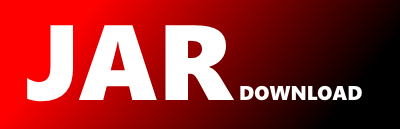
software.amazon.awssdk.services.ivs.IvsAsyncClient Maven / Gradle / Ivy
Show all versions of ivs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ivs;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.core.SdkClient;
import software.amazon.awssdk.services.ivs.model.BatchGetChannelRequest;
import software.amazon.awssdk.services.ivs.model.BatchGetChannelResponse;
import software.amazon.awssdk.services.ivs.model.BatchGetStreamKeyRequest;
import software.amazon.awssdk.services.ivs.model.BatchGetStreamKeyResponse;
import software.amazon.awssdk.services.ivs.model.CreateChannelRequest;
import software.amazon.awssdk.services.ivs.model.CreateChannelResponse;
import software.amazon.awssdk.services.ivs.model.CreateRecordingConfigurationRequest;
import software.amazon.awssdk.services.ivs.model.CreateRecordingConfigurationResponse;
import software.amazon.awssdk.services.ivs.model.CreateStreamKeyRequest;
import software.amazon.awssdk.services.ivs.model.CreateStreamKeyResponse;
import software.amazon.awssdk.services.ivs.model.DeleteChannelRequest;
import software.amazon.awssdk.services.ivs.model.DeleteChannelResponse;
import software.amazon.awssdk.services.ivs.model.DeletePlaybackKeyPairRequest;
import software.amazon.awssdk.services.ivs.model.DeletePlaybackKeyPairResponse;
import software.amazon.awssdk.services.ivs.model.DeleteRecordingConfigurationRequest;
import software.amazon.awssdk.services.ivs.model.DeleteRecordingConfigurationResponse;
import software.amazon.awssdk.services.ivs.model.DeleteStreamKeyRequest;
import software.amazon.awssdk.services.ivs.model.DeleteStreamKeyResponse;
import software.amazon.awssdk.services.ivs.model.GetChannelRequest;
import software.amazon.awssdk.services.ivs.model.GetChannelResponse;
import software.amazon.awssdk.services.ivs.model.GetPlaybackKeyPairRequest;
import software.amazon.awssdk.services.ivs.model.GetPlaybackKeyPairResponse;
import software.amazon.awssdk.services.ivs.model.GetRecordingConfigurationRequest;
import software.amazon.awssdk.services.ivs.model.GetRecordingConfigurationResponse;
import software.amazon.awssdk.services.ivs.model.GetStreamKeyRequest;
import software.amazon.awssdk.services.ivs.model.GetStreamKeyResponse;
import software.amazon.awssdk.services.ivs.model.GetStreamRequest;
import software.amazon.awssdk.services.ivs.model.GetStreamResponse;
import software.amazon.awssdk.services.ivs.model.GetStreamSessionRequest;
import software.amazon.awssdk.services.ivs.model.GetStreamSessionResponse;
import software.amazon.awssdk.services.ivs.model.ImportPlaybackKeyPairRequest;
import software.amazon.awssdk.services.ivs.model.ImportPlaybackKeyPairResponse;
import software.amazon.awssdk.services.ivs.model.ListChannelsRequest;
import software.amazon.awssdk.services.ivs.model.ListChannelsResponse;
import software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsRequest;
import software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsResponse;
import software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsRequest;
import software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsResponse;
import software.amazon.awssdk.services.ivs.model.ListStreamKeysRequest;
import software.amazon.awssdk.services.ivs.model.ListStreamKeysResponse;
import software.amazon.awssdk.services.ivs.model.ListStreamSessionsRequest;
import software.amazon.awssdk.services.ivs.model.ListStreamSessionsResponse;
import software.amazon.awssdk.services.ivs.model.ListStreamsRequest;
import software.amazon.awssdk.services.ivs.model.ListStreamsResponse;
import software.amazon.awssdk.services.ivs.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ivs.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ivs.model.PutMetadataRequest;
import software.amazon.awssdk.services.ivs.model.PutMetadataResponse;
import software.amazon.awssdk.services.ivs.model.StopStreamRequest;
import software.amazon.awssdk.services.ivs.model.StopStreamResponse;
import software.amazon.awssdk.services.ivs.model.TagResourceRequest;
import software.amazon.awssdk.services.ivs.model.TagResourceResponse;
import software.amazon.awssdk.services.ivs.model.UntagResourceRequest;
import software.amazon.awssdk.services.ivs.model.UntagResourceResponse;
import software.amazon.awssdk.services.ivs.model.UpdateChannelRequest;
import software.amazon.awssdk.services.ivs.model.UpdateChannelResponse;
import software.amazon.awssdk.services.ivs.paginators.ListChannelsPublisher;
import software.amazon.awssdk.services.ivs.paginators.ListPlaybackKeyPairsPublisher;
import software.amazon.awssdk.services.ivs.paginators.ListRecordingConfigurationsPublisher;
import software.amazon.awssdk.services.ivs.paginators.ListStreamKeysPublisher;
import software.amazon.awssdk.services.ivs.paginators.ListStreamSessionsPublisher;
import software.amazon.awssdk.services.ivs.paginators.ListStreamsPublisher;
/**
* Service client for accessing Amazon IVS asynchronously. This can be created using the static {@link #builder()}
* method.
*
*
* Introduction
*
*
* The Amazon Interactive Video Service (IVS) API is REST compatible, using a standard HTTP API and an Amazon Web
* Services EventBridge event stream for responses. JSON is used for both requests and responses, including errors.
*
*
* The API is an Amazon Web Services regional service. For a list of supported regions and Amazon IVS HTTPS service
* endpoints, see the Amazon IVS page in the
* Amazon Web Services General Reference.
*
*
* All API request parameters and URLs are case sensitive.
*
*
* For a summary of notable documentation changes in each release, see Document History.
*
*
* Allowed Header Values
*
*
* -
*
* Accept:
application/json
*
*
* -
*
* Accept-Encoding:
gzip, deflate
*
*
* -
*
* Content-Type:
application/json
*
*
*
*
* Resources
*
*
* The following resources contain information about your IVS live stream (see Getting Started with Amazon IVS):
*
*
* -
*
* Channel — Stores configuration data related to your live stream. You first create a channel and then use the
* channel’s stream key to start your live stream. See the Channel endpoints for more information.
*
*
* -
*
* Stream key — An identifier assigned by Amazon IVS when you create a channel, which is then used to authorize
* streaming. See the StreamKey endpoints for more information. Treat the stream key like a secret, since it
* allows anyone to stream to the channel.
*
*
* -
*
* Playback key pair — Video playback may be restricted using playback-authorization tokens, which use public-key
* encryption. A playback key pair is the public-private pair of keys used to sign and validate the
* playback-authorization token. See the PlaybackKeyPair endpoints for more information.
*
*
* -
*
* Recording configuration — Stores configuration related to recording a live stream and where to store the recorded
* content. Multiple channels can reference the same recording configuration. See the Recording Configuration endpoints
* for more information.
*
*
*
*
* Tagging
*
*
* A tag is a metadata label that you assign to an Amazon Web Services resource. A tag comprises a key and
* a value, both set by you. For example, you might set a tag as topic:nature
to label a particular
* video category. See Tagging Amazon Web
* Services Resources for more information, including restrictions that apply to tags.
*
*
* Tags can help you identify and organize your Amazon Web Services resources. For example, you can use the same tag for
* different resources to indicate that they are related. You can also use tags to manage access (see Access Tags).
*
*
* The Amazon IVS API has these tag-related endpoints: TagResource, UntagResource, and
* ListTagsForResource. The following resources support tagging: Channels, Stream Keys, Playback Key Pairs, and
* Recording Configurations.
*
*
* At most 50 tags can be applied to a resource.
*
*
* Authentication versus Authorization
*
*
* Note the differences between these concepts:
*
*
* -
*
* Authentication is about verifying identity. You need to be authenticated to sign Amazon IVS API requests.
*
*
* -
*
* Authorization is about granting permissions. You need to be authorized to view Amazon IVS private channels.
* (Private channels are channels that are enabled for "playback authorization.")
*
*
*
*
* Authentication
*
*
* All Amazon IVS API requests must be authenticated with a signature. The Amazon Web Services Command-Line Interface
* (CLI) and Amazon IVS Player SDKs take care of signing the underlying API calls for you. However, if your application
* calls the Amazon IVS API directly, it’s your responsibility to sign the requests.
*
*
* You generate a signature using valid Amazon Web Services credentials that have permission to perform the requested
* action. For example, you must sign PutMetadata requests with a signature generated from an IAM user account that has
* the ivs:PutMetadata
permission.
*
*
* For more information:
*
*
* -
*
* Authentication and generating signatures — See Authenticating Requests
* (Amazon Web Services Signature Version 4) in the Amazon Web Services General Reference.
*
*
* -
*
* Managing Amazon IVS permissions — See Identity and Access Management on the
* Security page of the Amazon IVS User Guide.
*
*
*
*
* Channel Endpoints
*
*
* -
*
* CreateChannel — Creates a new channel and an associated stream key to start streaming.
*
*
* -
*
* GetChannel — Gets the channel configuration for the specified channel ARN (Amazon Resource Name).
*
*
* -
*
* BatchGetChannel — Performs GetChannel on multiple ARNs simultaneously.
*
*
* -
*
* ListChannels — Gets summary information about all channels in your account, in the Amazon Web Services region
* where the API request is processed. This list can be filtered to match a specified name or recording-configuration
* ARN. Filters are mutually exclusive and cannot be used together. If you try to use both filters, you will get an
* error (409 Conflict Exception).
*
*
* -
*
* UpdateChannel — Updates a channel's configuration. This does not affect an ongoing stream of this channel. You
* must stop and restart the stream for the changes to take effect.
*
*
* -
*
* DeleteChannel — Deletes the specified channel.
*
*
*
*
* StreamKey Endpoints
*
*
* -
*
* CreateStreamKey — Creates a stream key, used to initiate a stream, for the specified channel ARN.
*
*
* -
*
* GetStreamKey — Gets stream key information for the specified ARN.
*
*
* -
*
* BatchGetStreamKey — Performs GetStreamKey on multiple ARNs simultaneously.
*
*
* -
*
* ListStreamKeys — Gets summary information about stream keys for the specified channel.
*
*
* -
*
* DeleteStreamKey — Deletes the stream key for the specified ARN, so it can no longer be used to stream.
*
*
*
*
* Stream Endpoints
*
*
* -
*
* GetStream — Gets information about the active (live) stream on a specified channel.
*
*
* -
*
* GetStreamSession — Gets metadata on a specified stream.
*
*
* -
*
* ListStreams — Gets summary information about live streams in your account, in the Amazon Web Services region
* where the API request is processed.
*
*
* -
*
* ListStreamSessions — Gets a summary of current and previous streams for a specified channel in your account,
* in the AWS region where the API request is processed.
*
*
* -
*
* StopStream — Disconnects the incoming RTMPS stream for the specified channel. Can be used in conjunction with
* DeleteStreamKey to prevent further streaming to a channel.
*
*
* -
*
* PutMetadata — Inserts metadata into the active stream of the specified channel. At most 5 requests per second
* per channel are allowed, each with a maximum 1 KB payload. (If 5 TPS is not sufficient for your needs, we recommend
* batching your data into a single PutMetadata call.) At most 155 requests per second per account are allowed.
*
*
*
*
* PlaybackKeyPair Endpoints
*
*
* For more information, see Setting Up
* Private Channels in the Amazon IVS User Guide.
*
*
* -
*
* ImportPlaybackKeyPair — Imports the public portion of a new key pair and returns its arn
and
* fingerprint
. The privateKey
can then be used to generate viewer authorization tokens, to
* grant viewers access to private channels (channels enabled for playback authorization).
*
*
* -
*
* GetPlaybackKeyPair — Gets a specified playback authorization key pair and returns the arn
and
* fingerprint
. The privateKey
held by the caller can be used to generate viewer authorization
* tokens, to grant viewers access to private channels.
*
*
* -
*
* ListPlaybackKeyPairs — Gets summary information about playback key pairs.
*
*
* -
*
* DeletePlaybackKeyPair — Deletes a specified authorization key pair. This invalidates future viewer tokens
* generated using the key pair’s privateKey
.
*
*
*
*
* RecordingConfiguration Endpoints
*
*
* -
*
* CreateRecordingConfiguration — Creates a new recording configuration, used to enable recording to Amazon S3.
*
*
* -
*
* GetRecordingConfiguration — Gets the recording-configuration metadata for the specified ARN.
*
*
* -
*
* ListRecordingConfigurations — Gets summary information about all recording configurations in your account, in
* the Amazon Web Services region where the API request is processed.
*
*
* -
*
* DeleteRecordingConfiguration — Deletes the recording configuration for the specified ARN.
*
*
*
*
* Amazon Web Services Tags Endpoints
*
*
* -
*
* TagResource — Adds or updates tags for the Amazon Web Services resource with the specified ARN.
*
*
* -
*
* UntagResource — Removes tags from the resource with the specified ARN.
*
*
* -
*
* ListTagsForResource — Gets information about Amazon Web Services tags for the specified ARN.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface IvsAsyncClient extends SdkClient {
String SERVICE_NAME = "ivs";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ivs";
/**
* Create a {@link IvsAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static IvsAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link IvsAsyncClient}.
*/
static IvsAsyncClientBuilder builder() {
return new DefaultIvsAsyncClientBuilder();
}
/**
*
* Performs GetChannel on multiple ARNs simultaneously.
*
*
* @param batchGetChannelRequest
* @return A Java Future containing the result of the BatchGetChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.BatchGetChannel
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetChannel(BatchGetChannelRequest batchGetChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Performs GetChannel on multiple ARNs simultaneously.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetChannelRequest.Builder} avoiding the need
* to create one manually via {@link BatchGetChannelRequest#builder()}
*
*
* @param batchGetChannelRequest
* A {@link Consumer} that will call methods on {@link BatchGetChannelRequest.Builder} to create a request.
* @return A Java Future containing the result of the BatchGetChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.BatchGetChannel
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetChannel(
Consumer batchGetChannelRequest) {
return batchGetChannel(BatchGetChannelRequest.builder().applyMutation(batchGetChannelRequest).build());
}
/**
*
* Performs GetStreamKey on multiple ARNs simultaneously.
*
*
* @param batchGetStreamKeyRequest
* @return A Java Future containing the result of the BatchGetStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.BatchGetStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetStreamKey(BatchGetStreamKeyRequest batchGetStreamKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Performs GetStreamKey on multiple ARNs simultaneously.
*
*
*
* This is a convenience which creates an instance of the {@link BatchGetStreamKeyRequest.Builder} avoiding the need
* to create one manually via {@link BatchGetStreamKeyRequest#builder()}
*
*
* @param batchGetStreamKeyRequest
* A {@link Consumer} that will call methods on {@link BatchGetStreamKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the BatchGetStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.BatchGetStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture batchGetStreamKey(
Consumer batchGetStreamKeyRequest) {
return batchGetStreamKey(BatchGetStreamKeyRequest.builder().applyMutation(batchGetStreamKeyRequest).build());
}
/**
*
* Creates a new channel and an associated stream key to start streaming.
*
*
* @param createChannelRequest
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.CreateChannel
* @see AWS API
* Documentation
*/
default CompletableFuture createChannel(CreateChannelRequest createChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new channel and an associated stream key to start streaming.
*
*
*
* This is a convenience which creates an instance of the {@link CreateChannelRequest.Builder} avoiding the need to
* create one manually via {@link CreateChannelRequest#builder()}
*
*
* @param createChannelRequest
* A {@link Consumer} that will call methods on {@link CreateChannelRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.CreateChannel
* @see AWS API
* Documentation
*/
default CompletableFuture createChannel(Consumer createChannelRequest) {
return createChannel(CreateChannelRequest.builder().applyMutation(createChannelRequest).build());
}
/**
*
* Creates a new recording configuration, used to enable recording to Amazon S3.
*
*
* Known issue: In the us-east-1 region, if you use the Amazon Web Services CLI to create a recording
* configuration, it returns success even if the S3 bucket is in a different region. In this case, the
* state
of the recording configuration is CREATE_FAILED
(instead of ACTIVE
).
* (In other regions, the CLI correctly returns failure if the bucket is in a different region.)
*
*
* Workaround: Ensure that your S3 bucket is in the same region as the recording configuration. If you create
* a recording configuration in a different region as your S3 bucket, delete that recording configuration and create
* a new one with an S3 bucket from the correct region.
*
*
* @param createRecordingConfigurationRequest
* @return A Java Future containing the result of the CreateRecordingConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.CreateRecordingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture createRecordingConfiguration(
CreateRecordingConfigurationRequest createRecordingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new recording configuration, used to enable recording to Amazon S3.
*
*
* Known issue: In the us-east-1 region, if you use the Amazon Web Services CLI to create a recording
* configuration, it returns success even if the S3 bucket is in a different region. In this case, the
* state
of the recording configuration is CREATE_FAILED
(instead of ACTIVE
).
* (In other regions, the CLI correctly returns failure if the bucket is in a different region.)
*
*
* Workaround: Ensure that your S3 bucket is in the same region as the recording configuration. If you create
* a recording configuration in a different region as your S3 bucket, delete that recording configuration and create
* a new one with an S3 bucket from the correct region.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRecordingConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link CreateRecordingConfigurationRequest#builder()}
*
*
* @param createRecordingConfigurationRequest
* A {@link Consumer} that will call methods on {@link CreateRecordingConfigurationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateRecordingConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.CreateRecordingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture createRecordingConfiguration(
Consumer createRecordingConfigurationRequest) {
return createRecordingConfiguration(CreateRecordingConfigurationRequest.builder()
.applyMutation(createRecordingConfigurationRequest).build());
}
/**
*
* Creates a stream key, used to initiate a stream, for the specified channel ARN.
*
*
* Note that CreateChannel creates a stream key. If you subsequently use CreateStreamKey on the same channel,
* it will fail because a stream key already exists and there is a limit of 1 stream key per channel. To reset the
* stream key on a channel, use DeleteStreamKey and then CreateStreamKey.
*
*
* @param createStreamKeyRequest
* @return A Java Future containing the result of the CreateStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.CreateStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture createStreamKey(CreateStreamKeyRequest createStreamKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a stream key, used to initiate a stream, for the specified channel ARN.
*
*
* Note that CreateChannel creates a stream key. If you subsequently use CreateStreamKey on the same channel,
* it will fail because a stream key already exists and there is a limit of 1 stream key per channel. To reset the
* stream key on a channel, use DeleteStreamKey and then CreateStreamKey.
*
*
*
* This is a convenience which creates an instance of the {@link CreateStreamKeyRequest.Builder} avoiding the need
* to create one manually via {@link CreateStreamKeyRequest#builder()}
*
*
* @param createStreamKeyRequest
* A {@link Consumer} that will call methods on {@link CreateStreamKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.CreateStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture createStreamKey(
Consumer createStreamKeyRequest) {
return createStreamKey(CreateStreamKeyRequest.builder().applyMutation(createStreamKeyRequest).build());
}
/**
*
* Deletes the specified channel and its associated stream keys.
*
*
* If you try to delete a live channel, you will get an error (409 ConflictException). To delete a channel that is
* live, call StopStream, wait for the Amazon EventBridge "Stream End" event (to verify that the stream's
* state was changed from Live to Offline), then call DeleteChannel. (See Using EventBridge with Amazon IVS.)
*
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeleteChannel
* @see AWS API
* Documentation
*/
default CompletableFuture deleteChannel(DeleteChannelRequest deleteChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified channel and its associated stream keys.
*
*
* If you try to delete a live channel, you will get an error (409 ConflictException). To delete a channel that is
* live, call StopStream, wait for the Amazon EventBridge "Stream End" event (to verify that the stream's
* state was changed from Live to Offline), then call DeleteChannel. (See Using EventBridge with Amazon IVS.)
*
*
*
* This is a convenience which creates an instance of the {@link DeleteChannelRequest.Builder} avoiding the need to
* create one manually via {@link DeleteChannelRequest#builder()}
*
*
* @param deleteChannelRequest
* A {@link Consumer} that will call methods on {@link DeleteChannelRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeleteChannel
* @see AWS API
* Documentation
*/
default CompletableFuture deleteChannel(Consumer deleteChannelRequest) {
return deleteChannel(DeleteChannelRequest.builder().applyMutation(deleteChannelRequest).build());
}
/**
*
* Deletes a specified authorization key pair. This invalidates future viewer tokens generated using the key pair’s
* privateKey
. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
* @param deletePlaybackKeyPairRequest
* @return A Java Future containing the result of the DeletePlaybackKeyPair operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeletePlaybackKeyPair
* @see AWS API
* Documentation
*/
default CompletableFuture deletePlaybackKeyPair(
DeletePlaybackKeyPairRequest deletePlaybackKeyPairRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes a specified authorization key pair. This invalidates future viewer tokens generated using the key pair’s
* privateKey
. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link DeletePlaybackKeyPairRequest.Builder} avoiding the
* need to create one manually via {@link DeletePlaybackKeyPairRequest#builder()}
*
*
* @param deletePlaybackKeyPairRequest
* A {@link Consumer} that will call methods on {@link DeletePlaybackKeyPairRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the DeletePlaybackKeyPair operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeletePlaybackKeyPair
* @see AWS API
* Documentation
*/
default CompletableFuture deletePlaybackKeyPair(
Consumer deletePlaybackKeyPairRequest) {
return deletePlaybackKeyPair(DeletePlaybackKeyPairRequest.builder().applyMutation(deletePlaybackKeyPairRequest).build());
}
/**
*
* Deletes the recording configuration for the specified ARN.
*
*
* If you try to delete a recording configuration that is associated with a channel, you will get an error (409
* ConflictException). To avoid this, for all channels that reference the recording configuration, first use
* UpdateChannel to set the recordingConfigurationArn
field to an empty string, then use
* DeleteRecordingConfiguration.
*
*
* @param deleteRecordingConfigurationRequest
* @return A Java Future containing the result of the DeleteRecordingConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeleteRecordingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteRecordingConfiguration(
DeleteRecordingConfigurationRequest deleteRecordingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the recording configuration for the specified ARN.
*
*
* If you try to delete a recording configuration that is associated with a channel, you will get an error (409
* ConflictException). To avoid this, for all channels that reference the recording configuration, first use
* UpdateChannel to set the recordingConfigurationArn
field to an empty string, then use
* DeleteRecordingConfiguration.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRecordingConfigurationRequest.Builder}
* avoiding the need to create one manually via {@link DeleteRecordingConfigurationRequest#builder()}
*
*
* @param deleteRecordingConfigurationRequest
* A {@link Consumer} that will call methods on {@link DeleteRecordingConfigurationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteRecordingConfiguration operation returned by the
* service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeleteRecordingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteRecordingConfiguration(
Consumer deleteRecordingConfigurationRequest) {
return deleteRecordingConfiguration(DeleteRecordingConfigurationRequest.builder()
.applyMutation(deleteRecordingConfigurationRequest).build());
}
/**
*
* Deletes the stream key for the specified ARN, so it can no longer be used to stream.
*
*
* @param deleteStreamKeyRequest
* @return A Java Future containing the result of the DeleteStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeleteStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture deleteStreamKey(DeleteStreamKeyRequest deleteStreamKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the stream key for the specified ARN, so it can no longer be used to stream.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteStreamKeyRequest.Builder} avoiding the need
* to create one manually via {@link DeleteStreamKeyRequest#builder()}
*
*
* @param deleteStreamKeyRequest
* A {@link Consumer} that will call methods on {@link DeleteStreamKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.DeleteStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture deleteStreamKey(
Consumer deleteStreamKeyRequest) {
return deleteStreamKey(DeleteStreamKeyRequest.builder().applyMutation(deleteStreamKeyRequest).build());
}
/**
*
* Gets the channel configuration for the specified channel ARN. See also BatchGetChannel.
*
*
* @param getChannelRequest
* @return A Java Future containing the result of the GetChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetChannel
* @see AWS API
* Documentation
*/
default CompletableFuture getChannel(GetChannelRequest getChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the channel configuration for the specified channel ARN. See also BatchGetChannel.
*
*
*
* This is a convenience which creates an instance of the {@link GetChannelRequest.Builder} avoiding the need to
* create one manually via {@link GetChannelRequest#builder()}
*
*
* @param getChannelRequest
* A {@link Consumer} that will call methods on {@link GetChannelRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetChannel
* @see AWS API
* Documentation
*/
default CompletableFuture getChannel(Consumer getChannelRequest) {
return getChannel(GetChannelRequest.builder().applyMutation(getChannelRequest).build());
}
/**
*
* Gets a specified playback authorization key pair and returns the arn
and fingerprint
.
* The privateKey
held by the caller can be used to generate viewer authorization tokens, to grant
* viewers access to private channels. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
* @param getPlaybackKeyPairRequest
* @return A Java Future containing the result of the GetPlaybackKeyPair operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetPlaybackKeyPair
* @see AWS API
* Documentation
*/
default CompletableFuture getPlaybackKeyPair(GetPlaybackKeyPairRequest getPlaybackKeyPairRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a specified playback authorization key pair and returns the arn
and fingerprint
.
* The privateKey
held by the caller can be used to generate viewer authorization tokens, to grant
* viewers access to private channels. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link GetPlaybackKeyPairRequest.Builder} avoiding the
* need to create one manually via {@link GetPlaybackKeyPairRequest#builder()}
*
*
* @param getPlaybackKeyPairRequest
* A {@link Consumer} that will call methods on {@link GetPlaybackKeyPairRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetPlaybackKeyPair operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetPlaybackKeyPair
* @see AWS API
* Documentation
*/
default CompletableFuture getPlaybackKeyPair(
Consumer getPlaybackKeyPairRequest) {
return getPlaybackKeyPair(GetPlaybackKeyPairRequest.builder().applyMutation(getPlaybackKeyPairRequest).build());
}
/**
*
* Gets the recording configuration for the specified ARN.
*
*
* @param getRecordingConfigurationRequest
* @return A Java Future containing the result of the GetRecordingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetRecordingConfiguration
* @see AWS
* API Documentation
*/
default CompletableFuture getRecordingConfiguration(
GetRecordingConfigurationRequest getRecordingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the recording configuration for the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetRecordingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link GetRecordingConfigurationRequest#builder()}
*
*
* @param getRecordingConfigurationRequest
* A {@link Consumer} that will call methods on {@link GetRecordingConfigurationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetRecordingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetRecordingConfiguration
* @see AWS
* API Documentation
*/
default CompletableFuture getRecordingConfiguration(
Consumer getRecordingConfigurationRequest) {
return getRecordingConfiguration(GetRecordingConfigurationRequest.builder()
.applyMutation(getRecordingConfigurationRequest).build());
}
/**
*
* Gets information about the active (live) stream on a specified channel.
*
*
* @param getStreamRequest
* @return A Java Future containing the result of the GetStream operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - ChannelNotBroadcastingException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetStream
* @see AWS API
* Documentation
*/
default CompletableFuture getStream(GetStreamRequest getStreamRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about the active (live) stream on a specified channel.
*
*
*
* This is a convenience which creates an instance of the {@link GetStreamRequest.Builder} avoiding the need to
* create one manually via {@link GetStreamRequest#builder()}
*
*
* @param getStreamRequest
* A {@link Consumer} that will call methods on {@link GetStreamRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetStream operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - ChannelNotBroadcastingException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetStream
* @see AWS API
* Documentation
*/
default CompletableFuture getStream(Consumer getStreamRequest) {
return getStream(GetStreamRequest.builder().applyMutation(getStreamRequest).build());
}
/**
*
* Gets stream-key information for a specified ARN.
*
*
* @param getStreamKeyRequest
* @return A Java Future containing the result of the GetStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture getStreamKey(GetStreamKeyRequest getStreamKeyRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets stream-key information for a specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link GetStreamKeyRequest.Builder} avoiding the need to
* create one manually via {@link GetStreamKeyRequest#builder()}
*
*
* @param getStreamKeyRequest
* A {@link Consumer} that will call methods on {@link GetStreamKeyRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetStreamKey operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetStreamKey
* @see AWS API
* Documentation
*/
default CompletableFuture getStreamKey(Consumer getStreamKeyRequest) {
return getStreamKey(GetStreamKeyRequest.builder().applyMutation(getStreamKeyRequest).build());
}
/**
*
* Gets metadata on a specified stream.
*
*
* @param getStreamSessionRequest
* @return A Java Future containing the result of the GetStreamSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetStreamSession
* @see AWS API
* Documentation
*/
default CompletableFuture getStreamSession(GetStreamSessionRequest getStreamSessionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets metadata on a specified stream.
*
*
*
* This is a convenience which creates an instance of the {@link GetStreamSessionRequest.Builder} avoiding the need
* to create one manually via {@link GetStreamSessionRequest#builder()}
*
*
* @param getStreamSessionRequest
* A {@link Consumer} that will call methods on {@link GetStreamSessionRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetStreamSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.GetStreamSession
* @see AWS API
* Documentation
*/
default CompletableFuture getStreamSession(
Consumer getStreamSessionRequest) {
return getStreamSession(GetStreamSessionRequest.builder().applyMutation(getStreamSessionRequest).build());
}
/**
*
* Imports the public portion of a new key pair and returns its arn
and fingerprint
. The
* privateKey
can then be used to generate viewer authorization tokens, to grant viewers access to
* private channels. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
* @param importPlaybackKeyPairRequest
* @return A Java Future containing the result of the ImportPlaybackKeyPair operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ImportPlaybackKeyPair
* @see AWS API
* Documentation
*/
default CompletableFuture importPlaybackKeyPair(
ImportPlaybackKeyPairRequest importPlaybackKeyPairRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Imports the public portion of a new key pair and returns its arn
and fingerprint
. The
* privateKey
can then be used to generate viewer authorization tokens, to grant viewers access to
* private channels. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ImportPlaybackKeyPairRequest.Builder} avoiding the
* need to create one manually via {@link ImportPlaybackKeyPairRequest#builder()}
*
*
* @param importPlaybackKeyPairRequest
* A {@link Consumer} that will call methods on {@link ImportPlaybackKeyPairRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ImportPlaybackKeyPair operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - ServiceQuotaExceededException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ImportPlaybackKeyPair
* @see AWS API
* Documentation
*/
default CompletableFuture importPlaybackKeyPair(
Consumer importPlaybackKeyPairRequest) {
return importPlaybackKeyPair(ImportPlaybackKeyPairRequest.builder().applyMutation(importPlaybackKeyPairRequest).build());
}
/**
*
* Gets summary information about all channels in your account, in the Amazon Web Services region where the API
* request is processed. This list can be filtered to match a specified name or recording-configuration ARN. Filters
* are mutually exclusive and cannot be used together. If you try to use both filters, you will get an error (409
* ConflictException).
*
*
* @param listChannelsRequest
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default CompletableFuture listChannels(ListChannelsRequest listChannelsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about all channels in your account, in the Amazon Web Services region where the API
* request is processed. This list can be filtered to match a specified name or recording-configuration ARN. Filters
* are mutually exclusive and cannot be used together. If you try to use both filters, you will get an error (409
* ConflictException).
*
*
*
* This is a convenience which creates an instance of the {@link ListChannelsRequest.Builder} avoiding the need to
* create one manually via {@link ListChannelsRequest#builder()}
*
*
* @param listChannelsRequest
* A {@link Consumer} that will call methods on {@link ListChannelsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListChannels operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default CompletableFuture listChannels(Consumer listChannelsRequest) {
return listChannels(ListChannelsRequest.builder().applyMutation(listChannelsRequest).build());
}
/**
*
* Gets summary information about all channels in your account, in the Amazon Web Services region where the API
* request is processed. This list can be filtered to match a specified name or recording-configuration ARN. Filters
* are mutually exclusive and cannot be used together. If you try to use both filters, you will get an error (409
* ConflictException).
*
*
*
* This is a variant of {@link #listChannels(software.amazon.awssdk.services.ivs.model.ListChannelsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListChannelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.ivs.model.ListChannelsRequest)} operation.
*
*
* @param listChannelsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsPublisher listChannelsPaginator(ListChannelsRequest listChannelsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about all channels in your account, in the Amazon Web Services region where the API
* request is processed. This list can be filtered to match a specified name or recording-configuration ARN. Filters
* are mutually exclusive and cannot be used together. If you try to use both filters, you will get an error (409
* ConflictException).
*
*
*
* This is a variant of {@link #listChannels(software.amazon.awssdk.services.ivs.model.ListChannelsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListChannelsPublisher publisher = client.listChannelsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListChannelsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listChannels(software.amazon.awssdk.services.ivs.model.ListChannelsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListChannelsRequest.Builder} avoiding the need to
* create one manually via {@link ListChannelsRequest#builder()}
*
*
* @param listChannelsRequest
* A {@link Consumer} that will call methods on {@link ListChannelsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListChannels
* @see AWS API
* Documentation
*/
default ListChannelsPublisher listChannelsPaginator(Consumer listChannelsRequest) {
return listChannelsPaginator(ListChannelsRequest.builder().applyMutation(listChannelsRequest).build());
}
/**
*
* Gets summary information about playback key pairs. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
* @param listPlaybackKeyPairsRequest
* @return A Java Future containing the result of the ListPlaybackKeyPairs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListPlaybackKeyPairs
* @see AWS API
* Documentation
*/
default CompletableFuture listPlaybackKeyPairs(
ListPlaybackKeyPairsRequest listPlaybackKeyPairsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about playback key pairs. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link ListPlaybackKeyPairsRequest.Builder} avoiding the
* need to create one manually via {@link ListPlaybackKeyPairsRequest#builder()}
*
*
* @param listPlaybackKeyPairsRequest
* A {@link Consumer} that will call methods on {@link ListPlaybackKeyPairsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListPlaybackKeyPairs operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListPlaybackKeyPairs
* @see AWS API
* Documentation
*/
default CompletableFuture listPlaybackKeyPairs(
Consumer listPlaybackKeyPairsRequest) {
return listPlaybackKeyPairs(ListPlaybackKeyPairsRequest.builder().applyMutation(listPlaybackKeyPairsRequest).build());
}
/**
*
* Gets summary information about playback key pairs. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
*
* This is a variant of
* {@link #listPlaybackKeyPairs(software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListPlaybackKeyPairsPublisher publisher = client.listPlaybackKeyPairsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListPlaybackKeyPairsPublisher publisher = client.listPlaybackKeyPairsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlaybackKeyPairs(software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsRequest)}
* operation.
*
*
* @param listPlaybackKeyPairsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListPlaybackKeyPairs
* @see AWS API
* Documentation
*/
default ListPlaybackKeyPairsPublisher listPlaybackKeyPairsPaginator(ListPlaybackKeyPairsRequest listPlaybackKeyPairsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about playback key pairs. For more information, see Setting Up Private Channels in
* the Amazon IVS User Guide.
*
*
*
* This is a variant of
* {@link #listPlaybackKeyPairs(software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsRequest)} operation.
* The return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListPlaybackKeyPairsPublisher publisher = client.listPlaybackKeyPairsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListPlaybackKeyPairsPublisher publisher = client.listPlaybackKeyPairsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listPlaybackKeyPairs(software.amazon.awssdk.services.ivs.model.ListPlaybackKeyPairsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListPlaybackKeyPairsRequest.Builder} avoiding the
* need to create one manually via {@link ListPlaybackKeyPairsRequest#builder()}
*
*
* @param listPlaybackKeyPairsRequest
* A {@link Consumer} that will call methods on {@link ListPlaybackKeyPairsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListPlaybackKeyPairs
* @see AWS API
* Documentation
*/
default ListPlaybackKeyPairsPublisher listPlaybackKeyPairsPaginator(
Consumer listPlaybackKeyPairsRequest) {
return listPlaybackKeyPairsPaginator(ListPlaybackKeyPairsRequest.builder().applyMutation(listPlaybackKeyPairsRequest)
.build());
}
/**
*
* Gets summary information about all recording configurations in your account, in the Amazon Web Services region
* where the API request is processed.
*
*
* @param listRecordingConfigurationsRequest
* @return A Java Future containing the result of the ListRecordingConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListRecordingConfigurations
* @see AWS API Documentation
*/
default CompletableFuture listRecordingConfigurations(
ListRecordingConfigurationsRequest listRecordingConfigurationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about all recording configurations in your account, in the Amazon Web Services region
* where the API request is processed.
*
*
*
* This is a convenience which creates an instance of the {@link ListRecordingConfigurationsRequest.Builder}
* avoiding the need to create one manually via {@link ListRecordingConfigurationsRequest#builder()}
*
*
* @param listRecordingConfigurationsRequest
* A {@link Consumer} that will call methods on {@link ListRecordingConfigurationsRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the ListRecordingConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListRecordingConfigurations
* @see AWS API Documentation
*/
default CompletableFuture listRecordingConfigurations(
Consumer listRecordingConfigurationsRequest) {
return listRecordingConfigurations(ListRecordingConfigurationsRequest.builder()
.applyMutation(listRecordingConfigurationsRequest).build());
}
/**
*
* Gets summary information about all recording configurations in your account, in the Amazon Web Services region
* where the API request is processed.
*
*
*
* This is a variant of
* {@link #listRecordingConfigurations(software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListRecordingConfigurationsPublisher publisher = client.listRecordingConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListRecordingConfigurationsPublisher publisher = client.listRecordingConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRecordingConfigurations(software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsRequest)}
* operation.
*
*
* @param listRecordingConfigurationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListRecordingConfigurations
* @see AWS API Documentation
*/
default ListRecordingConfigurationsPublisher listRecordingConfigurationsPaginator(
ListRecordingConfigurationsRequest listRecordingConfigurationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about all recording configurations in your account, in the Amazon Web Services region
* where the API request is processed.
*
*
*
* This is a variant of
* {@link #listRecordingConfigurations(software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListRecordingConfigurationsPublisher publisher = client.listRecordingConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListRecordingConfigurationsPublisher publisher = client.listRecordingConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRecordingConfigurations(software.amazon.awssdk.services.ivs.model.ListRecordingConfigurationsRequest)}
* operation.
*
*
* This is a convenience which creates an instance of the {@link ListRecordingConfigurationsRequest.Builder}
* avoiding the need to create one manually via {@link ListRecordingConfigurationsRequest#builder()}
*
*
* @param listRecordingConfigurationsRequest
* A {@link Consumer} that will call methods on {@link ListRecordingConfigurationsRequest.Builder} to create
* a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - InternalServerException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListRecordingConfigurations
* @see AWS API Documentation
*/
default ListRecordingConfigurationsPublisher listRecordingConfigurationsPaginator(
Consumer listRecordingConfigurationsRequest) {
return listRecordingConfigurationsPaginator(ListRecordingConfigurationsRequest.builder()
.applyMutation(listRecordingConfigurationsRequest).build());
}
/**
*
* Gets summary information about stream keys for the specified channel.
*
*
* @param listStreamKeysRequest
* @return A Java Future containing the result of the ListStreamKeys operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamKeys
* @see AWS API
* Documentation
*/
default CompletableFuture listStreamKeys(ListStreamKeysRequest listStreamKeysRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about stream keys for the specified channel.
*
*
*
* This is a convenience which creates an instance of the {@link ListStreamKeysRequest.Builder} avoiding the need to
* create one manually via {@link ListStreamKeysRequest#builder()}
*
*
* @param listStreamKeysRequest
* A {@link Consumer} that will call methods on {@link ListStreamKeysRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListStreamKeys operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamKeys
* @see AWS API
* Documentation
*/
default CompletableFuture listStreamKeys(Consumer listStreamKeysRequest) {
return listStreamKeys(ListStreamKeysRequest.builder().applyMutation(listStreamKeysRequest).build());
}
/**
*
* Gets summary information about stream keys for the specified channel.
*
*
*
* This is a variant of {@link #listStreamKeys(software.amazon.awssdk.services.ivs.model.ListStreamKeysRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamKeysPublisher publisher = client.listStreamKeysPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamKeysPublisher publisher = client.listStreamKeysPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListStreamKeysResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStreamKeys(software.amazon.awssdk.services.ivs.model.ListStreamKeysRequest)} operation.
*
*
* @param listStreamKeysRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamKeys
* @see AWS API
* Documentation
*/
default ListStreamKeysPublisher listStreamKeysPaginator(ListStreamKeysRequest listStreamKeysRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about stream keys for the specified channel.
*
*
*
* This is a variant of {@link #listStreamKeys(software.amazon.awssdk.services.ivs.model.ListStreamKeysRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamKeysPublisher publisher = client.listStreamKeysPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamKeysPublisher publisher = client.listStreamKeysPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListStreamKeysResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStreamKeys(software.amazon.awssdk.services.ivs.model.ListStreamKeysRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListStreamKeysRequest.Builder} avoiding the need to
* create one manually via {@link ListStreamKeysRequest#builder()}
*
*
* @param listStreamKeysRequest
* A {@link Consumer} that will call methods on {@link ListStreamKeysRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamKeys
* @see AWS API
* Documentation
*/
default ListStreamKeysPublisher listStreamKeysPaginator(Consumer listStreamKeysRequest) {
return listStreamKeysPaginator(ListStreamKeysRequest.builder().applyMutation(listStreamKeysRequest).build());
}
/**
*
* Gets a summary of current and previous streams for a specified channel in your account, in the AWS region where
* the API request is processed.
*
*
* @param listStreamSessionsRequest
* @return A Java Future containing the result of the ListStreamSessions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamSessions
* @see AWS API
* Documentation
*/
default CompletableFuture listStreamSessions(ListStreamSessionsRequest listStreamSessionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a summary of current and previous streams for a specified channel in your account, in the AWS region where
* the API request is processed.
*
*
*
* This is a convenience which creates an instance of the {@link ListStreamSessionsRequest.Builder} avoiding the
* need to create one manually via {@link ListStreamSessionsRequest#builder()}
*
*
* @param listStreamSessionsRequest
* A {@link Consumer} that will call methods on {@link ListStreamSessionsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListStreamSessions operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamSessions
* @see AWS API
* Documentation
*/
default CompletableFuture listStreamSessions(
Consumer listStreamSessionsRequest) {
return listStreamSessions(ListStreamSessionsRequest.builder().applyMutation(listStreamSessionsRequest).build());
}
/**
*
* Gets a summary of current and previous streams for a specified channel in your account, in the AWS region where
* the API request is processed.
*
*
*
* This is a variant of
* {@link #listStreamSessions(software.amazon.awssdk.services.ivs.model.ListStreamSessionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamSessionsPublisher publisher = client.listStreamSessionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamSessionsPublisher publisher = client.listStreamSessionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListStreamSessionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStreamSessions(software.amazon.awssdk.services.ivs.model.ListStreamSessionsRequest)} operation.
*
*
* @param listStreamSessionsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamSessions
* @see AWS API
* Documentation
*/
default ListStreamSessionsPublisher listStreamSessionsPaginator(ListStreamSessionsRequest listStreamSessionsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets a summary of current and previous streams for a specified channel in your account, in the AWS region where
* the API request is processed.
*
*
*
* This is a variant of
* {@link #listStreamSessions(software.amazon.awssdk.services.ivs.model.ListStreamSessionsRequest)} operation. The
* return type is a custom publisher that can be subscribed to request a stream of response pages. SDK will
* internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamSessionsPublisher publisher = client.listStreamSessionsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamSessionsPublisher publisher = client.listStreamSessionsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListStreamSessionsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStreamSessions(software.amazon.awssdk.services.ivs.model.ListStreamSessionsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListStreamSessionsRequest.Builder} avoiding the
* need to create one manually via {@link ListStreamSessionsRequest#builder()}
*
*
* @param listStreamSessionsRequest
* A {@link Consumer} that will call methods on {@link ListStreamSessionsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreamSessions
* @see AWS API
* Documentation
*/
default ListStreamSessionsPublisher listStreamSessionsPaginator(
Consumer listStreamSessionsRequest) {
return listStreamSessionsPaginator(ListStreamSessionsRequest.builder().applyMutation(listStreamSessionsRequest).build());
}
/**
*
* Gets summary information about live streams in your account, in the Amazon Web Services region where the API
* request is processed.
*
*
* @param listStreamsRequest
* @return A Java Future containing the result of the ListStreams operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreams
* @see AWS API
* Documentation
*/
default CompletableFuture listStreams(ListStreamsRequest listStreamsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about live streams in your account, in the Amazon Web Services region where the API
* request is processed.
*
*
*
* This is a convenience which creates an instance of the {@link ListStreamsRequest.Builder} avoiding the need to
* create one manually via {@link ListStreamsRequest#builder()}
*
*
* @param listStreamsRequest
* A {@link Consumer} that will call methods on {@link ListStreamsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListStreams operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreams
* @see AWS API
* Documentation
*/
default CompletableFuture listStreams(Consumer listStreamsRequest) {
return listStreams(ListStreamsRequest.builder().applyMutation(listStreamsRequest).build());
}
/**
*
* Gets summary information about live streams in your account, in the Amazon Web Services region where the API
* request is processed.
*
*
*
* This is a variant of {@link #listStreams(software.amazon.awssdk.services.ivs.model.ListStreamsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamsPublisher publisher = client.listStreamsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamsPublisher publisher = client.listStreamsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListStreamsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStreams(software.amazon.awssdk.services.ivs.model.ListStreamsRequest)} operation.
*
*
* @param listStreamsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreams
* @see AWS API
* Documentation
*/
default ListStreamsPublisher listStreamsPaginator(ListStreamsRequest listStreamsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about live streams in your account, in the Amazon Web Services region where the API
* request is processed.
*
*
*
* This is a variant of {@link #listStreams(software.amazon.awssdk.services.ivs.model.ListStreamsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamsPublisher publisher = client.listStreamsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivs.paginators.ListStreamsPublisher publisher = client.listStreamsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivs.model.ListStreamsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listStreams(software.amazon.awssdk.services.ivs.model.ListStreamsRequest)} operation.
*
*
* This is a convenience which creates an instance of the {@link ListStreamsRequest.Builder} avoiding the need to
* create one manually via {@link ListStreamsRequest#builder()}
*
*
* @param listStreamsRequest
* A {@link Consumer} that will call methods on {@link ListStreamsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListStreams
* @see AWS API
* Documentation
*/
default ListStreamsPublisher listStreamsPaginator(Consumer listStreamsRequest) {
return listStreamsPaginator(ListStreamsRequest.builder().applyMutation(listStreamsRequest).build());
}
/**
*
* Gets information about Amazon Web Services tags for the specified ARN.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about Amazon Web Services tags for the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on {@link ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.ListTagsForResource
* @see AWS API
* Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Inserts metadata into the active stream of the specified channel. At most 5 requests per second per channel are
* allowed, each with a maximum 1 KB payload. (If 5 TPS is not sufficient for your needs, we recommend batching your
* data into a single PutMetadata call.) At most 155 requests per second per account are allowed. Also see Embedding Metadata within a Video
* Stream in the Amazon IVS User Guide.
*
*
* @param putMetadataRequest
* @return A Java Future containing the result of the PutMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - ChannelNotBroadcastingException
* - ThrottlingException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.PutMetadata
* @see AWS API
* Documentation
*/
default CompletableFuture putMetadata(PutMetadataRequest putMetadataRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Inserts metadata into the active stream of the specified channel. At most 5 requests per second per channel are
* allowed, each with a maximum 1 KB payload. (If 5 TPS is not sufficient for your needs, we recommend batching your
* data into a single PutMetadata call.) At most 155 requests per second per account are allowed. Also see Embedding Metadata within a Video
* Stream in the Amazon IVS User Guide.
*
*
*
* This is a convenience which creates an instance of the {@link PutMetadataRequest.Builder} avoiding the need to
* create one manually via {@link PutMetadataRequest#builder()}
*
*
* @param putMetadataRequest
* A {@link Consumer} that will call methods on {@link PutMetadataRequest.Builder} to create a request.
* @return A Java Future containing the result of the PutMetadata operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - ChannelNotBroadcastingException
* - ThrottlingException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.PutMetadata
* @see AWS API
* Documentation
*/
default CompletableFuture putMetadata(Consumer putMetadataRequest) {
return putMetadata(PutMetadataRequest.builder().applyMutation(putMetadataRequest).build());
}
/**
*
* Disconnects the incoming RTMPS stream for the specified channel. Can be used in conjunction with
* DeleteStreamKey to prevent further streaming to a channel.
*
*
*
* Many streaming client-software libraries automatically reconnect a dropped RTMPS session, so to stop the stream
* permanently, you may want to first revoke the streamKey
attached to the channel.
*
*
*
* @param stopStreamRequest
* @return A Java Future containing the result of the StopStream operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - ChannelNotBroadcastingException
* - StreamUnavailableException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.StopStream
* @see AWS API
* Documentation
*/
default CompletableFuture stopStream(StopStreamRequest stopStreamRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disconnects the incoming RTMPS stream for the specified channel. Can be used in conjunction with
* DeleteStreamKey to prevent further streaming to a channel.
*
*
*
* Many streaming client-software libraries automatically reconnect a dropped RTMPS session, so to stop the stream
* permanently, you may want to first revoke the streamKey
attached to the channel.
*
*
*
* This is a convenience which creates an instance of the {@link StopStreamRequest.Builder} avoiding the need to
* create one manually via {@link StopStreamRequest#builder()}
*
*
* @param stopStreamRequest
* A {@link Consumer} that will call methods on {@link StopStreamRequest.Builder} to create a request.
* @return A Java Future containing the result of the StopStream operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - ChannelNotBroadcastingException
* - StreamUnavailableException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.StopStream
* @see AWS API
* Documentation
*/
default CompletableFuture stopStream(Consumer stopStreamRequest) {
return stopStream(StopStreamRequest.builder().applyMutation(stopStreamRequest).build());
}
/**
*
* Adds or updates tags for the Amazon Web Services resource with the specified ARN.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates tags for the Amazon Web Services resource with the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on {@link TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from the resource with the specified ARN.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from the resource with the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on {@link UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a channel's configuration. This does not affect an ongoing stream of this channel. You must stop and
* restart the stream for the changes to take effect.
*
*
* @param updateChannelRequest
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.UpdateChannel
* @see AWS API
* Documentation
*/
default CompletableFuture updateChannel(UpdateChannelRequest updateChannelRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a channel's configuration. This does not affect an ongoing stream of this channel. You must stop and
* restart the stream for the changes to take effect.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateChannelRequest.Builder} avoiding the need to
* create one manually via {@link UpdateChannelRequest#builder()}
*
*
* @param updateChannelRequest
* A {@link Consumer} that will call methods on {@link UpdateChannelRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateChannel operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions.
*
* - ResourceNotFoundException
* - AccessDeniedException
* - ValidationException
* - PendingVerificationException
* - ConflictException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvsException Base class for all service exceptions. Unknown exceptions will be thrown as an instance
* of this type.
*
* @sample IvsAsyncClient.UpdateChannel
* @see AWS API
* Documentation
*/
default CompletableFuture updateChannel(Consumer updateChannelRequest) {
return updateChannel(UpdateChannelRequest.builder().applyMutation(updateChannelRequest).build());
}
}