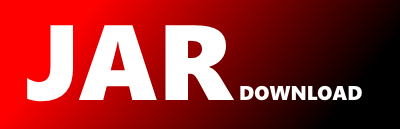
software.amazon.awssdk.services.firehose.model.DestinationDescription Maven / Gradle / Ivy
Show all versions of kinesis Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.firehose.model;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.firehose.transform.DestinationDescriptionMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the destination for a delivery stream.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class DestinationDescription implements StructuredPojo,
ToCopyableBuilder {
private final String destinationId;
private final S3DestinationDescription s3DestinationDescription;
private final ExtendedS3DestinationDescription extendedS3DestinationDescription;
private final RedshiftDestinationDescription redshiftDestinationDescription;
private final ElasticsearchDestinationDescription elasticsearchDestinationDescription;
private final SplunkDestinationDescription splunkDestinationDescription;
private DestinationDescription(BuilderImpl builder) {
this.destinationId = builder.destinationId;
this.s3DestinationDescription = builder.s3DestinationDescription;
this.extendedS3DestinationDescription = builder.extendedS3DestinationDescription;
this.redshiftDestinationDescription = builder.redshiftDestinationDescription;
this.elasticsearchDestinationDescription = builder.elasticsearchDestinationDescription;
this.splunkDestinationDescription = builder.splunkDestinationDescription;
}
/**
*
* The ID of the destination.
*
*
* @return The ID of the destination.
*/
public String destinationId() {
return destinationId;
}
/**
*
* [Deprecated] The destination in Amazon S3.
*
*
* @return [Deprecated] The destination in Amazon S3.
*/
public S3DestinationDescription s3DestinationDescription() {
return s3DestinationDescription;
}
/**
*
* The destination in Amazon S3.
*
*
* @return The destination in Amazon S3.
*/
public ExtendedS3DestinationDescription extendedS3DestinationDescription() {
return extendedS3DestinationDescription;
}
/**
*
* The destination in Amazon Redshift.
*
*
* @return The destination in Amazon Redshift.
*/
public RedshiftDestinationDescription redshiftDestinationDescription() {
return redshiftDestinationDescription;
}
/**
*
* The destination in Amazon ES.
*
*
* @return The destination in Amazon ES.
*/
public ElasticsearchDestinationDescription elasticsearchDestinationDescription() {
return elasticsearchDestinationDescription;
}
/**
*
* The destination in Splunk.
*
*
* @return The destination in Splunk.
*/
public SplunkDestinationDescription splunkDestinationDescription() {
return splunkDestinationDescription;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(destinationId());
hashCode = 31 * hashCode + Objects.hashCode(s3DestinationDescription());
hashCode = 31 * hashCode + Objects.hashCode(extendedS3DestinationDescription());
hashCode = 31 * hashCode + Objects.hashCode(redshiftDestinationDescription());
hashCode = 31 * hashCode + Objects.hashCode(elasticsearchDestinationDescription());
hashCode = 31 * hashCode + Objects.hashCode(splunkDestinationDescription());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof DestinationDescription)) {
return false;
}
DestinationDescription other = (DestinationDescription) obj;
return Objects.equals(destinationId(), other.destinationId())
&& Objects.equals(s3DestinationDescription(), other.s3DestinationDescription())
&& Objects.equals(extendedS3DestinationDescription(), other.extendedS3DestinationDescription())
&& Objects.equals(redshiftDestinationDescription(), other.redshiftDestinationDescription())
&& Objects.equals(elasticsearchDestinationDescription(), other.elasticsearchDestinationDescription())
&& Objects.equals(splunkDestinationDescription(), other.splunkDestinationDescription());
}
@Override
public String toString() {
return ToString.builder("DestinationDescription").add("DestinationId", destinationId())
.add("S3DestinationDescription", s3DestinationDescription())
.add("ExtendedS3DestinationDescription", extendedS3DestinationDescription())
.add("RedshiftDestinationDescription", redshiftDestinationDescription())
.add("ElasticsearchDestinationDescription", elasticsearchDestinationDescription())
.add("SplunkDestinationDescription", splunkDestinationDescription()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DestinationId":
return Optional.of(clazz.cast(destinationId()));
case "S3DestinationDescription":
return Optional.of(clazz.cast(s3DestinationDescription()));
case "ExtendedS3DestinationDescription":
return Optional.of(clazz.cast(extendedS3DestinationDescription()));
case "RedshiftDestinationDescription":
return Optional.of(clazz.cast(redshiftDestinationDescription()));
case "ElasticsearchDestinationDescription":
return Optional.of(clazz.cast(elasticsearchDestinationDescription()));
case "SplunkDestinationDescription":
return Optional.of(clazz.cast(splunkDestinationDescription()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
DestinationDescriptionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* The ID of the destination.
*
*
* @param destinationId
* The ID of the destination.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder destinationId(String destinationId);
/**
*
* [Deprecated] The destination in Amazon S3.
*
*
* @param s3DestinationDescription
* [Deprecated] The destination in Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder s3DestinationDescription(S3DestinationDescription s3DestinationDescription);
/**
*
* [Deprecated] The destination in Amazon S3.
*
* This is a convenience that creates an instance of the {@link S3DestinationDescription.Builder} avoiding the
* need to create one manually via {@link S3DestinationDescription#builder()}.
*
* When the {@link Consumer} completes, {@link S3DestinationDescription.Builder#build()} is called immediately
* and its result is passed to {@link #s3DestinationDescription(S3DestinationDescription)}.
*
* @param s3DestinationDescription
* a consumer that will call methods on {@link S3DestinationDescription.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #s3DestinationDescription(S3DestinationDescription)
*/
default Builder s3DestinationDescription(Consumer s3DestinationDescription) {
return s3DestinationDescription(S3DestinationDescription.builder().apply(s3DestinationDescription).build());
}
/**
*
* The destination in Amazon S3.
*
*
* @param extendedS3DestinationDescription
* The destination in Amazon S3.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder extendedS3DestinationDescription(ExtendedS3DestinationDescription extendedS3DestinationDescription);
/**
*
* The destination in Amazon S3.
*
* This is a convenience that creates an instance of the {@link ExtendedS3DestinationDescription.Builder}
* avoiding the need to create one manually via {@link ExtendedS3DestinationDescription#builder()}.
*
* When the {@link Consumer} completes, {@link ExtendedS3DestinationDescription.Builder#build()} is called
* immediately and its result is passed to
* {@link #extendedS3DestinationDescription(ExtendedS3DestinationDescription)}.
*
* @param extendedS3DestinationDescription
* a consumer that will call methods on {@link ExtendedS3DestinationDescription.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #extendedS3DestinationDescription(ExtendedS3DestinationDescription)
*/
default Builder extendedS3DestinationDescription(
Consumer extendedS3DestinationDescription) {
return extendedS3DestinationDescription(ExtendedS3DestinationDescription.builder()
.apply(extendedS3DestinationDescription).build());
}
/**
*
* The destination in Amazon Redshift.
*
*
* @param redshiftDestinationDescription
* The destination in Amazon Redshift.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder redshiftDestinationDescription(RedshiftDestinationDescription redshiftDestinationDescription);
/**
*
* The destination in Amazon Redshift.
*
* This is a convenience that creates an instance of the {@link RedshiftDestinationDescription.Builder} avoiding
* the need to create one manually via {@link RedshiftDestinationDescription#builder()}.
*
* When the {@link Consumer} completes, {@link RedshiftDestinationDescription.Builder#build()} is called
* immediately and its result is passed to
* {@link #redshiftDestinationDescription(RedshiftDestinationDescription)}.
*
* @param redshiftDestinationDescription
* a consumer that will call methods on {@link RedshiftDestinationDescription.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #redshiftDestinationDescription(RedshiftDestinationDescription)
*/
default Builder redshiftDestinationDescription(
Consumer redshiftDestinationDescription) {
return redshiftDestinationDescription(RedshiftDestinationDescription.builder().apply(redshiftDestinationDescription)
.build());
}
/**
*
* The destination in Amazon ES.
*
*
* @param elasticsearchDestinationDescription
* The destination in Amazon ES.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder elasticsearchDestinationDescription(ElasticsearchDestinationDescription elasticsearchDestinationDescription);
/**
*
* The destination in Amazon ES.
*
* This is a convenience that creates an instance of the {@link ElasticsearchDestinationDescription.Builder}
* avoiding the need to create one manually via {@link ElasticsearchDestinationDescription#builder()}.
*
* When the {@link Consumer} completes, {@link ElasticsearchDestinationDescription.Builder#build()} is called
* immediately and its result is passed to
* {@link #elasticsearchDestinationDescription(ElasticsearchDestinationDescription)}.
*
* @param elasticsearchDestinationDescription
* a consumer that will call methods on {@link ElasticsearchDestinationDescription.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #elasticsearchDestinationDescription(ElasticsearchDestinationDescription)
*/
default Builder elasticsearchDestinationDescription(
Consumer elasticsearchDestinationDescription) {
return elasticsearchDestinationDescription(ElasticsearchDestinationDescription.builder()
.apply(elasticsearchDestinationDescription).build());
}
/**
*
* The destination in Splunk.
*
*
* @param splunkDestinationDescription
* The destination in Splunk.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder splunkDestinationDescription(SplunkDestinationDescription splunkDestinationDescription);
/**
*
* The destination in Splunk.
*
* This is a convenience that creates an instance of the {@link SplunkDestinationDescription.Builder} avoiding
* the need to create one manually via {@link SplunkDestinationDescription#builder()}.
*
* When the {@link Consumer} completes, {@link SplunkDestinationDescription.Builder#build()} is called
* immediately and its result is passed to {@link #splunkDestinationDescription(SplunkDestinationDescription)}.
*
* @param splunkDestinationDescription
* a consumer that will call methods on {@link SplunkDestinationDescription.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #splunkDestinationDescription(SplunkDestinationDescription)
*/
default Builder splunkDestinationDescription(Consumer splunkDestinationDescription) {
return splunkDestinationDescription(SplunkDestinationDescription.builder().apply(splunkDestinationDescription)
.build());
}
}
static final class BuilderImpl implements Builder {
private String destinationId;
private S3DestinationDescription s3DestinationDescription;
private ExtendedS3DestinationDescription extendedS3DestinationDescription;
private RedshiftDestinationDescription redshiftDestinationDescription;
private ElasticsearchDestinationDescription elasticsearchDestinationDescription;
private SplunkDestinationDescription splunkDestinationDescription;
private BuilderImpl() {
}
private BuilderImpl(DestinationDescription model) {
destinationId(model.destinationId);
s3DestinationDescription(model.s3DestinationDescription);
extendedS3DestinationDescription(model.extendedS3DestinationDescription);
redshiftDestinationDescription(model.redshiftDestinationDescription);
elasticsearchDestinationDescription(model.elasticsearchDestinationDescription);
splunkDestinationDescription(model.splunkDestinationDescription);
}
public final String getDestinationId() {
return destinationId;
}
@Override
public final Builder destinationId(String destinationId) {
this.destinationId = destinationId;
return this;
}
public final void setDestinationId(String destinationId) {
this.destinationId = destinationId;
}
public final S3DestinationDescription.Builder getS3DestinationDescription() {
return s3DestinationDescription != null ? s3DestinationDescription.toBuilder() : null;
}
@Override
public final Builder s3DestinationDescription(S3DestinationDescription s3DestinationDescription) {
this.s3DestinationDescription = s3DestinationDescription;
return this;
}
public final void setS3DestinationDescription(S3DestinationDescription.BuilderImpl s3DestinationDescription) {
this.s3DestinationDescription = s3DestinationDescription != null ? s3DestinationDescription.build() : null;
}
public final ExtendedS3DestinationDescription.Builder getExtendedS3DestinationDescription() {
return extendedS3DestinationDescription != null ? extendedS3DestinationDescription.toBuilder() : null;
}
@Override
public final Builder extendedS3DestinationDescription(ExtendedS3DestinationDescription extendedS3DestinationDescription) {
this.extendedS3DestinationDescription = extendedS3DestinationDescription;
return this;
}
public final void setExtendedS3DestinationDescription(
ExtendedS3DestinationDescription.BuilderImpl extendedS3DestinationDescription) {
this.extendedS3DestinationDescription = extendedS3DestinationDescription != null ? extendedS3DestinationDescription
.build() : null;
}
public final RedshiftDestinationDescription.Builder getRedshiftDestinationDescription() {
return redshiftDestinationDescription != null ? redshiftDestinationDescription.toBuilder() : null;
}
@Override
public final Builder redshiftDestinationDescription(RedshiftDestinationDescription redshiftDestinationDescription) {
this.redshiftDestinationDescription = redshiftDestinationDescription;
return this;
}
public final void setRedshiftDestinationDescription(
RedshiftDestinationDescription.BuilderImpl redshiftDestinationDescription) {
this.redshiftDestinationDescription = redshiftDestinationDescription != null ? redshiftDestinationDescription.build()
: null;
}
public final ElasticsearchDestinationDescription.Builder getElasticsearchDestinationDescription() {
return elasticsearchDestinationDescription != null ? elasticsearchDestinationDescription.toBuilder() : null;
}
@Override
public final Builder elasticsearchDestinationDescription(
ElasticsearchDestinationDescription elasticsearchDestinationDescription) {
this.elasticsearchDestinationDescription = elasticsearchDestinationDescription;
return this;
}
public final void setElasticsearchDestinationDescription(
ElasticsearchDestinationDescription.BuilderImpl elasticsearchDestinationDescription) {
this.elasticsearchDestinationDescription = elasticsearchDestinationDescription != null ? elasticsearchDestinationDescription
.build() : null;
}
public final SplunkDestinationDescription.Builder getSplunkDestinationDescription() {
return splunkDestinationDescription != null ? splunkDestinationDescription.toBuilder() : null;
}
@Override
public final Builder splunkDestinationDescription(SplunkDestinationDescription splunkDestinationDescription) {
this.splunkDestinationDescription = splunkDestinationDescription;
return this;
}
public final void setSplunkDestinationDescription(SplunkDestinationDescription.BuilderImpl splunkDestinationDescription) {
this.splunkDestinationDescription = splunkDestinationDescription != null ? splunkDestinationDescription.build()
: null;
}
@Override
public DestinationDescription build() {
return new DestinationDescription(this);
}
}
}