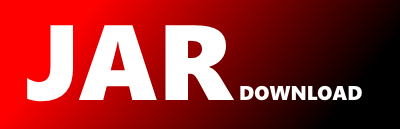
software.amazon.awssdk.services.kafka.model.BrokerNodeGroupInfo Maven / Gradle / Ivy
Show all versions of kafka Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kafka.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
* Describes the setup to be used for Apache Kafka broker nodes in the cluster.
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class BrokerNodeGroupInfo implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField BROKER_AZ_DISTRIBUTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("BrokerAZDistribution").getter(getter(BrokerNodeGroupInfo::brokerAZDistributionAsString))
.setter(setter(Builder::brokerAZDistribution))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("brokerAZDistribution").build())
.build();
private static final SdkField> CLIENT_SUBNETS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ClientSubnets")
.getter(getter(BrokerNodeGroupInfo::clientSubnets))
.setter(setter(Builder::clientSubnets))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clientSubnets").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField INSTANCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InstanceType").getter(getter(BrokerNodeGroupInfo::instanceType)).setter(setter(Builder::instanceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("instanceType").build()).build();
private static final SdkField> SECURITY_GROUPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("SecurityGroups")
.getter(getter(BrokerNodeGroupInfo::securityGroups))
.setter(setter(Builder::securityGroups))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("securityGroups").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField STORAGE_INFO_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("StorageInfo").getter(getter(BrokerNodeGroupInfo::storageInfo)).setter(setter(Builder::storageInfo))
.constructor(StorageInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("storageInfo").build()).build();
private static final SdkField CONNECTIVITY_INFO_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("ConnectivityInfo")
.getter(getter(BrokerNodeGroupInfo::connectivityInfo)).setter(setter(Builder::connectivityInfo))
.constructor(ConnectivityInfo::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("connectivityInfo").build()).build();
private static final SdkField> ZONE_IDS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ZoneIds")
.getter(getter(BrokerNodeGroupInfo::zoneIds))
.setter(setter(Builder::zoneIds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("zoneIds").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(BROKER_AZ_DISTRIBUTION_FIELD,
CLIENT_SUBNETS_FIELD, INSTANCE_TYPE_FIELD, SECURITY_GROUPS_FIELD, STORAGE_INFO_FIELD, CONNECTIVITY_INFO_FIELD,
ZONE_IDS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("brokerAZDistribution", BROKER_AZ_DISTRIBUTION_FIELD);
put("clientSubnets", CLIENT_SUBNETS_FIELD);
put("instanceType", INSTANCE_TYPE_FIELD);
put("securityGroups", SECURITY_GROUPS_FIELD);
put("storageInfo", STORAGE_INFO_FIELD);
put("connectivityInfo", CONNECTIVITY_INFO_FIELD);
put("zoneIds", ZONE_IDS_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String brokerAZDistribution;
private final List clientSubnets;
private final String instanceType;
private final List securityGroups;
private final StorageInfo storageInfo;
private final ConnectivityInfo connectivityInfo;
private final List zoneIds;
private BrokerNodeGroupInfo(BuilderImpl builder) {
this.brokerAZDistribution = builder.brokerAZDistribution;
this.clientSubnets = builder.clientSubnets;
this.instanceType = builder.instanceType;
this.securityGroups = builder.securityGroups;
this.storageInfo = builder.storageInfo;
this.connectivityInfo = builder.connectivityInfo;
this.zoneIds = builder.zoneIds;
}
/**
*
*
* The distribution of broker nodes across Availability Zones. This is an optional parameter. If you don't specify
* it, Amazon MSK gives it the value DEFAULT. You can also explicitly set this parameter to the value DEFAULT. No
* other values are currently allowed.
*
*
* Amazon MSK distributes the broker nodes evenly across the Availability Zones that correspond to the subnets you
* provide when you create the cluster.
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #brokerAZDistribution} will return {@link BrokerAZDistribution#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #brokerAZDistributionAsString}.
*
*
* @return
* The distribution of broker nodes across Availability Zones. This is an optional parameter. If you don't
* specify it, Amazon MSK gives it the value DEFAULT. You can also explicitly set this parameter to the
* value DEFAULT. No other values are currently allowed.
*
*
* Amazon MSK distributes the broker nodes evenly across the Availability Zones that correspond to the
* subnets you provide when you create the cluster.
*
* @see BrokerAZDistribution
*/
public final BrokerAZDistribution brokerAZDistribution() {
return BrokerAZDistribution.fromValue(brokerAZDistribution);
}
/**
*
*
* The distribution of broker nodes across Availability Zones. This is an optional parameter. If you don't specify
* it, Amazon MSK gives it the value DEFAULT. You can also explicitly set this parameter to the value DEFAULT. No
* other values are currently allowed.
*
*
* Amazon MSK distributes the broker nodes evenly across the Availability Zones that correspond to the subnets you
* provide when you create the cluster.
*
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #brokerAZDistribution} will return {@link BrokerAZDistribution#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #brokerAZDistributionAsString}.
*
*
* @return
* The distribution of broker nodes across Availability Zones. This is an optional parameter. If you don't
* specify it, Amazon MSK gives it the value DEFAULT. You can also explicitly set this parameter to the
* value DEFAULT. No other values are currently allowed.
*
*
* Amazon MSK distributes the broker nodes evenly across the Availability Zones that correspond to the
* subnets you provide when you create the cluster.
*
* @see BrokerAZDistribution
*/
public final String brokerAZDistributionAsString() {
return brokerAZDistribution;
}
/**
* For responses, this returns true if the service returned a value for the ClientSubnets property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasClientSubnets() {
return clientSubnets != null && !(clientSubnets instanceof SdkAutoConstructList);
}
/**
*
*
* The list of subnets to connect to in the client virtual private cloud (VPC). AWS creates elastic network
* interfaces inside these subnets. Client applications use elastic network interfaces to produce and consume data.
* Client subnets can't occupy the Availability Zone with ID use use1-az3.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasClientSubnets} method.
*
*
* @return
* The list of subnets to connect to in the client virtual private cloud (VPC). AWS creates elastic network
* interfaces inside these subnets. Client applications use elastic network interfaces to produce and
* consume data. Client subnets can't occupy the Availability Zone with ID use use1-az3.
*
*/
public final List clientSubnets() {
return clientSubnets;
}
/**
*
*
* The type of Amazon EC2 instances to use for Apache Kafka brokers. The following instance types are allowed:
* kafka.m5.large, kafka.m5.xlarge, kafka.m5.2xlarge, kafka.m5.4xlarge, kafka.m5.12xlarge, and kafka.m5.24xlarge.
*
*
* @return
* The type of Amazon EC2 instances to use for Apache Kafka brokers. The following instance types are
* allowed: kafka.m5.large, kafka.m5.xlarge, kafka.m5.2xlarge, kafka.m5.4xlarge, kafka.m5.12xlarge, and
* kafka.m5.24xlarge.
*
*/
public final String instanceType() {
return instanceType;
}
/**
* For responses, this returns true if the service returned a value for the SecurityGroups property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasSecurityGroups() {
return securityGroups != null && !(securityGroups instanceof SdkAutoConstructList);
}
/**
*
*
* The AWS security groups to associate with the elastic network interfaces in order to specify who can connect to
* and communicate with the Amazon MSK cluster. If you don't specify a security group, Amazon MSK uses the default
* security group associated with the VPC.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSecurityGroups} method.
*
*
* @return
* The AWS security groups to associate with the elastic network interfaces in order to specify who can
* connect to and communicate with the Amazon MSK cluster. If you don't specify a security group, Amazon MSK
* uses the default security group associated with the VPC.
*
*/
public final List securityGroups() {
return securityGroups;
}
/**
*
*
* Contains information about storage volumes attached to MSK broker nodes.
*
*
* @return
* Contains information about storage volumes attached to MSK broker nodes.
*
*/
public final StorageInfo storageInfo() {
return storageInfo;
}
/**
*
*
* Information about the broker access configuration.
*
*
* @return
* Information about the broker access configuration.
*
*/
public final ConnectivityInfo connectivityInfo() {
return connectivityInfo;
}
/**
* For responses, this returns true if the service returned a value for the ZoneIds property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasZoneIds() {
return zoneIds != null && !(zoneIds instanceof SdkAutoConstructList);
}
/**
*
*
* The list of zoneIds for the cluster in the virtual private cloud (VPC).
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasZoneIds} method.
*
*
* @return
* The list of zoneIds for the cluster in the virtual private cloud (VPC).
*
*/
public final List zoneIds() {
return zoneIds;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(brokerAZDistributionAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasClientSubnets() ? clientSubnets() : null);
hashCode = 31 * hashCode + Objects.hashCode(instanceType());
hashCode = 31 * hashCode + Objects.hashCode(hasSecurityGroups() ? securityGroups() : null);
hashCode = 31 * hashCode + Objects.hashCode(storageInfo());
hashCode = 31 * hashCode + Objects.hashCode(connectivityInfo());
hashCode = 31 * hashCode + Objects.hashCode(hasZoneIds() ? zoneIds() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof BrokerNodeGroupInfo)) {
return false;
}
BrokerNodeGroupInfo other = (BrokerNodeGroupInfo) obj;
return Objects.equals(brokerAZDistributionAsString(), other.brokerAZDistributionAsString())
&& hasClientSubnets() == other.hasClientSubnets() && Objects.equals(clientSubnets(), other.clientSubnets())
&& Objects.equals(instanceType(), other.instanceType()) && hasSecurityGroups() == other.hasSecurityGroups()
&& Objects.equals(securityGroups(), other.securityGroups()) && Objects.equals(storageInfo(), other.storageInfo())
&& Objects.equals(connectivityInfo(), other.connectivityInfo()) && hasZoneIds() == other.hasZoneIds()
&& Objects.equals(zoneIds(), other.zoneIds());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("BrokerNodeGroupInfo").add("BrokerAZDistribution", brokerAZDistributionAsString())
.add("ClientSubnets", hasClientSubnets() ? clientSubnets() : null).add("InstanceType", instanceType())
.add("SecurityGroups", hasSecurityGroups() ? securityGroups() : null).add("StorageInfo", storageInfo())
.add("ConnectivityInfo", connectivityInfo()).add("ZoneIds", hasZoneIds() ? zoneIds() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "BrokerAZDistribution":
return Optional.ofNullable(clazz.cast(brokerAZDistributionAsString()));
case "ClientSubnets":
return Optional.ofNullable(clazz.cast(clientSubnets()));
case "InstanceType":
return Optional.ofNullable(clazz.cast(instanceType()));
case "SecurityGroups":
return Optional.ofNullable(clazz.cast(securityGroups()));
case "StorageInfo":
return Optional.ofNullable(clazz.cast(storageInfo()));
case "ConnectivityInfo":
return Optional.ofNullable(clazz.cast(connectivityInfo()));
case "ZoneIds":
return Optional.ofNullable(clazz.cast(zoneIds()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function