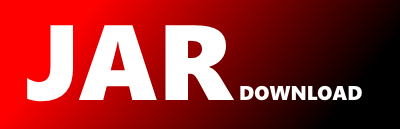
software.amazon.awssdk.services.kinesisanalytics.model.SourceSchema Maven / Gradle / Ivy
Show all versions of kinesis Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kinesisanalytics.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import javax.annotation.Generated;
import software.amazon.awssdk.annotations.SdkInternalApi;
import software.amazon.awssdk.core.protocol.ProtocolMarshaller;
import software.amazon.awssdk.core.protocol.StructuredPojo;
import software.amazon.awssdk.services.kinesisanalytics.transform.SourceSchemaMarshaller;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes the format of the data in the streaming source, and how each data element maps to corresponding columns
* created in the in-application stream.
*
*/
@Generated("software.amazon.awssdk:codegen")
public class SourceSchema implements StructuredPojo, ToCopyableBuilder {
private final RecordFormat recordFormat;
private final String recordEncoding;
private final List recordColumns;
private SourceSchema(BuilderImpl builder) {
this.recordFormat = builder.recordFormat;
this.recordEncoding = builder.recordEncoding;
this.recordColumns = builder.recordColumns;
}
/**
*
* Specifies the format of the records on the streaming source.
*
*
* @return Specifies the format of the records on the streaming source.
*/
public RecordFormat recordFormat() {
return recordFormat;
}
/**
*
* Specifies the encoding of the records in the streaming source. For example, UTF-8.
*
*
* @return Specifies the encoding of the records in the streaming source. For example, UTF-8.
*/
public String recordEncoding() {
return recordEncoding;
}
/**
*
* A list of RecordColumn
objects.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return A list of RecordColumn
objects.
*/
public List recordColumns() {
return recordColumns;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(recordFormat());
hashCode = 31 * hashCode + Objects.hashCode(recordEncoding());
hashCode = 31 * hashCode + Objects.hashCode(recordColumns());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof SourceSchema)) {
return false;
}
SourceSchema other = (SourceSchema) obj;
return Objects.equals(recordFormat(), other.recordFormat()) && Objects.equals(recordEncoding(), other.recordEncoding())
&& Objects.equals(recordColumns(), other.recordColumns());
}
@Override
public String toString() {
return ToString.builder("SourceSchema").add("RecordFormat", recordFormat()).add("RecordEncoding", recordEncoding())
.add("RecordColumns", recordColumns()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "RecordFormat":
return Optional.of(clazz.cast(recordFormat()));
case "RecordEncoding":
return Optional.of(clazz.cast(recordEncoding()));
case "RecordColumns":
return Optional.of(clazz.cast(recordColumns()));
default:
return Optional.empty();
}
}
@SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
SourceSchemaMarshaller.getInstance().marshall(this, protocolMarshaller);
}
public interface Builder extends CopyableBuilder {
/**
*
* Specifies the format of the records on the streaming source.
*
*
* @param recordFormat
* Specifies the format of the records on the streaming source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder recordFormat(RecordFormat recordFormat);
/**
*
* Specifies the format of the records on the streaming source.
*
* This is a convenience that creates an instance of the {@link RecordFormat.Builder} avoiding the need to
* create one manually via {@link RecordFormat#builder()}.
*
* When the {@link Consumer} completes, {@link RecordFormat.Builder#build()} is called immediately and its
* result is passed to {@link #recordFormat(RecordFormat)}.
*
* @param recordFormat
* a consumer that will call methods on {@link RecordFormat.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #recordFormat(RecordFormat)
*/
default Builder recordFormat(Consumer recordFormat) {
return recordFormat(RecordFormat.builder().apply(recordFormat).build());
}
/**
*
* Specifies the encoding of the records in the streaming source. For example, UTF-8.
*
*
* @param recordEncoding
* Specifies the encoding of the records in the streaming source. For example, UTF-8.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder recordEncoding(String recordEncoding);
/**
*
* A list of RecordColumn
objects.
*
*
* @param recordColumns
* A list of RecordColumn
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder recordColumns(Collection recordColumns);
/**
*
* A list of RecordColumn
objects.
*
*
* @param recordColumns
* A list of RecordColumn
objects.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder recordColumns(RecordColumn... recordColumns);
}
static final class BuilderImpl implements Builder {
private RecordFormat recordFormat;
private String recordEncoding;
private List recordColumns;
private BuilderImpl() {
}
private BuilderImpl(SourceSchema model) {
recordFormat(model.recordFormat);
recordEncoding(model.recordEncoding);
recordColumns(model.recordColumns);
}
public final RecordFormat.Builder getRecordFormat() {
return recordFormat != null ? recordFormat.toBuilder() : null;
}
@Override
public final Builder recordFormat(RecordFormat recordFormat) {
this.recordFormat = recordFormat;
return this;
}
public final void setRecordFormat(RecordFormat.BuilderImpl recordFormat) {
this.recordFormat = recordFormat != null ? recordFormat.build() : null;
}
public final String getRecordEncoding() {
return recordEncoding;
}
@Override
public final Builder recordEncoding(String recordEncoding) {
this.recordEncoding = recordEncoding;
return this;
}
public final void setRecordEncoding(String recordEncoding) {
this.recordEncoding = recordEncoding;
}
public final Collection getRecordColumns() {
return recordColumns != null ? recordColumns.stream().map(RecordColumn::toBuilder).collect(Collectors.toList())
: null;
}
@Override
public final Builder recordColumns(Collection recordColumns) {
this.recordColumns = RecordColumnsCopier.copy(recordColumns);
return this;
}
@Override
@SafeVarargs
public final Builder recordColumns(RecordColumn... recordColumns) {
recordColumns(Arrays.asList(recordColumns));
return this;
}
public final void setRecordColumns(Collection recordColumns) {
this.recordColumns = RecordColumnsCopier.copyFromBuilder(recordColumns);
}
@Override
public SourceSchema build() {
return new SourceSchema(this);
}
}
}