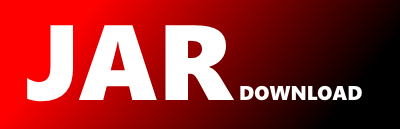
software.amazon.awssdk.services.kafka.model.ClusterOperationV2 Maven / Gradle / Ivy
Show all versions of kafka Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kafka.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
* Returns information about a cluster operation.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ClusterOperationV2 implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField CLUSTER_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterArn").getter(getter(ClusterOperationV2::clusterArn)).setter(setter(Builder::clusterArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterArn").build()).build();
private static final SdkField CLUSTER_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ClusterType").getter(getter(ClusterOperationV2::clusterTypeAsString))
.setter(setter(Builder::clusterType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clusterType").build()).build();
private static final SdkField START_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("StartTime")
.getter(getter(ClusterOperationV2::startTime))
.setter(setter(Builder::startTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("startTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField END_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("EndTime")
.getter(getter(ClusterOperationV2::endTime))
.setter(setter(Builder::endTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("endTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField ERROR_INFO_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("ErrorInfo").getter(getter(ClusterOperationV2::errorInfo)).setter(setter(Builder::errorInfo))
.constructor(ErrorInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("errorInfo").build()).build();
private static final SdkField OPERATION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OperationArn").getter(getter(ClusterOperationV2::operationArn)).setter(setter(Builder::operationArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("operationArn").build()).build();
private static final SdkField OPERATION_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OperationState").getter(getter(ClusterOperationV2::operationState))
.setter(setter(Builder::operationState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("operationState").build()).build();
private static final SdkField OPERATION_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("OperationType").getter(getter(ClusterOperationV2::operationType)).setter(setter(Builder::operationType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("operationType").build()).build();
private static final SdkField PROVISIONED_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Provisioned")
.getter(getter(ClusterOperationV2::provisioned)).setter(setter(Builder::provisioned))
.constructor(ClusterOperationV2Provisioned::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("provisioned").build()).build();
private static final SdkField SERVERLESS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Serverless")
.getter(getter(ClusterOperationV2::serverless)).setter(setter(Builder::serverless))
.constructor(ClusterOperationV2Serverless::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("serverless").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CLUSTER_ARN_FIELD,
CLUSTER_TYPE_FIELD, START_TIME_FIELD, END_TIME_FIELD, ERROR_INFO_FIELD, OPERATION_ARN_FIELD, OPERATION_STATE_FIELD,
OPERATION_TYPE_FIELD, PROVISIONED_FIELD, SERVERLESS_FIELD));
private static final long serialVersionUID = 1L;
private final String clusterArn;
private final String clusterType;
private final Instant startTime;
private final Instant endTime;
private final ErrorInfo errorInfo;
private final String operationArn;
private final String operationState;
private final String operationType;
private final ClusterOperationV2Provisioned provisioned;
private final ClusterOperationV2Serverless serverless;
private ClusterOperationV2(BuilderImpl builder) {
this.clusterArn = builder.clusterArn;
this.clusterType = builder.clusterType;
this.startTime = builder.startTime;
this.endTime = builder.endTime;
this.errorInfo = builder.errorInfo;
this.operationArn = builder.operationArn;
this.operationState = builder.operationState;
this.operationType = builder.operationType;
this.provisioned = builder.provisioned;
this.serverless = builder.serverless;
}
/**
*
*
* ARN of the cluster.
*
*
* @return
* ARN of the cluster.
*
*/
public final String clusterArn() {
return clusterArn;
}
/**
*
*
* Type of the backend cluster.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #clusterType} will
* return {@link ClusterType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #clusterTypeAsString}.
*
*
* @return
* Type of the backend cluster.
*
* @see ClusterType
*/
public final ClusterType clusterType() {
return ClusterType.fromValue(clusterType);
}
/**
*
*
* Type of the backend cluster.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #clusterType} will
* return {@link ClusterType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #clusterTypeAsString}.
*
*
* @return
* Type of the backend cluster.
*
* @see ClusterType
*/
public final String clusterTypeAsString() {
return clusterType;
}
/**
*
*
* The time at which operation was started.
*
*
* @return
* The time at which operation was started.
*
*/
public final Instant startTime() {
return startTime;
}
/**
*
*
* The time at which the operation finished.
*
*
* @return
* The time at which the operation finished.
*
*/
public final Instant endTime() {
return endTime;
}
/**
*
*
* If cluster operation failed from an error, it describes the error.
*
*
* @return
* If cluster operation failed from an error, it describes the error.
*
*/
public final ErrorInfo errorInfo() {
return errorInfo;
}
/**
*
*
* ARN of the cluster operation.
*
*
* @return
* ARN of the cluster operation.
*
*/
public final String operationArn() {
return operationArn;
}
/**
*
*
* State of the cluster operation.
*
*
* @return
* State of the cluster operation.
*
*/
public final String operationState() {
return operationState;
}
/**
*
*
* Type of the cluster operation.
*
*
* @return
* Type of the cluster operation.
*
*/
public final String operationType() {
return operationType;
}
/**
*
*
* Properties of a provisioned cluster.
*
*
* @return
* Properties of a provisioned cluster.
*
*/
public final ClusterOperationV2Provisioned provisioned() {
return provisioned;
}
/**
*
*
* Properties of a serverless cluster.
*
*
* @return
* Properties of a serverless cluster.
*
*/
public final ClusterOperationV2Serverless serverless() {
return serverless;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(clusterArn());
hashCode = 31 * hashCode + Objects.hashCode(clusterTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(startTime());
hashCode = 31 * hashCode + Objects.hashCode(endTime());
hashCode = 31 * hashCode + Objects.hashCode(errorInfo());
hashCode = 31 * hashCode + Objects.hashCode(operationArn());
hashCode = 31 * hashCode + Objects.hashCode(operationState());
hashCode = 31 * hashCode + Objects.hashCode(operationType());
hashCode = 31 * hashCode + Objects.hashCode(provisioned());
hashCode = 31 * hashCode + Objects.hashCode(serverless());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ClusterOperationV2)) {
return false;
}
ClusterOperationV2 other = (ClusterOperationV2) obj;
return Objects.equals(clusterArn(), other.clusterArn())
&& Objects.equals(clusterTypeAsString(), other.clusterTypeAsString())
&& Objects.equals(startTime(), other.startTime()) && Objects.equals(endTime(), other.endTime())
&& Objects.equals(errorInfo(), other.errorInfo()) && Objects.equals(operationArn(), other.operationArn())
&& Objects.equals(operationState(), other.operationState())
&& Objects.equals(operationType(), other.operationType()) && Objects.equals(provisioned(), other.provisioned())
&& Objects.equals(serverless(), other.serverless());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ClusterOperationV2").add("ClusterArn", clusterArn()).add("ClusterType", clusterTypeAsString())
.add("StartTime", startTime()).add("EndTime", endTime()).add("ErrorInfo", errorInfo())
.add("OperationArn", operationArn()).add("OperationState", operationState())
.add("OperationType", operationType()).add("Provisioned", provisioned()).add("Serverless", serverless()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ClusterArn":
return Optional.ofNullable(clazz.cast(clusterArn()));
case "ClusterType":
return Optional.ofNullable(clazz.cast(clusterTypeAsString()));
case "StartTime":
return Optional.ofNullable(clazz.cast(startTime()));
case "EndTime":
return Optional.ofNullable(clazz.cast(endTime()));
case "ErrorInfo":
return Optional.ofNullable(clazz.cast(errorInfo()));
case "OperationArn":
return Optional.ofNullable(clazz.cast(operationArn()));
case "OperationState":
return Optional.ofNullable(clazz.cast(operationState()));
case "OperationType":
return Optional.ofNullable(clazz.cast(operationType()));
case "Provisioned":
return Optional.ofNullable(clazz.cast(provisioned()));
case "Serverless":
return Optional.ofNullable(clazz.cast(serverless()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function