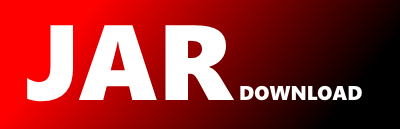
software.amazon.awssdk.services.iottwinmaker.model.MetadataTransferJobProgress Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iottwinmaker.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The metadata transfer job's progress.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class MetadataTransferJobProgress implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField TOTAL_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("totalCount").getter(getter(MetadataTransferJobProgress::totalCount)).setter(setter(Builder::totalCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("totalCount").build()).build();
private static final SdkField SUCCEEDED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("succeededCount").getter(getter(MetadataTransferJobProgress::succeededCount))
.setter(setter(Builder::succeededCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("succeededCount").build()).build();
private static final SdkField SKIPPED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("skippedCount").getter(getter(MetadataTransferJobProgress::skippedCount))
.setter(setter(Builder::skippedCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("skippedCount").build()).build();
private static final SdkField FAILED_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("failedCount").getter(getter(MetadataTransferJobProgress::failedCount))
.setter(setter(Builder::failedCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failedCount").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(TOTAL_COUNT_FIELD,
SUCCEEDED_COUNT_FIELD, SKIPPED_COUNT_FIELD, FAILED_COUNT_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("totalCount", TOTAL_COUNT_FIELD);
put("succeededCount", SUCCEEDED_COUNT_FIELD);
put("skippedCount", SKIPPED_COUNT_FIELD);
put("failedCount", FAILED_COUNT_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Integer totalCount;
private final Integer succeededCount;
private final Integer skippedCount;
private final Integer failedCount;
private MetadataTransferJobProgress(BuilderImpl builder) {
this.totalCount = builder.totalCount;
this.succeededCount = builder.succeededCount;
this.skippedCount = builder.skippedCount;
this.failedCount = builder.failedCount;
}
/**
*
* The total count. [of what]
*
*
* @return The total count. [of what]
*/
public final Integer totalCount() {
return totalCount;
}
/**
*
* The succeeded count.
*
*
* @return The succeeded count.
*/
public final Integer succeededCount() {
return succeededCount;
}
/**
*
* The skipped count.
*
*
* @return The skipped count.
*/
public final Integer skippedCount() {
return skippedCount;
}
/**
*
* The failed count.
*
*
* @return The failed count.
*/
public final Integer failedCount() {
return failedCount;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(totalCount());
hashCode = 31 * hashCode + Objects.hashCode(succeededCount());
hashCode = 31 * hashCode + Objects.hashCode(skippedCount());
hashCode = 31 * hashCode + Objects.hashCode(failedCount());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof MetadataTransferJobProgress)) {
return false;
}
MetadataTransferJobProgress other = (MetadataTransferJobProgress) obj;
return Objects.equals(totalCount(), other.totalCount()) && Objects.equals(succeededCount(), other.succeededCount())
&& Objects.equals(skippedCount(), other.skippedCount()) && Objects.equals(failedCount(), other.failedCount());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("MetadataTransferJobProgress").add("TotalCount", totalCount())
.add("SucceededCount", succeededCount()).add("SkippedCount", skippedCount()).add("FailedCount", failedCount())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "totalCount":
return Optional.ofNullable(clazz.cast(totalCount()));
case "succeededCount":
return Optional.ofNullable(clazz.cast(succeededCount()));
case "skippedCount":
return Optional.ofNullable(clazz.cast(skippedCount()));
case "failedCount":
return Optional.ofNullable(clazz.cast(failedCount()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy