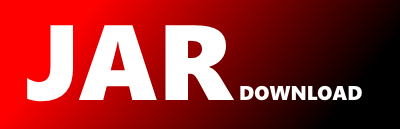
software.amazon.awssdk.services.kinesisvideo.model.ChannelInfo Maven / Gradle / Ivy
Show all versions of kinesisvideo Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kinesisvideo.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A structure that encapsulates a signaling channel's metadata and properties.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class ChannelInfo implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField CHANNEL_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChannelName").getter(getter(ChannelInfo::channelName)).setter(setter(Builder::channelName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChannelName").build()).build();
private static final SdkField CHANNEL_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChannelARN").getter(getter(ChannelInfo::channelARN)).setter(setter(Builder::channelARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChannelARN").build()).build();
private static final SdkField CHANNEL_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChannelType").getter(getter(ChannelInfo::channelTypeAsString)).setter(setter(Builder::channelType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChannelType").build()).build();
private static final SdkField CHANNEL_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ChannelStatus").getter(getter(ChannelInfo::channelStatusAsString))
.setter(setter(Builder::channelStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ChannelStatus").build()).build();
private static final SdkField CREATION_TIME_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("CreationTime").getter(getter(ChannelInfo::creationTime)).setter(setter(Builder::creationTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationTime").build()).build();
private static final SdkField SINGLE_MASTER_CONFIGURATION_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("SingleMasterConfiguration")
.getter(getter(ChannelInfo::singleMasterConfiguration)).setter(setter(Builder::singleMasterConfiguration))
.constructor(SingleMasterConfiguration::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SingleMasterConfiguration").build())
.build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Version")
.getter(getter(ChannelInfo::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Version").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CHANNEL_NAME_FIELD,
CHANNEL_ARN_FIELD, CHANNEL_TYPE_FIELD, CHANNEL_STATUS_FIELD, CREATION_TIME_FIELD, SINGLE_MASTER_CONFIGURATION_FIELD,
VERSION_FIELD));
private static final long serialVersionUID = 1L;
private final String channelName;
private final String channelARN;
private final String channelType;
private final String channelStatus;
private final Instant creationTime;
private final SingleMasterConfiguration singleMasterConfiguration;
private final String version;
private ChannelInfo(BuilderImpl builder) {
this.channelName = builder.channelName;
this.channelARN = builder.channelARN;
this.channelType = builder.channelType;
this.channelStatus = builder.channelStatus;
this.creationTime = builder.creationTime;
this.singleMasterConfiguration = builder.singleMasterConfiguration;
this.version = builder.version;
}
/**
*
* The name of the signaling channel.
*
*
* @return The name of the signaling channel.
*/
public final String channelName() {
return channelName;
}
/**
*
* The Amazon Resource Name (ARN) of the signaling channel.
*
*
* @return The Amazon Resource Name (ARN) of the signaling channel.
*/
public final String channelARN() {
return channelARN;
}
/**
*
* The type of the signaling channel.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #channelType} will
* return {@link ChannelType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #channelTypeAsString}.
*
*
* @return The type of the signaling channel.
* @see ChannelType
*/
public final ChannelType channelType() {
return ChannelType.fromValue(channelType);
}
/**
*
* The type of the signaling channel.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #channelType} will
* return {@link ChannelType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #channelTypeAsString}.
*
*
* @return The type of the signaling channel.
* @see ChannelType
*/
public final String channelTypeAsString() {
return channelType;
}
/**
*
* Current status of the signaling channel.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #channelStatus}
* will return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #channelStatusAsString}.
*
*
* @return Current status of the signaling channel.
* @see Status
*/
public final Status channelStatus() {
return Status.fromValue(channelStatus);
}
/**
*
* Current status of the signaling channel.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #channelStatus}
* will return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #channelStatusAsString}.
*
*
* @return Current status of the signaling channel.
* @see Status
*/
public final String channelStatusAsString() {
return channelStatus;
}
/**
*
* The time at which the signaling channel was created.
*
*
* @return The time at which the signaling channel was created.
*/
public final Instant creationTime() {
return creationTime;
}
/**
*
* A structure that contains the configuration for the SINGLE_MASTER
channel type.
*
*
* @return A structure that contains the configuration for the SINGLE_MASTER
channel type.
*/
public final SingleMasterConfiguration singleMasterConfiguration() {
return singleMasterConfiguration;
}
/**
*
* The current version of the signaling channel.
*
*
* @return The current version of the signaling channel.
*/
public final String version() {
return version;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(channelName());
hashCode = 31 * hashCode + Objects.hashCode(channelARN());
hashCode = 31 * hashCode + Objects.hashCode(channelTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(channelStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(creationTime());
hashCode = 31 * hashCode + Objects.hashCode(singleMasterConfiguration());
hashCode = 31 * hashCode + Objects.hashCode(version());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ChannelInfo)) {
return false;
}
ChannelInfo other = (ChannelInfo) obj;
return Objects.equals(channelName(), other.channelName()) && Objects.equals(channelARN(), other.channelARN())
&& Objects.equals(channelTypeAsString(), other.channelTypeAsString())
&& Objects.equals(channelStatusAsString(), other.channelStatusAsString())
&& Objects.equals(creationTime(), other.creationTime())
&& Objects.equals(singleMasterConfiguration(), other.singleMasterConfiguration())
&& Objects.equals(version(), other.version());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ChannelInfo").add("ChannelName", channelName()).add("ChannelARN", channelARN())
.add("ChannelType", channelTypeAsString()).add("ChannelStatus", channelStatusAsString())
.add("CreationTime", creationTime()).add("SingleMasterConfiguration", singleMasterConfiguration())
.add("Version", version()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "ChannelName":
return Optional.ofNullable(clazz.cast(channelName()));
case "ChannelARN":
return Optional.ofNullable(clazz.cast(channelARN()));
case "ChannelType":
return Optional.ofNullable(clazz.cast(channelTypeAsString()));
case "ChannelStatus":
return Optional.ofNullable(clazz.cast(channelStatusAsString()));
case "CreationTime":
return Optional.ofNullable(clazz.cast(creationTime()));
case "SingleMasterConfiguration":
return Optional.ofNullable(clazz.cast(singleMasterConfiguration()));
case "Version":
return Optional.ofNullable(clazz.cast(version()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function