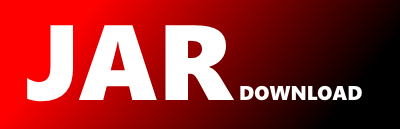
software.amazon.awssdk.services.ivs.model.StreamSession Maven / Gradle / Ivy
Show all versions of ivs Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ivs.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Object that captures the Amazon IVS configuration that the customer provisioned, the ingest configurations that the
* broadcaster used, and the most recent Amazon IVS stream events it encountered.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class StreamSession implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField STREAM_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("streamId").getter(getter(StreamSession::streamId)).setter(setter(Builder::streamId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("streamId").build()).build();
private static final SdkField START_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("startTime")
.getter(getter(StreamSession::startTime))
.setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField END_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("endTime")
.getter(getter(StreamSession::endTime))
.setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField CHANNEL_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("channel").getter(getter(StreamSession::channel)).setter(setter(Builder::channel))
.constructor(Channel::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("channel").build()).build();
private static final SdkField INGEST_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ingestConfiguration")
.getter(getter(StreamSession::ingestConfiguration)).setter(setter(Builder::ingestConfiguration))
.constructor(IngestConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ingestConfiguration").build())
.build();
private static final SdkField RECORDING_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("recordingConfiguration")
.getter(getter(StreamSession::recordingConfiguration)).setter(setter(Builder::recordingConfiguration))
.constructor(RecordingConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("recordingConfiguration").build())
.build();
private static final SdkField> TRUNCATED_EVENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("truncatedEvents")
.getter(getter(StreamSession::truncatedEvents))
.setter(setter(Builder::truncatedEvents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("truncatedEvents").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(StreamEvent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STREAM_ID_FIELD,
START_TIME_FIELD, END_TIME_FIELD, CHANNEL_FIELD, INGEST_CONFIGURATION_FIELD, RECORDING_CONFIGURATION_FIELD,
TRUNCATED_EVENTS_FIELD));
private static final long serialVersionUID = 1L;
private final String streamId;
private final Instant startTime;
private final Instant endTime;
private final Channel channel;
private final IngestConfiguration ingestConfiguration;
private final RecordingConfiguration recordingConfiguration;
private final List truncatedEvents;
private StreamSession(BuilderImpl builder) {
this.streamId = builder.streamId;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.channel = builder.channel;
this.ingestConfiguration = builder.ingestConfiguration;
this.recordingConfiguration = builder.recordingConfiguration;
this.truncatedEvents = builder.truncatedEvents;
}
/**
*
* Unique identifier for a live or previously live stream in the specified channel.
*
*
* @return Unique identifier for a live or previously live stream in the specified channel.
*/
public final String streamId() {
return streamId;
}
/**
*
* Time when the channel went live. This is an ISO 8601 timestamp; note that this is returned as a string.
*
*
* @return Time when the channel went live. This is an ISO 8601 timestamp; note that this is returned as a
* string.
*/
public final Instant startTime() {
return startTime;
}
/**
*
* Time when the channel went offline. This is an ISO 8601 timestamp; note that this is returned as a string.
* For live streams, this is NULL
.
*
*
* @return Time when the channel went offline. This is an ISO 8601 timestamp; note that this is returned as a
* string. For live streams, this is NULL
.
*/
public final Instant endTime() {
return endTime;
}
/**
*
* The properties of the channel at the time of going live.
*
*
* @return The properties of the channel at the time of going live.
*/
public final Channel channel() {
return channel;
}
/**
*
* The properties of the incoming RTMP stream for the stream.
*
*
* @return The properties of the incoming RTMP stream for the stream.
*/
public final IngestConfiguration ingestConfiguration() {
return ingestConfiguration;
}
/**
*
* The properties of recording the live stream.
*
*
* @return The properties of recording the live stream.
*/
public final RecordingConfiguration recordingConfiguration() {
return recordingConfiguration;
}
/**
* For responses, this returns true if the service returned a value for the TruncatedEvents property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasTruncatedEvents() {
return truncatedEvents != null && !(truncatedEvents instanceof SdkAutoConstructList);
}
/**
*
* List of Amazon IVS events that the stream encountered. The list is sorted by most recent events and contains up
* to 500 events. For Amazon IVS events, see Using Amazon EventBridge with Amazon
* IVS.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTruncatedEvents} method.
*
*
* @return List of Amazon IVS events that the stream encountered. The list is sorted by most recent events and
* contains up to 500 events. For Amazon IVS events, see Using Amazon EventBridge with
* Amazon IVS.
*/
public final List truncatedEvents() {
return truncatedEvents;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(streamId());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(channel());
hashCode = 31 * hashCode + Objects.hashCode(ingestConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(recordingConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(hasTruncatedEvents() ? truncatedEvents() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StreamSession)) {
return false;
}
StreamSession other = (StreamSession) obj;
return Objects.equals(streamId(), other.streamId()) && Objects.equals(startTime(), other.startTime())
&& Objects.equals(endTime(), other.endTime()) && Objects.equals(channel(), other.channel())
&& Objects.equals(ingestConfiguration(), other.ingestConfiguration())
&& Objects.equals(recordingConfiguration(), other.recordingConfiguration())
&& hasTruncatedEvents() == other.hasTruncatedEvents()
&& Objects.equals(truncatedEvents(), other.truncatedEvents());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StreamSession").add("StreamId", streamId()).add("StartTime", startTime())
.add("EndTime", endTime()).add("Channel", channel()).add("IngestConfiguration", ingestConfiguration())
.add("RecordingConfiguration", recordingConfiguration())
.add("TruncatedEvents", hasTruncatedEvents() ? truncatedEvents() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "streamId":
return Optional.ofNullable(clazz.cast(streamId()));
case "startTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "endTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "channel":
return Optional.ofNullable(clazz.cast(channel()));
case "ingestConfiguration":
return Optional.ofNullable(clazz.cast(ingestConfiguration()));
case "recordingConfiguration":
return Optional.ofNullable(clazz.cast(recordingConfiguration()));
case "truncatedEvents":
return Optional.ofNullable(clazz.cast(truncatedEvents()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function