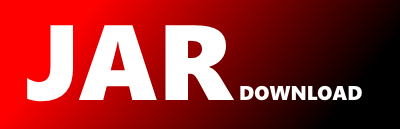
software.amazon.awssdk.services.iottwinmaker.model.GetPropertyValueHistoryRequest Maven / Gradle / Ivy
Show all versions of iottwinmaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iottwinmaker.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetPropertyValueHistoryRequest extends IoTTwinMakerRequest implements
ToCopyableBuilder {
private static final SdkField WORKSPACE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("workspaceId").getter(getter(GetPropertyValueHistoryRequest::workspaceId))
.setter(setter(Builder::workspaceId))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("workspaceId").build()).build();
private static final SdkField ENTITY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("entityId").getter(getter(GetPropertyValueHistoryRequest::entityId)).setter(setter(Builder::entityId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("entityId").build()).build();
private static final SdkField COMPONENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("componentName").getter(getter(GetPropertyValueHistoryRequest::componentName))
.setter(setter(Builder::componentName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("componentName").build()).build();
private static final SdkField COMPONENT_PATH_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("componentPath").getter(getter(GetPropertyValueHistoryRequest::componentPath))
.setter(setter(Builder::componentPath))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("componentPath").build()).build();
private static final SdkField COMPONENT_TYPE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("componentTypeId").getter(getter(GetPropertyValueHistoryRequest::componentTypeId))
.setter(setter(Builder::componentTypeId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("componentTypeId").build()).build();
private static final SdkField> SELECTED_PROPERTIES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("selectedProperties")
.getter(getter(GetPropertyValueHistoryRequest::selectedProperties))
.setter(setter(Builder::selectedProperties))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("selectedProperties").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> PROPERTY_FILTERS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("propertyFilters")
.getter(getter(GetPropertyValueHistoryRequest::propertyFilters))
.setter(setter(Builder::propertyFilters))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("propertyFilters").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(PropertyFilter::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField START_DATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("startDateTime").getter(getter(GetPropertyValueHistoryRequest::startDateTime))
.setter(setter(Builder::startDateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startDateTime").build()).build();
private static final SdkField END_DATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("endDateTime").getter(getter(GetPropertyValueHistoryRequest::endDateTime))
.setter(setter(Builder::endDateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endDateTime").build()).build();
private static final SdkField INTERPOLATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("interpolation")
.getter(getter(GetPropertyValueHistoryRequest::interpolation)).setter(setter(Builder::interpolation))
.constructor(InterpolationParameters::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("interpolation").build()).build();
private static final SdkField NEXT_TOKEN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("nextToken").getter(getter(GetPropertyValueHistoryRequest::nextToken)).setter(setter(Builder::nextToken))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("nextToken").build()).build();
private static final SdkField MAX_RESULTS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("maxResults").getter(getter(GetPropertyValueHistoryRequest::maxResults))
.setter(setter(Builder::maxResults))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("maxResults").build()).build();
private static final SdkField ORDER_BY_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("orderByTime").getter(getter(GetPropertyValueHistoryRequest::orderByTimeAsString))
.setter(setter(Builder::orderByTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("orderByTime").build()).build();
private static final SdkField START_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("startTime").getter(getter(GetPropertyValueHistoryRequest::startTime)).setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build()).build();
private static final SdkField END_TIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("endTime").getter(getter(GetPropertyValueHistoryRequest::endTime)).setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(WORKSPACE_ID_FIELD,
ENTITY_ID_FIELD, COMPONENT_NAME_FIELD, COMPONENT_PATH_FIELD, COMPONENT_TYPE_ID_FIELD, SELECTED_PROPERTIES_FIELD,
PROPERTY_FILTERS_FIELD, START_DATE_TIME_FIELD, END_DATE_TIME_FIELD, INTERPOLATION_FIELD, NEXT_TOKEN_FIELD,
MAX_RESULTS_FIELD, ORDER_BY_TIME_FIELD, START_TIME_FIELD, END_TIME_FIELD));
private final String workspaceId;
private final String entityId;
private final String componentName;
private final String componentPath;
private final String componentTypeId;
private final List selectedProperties;
private final List propertyFilters;
private final Instant startDateTime;
private final Instant endDateTime;
private final InterpolationParameters interpolation;
private final String nextToken;
private final Integer maxResults;
private final String orderByTime;
private final String startTime;
private final String endTime;
private GetPropertyValueHistoryRequest(BuilderImpl builder) {
super(builder);
this.workspaceId = builder.workspaceId;
this.entityId = builder.entityId;
this.componentName = builder.componentName;
this.componentPath = builder.componentPath;
this.componentTypeId = builder.componentTypeId;
this.selectedProperties = builder.selectedProperties;
this.propertyFilters = builder.propertyFilters;
this.startDateTime = builder.startDateTime;
this.endDateTime = builder.endDateTime;
this.interpolation = builder.interpolation;
this.nextToken = builder.nextToken;
this.maxResults = builder.maxResults;
this.orderByTime = builder.orderByTime;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
}
/**
*
* The ID of the workspace.
*
*
* @return The ID of the workspace.
*/
public final String workspaceId() {
return workspaceId;
}
/**
*
* The ID of the entity.
*
*
* @return The ID of the entity.
*/
public final String entityId() {
return entityId;
}
/**
*
* The name of the component.
*
*
* @return The name of the component.
*/
public final String componentName() {
return componentName;
}
/**
*
* This string specifies the path to the composite component, starting from the top-level component.
*
*
* @return This string specifies the path to the composite component, starting from the top-level component.
*/
public final String componentPath() {
return componentPath;
}
/**
*
* The ID of the component type.
*
*
* @return The ID of the component type.
*/
public final String componentTypeId() {
return componentTypeId;
}
/**
* For responses, this returns true if the service returned a value for the SelectedProperties property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSelectedProperties() {
return selectedProperties != null && !(selectedProperties instanceof SdkAutoConstructList);
}
/**
*
* A list of properties whose value histories the request retrieves.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSelectedProperties} method.
*
*
* @return A list of properties whose value histories the request retrieves.
*/
public final List selectedProperties() {
return selectedProperties;
}
/**
* For responses, this returns true if the service returned a value for the PropertyFilters property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasPropertyFilters() {
return propertyFilters != null && !(propertyFilters instanceof SdkAutoConstructList);
}
/**
*
* A list of objects that filter the property value history request.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasPropertyFilters} method.
*
*
* @return A list of objects that filter the property value history request.
*/
public final List propertyFilters() {
return propertyFilters;
}
/**
*
* The date and time of the earliest property value to return.
*
*
* @return The date and time of the earliest property value to return.
* @deprecated This field is deprecated and will throw an error in the future. Use startTime instead.
*/
@Deprecated
public final Instant startDateTime() {
return startDateTime;
}
/**
*
* The date and time of the latest property value to return.
*
*
* @return The date and time of the latest property value to return.
* @deprecated This field is deprecated and will throw an error in the future. Use endTime instead.
*/
@Deprecated
public final Instant endDateTime() {
return endDateTime;
}
/**
*
* An object that specifies the interpolation type and the interval over which to interpolate data.
*
*
* @return An object that specifies the interpolation type and the interval over which to interpolate data.
*/
public final InterpolationParameters interpolation() {
return interpolation;
}
/**
*
* The string that specifies the next page of results.
*
*
* @return The string that specifies the next page of results.
*/
public final String nextToken() {
return nextToken;
}
/**
*
* The maximum number of results to return at one time. The default is 25.
*
*
* Valid Range: Minimum value of 1. Maximum value of 250.
*
*
* @return The maximum number of results to return at one time. The default is 25.
*
* Valid Range: Minimum value of 1. Maximum value of 250.
*/
public final Integer maxResults() {
return maxResults;
}
/**
*
* The time direction to use in the result order.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #orderByTime} will
* return {@link OrderByTime#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #orderByTimeAsString}.
*
*
* @return The time direction to use in the result order.
* @see OrderByTime
*/
public final OrderByTime orderByTime() {
return OrderByTime.fromValue(orderByTime);
}
/**
*
* The time direction to use in the result order.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #orderByTime} will
* return {@link OrderByTime#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #orderByTimeAsString}.
*
*
* @return The time direction to use in the result order.
* @see OrderByTime
*/
public final String orderByTimeAsString() {
return orderByTime;
}
/**
*
* The ISO8601 DateTime of the earliest property value to return.
*
*
* For more information about the ISO8601 DateTime format, see the data type PropertyValue.
*
*
* @return The ISO8601 DateTime of the earliest property value to return.
*
* For more information about the ISO8601 DateTime format, see the data type PropertyValue.
*/
public final String startTime() {
return startTime;
}
/**
*
* The ISO8601 DateTime of the latest property value to return.
*
*
* For more information about the ISO8601 DateTime format, see the data type PropertyValue.
*
*
* @return The ISO8601 DateTime of the latest property value to return.
*
* For more information about the ISO8601 DateTime format, see the data type PropertyValue.
*/
public final String endTime() {
return endTime;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(workspaceId());
hashCode = 31 * hashCode + Objects.hashCode(entityId());
hashCode = 31 * hashCode + Objects.hashCode(componentName());
hashCode = 31 * hashCode + Objects.hashCode(componentPath());
hashCode = 31 * hashCode + Objects.hashCode(componentTypeId());
hashCode = 31 * hashCode + Objects.hashCode(hasSelectedProperties() ? selectedProperties() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasPropertyFilters() ? propertyFilters() : null);
hashCode = 31 * hashCode + Objects.hashCode(startDateTime());
hashCode = 31 * hashCode + Objects.hashCode(endDateTime());
hashCode = 31 * hashCode + Objects.hashCode(interpolation());
hashCode = 31 * hashCode + Objects.hashCode(nextToken());
hashCode = 31 * hashCode + Objects.hashCode(maxResults());
hashCode = 31 * hashCode + Objects.hashCode(orderByTimeAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetPropertyValueHistoryRequest)) {
return false;
}
GetPropertyValueHistoryRequest other = (GetPropertyValueHistoryRequest) obj;
return Objects.equals(workspaceId(), other.workspaceId()) && Objects.equals(entityId(), other.entityId())
&& Objects.equals(componentName(), other.componentName())
&& Objects.equals(componentPath(), other.componentPath())
&& Objects.equals(componentTypeId(), other.componentTypeId())
&& hasSelectedProperties() == other.hasSelectedProperties()
&& Objects.equals(selectedProperties(), other.selectedProperties())
&& hasPropertyFilters() == other.hasPropertyFilters()
&& Objects.equals(propertyFilters(), other.propertyFilters())
&& Objects.equals(startDateTime(), other.startDateTime()) && Objects.equals(endDateTime(), other.endDateTime())
&& Objects.equals(interpolation(), other.interpolation()) && Objects.equals(nextToken(), other.nextToken())
&& Objects.equals(maxResults(), other.maxResults())
&& Objects.equals(orderByTimeAsString(), other.orderByTimeAsString())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetPropertyValueHistoryRequest").add("WorkspaceId", workspaceId()).add("EntityId", entityId())
.add("ComponentName", componentName()).add("ComponentPath", componentPath())
.add("ComponentTypeId", componentTypeId())
.add("SelectedProperties", hasSelectedProperties() ? selectedProperties() : null)
.add("PropertyFilters", hasPropertyFilters() ? propertyFilters() : null).add("StartDateTime", startDateTime())
.add("EndDateTime", endDateTime()).add("Interpolation", interpolation()).add("NextToken", nextToken())
.add("MaxResults", maxResults()).add("OrderByTime", orderByTimeAsString()).add("StartTime", startTime())
.add("EndTime", endTime()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "workspaceId":
return Optional.ofNullable(clazz.cast(workspaceId()));
case "entityId":
return Optional.ofNullable(clazz.cast(entityId()));
case "componentName":
return Optional.ofNullable(clazz.cast(componentName()));
case "componentPath":
return Optional.ofNullable(clazz.cast(componentPath()));
case "componentTypeId":
return Optional.ofNullable(clazz.cast(componentTypeId()));
case "selectedProperties":
return Optional.ofNullable(clazz.cast(selectedProperties()));
case "propertyFilters":
return Optional.ofNullable(clazz.cast(propertyFilters()));
case "startDateTime":
return Optional.ofNullable(clazz.cast(startDateTime()));
case "endDateTime":
return Optional.ofNullable(clazz.cast(endDateTime()));
case "interpolation":
return Optional.ofNullable(clazz.cast(interpolation()));
case "nextToken":
return Optional.ofNullable(clazz.cast(nextToken()));
case "maxResults":
return Optional.ofNullable(clazz.cast(maxResults()));
case "orderByTime":
return Optional.ofNullable(clazz.cast(orderByTimeAsString()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* For more information about the ISO8601 DateTime format, see the data type PropertyValue.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder endTime(String endTime);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends IoTTwinMakerRequest.BuilderImpl implements Builder {
private String workspaceId;
private String entityId;
private String componentName;
private String componentPath;
private String componentTypeId;
private List selectedProperties = DefaultSdkAutoConstructList.getInstance();
private List propertyFilters = DefaultSdkAutoConstructList.getInstance();
private Instant startDateTime;
private Instant endDateTime;
private InterpolationParameters interpolation;
private String nextToken;
private Integer maxResults;
private String orderByTime;
private String startTime;
private String endTime;
private BuilderImpl() {
}
private BuilderImpl(GetPropertyValueHistoryRequest model) {
super(model);
workspaceId(model.workspaceId);
entityId(model.entityId);
componentName(model.componentName);
componentPath(model.componentPath);
componentTypeId(model.componentTypeId);
selectedProperties(model.selectedProperties);
propertyFilters(model.propertyFilters);
startDateTime(model.startDateTime);
endDateTime(model.endDateTime);
interpolation(model.interpolation);
nextToken(model.nextToken);
maxResults(model.maxResults);
orderByTime(model.orderByTime);
startTime(model.startTime);
endTime(model.endTime);
}
public final String getWorkspaceId() {
return workspaceId;
}
public final void setWorkspaceId(String workspaceId) {
this.workspaceId = workspaceId;
}
@Override
public final Builder workspaceId(String workspaceId) {
this.workspaceId = workspaceId;
return this;
}
public final String getEntityId() {
return entityId;
}
public final void setEntityId(String entityId) {
this.entityId = entityId;
}
@Override
public final Builder entityId(String entityId) {
this.entityId = entityId;
return this;
}
public final String getComponentName() {
return componentName;
}
public final void setComponentName(String componentName) {
this.componentName = componentName;
}
@Override
public final Builder componentName(String componentName) {
this.componentName = componentName;
return this;
}
public final String getComponentPath() {
return componentPath;
}
public final void setComponentPath(String componentPath) {
this.componentPath = componentPath;
}
@Override
public final Builder componentPath(String componentPath) {
this.componentPath = componentPath;
return this;
}
public final String getComponentTypeId() {
return componentTypeId;
}
public final void setComponentTypeId(String componentTypeId) {
this.componentTypeId = componentTypeId;
}
@Override
public final Builder componentTypeId(String componentTypeId) {
this.componentTypeId = componentTypeId;
return this;
}
public final Collection getSelectedProperties() {
if (selectedProperties instanceof SdkAutoConstructList) {
return null;
}
return selectedProperties;
}
public final void setSelectedProperties(Collection selectedProperties) {
this.selectedProperties = SelectedPropertyListCopier.copy(selectedProperties);
}
@Override
public final Builder selectedProperties(Collection selectedProperties) {
this.selectedProperties = SelectedPropertyListCopier.copy(selectedProperties);
return this;
}
@Override
@SafeVarargs
public final Builder selectedProperties(String... selectedProperties) {
selectedProperties(Arrays.asList(selectedProperties));
return this;
}
public final List getPropertyFilters() {
List result = PropertyFiltersCopier.copyToBuilder(this.propertyFilters);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setPropertyFilters(Collection propertyFilters) {
this.propertyFilters = PropertyFiltersCopier.copyFromBuilder(propertyFilters);
}
@Override
public final Builder propertyFilters(Collection propertyFilters) {
this.propertyFilters = PropertyFiltersCopier.copy(propertyFilters);
return this;
}
@Override
@SafeVarargs
public final Builder propertyFilters(PropertyFilter... propertyFilters) {
propertyFilters(Arrays.asList(propertyFilters));
return this;
}
@Override
@SafeVarargs
public final Builder propertyFilters(Consumer... propertyFilters) {
propertyFilters(Stream.of(propertyFilters).map(c -> PropertyFilter.builder().applyMutation(c).build())
.collect(Collectors.toList()));
return this;
}
@Deprecated
public final Instant getStartDateTime() {
return startDateTime;
}
@Deprecated
public final void setStartDateTime(Instant startDateTime) {
this.startDateTime = startDateTime;
}
@Override
@Deprecated
public final Builder startDateTime(Instant startDateTime) {
this.startDateTime = startDateTime;
return this;
}
@Deprecated
public final Instant getEndDateTime() {
return endDateTime;
}
@Deprecated
public final void setEndDateTime(Instant endDateTime) {
this.endDateTime = endDateTime;
}
@Override
@Deprecated
public final Builder endDateTime(Instant endDateTime) {
this.endDateTime = endDateTime;
return this;
}
public final InterpolationParameters.Builder getInterpolation() {
return interpolation != null ? interpolation.toBuilder() : null;
}
public final void setInterpolation(InterpolationParameters.BuilderImpl interpolation) {
this.interpolation = interpolation != null ? interpolation.build() : null;
}
@Override
public final Builder interpolation(InterpolationParameters interpolation) {
this.interpolation = interpolation;
return this;
}
public final String getNextToken() {
return nextToken;
}
public final void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
@Override
public final Builder nextToken(String nextToken) {
this.nextToken = nextToken;
return this;
}
public final Integer getMaxResults() {
return maxResults;
}
public final void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
@Override
public final Builder maxResults(Integer maxResults) {
this.maxResults = maxResults;
return this;
}
public final String getOrderByTime() {
return orderByTime;
}
public final void setOrderByTime(String orderByTime) {
this.orderByTime = orderByTime;
}
@Override
public final Builder orderByTime(String orderByTime) {
this.orderByTime = orderByTime;
return this;
}
@Override
public final Builder orderByTime(OrderByTime orderByTime) {
this.orderByTime(orderByTime == null ? null : orderByTime.toString());
return this;
}
public final String getStartTime() {
return startTime;
}
public final void setStartTime(String startTime) {
this.startTime = startTime;
}
@Override
public final Builder startTime(String startTime) {
this.startTime = startTime;
return this;
}
public final String getEndTime() {
return endTime;
}
public final void setEndTime(String endTime) {
this.endTime = endTime;
}
@Override
public final Builder endTime(String endTime) {
this.endTime = endTime;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public GetPropertyValueHistoryRequest build() {
return new GetPropertyValueHistoryRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}