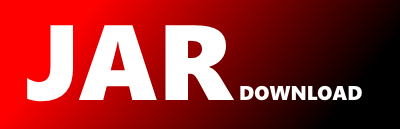
software.amazon.awssdk.services.ivschat.DefaultIvschatAsyncClient Maven / Gradle / Ivy
Show all versions of ivschat Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ivschat;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import java.util.function.Function;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.awscore.client.handler.AwsAsyncClientHandler;
import software.amazon.awssdk.awscore.exception.AwsServiceException;
import software.amazon.awssdk.awscore.internal.AwsProtocolMetadata;
import software.amazon.awssdk.awscore.internal.AwsServiceProtocol;
import software.amazon.awssdk.awscore.retry.AwsRetryStrategy;
import software.amazon.awssdk.core.RequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkPlugin;
import software.amazon.awssdk.core.SdkRequest;
import software.amazon.awssdk.core.client.config.ClientOverrideConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientConfiguration;
import software.amazon.awssdk.core.client.config.SdkClientOption;
import software.amazon.awssdk.core.client.handler.AsyncClientHandler;
import software.amazon.awssdk.core.client.handler.ClientExecutionParams;
import software.amazon.awssdk.core.http.HttpResponseHandler;
import software.amazon.awssdk.core.metrics.CoreMetric;
import software.amazon.awssdk.core.retry.RetryMode;
import software.amazon.awssdk.metrics.MetricCollector;
import software.amazon.awssdk.metrics.MetricPublisher;
import software.amazon.awssdk.metrics.NoOpMetricCollector;
import software.amazon.awssdk.protocols.core.ExceptionMetadata;
import software.amazon.awssdk.protocols.json.AwsJsonProtocol;
import software.amazon.awssdk.protocols.json.AwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.BaseAwsJsonProtocolFactory;
import software.amazon.awssdk.protocols.json.JsonOperationMetadata;
import software.amazon.awssdk.retries.api.RetryStrategy;
import software.amazon.awssdk.services.ivschat.internal.IvschatServiceClientConfigurationBuilder;
import software.amazon.awssdk.services.ivschat.model.AccessDeniedException;
import software.amazon.awssdk.services.ivschat.model.ConflictException;
import software.amazon.awssdk.services.ivschat.model.CreateChatTokenRequest;
import software.amazon.awssdk.services.ivschat.model.CreateChatTokenResponse;
import software.amazon.awssdk.services.ivschat.model.CreateLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.CreateLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.CreateRoomRequest;
import software.amazon.awssdk.services.ivschat.model.CreateRoomResponse;
import software.amazon.awssdk.services.ivschat.model.DeleteLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.DeleteLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.DeleteMessageRequest;
import software.amazon.awssdk.services.ivschat.model.DeleteMessageResponse;
import software.amazon.awssdk.services.ivschat.model.DeleteRoomRequest;
import software.amazon.awssdk.services.ivschat.model.DeleteRoomResponse;
import software.amazon.awssdk.services.ivschat.model.DisconnectUserRequest;
import software.amazon.awssdk.services.ivschat.model.DisconnectUserResponse;
import software.amazon.awssdk.services.ivschat.model.GetLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.GetLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.GetRoomRequest;
import software.amazon.awssdk.services.ivschat.model.GetRoomResponse;
import software.amazon.awssdk.services.ivschat.model.InternalServerException;
import software.amazon.awssdk.services.ivschat.model.IvschatException;
import software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest;
import software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsResponse;
import software.amazon.awssdk.services.ivschat.model.ListRoomsRequest;
import software.amazon.awssdk.services.ivschat.model.ListRoomsResponse;
import software.amazon.awssdk.services.ivschat.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ivschat.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ivschat.model.PendingVerificationException;
import software.amazon.awssdk.services.ivschat.model.ResourceNotFoundException;
import software.amazon.awssdk.services.ivschat.model.SendEventRequest;
import software.amazon.awssdk.services.ivschat.model.SendEventResponse;
import software.amazon.awssdk.services.ivschat.model.ServiceQuotaExceededException;
import software.amazon.awssdk.services.ivschat.model.TagResourceRequest;
import software.amazon.awssdk.services.ivschat.model.TagResourceResponse;
import software.amazon.awssdk.services.ivschat.model.ThrottlingException;
import software.amazon.awssdk.services.ivschat.model.UntagResourceRequest;
import software.amazon.awssdk.services.ivschat.model.UntagResourceResponse;
import software.amazon.awssdk.services.ivschat.model.UpdateLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.UpdateLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.UpdateRoomRequest;
import software.amazon.awssdk.services.ivschat.model.UpdateRoomResponse;
import software.amazon.awssdk.services.ivschat.model.ValidationException;
import software.amazon.awssdk.services.ivschat.transform.CreateChatTokenRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.CreateLoggingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.CreateRoomRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.DeleteLoggingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.DeleteMessageRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.DeleteRoomRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.DisconnectUserRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.GetLoggingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.GetRoomRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.ListLoggingConfigurationsRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.ListRoomsRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.ListTagsForResourceRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.SendEventRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.TagResourceRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.UntagResourceRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.UpdateLoggingConfigurationRequestMarshaller;
import software.amazon.awssdk.services.ivschat.transform.UpdateRoomRequestMarshaller;
import software.amazon.awssdk.utils.CompletableFutureUtils;
/**
* Internal implementation of {@link IvschatAsyncClient}.
*
* @see IvschatAsyncClient#builder()
*/
@Generated("software.amazon.awssdk:codegen")
@SdkInternalApi
final class DefaultIvschatAsyncClient implements IvschatAsyncClient {
private static final Logger log = LoggerFactory.getLogger(DefaultIvschatAsyncClient.class);
private static final AwsProtocolMetadata protocolMetadata = AwsProtocolMetadata.builder()
.serviceProtocol(AwsServiceProtocol.REST_JSON).build();
private final AsyncClientHandler clientHandler;
private final AwsJsonProtocolFactory protocolFactory;
private final SdkClientConfiguration clientConfiguration;
protected DefaultIvschatAsyncClient(SdkClientConfiguration clientConfiguration) {
this.clientHandler = new AwsAsyncClientHandler(clientConfiguration);
this.clientConfiguration = clientConfiguration.toBuilder().option(SdkClientOption.SDK_CLIENT, this).build();
this.protocolFactory = init(AwsJsonProtocolFactory.builder()).build();
}
/**
*
* Creates an encrypted token that is used by a chat participant to establish an individual WebSocket chat
* connection to a room. When the token is used to connect to chat, the connection is valid for the session duration
* specified in the request. The token becomes invalid at the token-expiration timestamp included in the response.
*
*
* Use the capabilities
field to permit an end user to send messages or moderate a room.
*
*
* The attributes
field securely attaches structured data to the chat session; the data is included
* within each message sent by the end user and received by other participants in the room. Common use cases for
* attributes include passing end-user profile data like an icon, display name, colors, badges, and other display
* features.
*
*
* Encryption keys are owned by Amazon IVS Chat and never used directly by your application.
*
*
* @param createChatTokenRequest
* @return A Java Future containing the result of the CreateChatToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateChatToken
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createChatToken(CreateChatTokenRequest createChatTokenRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createChatTokenRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createChatTokenRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateChatToken");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateChatTokenResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateChatToken").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateChatTokenRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createChatTokenRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a logging configuration that allows clients to store and record sent messages.
*
*
* @param createLoggingConfigurationRequest
* @return A Java Future containing the result of the CreateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - ServiceQuotaExceededException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture createLoggingConfiguration(
CreateLoggingConfigurationRequest createLoggingConfigurationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createLoggingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createLoggingConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateLoggingConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, CreateLoggingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("CreateLoggingConfiguration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateLoggingConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createLoggingConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Creates a room that allows clients to connect and pass messages.
*
*
* @param createRoomRequest
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - ServiceQuotaExceededException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateRoom
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture createRoom(CreateRoomRequest createRoomRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(createRoomRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, createRoomRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "CreateRoom");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
CreateRoomResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("CreateRoom")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new CreateRoomRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(createRoomRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified logging configuration.
*
*
* @param deleteLoggingConfigurationRequest
* @return A Java Future containing the result of the DeleteLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture deleteLoggingConfiguration(
DeleteLoggingConfigurationRequest deleteLoggingConfigurationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteLoggingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteLoggingConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteLoggingConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DeleteLoggingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteLoggingConfiguration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteLoggingConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteLoggingConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sends an event to a specific room which directs clients to delete a specific message; that is, unrender it from
* view and delete it from the client’s chat history. This event’s EventName
is
* aws:DELETE_MESSAGE
. This replicates the
* DeleteMessage WebSocket operation in the Amazon IVS Chat Messaging API.
*
*
* @param deleteMessageRequest
* @return A Java Future containing the result of the DeleteMessage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteMessage
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteMessage(DeleteMessageRequest deleteMessageRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteMessageRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteMessageRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteMessage");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteMessageResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DeleteMessage").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteMessageRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteMessageRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Deletes the specified room.
*
*
* @param deleteRoomRequest
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteRoom
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture deleteRoom(DeleteRoomRequest deleteRoomRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(deleteRoomRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, deleteRoomRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DeleteRoom");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
DeleteRoomResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("DeleteRoom")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new DeleteRoomRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(deleteRoomRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Disconnects all connections using a specified user ID from a room. This replicates the
* DisconnectUser WebSocket operation in the Amazon IVS Chat Messaging API.
*
*
* @param disconnectUserRequest
* @return A Java Future containing the result of the DisconnectUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DisconnectUser
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture disconnectUser(DisconnectUserRequest disconnectUserRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(disconnectUserRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, disconnectUserRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "DisconnectUser");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, DisconnectUserResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("DisconnectUser").withProtocolMetadata(protocolMetadata)
.withMarshaller(new DisconnectUserRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(disconnectUserRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the specified logging configuration.
*
*
* @param getLoggingConfigurationRequest
* @return A Java Future containing the result of the GetLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.GetLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture getLoggingConfiguration(
GetLoggingConfigurationRequest getLoggingConfigurationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getLoggingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getLoggingConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetLoggingConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, GetLoggingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("GetLoggingConfiguration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new GetLoggingConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getLoggingConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets the specified room.
*
*
* @param getRoomRequest
* @return A Java Future containing the result of the GetRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.GetRoom
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture getRoom(GetRoomRequest getRoomRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(getRoomRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, getRoomRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "GetRoom");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
GetRoomResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("GetRoom")
.withProtocolMetadata(protocolMetadata).withMarshaller(new GetRoomRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(getRoomRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets summary information about all your logging configurations in the AWS region where the API request is
* processed.
*
*
* @param listLoggingConfigurationsRequest
* @return A Java Future containing the result of the ListLoggingConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListLoggingConfigurations
* @see AWS API Documentation
*/
@Override
public CompletableFuture listLoggingConfigurations(
ListLoggingConfigurationsRequest listLoggingConfigurationsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listLoggingConfigurationsRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listLoggingConfigurationsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListLoggingConfigurations");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListLoggingConfigurationsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListLoggingConfigurations").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListLoggingConfigurationsRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listLoggingConfigurationsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets summary information about all your rooms in the AWS region where the API request is processed. Results are
* sorted in descending order of updateTime
.
*
*
* @param listRoomsRequest
* @return A Java Future containing the result of the ListRooms operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListRooms
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture listRooms(ListRoomsRequest listRoomsRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listRoomsRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listRoomsRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListRooms");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
ListRoomsResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("ListRooms")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListRoomsRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(listRoomsRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Gets information about AWS tags for the specified ARN.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
@Override
public CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(listTagsForResourceRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, listTagsForResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "ListTagsForResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, ListTagsForResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("ListTagsForResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new ListTagsForResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(listTagsForResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Sends an event to a room. Use this within your application’s business logic to send events to clients of a room;
* e.g., to notify clients to change the way the chat UI is rendered.
*
*
* @param sendEventRequest
* @return A Java Future containing the result of the SendEvent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.SendEvent
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture sendEvent(SendEventRequest sendEventRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(sendEventRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, sendEventRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "SendEvent");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
SendEventResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("SendEvent")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new SendEventRequestMarshaller(protocolFactory)).withResponseHandler(responseHandler)
.withErrorResponseHandler(errorResponseHandler).withRequestConfiguration(clientConfiguration)
.withMetricCollector(apiCallMetricCollector).withInput(sendEventRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Adds or updates tags for the AWS resource with the specified ARN.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.TagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(tagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, tagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "TagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
TagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("TagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new TagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(tagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Removes tags from the resource with the specified ARN.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(untagResourceRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, untagResourceRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UntagResource");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UntagResourceResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UntagResource").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UntagResourceRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(untagResourceRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates a specified logging configuration.
*
*
* @param updateLoggingConfigurationRequest
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public CompletableFuture updateLoggingConfiguration(
UpdateLoggingConfigurationRequest updateLoggingConfigurationRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateLoggingConfigurationRequest,
this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateLoggingConfigurationRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateLoggingConfiguration");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(
operationMetadata, UpdateLoggingConfigurationResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams()
.withOperationName("UpdateLoggingConfiguration").withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateLoggingConfigurationRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateLoggingConfigurationRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
/**
*
* Updates a room’s configuration.
*
*
* @param updateRoomRequest
* @return A Java Future containing the result of the UpdateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UpdateRoom
* @see AWS API
* Documentation
*/
@Override
public CompletableFuture updateRoom(UpdateRoomRequest updateRoomRequest) {
SdkClientConfiguration clientConfiguration = updateSdkClientConfiguration(updateRoomRequest, this.clientConfiguration);
List metricPublishers = resolveMetricPublishers(clientConfiguration, updateRoomRequest
.overrideConfiguration().orElse(null));
MetricCollector apiCallMetricCollector = metricPublishers.isEmpty() ? NoOpMetricCollector.create() : MetricCollector
.create("ApiCall");
try {
apiCallMetricCollector.reportMetric(CoreMetric.SERVICE_ID, "ivschat");
apiCallMetricCollector.reportMetric(CoreMetric.OPERATION_NAME, "UpdateRoom");
JsonOperationMetadata operationMetadata = JsonOperationMetadata.builder().hasStreamingSuccessResponse(false)
.isPayloadJson(true).build();
HttpResponseHandler responseHandler = protocolFactory.createResponseHandler(operationMetadata,
UpdateRoomResponse::builder);
HttpResponseHandler errorResponseHandler = createErrorResponseHandler(protocolFactory,
operationMetadata);
CompletableFuture executeFuture = clientHandler
.execute(new ClientExecutionParams().withOperationName("UpdateRoom")
.withProtocolMetadata(protocolMetadata)
.withMarshaller(new UpdateRoomRequestMarshaller(protocolFactory))
.withResponseHandler(responseHandler).withErrorResponseHandler(errorResponseHandler)
.withRequestConfiguration(clientConfiguration).withMetricCollector(apiCallMetricCollector)
.withInput(updateRoomRequest));
CompletableFuture whenCompleted = executeFuture.whenComplete((r, e) -> {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
});
executeFuture = CompletableFutureUtils.forwardExceptionTo(whenCompleted, executeFuture);
return executeFuture;
} catch (Throwable t) {
metricPublishers.forEach(p -> p.publish(apiCallMetricCollector.collect()));
return CompletableFutureUtils.failedFuture(t);
}
}
@Override
public final IvschatServiceClientConfiguration serviceClientConfiguration() {
return new IvschatServiceClientConfigurationBuilder(this.clientConfiguration.toBuilder()).build();
}
@Override
public final String serviceName() {
return SERVICE_NAME;
}
private > T init(T builder) {
return builder
.clientConfiguration(clientConfiguration)
.defaultServiceExceptionSupplier(IvschatException::builder)
.protocol(AwsJsonProtocol.REST_JSON)
.protocolVersion("1.1")
.registerModeledException(
ExceptionMetadata.builder().errorCode("AccessDeniedException")
.exceptionBuilderSupplier(AccessDeniedException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ConflictException")
.exceptionBuilderSupplier(ConflictException::builder).httpStatusCode(409).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ResourceNotFoundException")
.exceptionBuilderSupplier(ResourceNotFoundException::builder).httpStatusCode(404).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ThrottlingException")
.exceptionBuilderSupplier(ThrottlingException::builder).httpStatusCode(429).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ValidationException")
.exceptionBuilderSupplier(ValidationException::builder).httpStatusCode(400).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("ServiceQuotaExceededException")
.exceptionBuilderSupplier(ServiceQuotaExceededException::builder).httpStatusCode(402).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("PendingVerification")
.exceptionBuilderSupplier(PendingVerificationException::builder).httpStatusCode(403).build())
.registerModeledException(
ExceptionMetadata.builder().errorCode("InternalServerException")
.exceptionBuilderSupplier(InternalServerException::builder).httpStatusCode(500).build());
}
private static List resolveMetricPublishers(SdkClientConfiguration clientConfiguration,
RequestOverrideConfiguration requestOverrideConfiguration) {
List publishers = null;
if (requestOverrideConfiguration != null) {
publishers = requestOverrideConfiguration.metricPublishers();
}
if (publishers == null || publishers.isEmpty()) {
publishers = clientConfiguration.option(SdkClientOption.METRIC_PUBLISHERS);
}
if (publishers == null) {
publishers = Collections.emptyList();
}
return publishers;
}
private void updateRetryStrategyClientConfiguration(SdkClientConfiguration.Builder configuration) {
ClientOverrideConfiguration.Builder builder = configuration.asOverrideConfigurationBuilder();
RetryMode retryMode = builder.retryMode();
if (retryMode != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, AwsRetryStrategy.forRetryMode(retryMode));
} else {
Consumer> configurator = builder.retryStrategyConfigurator();
if (configurator != null) {
RetryStrategy.Builder, ?> defaultBuilder = AwsRetryStrategy.defaultRetryStrategy().toBuilder();
configurator.accept(defaultBuilder);
configuration.option(SdkClientOption.RETRY_STRATEGY, defaultBuilder.build());
} else {
RetryStrategy retryStrategy = builder.retryStrategy();
if (retryStrategy != null) {
configuration.option(SdkClientOption.RETRY_STRATEGY, retryStrategy);
}
}
}
configuration.option(SdkClientOption.CONFIGURED_RETRY_MODE, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_STRATEGY, null);
configuration.option(SdkClientOption.CONFIGURED_RETRY_CONFIGURATOR, null);
}
private SdkClientConfiguration updateSdkClientConfiguration(SdkRequest request, SdkClientConfiguration clientConfiguration) {
List plugins = request.overrideConfiguration().map(c -> c.plugins()).orElse(Collections.emptyList());
SdkClientConfiguration.Builder configuration = clientConfiguration.toBuilder();
if (plugins.isEmpty()) {
return configuration.build();
}
IvschatServiceClientConfigurationBuilder serviceConfigBuilder = new IvschatServiceClientConfigurationBuilder(
configuration);
for (SdkPlugin plugin : plugins) {
plugin.configureClient(serviceConfigBuilder);
}
updateRetryStrategyClientConfiguration(configuration);
return configuration.build();
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata) {
return protocolFactory.createErrorResponseHandler(operationMetadata);
}
private HttpResponseHandler createErrorResponseHandler(BaseAwsJsonProtocolFactory protocolFactory,
JsonOperationMetadata operationMetadata, Function> exceptionMetadataMapper) {
return protocolFactory.createErrorResponseHandler(operationMetadata, exceptionMetadataMapper);
}
@Override
public void close() {
clientHandler.close();
}
}