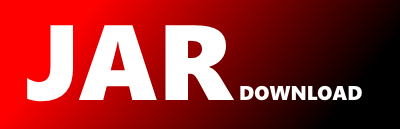
software.amazon.awssdk.services.kinesisanalytics.model.InputUpdate Maven / Gradle / Ivy
Show all versions of kinesisanalytics Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kinesisanalytics.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Describes updates to a specific input configuration (identified by the InputId
of an application).
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class InputUpdate implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField INPUT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("InputId").getter(getter(InputUpdate::inputId)).setter(setter(Builder::inputId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputId").build()).build();
private static final SdkField NAME_PREFIX_UPDATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("NamePrefixUpdate").getter(getter(InputUpdate::namePrefixUpdate))
.setter(setter(Builder::namePrefixUpdate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("NamePrefixUpdate").build()).build();
private static final SdkField INPUT_PROCESSING_CONFIGURATION_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("InputProcessingConfigurationUpdate")
.getter(getter(InputUpdate::inputProcessingConfigurationUpdate))
.setter(setter(Builder::inputProcessingConfigurationUpdate))
.constructor(InputProcessingConfigurationUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputProcessingConfigurationUpdate")
.build()).build();
private static final SdkField KINESIS_STREAMS_INPUT_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("KinesisStreamsInputUpdate")
.getter(getter(InputUpdate::kinesisStreamsInputUpdate)).setter(setter(Builder::kinesisStreamsInputUpdate))
.constructor(KinesisStreamsInputUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KinesisStreamsInputUpdate").build())
.build();
private static final SdkField KINESIS_FIREHOSE_INPUT_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.memberName("KinesisFirehoseInputUpdate")
.getter(getter(InputUpdate::kinesisFirehoseInputUpdate))
.setter(setter(Builder::kinesisFirehoseInputUpdate))
.constructor(KinesisFirehoseInputUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KinesisFirehoseInputUpdate").build())
.build();
private static final SdkField INPUT_SCHEMA_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputSchemaUpdate")
.getter(getter(InputUpdate::inputSchemaUpdate)).setter(setter(Builder::inputSchemaUpdate))
.constructor(InputSchemaUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputSchemaUpdate").build()).build();
private static final SdkField INPUT_PARALLELISM_UPDATE_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("InputParallelismUpdate")
.getter(getter(InputUpdate::inputParallelismUpdate)).setter(setter(Builder::inputParallelismUpdate))
.constructor(InputParallelismUpdate::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputParallelismUpdate").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(INPUT_ID_FIELD,
NAME_PREFIX_UPDATE_FIELD, INPUT_PROCESSING_CONFIGURATION_UPDATE_FIELD, KINESIS_STREAMS_INPUT_UPDATE_FIELD,
KINESIS_FIREHOSE_INPUT_UPDATE_FIELD, INPUT_SCHEMA_UPDATE_FIELD, INPUT_PARALLELISM_UPDATE_FIELD));
private static final long serialVersionUID = 1L;
private final String inputId;
private final String namePrefixUpdate;
private final InputProcessingConfigurationUpdate inputProcessingConfigurationUpdate;
private final KinesisStreamsInputUpdate kinesisStreamsInputUpdate;
private final KinesisFirehoseInputUpdate kinesisFirehoseInputUpdate;
private final InputSchemaUpdate inputSchemaUpdate;
private final InputParallelismUpdate inputParallelismUpdate;
private InputUpdate(BuilderImpl builder) {
this.inputId = builder.inputId;
this.namePrefixUpdate = builder.namePrefixUpdate;
this.inputProcessingConfigurationUpdate = builder.inputProcessingConfigurationUpdate;
this.kinesisStreamsInputUpdate = builder.kinesisStreamsInputUpdate;
this.kinesisFirehoseInputUpdate = builder.kinesisFirehoseInputUpdate;
this.inputSchemaUpdate = builder.inputSchemaUpdate;
this.inputParallelismUpdate = builder.inputParallelismUpdate;
}
/**
*
* Input ID of the application input to be updated.
*
*
* @return Input ID of the application input to be updated.
*/
public final String inputId() {
return inputId;
}
/**
*
* Name prefix for in-application streams that Amazon Kinesis Analytics creates for the specific streaming source.
*
*
* @return Name prefix for in-application streams that Amazon Kinesis Analytics creates for the specific streaming
* source.
*/
public final String namePrefixUpdate() {
return namePrefixUpdate;
}
/**
*
* Describes updates for an input processing configuration.
*
*
* @return Describes updates for an input processing configuration.
*/
public final InputProcessingConfigurationUpdate inputProcessingConfigurationUpdate() {
return inputProcessingConfigurationUpdate;
}
/**
*
* If an Amazon Kinesis stream is the streaming source to be updated, provides an updated stream Amazon Resource
* Name (ARN) and IAM role ARN.
*
*
* @return If an Amazon Kinesis stream is the streaming source to be updated, provides an updated stream Amazon
* Resource Name (ARN) and IAM role ARN.
*/
public final KinesisStreamsInputUpdate kinesisStreamsInputUpdate() {
return kinesisStreamsInputUpdate;
}
/**
*
* If an Amazon Kinesis Firehose delivery stream is the streaming source to be updated, provides an updated stream
* ARN and IAM role ARN.
*
*
* @return If an Amazon Kinesis Firehose delivery stream is the streaming source to be updated, provides an updated
* stream ARN and IAM role ARN.
*/
public final KinesisFirehoseInputUpdate kinesisFirehoseInputUpdate() {
return kinesisFirehoseInputUpdate;
}
/**
*
* Describes the data format on the streaming source, and how record elements on the streaming source map to columns
* of the in-application stream that is created.
*
*
* @return Describes the data format on the streaming source, and how record elements on the streaming source map to
* columns of the in-application stream that is created.
*/
public final InputSchemaUpdate inputSchemaUpdate() {
return inputSchemaUpdate;
}
/**
*
* Describes the parallelism updates (the number in-application streams Amazon Kinesis Analytics creates for the
* specific streaming source).
*
*
* @return Describes the parallelism updates (the number in-application streams Amazon Kinesis Analytics creates for
* the specific streaming source).
*/
public final InputParallelismUpdate inputParallelismUpdate() {
return inputParallelismUpdate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(inputId());
hashCode = 31 * hashCode + Objects.hashCode(namePrefixUpdate());
hashCode = 31 * hashCode + Objects.hashCode(inputProcessingConfigurationUpdate());
hashCode = 31 * hashCode + Objects.hashCode(kinesisStreamsInputUpdate());
hashCode = 31 * hashCode + Objects.hashCode(kinesisFirehoseInputUpdate());
hashCode = 31 * hashCode + Objects.hashCode(inputSchemaUpdate());
hashCode = 31 * hashCode + Objects.hashCode(inputParallelismUpdate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof InputUpdate)) {
return false;
}
InputUpdate other = (InputUpdate) obj;
return Objects.equals(inputId(), other.inputId()) && Objects.equals(namePrefixUpdate(), other.namePrefixUpdate())
&& Objects.equals(inputProcessingConfigurationUpdate(), other.inputProcessingConfigurationUpdate())
&& Objects.equals(kinesisStreamsInputUpdate(), other.kinesisStreamsInputUpdate())
&& Objects.equals(kinesisFirehoseInputUpdate(), other.kinesisFirehoseInputUpdate())
&& Objects.equals(inputSchemaUpdate(), other.inputSchemaUpdate())
&& Objects.equals(inputParallelismUpdate(), other.inputParallelismUpdate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("InputUpdate").add("InputId", inputId()).add("NamePrefixUpdate", namePrefixUpdate())
.add("InputProcessingConfigurationUpdate", inputProcessingConfigurationUpdate())
.add("KinesisStreamsInputUpdate", kinesisStreamsInputUpdate())
.add("KinesisFirehoseInputUpdate", kinesisFirehoseInputUpdate()).add("InputSchemaUpdate", inputSchemaUpdate())
.add("InputParallelismUpdate", inputParallelismUpdate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "InputId":
return Optional.ofNullable(clazz.cast(inputId()));
case "NamePrefixUpdate":
return Optional.ofNullable(clazz.cast(namePrefixUpdate()));
case "InputProcessingConfigurationUpdate":
return Optional.ofNullable(clazz.cast(inputProcessingConfigurationUpdate()));
case "KinesisStreamsInputUpdate":
return Optional.ofNullable(clazz.cast(kinesisStreamsInputUpdate()));
case "KinesisFirehoseInputUpdate":
return Optional.ofNullable(clazz.cast(kinesisFirehoseInputUpdate()));
case "InputSchemaUpdate":
return Optional.ofNullable(clazz.cast(inputSchemaUpdate()));
case "InputParallelismUpdate":
return Optional.ofNullable(clazz.cast(inputParallelismUpdate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function