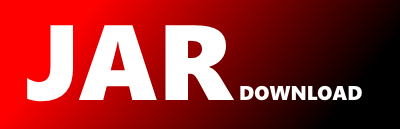
software.amazon.awssdk.services.kinesisanalytics.model.ApplicationDetail Maven / Gradle / Ivy
Show all versions of kinesisanalytics Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kinesisanalytics.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
*
* This documentation is for version 1 of the Amazon Kinesis Data Analytics API, which only supports SQL applications.
* Version 2 of the API supports SQL and Java applications. For more information about version 2, see Amazon Kinesis Data Analytics API V2 Documentation.
*
*
*
* Provides a description of the application, including the application Amazon Resource Name (ARN), status, latest
* version, and input and output configuration.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ApplicationDetail implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField APPLICATION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationName").getter(getter(ApplicationDetail::applicationName))
.setter(setter(Builder::applicationName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationName").build()).build();
private static final SdkField APPLICATION_DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationDescription").getter(getter(ApplicationDetail::applicationDescription))
.setter(setter(Builder::applicationDescription))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationDescription").build())
.build();
private static final SdkField APPLICATION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationARN").getter(getter(ApplicationDetail::applicationARN))
.setter(setter(Builder::applicationARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationARN").build()).build();
private static final SdkField APPLICATION_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationStatus").getter(getter(ApplicationDetail::applicationStatusAsString))
.setter(setter(Builder::applicationStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationStatus").build()).build();
private static final SdkField CREATE_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreateTimestamp").getter(getter(ApplicationDetail::createTimestamp))
.setter(setter(Builder::createTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreateTimestamp").build()).build();
private static final SdkField LAST_UPDATE_TIMESTAMP_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("LastUpdateTimestamp").getter(getter(ApplicationDetail::lastUpdateTimestamp))
.setter(setter(Builder::lastUpdateTimestamp))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LastUpdateTimestamp").build())
.build();
private static final SdkField> INPUT_DESCRIPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("InputDescriptions")
.getter(getter(ApplicationDetail::inputDescriptions))
.setter(setter(Builder::inputDescriptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("InputDescriptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(InputDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> OUTPUT_DESCRIPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("OutputDescriptions")
.getter(getter(ApplicationDetail::outputDescriptions))
.setter(setter(Builder::outputDescriptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("OutputDescriptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(OutputDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> REFERENCE_DATA_SOURCE_DESCRIPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("ReferenceDataSourceDescriptions")
.getter(getter(ApplicationDetail::referenceDataSourceDescriptions))
.setter(setter(Builder::referenceDataSourceDescriptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ReferenceDataSourceDescriptions")
.build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(ReferenceDataSourceDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> CLOUD_WATCH_LOGGING_OPTION_DESCRIPTIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("CloudWatchLoggingOptionDescriptions")
.getter(getter(ApplicationDetail::cloudWatchLoggingOptionDescriptions))
.setter(setter(Builder::cloudWatchLoggingOptionDescriptions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("CloudWatchLoggingOptionDescriptions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(CloudWatchLoggingOptionDescription::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField APPLICATION_CODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationCode").getter(getter(ApplicationDetail::applicationCode))
.setter(setter(Builder::applicationCode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationCode").build()).build();
private static final SdkField APPLICATION_VERSION_ID_FIELD = SdkField. builder(MarshallingType.LONG)
.memberName("ApplicationVersionId").getter(getter(ApplicationDetail::applicationVersionId))
.setter(setter(Builder::applicationVersionId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationVersionId").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(APPLICATION_NAME_FIELD,
APPLICATION_DESCRIPTION_FIELD, APPLICATION_ARN_FIELD, APPLICATION_STATUS_FIELD, CREATE_TIMESTAMP_FIELD,
LAST_UPDATE_TIMESTAMP_FIELD, INPUT_DESCRIPTIONS_FIELD, OUTPUT_DESCRIPTIONS_FIELD,
REFERENCE_DATA_SOURCE_DESCRIPTIONS_FIELD, CLOUD_WATCH_LOGGING_OPTION_DESCRIPTIONS_FIELD, APPLICATION_CODE_FIELD,
APPLICATION_VERSION_ID_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("ApplicationName", APPLICATION_NAME_FIELD);
put("ApplicationDescription", APPLICATION_DESCRIPTION_FIELD);
put("ApplicationARN", APPLICATION_ARN_FIELD);
put("ApplicationStatus", APPLICATION_STATUS_FIELD);
put("CreateTimestamp", CREATE_TIMESTAMP_FIELD);
put("LastUpdateTimestamp", LAST_UPDATE_TIMESTAMP_FIELD);
put("InputDescriptions", INPUT_DESCRIPTIONS_FIELD);
put("OutputDescriptions", OUTPUT_DESCRIPTIONS_FIELD);
put("ReferenceDataSourceDescriptions", REFERENCE_DATA_SOURCE_DESCRIPTIONS_FIELD);
put("CloudWatchLoggingOptionDescriptions", CLOUD_WATCH_LOGGING_OPTION_DESCRIPTIONS_FIELD);
put("ApplicationCode", APPLICATION_CODE_FIELD);
put("ApplicationVersionId", APPLICATION_VERSION_ID_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String applicationName;
private final String applicationDescription;
private final String applicationARN;
private final String applicationStatus;
private final Instant createTimestamp;
private final Instant lastUpdateTimestamp;
private final List inputDescriptions;
private final List outputDescriptions;
private final List referenceDataSourceDescriptions;
private final List cloudWatchLoggingOptionDescriptions;
private final String applicationCode;
private final Long applicationVersionId;
private ApplicationDetail(BuilderImpl builder) {
this.applicationName = builder.applicationName;
this.applicationDescription = builder.applicationDescription;
this.applicationARN = builder.applicationARN;
this.applicationStatus = builder.applicationStatus;
this.createTimestamp = builder.createTimestamp;
this.lastUpdateTimestamp = builder.lastUpdateTimestamp;
this.inputDescriptions = builder.inputDescriptions;
this.outputDescriptions = builder.outputDescriptions;
this.referenceDataSourceDescriptions = builder.referenceDataSourceDescriptions;
this.cloudWatchLoggingOptionDescriptions = builder.cloudWatchLoggingOptionDescriptions;
this.applicationCode = builder.applicationCode;
this.applicationVersionId = builder.applicationVersionId;
}
/**
*
* Name of the application.
*
*
* @return Name of the application.
*/
public final String applicationName() {
return applicationName;
}
/**
*
* Description of the application.
*
*
* @return Description of the application.
*/
public final String applicationDescription() {
return applicationDescription;
}
/**
*
* ARN of the application.
*
*
* @return ARN of the application.
*/
public final String applicationARN() {
return applicationARN;
}
/**
*
* Status of the application.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #applicationStatus}
* will return {@link ApplicationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #applicationStatusAsString}.
*
*
* @return Status of the application.
* @see ApplicationStatus
*/
public final ApplicationStatus applicationStatus() {
return ApplicationStatus.fromValue(applicationStatus);
}
/**
*
* Status of the application.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #applicationStatus}
* will return {@link ApplicationStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #applicationStatusAsString}.
*
*
* @return Status of the application.
* @see ApplicationStatus
*/
public final String applicationStatusAsString() {
return applicationStatus;
}
/**
*
* Time stamp when the application version was created.
*
*
* @return Time stamp when the application version was created.
*/
public final Instant createTimestamp() {
return createTimestamp;
}
/**
*
* Time stamp when the application was last updated.
*
*
* @return Time stamp when the application was last updated.
*/
public final Instant lastUpdateTimestamp() {
return lastUpdateTimestamp;
}
/**
* For responses, this returns true if the service returned a value for the InputDescriptions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasInputDescriptions() {
return inputDescriptions != null && !(inputDescriptions instanceof SdkAutoConstructList);
}
/**
*
* Describes the application input configuration. For more information, see Configuring Application
* Input.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasInputDescriptions} method.
*
*
* @return Describes the application input configuration. For more information, see Configuring
* Application Input.
*/
public final List inputDescriptions() {
return inputDescriptions;
}
/**
* For responses, this returns true if the service returned a value for the OutputDescriptions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasOutputDescriptions() {
return outputDescriptions != null && !(outputDescriptions instanceof SdkAutoConstructList);
}
/**
*
* Describes the application output configuration. For more information, see Configuring Application
* Output.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOutputDescriptions} method.
*
*
* @return Describes the application output configuration. For more information, see Configuring
* Application Output.
*/
public final List outputDescriptions() {
return outputDescriptions;
}
/**
* For responses, this returns true if the service returned a value for the ReferenceDataSourceDescriptions
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasReferenceDataSourceDescriptions() {
return referenceDataSourceDescriptions != null && !(referenceDataSourceDescriptions instanceof SdkAutoConstructList);
}
/**
*
* Describes reference data sources configured for the application. For more information, see Configuring Application
* Input.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasReferenceDataSourceDescriptions}
* method.
*
*
* @return Describes reference data sources configured for the application. For more information, see Configuring
* Application Input.
*/
public final List referenceDataSourceDescriptions() {
return referenceDataSourceDescriptions;
}
/**
* For responses, this returns true if the service returned a value for the CloudWatchLoggingOptionDescriptions
* property. This DOES NOT check that the value is non-empty (for which, you should check the {@code isEmpty()}
* method on the property). This is useful because the SDK will never return a null collection or map, but you may
* need to differentiate between the service returning nothing (or null) and the service returning an empty
* collection or map. For requests, this returns true if a value for the property was specified in the request
* builder, and false if a value was not specified.
*/
public final boolean hasCloudWatchLoggingOptionDescriptions() {
return cloudWatchLoggingOptionDescriptions != null
&& !(cloudWatchLoggingOptionDescriptions instanceof SdkAutoConstructList);
}
/**
*
* Describes the CloudWatch log streams that are configured to receive application messages. For more information
* about using CloudWatch log streams with Amazon Kinesis Analytics applications, see Working with Amazon
* CloudWatch Logs.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasCloudWatchLoggingOptionDescriptions}
* method.
*
*
* @return Describes the CloudWatch log streams that are configured to receive application messages. For more
* information about using CloudWatch log streams with Amazon Kinesis Analytics applications, see Working with Amazon
* CloudWatch Logs.
*/
public final List cloudWatchLoggingOptionDescriptions() {
return cloudWatchLoggingOptionDescriptions;
}
/**
*
* Returns the application code that you provided to perform data analysis on any of the in-application streams in
* your application.
*
*
* @return Returns the application code that you provided to perform data analysis on any of the in-application
* streams in your application.
*/
public final String applicationCode() {
return applicationCode;
}
/**
*
* Provides the current application version.
*
*
* @return Provides the current application version.
*/
public final Long applicationVersionId() {
return applicationVersionId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(applicationName());
hashCode = 31 * hashCode + Objects.hashCode(applicationDescription());
hashCode = 31 * hashCode + Objects.hashCode(applicationARN());
hashCode = 31 * hashCode + Objects.hashCode(applicationStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(createTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdateTimestamp());
hashCode = 31 * hashCode + Objects.hashCode(hasInputDescriptions() ? inputDescriptions() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasOutputDescriptions() ? outputDescriptions() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasReferenceDataSourceDescriptions() ? referenceDataSourceDescriptions() : null);
hashCode = 31 * hashCode
+ Objects.hashCode(hasCloudWatchLoggingOptionDescriptions() ? cloudWatchLoggingOptionDescriptions() : null);
hashCode = 31 * hashCode + Objects.hashCode(applicationCode());
hashCode = 31 * hashCode + Objects.hashCode(applicationVersionId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ApplicationDetail)) {
return false;
}
ApplicationDetail other = (ApplicationDetail) obj;
return Objects.equals(applicationName(), other.applicationName())
&& Objects.equals(applicationDescription(), other.applicationDescription())
&& Objects.equals(applicationARN(), other.applicationARN())
&& Objects.equals(applicationStatusAsString(), other.applicationStatusAsString())
&& Objects.equals(createTimestamp(), other.createTimestamp())
&& Objects.equals(lastUpdateTimestamp(), other.lastUpdateTimestamp())
&& hasInputDescriptions() == other.hasInputDescriptions()
&& Objects.equals(inputDescriptions(), other.inputDescriptions())
&& hasOutputDescriptions() == other.hasOutputDescriptions()
&& Objects.equals(outputDescriptions(), other.outputDescriptions())
&& hasReferenceDataSourceDescriptions() == other.hasReferenceDataSourceDescriptions()
&& Objects.equals(referenceDataSourceDescriptions(), other.referenceDataSourceDescriptions())
&& hasCloudWatchLoggingOptionDescriptions() == other.hasCloudWatchLoggingOptionDescriptions()
&& Objects.equals(cloudWatchLoggingOptionDescriptions(), other.cloudWatchLoggingOptionDescriptions())
&& Objects.equals(applicationCode(), other.applicationCode())
&& Objects.equals(applicationVersionId(), other.applicationVersionId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString
.builder("ApplicationDetail")
.add("ApplicationName", applicationName())
.add("ApplicationDescription", applicationDescription())
.add("ApplicationARN", applicationARN())
.add("ApplicationStatus", applicationStatusAsString())
.add("CreateTimestamp", createTimestamp())
.add("LastUpdateTimestamp", lastUpdateTimestamp())
.add("InputDescriptions", hasInputDescriptions() ? inputDescriptions() : null)
.add("OutputDescriptions", hasOutputDescriptions() ? outputDescriptions() : null)
.add("ReferenceDataSourceDescriptions",
hasReferenceDataSourceDescriptions() ? referenceDataSourceDescriptions() : null)
.add("CloudWatchLoggingOptionDescriptions",
hasCloudWatchLoggingOptionDescriptions() ? cloudWatchLoggingOptionDescriptions() : null)
.add("ApplicationCode", applicationCode()).add("ApplicationVersionId", applicationVersionId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ApplicationName":
return Optional.ofNullable(clazz.cast(applicationName()));
case "ApplicationDescription":
return Optional.ofNullable(clazz.cast(applicationDescription()));
case "ApplicationARN":
return Optional.ofNullable(clazz.cast(applicationARN()));
case "ApplicationStatus":
return Optional.ofNullable(clazz.cast(applicationStatusAsString()));
case "CreateTimestamp":
return Optional.ofNullable(clazz.cast(createTimestamp()));
case "LastUpdateTimestamp":
return Optional.ofNullable(clazz.cast(lastUpdateTimestamp()));
case "InputDescriptions":
return Optional.ofNullable(clazz.cast(inputDescriptions()));
case "OutputDescriptions":
return Optional.ofNullable(clazz.cast(outputDescriptions()));
case "ReferenceDataSourceDescriptions":
return Optional.ofNullable(clazz.cast(referenceDataSourceDescriptions()));
case "CloudWatchLoggingOptionDescriptions":
return Optional.ofNullable(clazz.cast(cloudWatchLoggingOptionDescriptions()));
case "ApplicationCode":
return Optional.ofNullable(clazz.cast(applicationCode()));
case "ApplicationVersionId":
return Optional.ofNullable(clazz.cast(applicationVersionId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function