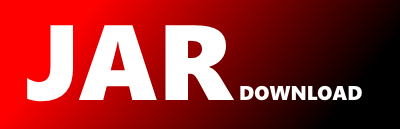
software.amazon.awssdk.services.ivschat.IvschatAsyncClient Maven / Gradle / Ivy
Show all versions of ivschat Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.ivschat;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.services.ivschat.model.CreateChatTokenRequest;
import software.amazon.awssdk.services.ivschat.model.CreateChatTokenResponse;
import software.amazon.awssdk.services.ivschat.model.CreateLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.CreateLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.CreateRoomRequest;
import software.amazon.awssdk.services.ivschat.model.CreateRoomResponse;
import software.amazon.awssdk.services.ivschat.model.DeleteLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.DeleteLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.DeleteMessageRequest;
import software.amazon.awssdk.services.ivschat.model.DeleteMessageResponse;
import software.amazon.awssdk.services.ivschat.model.DeleteRoomRequest;
import software.amazon.awssdk.services.ivschat.model.DeleteRoomResponse;
import software.amazon.awssdk.services.ivschat.model.DisconnectUserRequest;
import software.amazon.awssdk.services.ivschat.model.DisconnectUserResponse;
import software.amazon.awssdk.services.ivschat.model.GetLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.GetLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.GetRoomRequest;
import software.amazon.awssdk.services.ivschat.model.GetRoomResponse;
import software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest;
import software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsResponse;
import software.amazon.awssdk.services.ivschat.model.ListRoomsRequest;
import software.amazon.awssdk.services.ivschat.model.ListRoomsResponse;
import software.amazon.awssdk.services.ivschat.model.ListTagsForResourceRequest;
import software.amazon.awssdk.services.ivschat.model.ListTagsForResourceResponse;
import software.amazon.awssdk.services.ivschat.model.SendEventRequest;
import software.amazon.awssdk.services.ivschat.model.SendEventResponse;
import software.amazon.awssdk.services.ivschat.model.TagResourceRequest;
import software.amazon.awssdk.services.ivschat.model.TagResourceResponse;
import software.amazon.awssdk.services.ivschat.model.UntagResourceRequest;
import software.amazon.awssdk.services.ivschat.model.UntagResourceResponse;
import software.amazon.awssdk.services.ivschat.model.UpdateLoggingConfigurationRequest;
import software.amazon.awssdk.services.ivschat.model.UpdateLoggingConfigurationResponse;
import software.amazon.awssdk.services.ivschat.model.UpdateRoomRequest;
import software.amazon.awssdk.services.ivschat.model.UpdateRoomResponse;
import software.amazon.awssdk.services.ivschat.paginators.ListLoggingConfigurationsPublisher;
import software.amazon.awssdk.services.ivschat.paginators.ListRoomsPublisher;
/**
* Service client for accessing ivschat asynchronously. This can be created using the static {@link #builder()}
* method.The asynchronous client performs non-blocking I/O when configured with any {@code SdkAsyncHttpClient}
* supported in the SDK. However, full non-blocking is not guaranteed as the async client may perform blocking calls in
* some cases such as credentials retrieval and endpoint discovery as part of the async API call.
*
*
* Introduction
*
*
* The Amazon IVS Chat control-plane API enables you to create and manage Amazon IVS Chat resources. You also need to
* integrate with the
* Amazon IVS Chat Messaging API, to enable users to interact with chat rooms in real time.
*
*
* The API is an AWS regional service. For a list of supported regions and Amazon IVS Chat HTTPS service endpoints, see
* the Amazon IVS Chat information on the Amazon IVS
* page in the AWS General Reference.
*
*
* This document describes HTTP operations. There is a separate messaging API for managing Chat resources; see
* the Amazon IVS Chat
* Messaging API Reference.
*
*
* Notes on terminology:
*
*
* -
*
* You create service applications using the Amazon IVS Chat API. We refer to these as applications.
*
*
* -
*
* You create front-end client applications (browser and Android/iOS apps) using the Amazon IVS Chat Messaging API. We
* refer to these as clients.
*
*
*
*
* Resources
*
*
* The following resources are part of Amazon IVS Chat:
*
*
* -
*
* LoggingConfiguration — A configuration that allows customers to store and record sent messages in a chat room.
* See the Logging Configuration endpoints for more information.
*
*
* -
*
* Room — The central Amazon IVS Chat resource through which clients connect to and exchange chat messages. See
* the Room endpoints for more information.
*
*
*
*
* Tagging
*
*
* A tag is a metadata label that you assign to an AWS resource. A tag comprises a key and a value,
* both set by you. For example, you might set a tag as topic:nature
to label a particular video category.
* See Best practices
* and strategies in Tagging Amazon Web Services Resources and Tag Editor for details, including restrictions
* that apply to tags and "Tag naming limits and requirements"; Amazon IVS Chat has no service-specific constraints
* beyond what is documented there.
*
*
* Tags can help you identify and organize your AWS resources. For example, you can use the same tag for different
* resources to indicate that they are related. You can also use tags to manage access (see Access Tags).
*
*
* The Amazon IVS Chat API has these tag-related operations: TagResource, UntagResource, and
* ListTagsForResource. The following resource supports tagging: Room.
*
*
* At most 50 tags can be applied to a resource.
*
*
* API Access Security
*
*
* Your Amazon IVS Chat applications (service applications and clients) must be authenticated and authorized to access
* Amazon IVS Chat resources. Note the differences between these concepts:
*
*
* -
*
* Authentication is about verifying identity. Requests to the Amazon IVS Chat API must be signed to verify your
* identity.
*
*
* -
*
* Authorization is about granting permissions. Your IAM roles need to have permissions for Amazon IVS Chat API
* requests.
*
*
*
*
* Users (viewers) connect to a room using secure access tokens that you create using the CreateChatToken
* operation through the AWS SDK. You call CreateChatToken for every user’s chat session, passing identity and
* authorization information about the user.
*
*
* Signing API Requests
*
*
* HTTP API requests must be signed with an AWS SigV4 signature using your AWS security credentials. The AWS Command
* Line Interface (CLI) and the AWS SDKs take care of signing the underlying API calls for you. However, if your
* application calls the Amazon IVS Chat HTTP API directly, it’s your responsibility to sign the requests.
*
*
* You generate a signature using valid AWS credentials for an IAM role that has permission to perform the requested
* action. For example, DeleteMessage requests must be made using an IAM role that has the
* ivschat:DeleteMessage
permission.
*
*
* For more information:
*
*
* -
*
* Authentication and generating signatures — See Authenticating Requests
* (Amazon Web Services Signature Version 4) in the Amazon Web Services General Reference.
*
*
* -
*
* Managing Amazon IVS permissions — See Identity and Access Management on the
* Security page of the Amazon IVS User Guide.
*
*
*
*
* Amazon Resource Names (ARNs)
*
*
* ARNs uniquely identify AWS resources. An ARN is required when you need to specify a resource unambiguously across all
* of AWS, such as in IAM policies and API calls. For more information, see Amazon Resource Names in the
* AWS General Reference.
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface IvschatAsyncClient extends AwsClient {
String SERVICE_NAME = "ivschat";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "ivschat";
/**
*
* Creates an encrypted token that is used by a chat participant to establish an individual WebSocket chat
* connection to a room. When the token is used to connect to chat, the connection is valid for the session duration
* specified in the request. The token becomes invalid at the token-expiration timestamp included in the response.
*
*
* Use the capabilities
field to permit an end user to send messages or moderate a room.
*
*
* The attributes
field securely attaches structured data to the chat session; the data is included
* within each message sent by the end user and received by other participants in the room. Common use cases for
* attributes include passing end-user profile data like an icon, display name, colors, badges, and other display
* features.
*
*
* Encryption keys are owned by Amazon IVS Chat and never used directly by your application.
*
*
* @param createChatTokenRequest
* @return A Java Future containing the result of the CreateChatToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateChatToken
* @see AWS API
* Documentation
*/
default CompletableFuture createChatToken(CreateChatTokenRequest createChatTokenRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates an encrypted token that is used by a chat participant to establish an individual WebSocket chat
* connection to a room. When the token is used to connect to chat, the connection is valid for the session duration
* specified in the request. The token becomes invalid at the token-expiration timestamp included in the response.
*
*
* Use the capabilities
field to permit an end user to send messages or moderate a room.
*
*
* The attributes
field securely attaches structured data to the chat session; the data is included
* within each message sent by the end user and received by other participants in the room. Common use cases for
* attributes include passing end-user profile data like an icon, display name, colors, badges, and other display
* features.
*
*
* Encryption keys are owned by Amazon IVS Chat and never used directly by your application.
*
*
*
* This is a convenience which creates an instance of the {@link CreateChatTokenRequest.Builder} avoiding the need
* to create one manually via {@link CreateChatTokenRequest#builder()}
*
*
* @param createChatTokenRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.CreateChatTokenRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateChatToken operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateChatToken
* @see AWS API
* Documentation
*/
default CompletableFuture createChatToken(
Consumer createChatTokenRequest) {
return createChatToken(CreateChatTokenRequest.builder().applyMutation(createChatTokenRequest).build());
}
/**
*
* Creates a logging configuration that allows clients to store and record sent messages.
*
*
* @param createLoggingConfigurationRequest
* @return A Java Future containing the result of the CreateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - ServiceQuotaExceededException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture createLoggingConfiguration(
CreateLoggingConfigurationRequest createLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a logging configuration that allows clients to store and record sent messages.
*
*
*
* This is a convenience which creates an instance of the {@link CreateLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link CreateLoggingConfigurationRequest#builder()}
*
*
* @param createLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.CreateLoggingConfigurationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the CreateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - ServiceQuotaExceededException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture createLoggingConfiguration(
Consumer createLoggingConfigurationRequest) {
return createLoggingConfiguration(CreateLoggingConfigurationRequest.builder()
.applyMutation(createLoggingConfigurationRequest).build());
}
/**
*
* Creates a room that allows clients to connect and pass messages.
*
*
* @param createRoomRequest
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - ServiceQuotaExceededException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateRoom
* @see AWS API
* Documentation
*/
default CompletableFuture createRoom(CreateRoomRequest createRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a room that allows clients to connect and pass messages.
*
*
*
* This is a convenience which creates an instance of the {@link CreateRoomRequest.Builder} avoiding the need to
* create one manually via {@link CreateRoomRequest#builder()}
*
*
* @param createRoomRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.CreateRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - ServiceQuotaExceededException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.CreateRoom
* @see AWS API
* Documentation
*/
default CompletableFuture createRoom(Consumer createRoomRequest) {
return createRoom(CreateRoomRequest.builder().applyMutation(createRoomRequest).build());
}
/**
*
* Deletes the specified logging configuration.
*
*
* @param deleteLoggingConfigurationRequest
* @return A Java Future containing the result of the DeleteLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteLoggingConfiguration(
DeleteLoggingConfigurationRequest deleteLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified logging configuration.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link DeleteLoggingConfigurationRequest#builder()}
*
*
* @param deleteLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.DeleteLoggingConfigurationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the DeleteLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture deleteLoggingConfiguration(
Consumer deleteLoggingConfigurationRequest) {
return deleteLoggingConfiguration(DeleteLoggingConfigurationRequest.builder()
.applyMutation(deleteLoggingConfigurationRequest).build());
}
/**
*
* Sends an event to a specific room which directs clients to delete a specific message; that is, unrender it from
* view and delete it from the client’s chat history. This event’s EventName
is
* aws:DELETE_MESSAGE
. This replicates the
* DeleteMessage WebSocket operation in the Amazon IVS Chat Messaging API.
*
*
* @param deleteMessageRequest
* @return A Java Future containing the result of the DeleteMessage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteMessage
* @see AWS API
* Documentation
*/
default CompletableFuture deleteMessage(DeleteMessageRequest deleteMessageRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sends an event to a specific room which directs clients to delete a specific message; that is, unrender it from
* view and delete it from the client’s chat history. This event’s EventName
is
* aws:DELETE_MESSAGE
. This replicates the
* DeleteMessage WebSocket operation in the Amazon IVS Chat Messaging API.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteMessageRequest.Builder} avoiding the need to
* create one manually via {@link DeleteMessageRequest#builder()}
*
*
* @param deleteMessageRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.DeleteMessageRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteMessage operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteMessage
* @see AWS API
* Documentation
*/
default CompletableFuture deleteMessage(Consumer deleteMessageRequest) {
return deleteMessage(DeleteMessageRequest.builder().applyMutation(deleteMessageRequest).build());
}
/**
*
* Deletes the specified room.
*
*
* @param deleteRoomRequest
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteRoom
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRoom(DeleteRoomRequest deleteRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Deletes the specified room.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteRoomRequest.Builder} avoiding the need to
* create one manually via {@link DeleteRoomRequest#builder()}
*
*
* @param deleteRoomRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.DeleteRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DeleteRoom
* @see AWS API
* Documentation
*/
default CompletableFuture deleteRoom(Consumer deleteRoomRequest) {
return deleteRoom(DeleteRoomRequest.builder().applyMutation(deleteRoomRequest).build());
}
/**
*
* Disconnects all connections using a specified user ID from a room. This replicates the
* DisconnectUser WebSocket operation in the Amazon IVS Chat Messaging API.
*
*
* @param disconnectUserRequest
* @return A Java Future containing the result of the DisconnectUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DisconnectUser
* @see AWS API
* Documentation
*/
default CompletableFuture disconnectUser(DisconnectUserRequest disconnectUserRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Disconnects all connections using a specified user ID from a room. This replicates the
* DisconnectUser WebSocket operation in the Amazon IVS Chat Messaging API.
*
*
*
* This is a convenience which creates an instance of the {@link DisconnectUserRequest.Builder} avoiding the need to
* create one manually via {@link DisconnectUserRequest#builder()}
*
*
* @param disconnectUserRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.DisconnectUserRequest.Builder} to create a request.
* @return A Java Future containing the result of the DisconnectUser operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.DisconnectUser
* @see AWS API
* Documentation
*/
default CompletableFuture disconnectUser(Consumer disconnectUserRequest) {
return disconnectUser(DisconnectUserRequest.builder().applyMutation(disconnectUserRequest).build());
}
/**
*
* Gets the specified logging configuration.
*
*
* @param getLoggingConfigurationRequest
* @return A Java Future containing the result of the GetLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.GetLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getLoggingConfiguration(
GetLoggingConfigurationRequest getLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the specified logging configuration.
*
*
*
* This is a convenience which creates an instance of the {@link GetLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link GetLoggingConfigurationRequest#builder()}
*
*
* @param getLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.GetLoggingConfigurationRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the GetLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.GetLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture getLoggingConfiguration(
Consumer getLoggingConfigurationRequest) {
return getLoggingConfiguration(GetLoggingConfigurationRequest.builder().applyMutation(getLoggingConfigurationRequest)
.build());
}
/**
*
* Gets the specified room.
*
*
* @param getRoomRequest
* @return A Java Future containing the result of the GetRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.GetRoom
* @see AWS API
* Documentation
*/
default CompletableFuture getRoom(GetRoomRequest getRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets the specified room.
*
*
*
* This is a convenience which creates an instance of the {@link GetRoomRequest.Builder} avoiding the need to create
* one manually via {@link GetRoomRequest#builder()}
*
*
* @param getRoomRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.GetRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.GetRoom
* @see AWS API
* Documentation
*/
default CompletableFuture getRoom(Consumer getRoomRequest) {
return getRoom(GetRoomRequest.builder().applyMutation(getRoomRequest).build());
}
/**
*
* Gets summary information about all your logging configurations in the AWS region where the API request is
* processed.
*
*
* @param listLoggingConfigurationsRequest
* @return A Java Future containing the result of the ListLoggingConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListLoggingConfigurations
* @see AWS API Documentation
*/
default CompletableFuture listLoggingConfigurations(
ListLoggingConfigurationsRequest listLoggingConfigurationsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about all your logging configurations in the AWS region where the API request is
* processed.
*
*
*
* This is a convenience which creates an instance of the {@link ListLoggingConfigurationsRequest.Builder} avoiding
* the need to create one manually via {@link ListLoggingConfigurationsRequest#builder()}
*
*
* @param listLoggingConfigurationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListLoggingConfigurations operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListLoggingConfigurations
* @see AWS API Documentation
*/
default CompletableFuture listLoggingConfigurations(
Consumer listLoggingConfigurationsRequest) {
return listLoggingConfigurations(ListLoggingConfigurationsRequest.builder()
.applyMutation(listLoggingConfigurationsRequest).build());
}
/**
*
* This is a variant of
* {@link #listLoggingConfigurations(software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListLoggingConfigurationsPublisher publisher = client.listLoggingConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListLoggingConfigurationsPublisher publisher = client.listLoggingConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLoggingConfigurations(software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest)}
* operation.
*
*
* @param listLoggingConfigurationsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListLoggingConfigurations
* @see AWS API Documentation
*/
default ListLoggingConfigurationsPublisher listLoggingConfigurationsPaginator(
ListLoggingConfigurationsRequest listLoggingConfigurationsRequest) {
return new ListLoggingConfigurationsPublisher(this, listLoggingConfigurationsRequest);
}
/**
*
* This is a variant of
* {@link #listLoggingConfigurations(software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListLoggingConfigurationsPublisher publisher = client.listLoggingConfigurationsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListLoggingConfigurationsPublisher publisher = client.listLoggingConfigurationsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listLoggingConfigurations(software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest)}
* operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListLoggingConfigurationsRequest.Builder} avoiding
* the need to create one manually via {@link ListLoggingConfigurationsRequest#builder()}
*
*
* @param listLoggingConfigurationsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.ListLoggingConfigurationsRequest.Builder} to create a
* request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListLoggingConfigurations
* @see AWS API Documentation
*/
default ListLoggingConfigurationsPublisher listLoggingConfigurationsPaginator(
Consumer listLoggingConfigurationsRequest) {
return listLoggingConfigurationsPaginator(ListLoggingConfigurationsRequest.builder()
.applyMutation(listLoggingConfigurationsRequest).build());
}
/**
*
* Gets summary information about all your rooms in the AWS region where the API request is processed. Results are
* sorted in descending order of updateTime
.
*
*
* @param listRoomsRequest
* @return A Java Future containing the result of the ListRooms operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListRooms
* @see AWS API
* Documentation
*/
default CompletableFuture listRooms(ListRoomsRequest listRoomsRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets summary information about all your rooms in the AWS region where the API request is processed. Results are
* sorted in descending order of updateTime
.
*
*
*
* This is a convenience which creates an instance of the {@link ListRoomsRequest.Builder} avoiding the need to
* create one manually via {@link ListRoomsRequest#builder()}
*
*
* @param listRoomsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.ListRoomsRequest.Builder} to create a request.
* @return A Java Future containing the result of the ListRooms operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListRooms
* @see AWS API
* Documentation
*/
default CompletableFuture listRooms(Consumer listRoomsRequest) {
return listRooms(ListRoomsRequest.builder().applyMutation(listRoomsRequest).build());
}
/**
*
* This is a variant of {@link #listRooms(software.amazon.awssdk.services.ivschat.model.ListRoomsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListRoomsPublisher publisher = client.listRoomsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListRoomsPublisher publisher = client.listRoomsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivschat.model.ListRoomsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRooms(software.amazon.awssdk.services.ivschat.model.ListRoomsRequest)} operation.
*
*
* @param listRoomsRequest
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListRooms
* @see AWS API
* Documentation
*/
default ListRoomsPublisher listRoomsPaginator(ListRoomsRequest listRoomsRequest) {
return new ListRoomsPublisher(this, listRoomsRequest);
}
/**
*
* This is a variant of {@link #listRooms(software.amazon.awssdk.services.ivschat.model.ListRoomsRequest)}
* operation. The return type is a custom publisher that can be subscribed to request a stream of response pages.
* SDK will internally handle making service calls for you.
*
*
* When the operation is called, an instance of this class is returned. At this point, no service calls are made yet
* and so there is no guarantee that the request is valid. If there are errors in your request, you will see the
* failures only after you start streaming the data. The subscribe method should be called as a request to start
* streaming data. For more info, see
* {@link org.reactivestreams.Publisher#subscribe(org.reactivestreams.Subscriber)}. Each call to the subscribe
* method will result in a new {@link org.reactivestreams.Subscription} i.e., a new contract to stream data from the
* starting request.
*
*
*
* The following are few ways to use the response class:
*
* 1) Using the subscribe helper method
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListRoomsPublisher publisher = client.listRoomsPaginator(request);
* CompletableFuture future = publisher.subscribe(res -> { // Do something with the response });
* future.get();
* }
*
*
* 2) Using a custom subscriber
*
*
* {@code
* software.amazon.awssdk.services.ivschat.paginators.ListRoomsPublisher publisher = client.listRoomsPaginator(request);
* publisher.subscribe(new Subscriber() {
*
* public void onSubscribe(org.reactivestreams.Subscriber subscription) { //... };
*
*
* public void onNext(software.amazon.awssdk.services.ivschat.model.ListRoomsResponse response) { //... };
* });}
*
*
* As the response is a publisher, it can work well with third party reactive streams implementations like RxJava2.
*
* Please notice that the configuration of maxResults won't limit the number of results you get with the
* paginator. It only limits the number of results in each page.
*
*
* Note: If you prefer to have control on service calls, use the
* {@link #listRooms(software.amazon.awssdk.services.ivschat.model.ListRoomsRequest)} operation.
*
*
*
* This is a convenience which creates an instance of the {@link ListRoomsRequest.Builder} avoiding the need to
* create one manually via {@link ListRoomsRequest#builder()}
*
*
* @param listRoomsRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.ListRoomsRequest.Builder} to create a request.
* @return A custom publisher that can be subscribed to request a stream of response pages.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListRooms
* @see AWS API
* Documentation
*/
default ListRoomsPublisher listRoomsPaginator(Consumer listRoomsRequest) {
return listRoomsPaginator(ListRoomsRequest.builder().applyMutation(listRoomsRequest).build());
}
/**
*
* Gets information about AWS tags for the specified ARN.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
ListTagsForResourceRequest listTagsForResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Gets information about AWS tags for the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link ListTagsForResourceRequest.Builder} avoiding the
* need to create one manually via {@link ListTagsForResourceRequest#builder()}
*
*
* @param listTagsForResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.ListTagsForResourceRequest.Builder} to create a
* request.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.ListTagsForResource
* @see AWS
* API Documentation
*/
default CompletableFuture listTagsForResource(
Consumer listTagsForResourceRequest) {
return listTagsForResource(ListTagsForResourceRequest.builder().applyMutation(listTagsForResourceRequest).build());
}
/**
*
* Sends an event to a room. Use this within your application’s business logic to send events to clients of a room;
* e.g., to notify clients to change the way the chat UI is rendered.
*
*
* @param sendEventRequest
* @return A Java Future containing the result of the SendEvent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.SendEvent
* @see AWS API
* Documentation
*/
default CompletableFuture sendEvent(SendEventRequest sendEventRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sends an event to a room. Use this within your application’s business logic to send events to clients of a room;
* e.g., to notify clients to change the way the chat UI is rendered.
*
*
*
* This is a convenience which creates an instance of the {@link SendEventRequest.Builder} avoiding the need to
* create one manually via {@link SendEventRequest#builder()}
*
*
* @param sendEventRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.SendEventRequest.Builder} to create a request.
* @return A Java Future containing the result of the SendEvent operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ThrottlingException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.SendEvent
* @see AWS API
* Documentation
*/
default CompletableFuture sendEvent(Consumer sendEventRequest) {
return sendEvent(SendEventRequest.builder().applyMutation(sendEventRequest).build());
}
/**
*
* Adds or updates tags for the AWS resource with the specified ARN.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(TagResourceRequest tagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Adds or updates tags for the AWS resource with the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link TagResourceRequest.Builder} avoiding the need to
* create one manually via {@link TagResourceRequest#builder()}
*
*
* @param tagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.TagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.TagResource
* @see AWS API
* Documentation
*/
default CompletableFuture tagResource(Consumer tagResourceRequest) {
return tagResource(TagResourceRequest.builder().applyMutation(tagResourceRequest).build());
}
/**
*
* Removes tags from the resource with the specified ARN.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(UntagResourceRequest untagResourceRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes tags from the resource with the specified ARN.
*
*
*
* This is a convenience which creates an instance of the {@link UntagResourceRequest.Builder} avoiding the need to
* create one manually via {@link UntagResourceRequest#builder()}
*
*
* @param untagResourceRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.UntagResourceRequest.Builder} to create a request.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ResourceNotFoundException
* - InternalServerException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UntagResource
* @see AWS API
* Documentation
*/
default CompletableFuture untagResource(Consumer untagResourceRequest) {
return untagResource(UntagResourceRequest.builder().applyMutation(untagResourceRequest).build());
}
/**
*
* Updates a specified logging configuration.
*
*
* @param updateLoggingConfigurationRequest
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture updateLoggingConfiguration(
UpdateLoggingConfigurationRequest updateLoggingConfigurationRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a specified logging configuration.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateLoggingConfigurationRequest.Builder} avoiding
* the need to create one manually via {@link UpdateLoggingConfigurationRequest#builder()}
*
*
* @param updateLoggingConfigurationRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.UpdateLoggingConfigurationRequest.Builder} to create
* a request.
* @return A Java Future containing the result of the UpdateLoggingConfiguration operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - ConflictException
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UpdateLoggingConfiguration
* @see AWS API Documentation
*/
default CompletableFuture updateLoggingConfiguration(
Consumer updateLoggingConfigurationRequest) {
return updateLoggingConfiguration(UpdateLoggingConfigurationRequest.builder()
.applyMutation(updateLoggingConfigurationRequest).build());
}
/**
*
* Updates a room’s configuration.
*
*
* @param updateRoomRequest
* @return A Java Future containing the result of the UpdateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UpdateRoom
* @see AWS API
* Documentation
*/
default CompletableFuture updateRoom(UpdateRoomRequest updateRoomRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Updates a room’s configuration.
*
*
*
* This is a convenience which creates an instance of the {@link UpdateRoomRequest.Builder} avoiding the need to
* create one manually via {@link UpdateRoomRequest#builder()}
*
*
* @param updateRoomRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.ivschat.model.UpdateRoomRequest.Builder} to create a request.
* @return A Java Future containing the result of the UpdateRoom operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - AccessDeniedException
* - ResourceNotFoundException
* - PendingVerificationException
* - ValidationException
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - IvschatException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample IvschatAsyncClient.UpdateRoom
* @see AWS API
* Documentation
*/
default CompletableFuture updateRoom(Consumer updateRoomRequest) {
return updateRoom(UpdateRoomRequest.builder().applyMutation(updateRoomRequest).build());
}
@Override
default IvschatServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link IvschatAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static IvschatAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link IvschatAsyncClient}.
*/
static IvschatAsyncClientBuilder builder() {
return new DefaultIvschatAsyncClientBuilder();
}
}