME = "iottwinmaker";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "iottwinmaker";
/**
* Create a {@link IoTTwinMakerAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static IoTTwinMakerAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link IoTTwinMakerAsyncClient}.
*/
static IoTTwinMakerAsyncClientBuilder builder() {
return new DefaultIoTTwinMakerAsyncClientBuilder();
}
/**
*
* Sets values for multiple time series properties.
*
*
* @param batchPutPropertyValuesRequest
* @return A Java Future containing the result of the BatchPutPropertyValues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.BatchPutPropertyValues
* @see AWS API Documentation
*/
default CompletableFuture batchPutPropertyValues(
BatchPutPropertyValuesRequest batchPutPropertyValuesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sets values for multiple time series properties.
*
*
*
* This is a convenience which creates an instance of the {@link BatchPutPropertyValuesRequest.Builder} avoiding the
* need to create one manually via {@link BatchPutPropertyValuesRequest#builder()}
*
*
* @param batchPutPropertyValuesRequest
* A {@link Consumer} that will call methods on {@link BatchPutPropertyValuesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the BatchPutPropertyValues operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.BatchPutPropertyValues
* @see AWS API Documentation
*/
default CompletableFuture batchPutPropertyValues(
Consumer batchPutPropertyValuesRequest) {
return batchPutPropertyValues(BatchPutPropertyValuesRequest.builder().applyMutation(batchPutPropertyValuesRequest)
.build());
}
/**
*
* Creates a component type.
*
*
* @param createComponentTypeRequest
* @return A Java Future containing the result of the CreateComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateComponentType
* @see AWS API Documentation
*/
default CompletableFuture createComponentType(
CreateComponentTypeRequest createComponentTypeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a component type.
*
*
*
* This is a convenience which creates an instance of the {@link CreateComponentTypeRequest.Builder} avoiding the
* need to create one manually via {@link CreateComponentTypeRequest#builder()}
*
*
* @param createComponentTypeRequest
* A {@link Consumer} that will call methods on {@link CreateComponentTypeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the CreateComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateComponentType
* @see AWS API Documentation
*/
default CompletableFuture createComponentType(
Consumer createComponentTypeRequest) {
return createComponentType(CreateComponentTypeRequest.builder().applyMutation(createComponentTypeRequest).build());
}
/**
*
* Creates an entity.
*
*
* @param createEntityRequest
* @return A Java Future containing the result of the CreateEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateEntity
* @see AWS API
* Documentation
*/
default CompletableFuture createEntity(CreateEntityRequest createEntityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an entity.
*
*
*
* This is a convenience which creates an instance of the {@link CreateEntityRequest.Builder} avoiding the need to
* create one manually via {@link CreateEntityRequest#builder()}
*
*
* @param createEntityRequest
* A {@link Consumer} that will call methods on {@link CreateEntityRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateEntity
* @see AWS API
* Documentation
*/
default CompletableFuture createEntity(Consumer createEntityRequest) {
return createEntity(CreateEntityRequest.builder().applyMutation(createEntityRequest).build());
}
/**
*
* Creates a scene.
*
*
* @param createSceneRequest
* @return A Java Future containing the result of the CreateScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateScene
* @see AWS API
* Documentation
*/
default CompletableFuture createScene(CreateSceneRequest createSceneRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a scene.
*
*
*
* This is a convenience which creates an instance of the {@link CreateSceneRequest.Builder} avoiding the need to
* create one manually via {@link CreateSceneRequest#builder()}
*
*
* @param createSceneRequest
* A {@link Consumer} that will call methods on {@link CreateSceneRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateScene
* @see AWS API
* Documentation
*/
default CompletableFuture createScene(Consumer createSceneRequest) {
return createScene(CreateSceneRequest.builder().applyMutation(createSceneRequest).build());
}
/**
*
* Creates a workplace.
*
*
* @param createWorkspaceRequest
* @return A Java Future containing the result of the CreateWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateWorkspace
* @see AWS
* API Documentation
*/
default CompletableFuture createWorkspace(CreateWorkspaceRequest createWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a workplace.
*
*
*
* This is a convenience which creates an instance of the {@link CreateWorkspaceRequest.Builder} avoiding the need
* to create one manually via {@link CreateWorkspaceRequest#builder()}
*
*
* @param createWorkspaceRequest
* A {@link Consumer} that will call methods on {@link CreateWorkspaceRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.CreateWorkspace
* @see AWS
* API Documentation
*/
default CompletableFuture createWorkspace(
Consumer createWorkspaceRequest) {
return createWorkspace(CreateWorkspaceRequest.builder().applyMutation(createWorkspaceRequest).build());
}
/**
*
* Deletes a component type.
*
*
* @param deleteComponentTypeRequest
* @return A Java Future containing the result of the DeleteComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteComponentType
* @see AWS API Documentation
*/
default CompletableFuture deleteComponentType(
DeleteComponentTypeRequest deleteComponentTypeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a component type.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteComponentTypeRequest.Builder} avoiding the
* need to create one manually via {@link DeleteComponentTypeRequest#builder()}
*
*
* @param deleteComponentTypeRequest
* A {@link Consumer} that will call methods on {@link DeleteComponentTypeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeleteComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteComponentType
* @see AWS API Documentation
*/
default CompletableFuture deleteComponentType(
Consumer deleteComponentTypeRequest) {
return deleteComponentType(DeleteComponentTypeRequest.builder().applyMutation(deleteComponentTypeRequest).build());
}
/**
*
* Deletes an entity.
*
*
* @param deleteEntityRequest
* @return A Java Future containing the result of the DeleteEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteEntity
* @see AWS API
* Documentation
*/
default CompletableFuture deleteEntity(DeleteEntityRequest deleteEntityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes an entity.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteEntityRequest.Builder} avoiding the need to
* create one manually via {@link DeleteEntityRequest#builder()}
*
*
* @param deleteEntityRequest
* A {@link Consumer} that will call methods on {@link DeleteEntityRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteEntity
* @see AWS API
* Documentation
*/
default CompletableFuture deleteEntity(Consumer deleteEntityRequest) {
return deleteEntity(DeleteEntityRequest.builder().applyMutation(deleteEntityRequest).build());
}
/**
*
* Deletes a scene.
*
*
* @param deleteSceneRequest
* @return A Java Future containing the result of the DeleteScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteScene
* @see AWS API
* Documentation
*/
default CompletableFuture deleteScene(DeleteSceneRequest deleteSceneRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a scene.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSceneRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSceneRequest#builder()}
*
*
* @param deleteSceneRequest
* A {@link Consumer} that will call methods on {@link DeleteSceneRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteScene
* @see AWS API
* Documentation
*/
default CompletableFuture deleteScene(Consumer deleteSceneRequest) {
return deleteScene(DeleteSceneRequest.builder().applyMutation(deleteSceneRequest).build());
}
/**
*
* Deletes a workspace.
*
*
* @param deleteWorkspaceRequest
* @return A Java Future containing the result of the DeleteWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteWorkspace
* @see AWS
* API Documentation
*/
default CompletableFuture deleteWorkspace(DeleteWorkspaceRequest deleteWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteWorkspaceRequest.Builder} avoiding the need
* to create one manually via {@link DeleteWorkspaceRequest#builder()}
*
*
* @param deleteWorkspaceRequest
* A {@link Consumer} that will call methods on {@link DeleteWorkspaceRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.DeleteWorkspace
* @see AWS
* API Documentation
*/
default CompletableFuture deleteWorkspace(
Consumer deleteWorkspaceRequest) {
return deleteWorkspace(DeleteWorkspaceRequest.builder().applyMutation(deleteWorkspaceRequest).build());
}
/**
*
* Retrieves information about a component type.
*
*
* @param getComponentTypeRequest
* @return A Java Future containing the result of the GetComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetComponentType
* @see AWS
* API Documentation
*/
default CompletableFuture getComponentType(GetComponentTypeRequest getComponentTypeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about a component type.
*
*
*
* This is a convenience which creates an instance of the {@link GetComponentTypeRequest.Builder} avoiding the need
* to create one manually via {@link GetComponentTypeRequest#builder()}
*
*
* @param getComponentTypeRequest
* A {@link Consumer} that will call methods on {@link GetComponentTypeRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetComponentType
* @see AWS
* API Documentation
*/
default CompletableFuture getComponentType(
Consumer getComponentTypeRequest) {
return getComponentType(GetComponentTypeRequest.builder().applyMutation(getComponentTypeRequest).build());
}
/**
*
* Retrieves information about an entity.
*
*
* @param getEntityRequest
* @return A Java Future containing the result of the GetEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetEntity
* @see AWS API
* Documentation
*/
default CompletableFuture getEntity(GetEntityRequest getEntityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about an entity.
*
*
*
* This is a convenience which creates an instance of the {@link GetEntityRequest.Builder} avoiding the need to
* create one manually via {@link GetEntityRequest#builder()}
*
*
* @param getEntityRequest
* A {@link Consumer} that will call methods on {@link GetEntityRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetEntity
* @see AWS API
* Documentation
*/
default CompletableFuture getEntity(Consumer getEntityRequest) {
return getEntity(GetEntityRequest.builder().applyMutation(getEntityRequest).build());
}
/**
*
* Gets the property values for a component, component type, entity, or workspace.
*
*
* You must specify a value for either componentName
, componentTypeId
,
* entityId
, or workspaceId
.
*
*
* @param getPropertyValueRequest
* @return A Java Future containing the result of the GetPropertyValue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ConnectorFailureException The connector failed.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConnectorTimeoutException The connector timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetPropertyValue
* @see AWS
* API Documentation
*/
default CompletableFuture getPropertyValue(GetPropertyValueRequest getPropertyValueRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the property values for a component, component type, entity, or workspace.
*
*
* You must specify a value for either componentName
, componentTypeId
,
* entityId
, or workspaceId
.
*
*
*
* This is a convenience which creates an instance of the {@link GetPropertyValueRequest.Builder} avoiding the need
* to create one manually via {@link GetPropertyValueRequest#builder()}
*
*
* @param getPropertyValueRequest
* A {@link Consumer} that will call methods on {@link GetPropertyValueRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetPropertyValue operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ConnectorFailureException The connector failed.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConnectorTimeoutException The connector timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetPropertyValue
* @see AWS
* API Documentation
*/
default CompletableFuture getPropertyValue(
Consumer getPropertyValueRequest) {
return getPropertyValue(GetPropertyValueRequest.builder().applyMutation(getPropertyValueRequest).build());
}
/**
*
* Retrieves information about the history of a time series property value for a component, component type, entity,
* or workspace.
*
*
* You must specify a value for workspaceId
. For entity-specific queries, specify values for
* componentName
and entityId
. For cross-entity quries, specify a value for
* componentTypeId
.
*
*
* @param getPropertyValueHistoryRequest
* @return A Java Future containing the result of the GetPropertyValueHistory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ConnectorFailureException The connector failed.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConnectorTimeoutException The connector timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetPropertyValueHistory
* @see AWS API Documentation
*/
default CompletableFuture getPropertyValueHistory(
GetPropertyValueHistoryRequest getPropertyValueHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the history of a time series property value for a component, component type, entity,
* or workspace.
*
*
* You must specify a value for workspaceId
. For entity-specific queries, specify values for
* componentName
and entityId
. For cross-entity quries, specify a value for
* componentTypeId
.
*
*
*
* This is a convenience which creates an instance of the {@link GetPropertyValueHistoryRequest.Builder} avoiding
* the need to create one manually via {@link GetPropertyValueHistoryRequest#builder()}
*
*
* @param getPropertyValueHistoryRequest
* A {@link Consumer} that will call methods on {@link GetPropertyValueHistoryRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetPropertyValueHistory operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ConnectorFailureException The connector failed.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConnectorTimeoutException The connector timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetPropertyValueHistory
* @see AWS API Documentation
*/
default CompletableFuture getPropertyValueHistory(
Consumer getPropertyValueHistoryRequest) {
return getPropertyValueHistory(GetPropertyValueHistoryRequest.builder().applyMutation(getPropertyValueHistoryRequest)
.build());
}
/**
*
* Retrieves information about the history of a time series property value for a component, component type, entity,
* or workspace.
*
*
* You must specify a value for workspaceId
. For entity-specific queries, specify values for
* componentName
and entityId
. For cross-entity quries, specify a value for
* componentTypeId
.
*
*
*
* This is a variant of
* {@link #getPropertyValueHistory(software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.GetPropertyValueHistoryPublisher publisher = client.getPropertyValueHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.GetPropertyValueHistoryPublisher publisher = client.getPropertyValueHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getPropertyValueHistory(software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryRequest)}
* operation.
*
*
* @param getPropertyValueHistoryRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ConnectorFailureException The connector failed.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConnectorTimeoutException The connector timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetPropertyValueHistory
* @see AWS API Documentation
*/
default GetPropertyValueHistoryPublisher getPropertyValueHistoryPaginator(
GetPropertyValueHistoryRequest getPropertyValueHistoryRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about the history of a time series property value for a component, component type, entity,
* or workspace.
*
*
* You must specify a value for workspaceId
. For entity-specific queries, specify values for
* componentName
and entityId
. For cross-entity quries, specify a value for
* componentTypeId
.
*
*
*
* This is a variant of
* {@link #getPropertyValueHistory(software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.GetPropertyValueHistoryPublisher publisher = client.getPropertyValueHistoryPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.GetPropertyValueHistoryPublisher publisher = client.getPropertyValueHistoryPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #getPropertyValueHistory(software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link GetPropertyValueHistoryRequest.Builder} avoiding
* the need to create one manually via {@link GetPropertyValueHistoryRequest#builder()}
*
*
* @param getPropertyValueHistoryRequest
* A {@link Consumer} that will call methods on {@link GetPropertyValueHistoryRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ConnectorFailureException The connector failed.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConnectorTimeoutException The connector timed out.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetPropertyValueHistory
* @see AWS API Documentation
*/
default GetPropertyValueHistoryPublisher getPropertyValueHistoryPaginator(
Consumer getPropertyValueHistoryRequest) {
return getPropertyValueHistoryPaginator(GetPropertyValueHistoryRequest.builder()
.applyMutation(getPropertyValueHistoryRequest).build());
}
/**
*
* Retrieves information about a scene.
*
*
* @param getSceneRequest
* @return A Java Future containing the result of the GetScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetScene
* @see AWS API
* Documentation
*/
default CompletableFuture getScene(GetSceneRequest getSceneRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about a scene.
*
*
*
* This is a convenience which creates an instance of the {@link GetSceneRequest.Builder} avoiding the need to
* create one manually via {@link GetSceneRequest#builder()}
*
*
* @param getSceneRequest
* A {@link Consumer} that will call methods on {@link GetSceneRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetScene
* @see AWS API
* Documentation
*/
default CompletableFuture getScene(Consumer getSceneRequest) {
return getScene(GetSceneRequest.builder().applyMutation(getSceneRequest).build());
}
/**
*
* Retrieves information about a workspace.
*
*
* @param getWorkspaceRequest
* @return A Java Future containing the result of the GetWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture getWorkspace(GetWorkspaceRequest getWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link GetWorkspaceRequest.Builder} avoiding the need to
* create one manually via {@link GetWorkspaceRequest#builder()}
*
*
* @param getWorkspaceRequest
* A {@link Consumer} that will call methods on {@link GetWorkspaceRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.GetWorkspace
* @see AWS API
* Documentation
*/
default CompletableFuture getWorkspace(Consumer getWorkspaceRequest) {
return getWorkspace(GetWorkspaceRequest.builder().applyMutation(getWorkspaceRequest).build());
}
/**
*
* Lists all component types in a workspace.
*
*
* @param listComponentTypesRequest
* @return A Java Future containing the result of the ListComponentTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListComponentTypes
* @see AWS API Documentation
*/
default CompletableFuture listComponentTypes(ListComponentTypesRequest listComponentTypesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all component types in a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link ListComponentTypesRequest.Builder} avoiding the
* need to create one manually via {@link ListComponentTypesRequest#builder()}
*
*
* @param listComponentTypesRequest
* A {@link Consumer} that will call methods on {@link ListComponentTypesRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListComponentTypes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListComponentTypes
* @see AWS API Documentation
*/
default CompletableFuture listComponentTypes(
Consumer listComponentTypesRequest) {
return listComponentTypes(ListComponentTypesRequest.builder().applyMutation(listComponentTypesRequest).build());
}
/**
*
* Lists all component types in a workspace.
*
*
*
* This is a variant of
* {@link #listComponentTypes(software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListComponentTypesPublisher publisher = client.listComponentTypesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListComponentTypesPublisher publisher = client.listComponentTypesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComponentTypes(software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesRequest)}
* operation.
*
*
* @param listComponentTypesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListComponentTypes
* @see AWS API Documentation
*/
default ListComponentTypesPublisher listComponentTypesPaginator(ListComponentTypesRequest listComponentTypesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all component types in a workspace.
*
*
*
* This is a variant of
* {@link #listComponentTypes(software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListComponentTypesPublisher publisher = client.listComponentTypesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListComponentTypesPublisher publisher = client.listComponentTypesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listComponentTypes(software.amazon.awssdk.services.iottwinmaker.model.ListComponentTypesRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListComponentTypesRequest.Builder} avoiding the
* need to create one manually via {@link ListComponentTypesRequest#builder()}
*
*
* @param listComponentTypesRequest
* A {@link Consumer} that will call methods on {@link ListComponentTypesRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListComponentTypes
* @see AWS API Documentation
*/
default ListComponentTypesPublisher listComponentTypesPaginator(
Consumer listComponentTypesRequest) {
return listComponentTypesPaginator(ListComponentTypesRequest.builder().applyMutation(listComponentTypesRequest).build());
}
/**
*
* Lists all entities in a workspace.
*
*
* @param listEntitiesRequest
* @return A Java Future containing the result of the ListEntities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListEntities
* @see AWS API
* Documentation
*/
default CompletableFuture listEntities(ListEntitiesRequest listEntitiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all entities in a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link ListEntitiesRequest.Builder} avoiding the need to
* create one manually via {@link ListEntitiesRequest#builder()}
*
*
* @param listEntitiesRequest
* A {@link Consumer} that will call methods on {@link ListEntitiesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListEntities operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListEntities
* @see AWS API
* Documentation
*/
default CompletableFuture listEntities(Consumer listEntitiesRequest) {
return listEntities(ListEntitiesRequest.builder().applyMutation(listEntitiesRequest).build());
}
/**
*
* Lists all entities in a workspace.
*
*
*
* This is a variant of
* {@link #listEntities(software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListEntitiesPublisher publisher = client.listEntitiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListEntitiesPublisher publisher = client.listEntitiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEntities(software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesRequest)} operation.
*
*
* @param listEntitiesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListEntities
* @see AWS API
* Documentation
*/
default ListEntitiesPublisher listEntitiesPaginator(ListEntitiesRequest listEntitiesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all entities in a workspace.
*
*
*
* This is a variant of
* {@link #listEntities(software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListEntitiesPublisher publisher = client.listEntitiesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListEntitiesPublisher publisher = client.listEntitiesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listEntities(software.amazon.awssdk.services.iottwinmaker.model.ListEntitiesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListEntitiesRequest.Builder} avoiding the need to
* create one manually via {@link ListEntitiesRequest#builder()}
*
*
* @param listEntitiesRequest
* A {@link Consumer} that will call methods on {@link ListEntitiesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListEntities
* @see AWS API
* Documentation
*/
default ListEntitiesPublisher listEntitiesPaginator(Consumer listEntitiesRequest) {
return listEntitiesPaginator(ListEntitiesRequest.builder().applyMutation(listEntitiesRequest).build());
}
/**
*
* Lists all scenes in a workspace.
*
*
* @param listScenesRequest
* @return A Java Future containing the result of the ListScenes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListScenes
* @see AWS API
* Documentation
*/
default CompletableFuture listScenes(ListScenesRequest listScenesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all scenes in a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link ListScenesRequest.Builder} avoiding the need to
* create one manually via {@link ListScenesRequest#builder()}
*
*
* @param listScenesRequest
* A {@link Consumer} that will call methods on {@link ListScenesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListScenes operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListScenes
* @see AWS API
* Documentation
*/
default CompletableFuture listScenes(Consumer listScenesRequest) {
return listScenes(ListScenesRequest.builder().applyMutation(listScenesRequest).build());
}
/**
*
* Lists all scenes in a workspace.
*
*
*
* This is a variant of {@link #listScenes(software.amazon.awssdk.services.iottwinmaker.model.ListScenesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListScenesPublisher publisher = client.listScenesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListScenesPublisher publisher = client.listScenesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListScenesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listScenes(software.amazon.awssdk.services.iottwinmaker.model.ListScenesRequest)} operation.
*
*
* @param listScenesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListScenes
* @see AWS API
* Documentation
*/
default ListScenesPublisher listScenesPaginator(ListScenesRequest listScenesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all scenes in a workspace.
*
*
*
* This is a variant of {@link #listScenes(software.amazon.awssdk.services.iottwinmaker.model.ListScenesRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListScenesPublisher publisher = client.listScenesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListScenesPublisher publisher = client.listScenesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListScenesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listScenes(software.amazon.awssdk.services.iottwinmaker.model.ListScenesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListScenesRequest.Builder} avoiding the need to
* create one manually via {@link ListScenesRequest#builder()}
*
*
* @param listScenesRequest
* A {@link Consumer} that will call methods on {@link ListScenesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListScenes
* @see AWS API
* Documentation
*/
default ListScenesPublisher listScenesPaginator(Consumer listScenesRequest) {
return listScenesPaginator(ListScenesRequest.builder().applyMutation(listScenesRequest).build());
}
/**
*
* Lists all tags associated with a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Lists all tags associated with a resource.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListTagsForResource
* @see AWS API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Retrieves information about workspaces in the current account.
*
*
* @param listWorkspacesRequest
* @return A Java Future containing the result of the ListWorkspaces operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListWorkspaces
* @see AWS
* API Documentation
*/
default CompletableFuture listWorkspaces(ListWorkspacesRequest listWorkspacesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about workspaces in the current account.
*
*
*
* This is a convenience which creates an instance of the {@link ListWorkspacesRequest.Builder} avoiding the need to
* create one manually via {@link ListWorkspacesRequest#builder()}
*
*
* @param listWorkspacesRequest
* A {@link Consumer} that will call methods on {@link ListWorkspacesRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListWorkspaces operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListWorkspaces
* @see AWS
* API Documentation
*/
default CompletableFuture listWorkspaces(Consumer listWorkspacesRequest) {
return listWorkspaces(ListWorkspacesRequest.builder().applyMutation(listWorkspacesRequest).build());
}
/**
*
* Retrieves information about workspaces in the current account.
*
*
*
* This is a variant of
* {@link #listWorkspaces(software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listWorkspaces(software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesRequest)} operation.
*
*
* @param listWorkspacesRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListWorkspaces
* @see AWS
* API Documentation
*/
default ListWorkspacesPublisher listWorkspacesPaginator(ListWorkspacesRequest listWorkspacesRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Retrieves information about workspaces in the current account.
*
*
*
* This is a variant of
* {@link #listWorkspaces(software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.iottwinmaker.paginators.ListWorkspacesPublisher publisher = client.listWorkspacesPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listWorkspaces(software.amazon.awssdk.services.iottwinmaker.model.ListWorkspacesRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListWorkspacesRequest.Builder} avoiding the need to
* create one manually via {@link ListWorkspacesRequest#builder()}
*
*
* @param listWorkspacesRequest
* A {@link Consumer} that will call methods on {@link ListWorkspacesRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.ListWorkspaces
* @see AWS
* API Documentation
*/
default ListWorkspacesPublisher listWorkspacesPaginator(Consumer listWorkspacesRequest) {
return listWorkspacesPaginator(ListWorkspacesRequest.builder().applyMutation(listWorkspacesRequest).build());
}
/**
*
* Adds tags to a resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyTagsException The number of tags exceeds the limit.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds tags to a resource.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - TooManyTagsException The number of tags exceeds the limit.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from a resource.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UntagResource
* @see AWS
* API Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates information in a component type.
*
*
* @param updateComponentTypeRequest
* @return A Java Future containing the result of the UpdateComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateComponentType
* @see AWS API Documentation
*/
default CompletableFuture updateComponentType(
UpdateComponentTypeRequest updateComponentTypeRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates information in a component type.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateComponentTypeRequest.Builder} avoiding the
* need to create one manually via {@link UpdateComponentTypeRequest#builder()}
*
*
* @param updateComponentTypeRequest
* A {@link Consumer} that will call methods on {@link UpdateComponentTypeRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the UpdateComponentType operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateComponentType
* @see AWS API Documentation
*/
default CompletableFuture updateComponentType(
Consumer updateComponentTypeRequest) {
return updateComponentType(UpdateComponentTypeRequest.builder().applyMutation(updateComponentTypeRequest).build());
}
/**
*
* Updates an entity.
*
*
* @param updateEntityRequest
* @return A Java Future containing the result of the UpdateEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateEntity
* @see AWS API
* Documentation
*/
default CompletableFuture updateEntity(UpdateEntityRequest updateEntityRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates an entity.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateEntityRequest.Builder} avoiding the need to
* create one manually via {@link UpdateEntityRequest#builder()}
*
*
* @param updateEntityRequest
* A {@link Consumer} that will call methods on {@link UpdateEntityRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateEntity operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ConflictException A conflict occurred.
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateEntity
* @see AWS API
* Documentation
*/
default CompletableFuture updateEntity(Consumer updateEntityRequest) {
return updateEntity(UpdateEntityRequest.builder().applyMutation(updateEntityRequest).build());
}
/**
*
* Updates a scene.
*
*
* @param updateSceneRequest
* @return A Java Future containing the result of the UpdateScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateScene
* @see AWS API
* Documentation
*/
default CompletableFuture updateScene(UpdateSceneRequest updateSceneRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a scene.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateSceneRequest.Builder} avoiding the need to
* create one manually via {@link UpdateSceneRequest#builder()}
*
*
* @param updateSceneRequest
* A {@link Consumer} that will call methods on {@link UpdateSceneRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateScene operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateScene
* @see AWS API
* Documentation
*/
default CompletableFuture updateScene(Consumer updateSceneRequest) {
return updateScene(UpdateSceneRequest.builder().applyMutation(updateSceneRequest).build());
}
/**
*
* Updates a workspace.
*
*
* @param updateWorkspaceRequest
* @return A Java Future containing the result of the UpdateWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateWorkspace
* @see AWS
* API Documentation
*/
default CompletableFuture updateWorkspace(UpdateWorkspaceRequest updateWorkspaceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a workspace.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateWorkspaceRequest.Builder} avoiding the need
* to create one manually via {@link UpdateWorkspaceRequest#builder()}
*
*
* @param updateWorkspaceRequest
* A {@link Consumer} that will call methods on {@link UpdateWorkspaceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateWorkspace operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException An unexpected error has occurred.
* - AccessDeniedException Access is denied.
* - ResourceNotFoundException The resource wasn't found.
* - ThrottlingException The rate exceeds the limit.
* - ValidationException Failed
* - ServiceQuotaExceededException The service quota was exceeded.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IoTTwinMakerException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IoTTwinMakerAsyncClient.UpdateWorkspace
* @see AWS
* API Documentation
*/
default CompletableFuture updateWorkspace(
Consumer updateWorkspaceRequest) {
return updateWorkspace(UpdateWorkspaceRequest.builder().applyMutation(updateWorkspaceRequest).build());
}
}