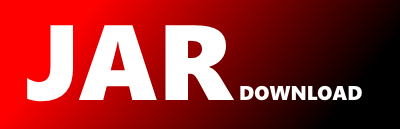
software.amazon.awssdk.services.iottwinmaker.model.PricingPlan Maven / Gradle / Ivy
Show all versions of iottwinmaker Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.iottwinmaker.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The pricing plan.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class PricingPlan implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField BILLABLE_ENTITY_COUNT_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("billableEntityCount").getter(getter(PricingPlan::billableEntityCount))
.setter(setter(Builder::billableEntityCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("billableEntityCount").build())
.build();
private static final SdkField BUNDLE_INFORMATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("bundleInformation")
.getter(getter(PricingPlan::bundleInformation)).setter(setter(Builder::bundleInformation))
.constructor(BundleInformation::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("bundleInformation").build()).build();
private static final SdkField EFFECTIVE_DATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("effectiveDateTime").getter(getter(PricingPlan::effectiveDateTime))
.setter(setter(Builder::effectiveDateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("effectiveDateTime").build()).build();
private static final SdkField PRICING_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("pricingMode").getter(getter(PricingPlan::pricingModeAsString)).setter(setter(Builder::pricingMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("pricingMode").build()).build();
private static final SdkField UPDATE_DATE_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("updateDateTime").getter(getter(PricingPlan::updateDateTime)).setter(setter(Builder::updateDateTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updateDateTime").build()).build();
private static final SdkField UPDATE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("updateReason").getter(getter(PricingPlan::updateReasonAsString)).setter(setter(Builder::updateReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("updateReason").build()).build();
private static final List> SDK_FIELDS = Collections
.unmodifiableList(Arrays.asList(BILLABLE_ENTITY_COUNT_FIELD, BUNDLE_INFORMATION_FIELD, EFFECTIVE_DATE_TIME_FIELD,
PRICING_MODE_FIELD, UPDATE_DATE_TIME_FIELD, UPDATE_REASON_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("billableEntityCount", BILLABLE_ENTITY_COUNT_FIELD);
put("bundleInformation", BUNDLE_INFORMATION_FIELD);
put("effectiveDateTime", EFFECTIVE_DATE_TIME_FIELD);
put("pricingMode", PRICING_MODE_FIELD);
put("updateDateTime", UPDATE_DATE_TIME_FIELD);
put("updateReason", UPDATE_REASON_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final Long billableEntityCount;
private final BundleInformation bundleInformation;
private final Instant effectiveDateTime;
private final String pricingMode;
private final Instant updateDateTime;
private final String updateReason;
private PricingPlan(BuilderImpl builder) {
this.billableEntityCount = builder.billableEntityCount;
this.bundleInformation = builder.bundleInformation;
this.effectiveDateTime = builder.effectiveDateTime;
this.pricingMode = builder.pricingMode;
this.updateDateTime = builder.updateDateTime;
this.updateReason = builder.updateReason;
}
/**
*
* The billable entity count.
*
*
* @return The billable entity count.
*/
public final Long billableEntityCount() {
return billableEntityCount;
}
/**
*
* The pricing plan's bundle information.
*
*
* @return The pricing plan's bundle information.
*/
public final BundleInformation bundleInformation() {
return bundleInformation;
}
/**
*
* The effective date and time of the pricing plan.
*
*
* @return The effective date and time of the pricing plan.
*/
public final Instant effectiveDateTime() {
return effectiveDateTime;
}
/**
*
* The pricing mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pricingMode} will
* return {@link PricingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pricingModeAsString}.
*
*
* @return The pricing mode.
* @see PricingMode
*/
public final PricingMode pricingMode() {
return PricingMode.fromValue(pricingMode);
}
/**
*
* The pricing mode.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #pricingMode} will
* return {@link PricingMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #pricingModeAsString}.
*
*
* @return The pricing mode.
* @see PricingMode
*/
public final String pricingModeAsString() {
return pricingMode;
}
/**
*
* The set date and time for updating a pricing plan.
*
*
* @return The set date and time for updating a pricing plan.
*/
public final Instant updateDateTime() {
return updateDateTime;
}
/**
*
* The update reason for changing a pricing plan.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #updateReason} will
* return {@link UpdateReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #updateReasonAsString}.
*
*
* @return The update reason for changing a pricing plan.
* @see UpdateReason
*/
public final UpdateReason updateReason() {
return UpdateReason.fromValue(updateReason);
}
/**
*
* The update reason for changing a pricing plan.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #updateReason} will
* return {@link UpdateReason#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #updateReasonAsString}.
*
*
* @return The update reason for changing a pricing plan.
* @see UpdateReason
*/
public final String updateReasonAsString() {
return updateReason;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(billableEntityCount());
hashCode = 31 * hashCode + Objects.hashCode(bundleInformation());
hashCode = 31 * hashCode + Objects.hashCode(effectiveDateTime());
hashCode = 31 * hashCode + Objects.hashCode(pricingModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(updateDateTime());
hashCode = 31 * hashCode + Objects.hashCode(updateReasonAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PricingPlan)) {
return false;
}
PricingPlan other = (PricingPlan) obj;
return Objects.equals(billableEntityCount(), other.billableEntityCount())
&& Objects.equals(bundleInformation(), other.bundleInformation())
&& Objects.equals(effectiveDateTime(), other.effectiveDateTime())
&& Objects.equals(pricingModeAsString(), other.pricingModeAsString())
&& Objects.equals(updateDateTime(), other.updateDateTime())
&& Objects.equals(updateReasonAsString(), other.updateReasonAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PricingPlan").add("BillableEntityCount", billableEntityCount())
.add("BundleInformation", bundleInformation()).add("EffectiveDateTime", effectiveDateTime())
.add("PricingMode", pricingModeAsString()).add("UpdateDateTime", updateDateTime())
.add("UpdateReason", updateReasonAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "billableEntityCount":
return Optional.ofNullable(clazz.cast(billableEntityCount()));
case "bundleInformation":
return Optional.ofNullable(clazz.cast(bundleInformation()));
case "effectiveDateTime":
return Optional.ofNullable(clazz.cast(effectiveDateTime()));
case "pricingMode":
return Optional.ofNullable(clazz.cast(pricingModeAsString()));
case "updateDateTime":
return Optional.ofNullable(clazz.cast(updateDateTime()));
case "updateReason":
return Optional.ofNullable(clazz.cast(updateReasonAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function