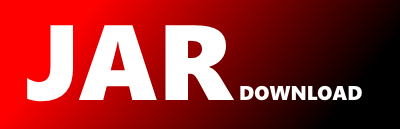
software.amazon.awssdk.services.kinesisanalytics.model.CreateApplicationRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kinesisanalytics.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* TBD
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateApplicationRequest extends KinesisAnalyticsRequest implements
ToCopyableBuilder {
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(CreateApplicationRequest::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField APPLICATION_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationDescription").getter(getter(CreateApplicationRequest::applicationDescription))
.setter(setter(Builder::applicationDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationDescription").build())
.build();
private static final SdkField> INPUTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Inputs")
.getter(getter(CreateApplicationRequest::inputs))
.setter(setter(Builder::inputs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Inputs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Input::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OUTPUTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Outputs")
.getter(getter(CreateApplicationRequest::outputs))
.setter(setter(Builder::outputs))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Outputs").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy