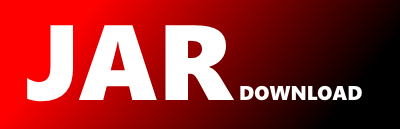
software.amazon.awssdk.services.kinesisvideo.model.UpdateStreamRequest Maven / Gradle / Ivy
Show all versions of kinesisvideo Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kinesisvideo.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class UpdateStreamRequest extends KinesisVideoRequest implements
ToCopyableBuilder {
private static final SdkField STREAM_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StreamName").getter(getter(UpdateStreamRequest::streamName)).setter(setter(Builder::streamName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StreamName").build()).build();
private static final SdkField STREAM_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("StreamARN").getter(getter(UpdateStreamRequest::streamARN)).setter(setter(Builder::streamARN))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StreamARN").build()).build();
private static final SdkField CURRENT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CurrentVersion").getter(getter(UpdateStreamRequest::currentVersion))
.setter(setter(Builder::currentVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CurrentVersion").build()).build();
private static final SdkField DEVICE_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DeviceName").getter(getter(UpdateStreamRequest::deviceName)).setter(setter(Builder::deviceName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeviceName").build()).build();
private static final SdkField MEDIA_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("MediaType").getter(getter(UpdateStreamRequest::mediaType)).setter(setter(Builder::mediaType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MediaType").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(STREAM_NAME_FIELD,
STREAM_ARN_FIELD, CURRENT_VERSION_FIELD, DEVICE_NAME_FIELD, MEDIA_TYPE_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String streamName;
private final String streamARN;
private final String currentVersion;
private final String deviceName;
private final String mediaType;
private UpdateStreamRequest(BuilderImpl builder) {
super(builder);
this.streamName = builder.streamName;
this.streamARN = builder.streamARN;
this.currentVersion = builder.currentVersion;
this.deviceName = builder.deviceName;
this.mediaType = builder.mediaType;
}
/**
*
* The name of the stream whose metadata you want to update.
*
*
* The stream name is an identifier for the stream, and must be unique for each account and region.
*
*
* @return The name of the stream whose metadata you want to update.
*
* The stream name is an identifier for the stream, and must be unique for each account and region.
*/
public final String streamName() {
return streamName;
}
/**
*
* The ARN of the stream whose metadata you want to update.
*
*
* @return The ARN of the stream whose metadata you want to update.
*/
public final String streamARN() {
return streamARN;
}
/**
*
* The version of the stream whose metadata you want to update.
*
*
* @return The version of the stream whose metadata you want to update.
*/
public final String currentVersion() {
return currentVersion;
}
/**
*
* The name of the device that is writing to the stream.
*
*
*
* In the current implementation, Kinesis Video Streams does not use this name.
*
*
*
* @return The name of the device that is writing to the stream.
*
* In the current implementation, Kinesis Video Streams does not use this name.
*
*/
public final String deviceName() {
return deviceName;
}
/**
*
* The stream's media type. Use MediaType
to specify the type of content that the stream contains to
* the consumers of the stream. For more information about media types, see Media Types. If you choose to specify
* the MediaType
, see Naming
* Requirements.
*
*
* To play video on the console, you must specify the correct video type. For example, if the video in the stream is
* H.264, specify video/h264
as the MediaType
.
*
*
* @return The stream's media type. Use MediaType
to specify the type of content that the stream
* contains to the consumers of the stream. For more information about media types, see Media Types. If you choose to
* specify the MediaType
, see Naming
* Requirements.
*
* To play video on the console, you must specify the correct video type. For example, if the video in the
* stream is H.264, specify video/h264
as the MediaType
.
*/
public final String mediaType() {
return mediaType;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(streamName());
hashCode = 31 * hashCode + Objects.hashCode(streamARN());
hashCode = 31 * hashCode + Objects.hashCode(currentVersion());
hashCode = 31 * hashCode + Objects.hashCode(deviceName());
hashCode = 31 * hashCode + Objects.hashCode(mediaType());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof UpdateStreamRequest)) {
return false;
}
UpdateStreamRequest other = (UpdateStreamRequest) obj;
return Objects.equals(streamName(), other.streamName()) && Objects.equals(streamARN(), other.streamARN())
&& Objects.equals(currentVersion(), other.currentVersion()) && Objects.equals(deviceName(), other.deviceName())
&& Objects.equals(mediaType(), other.mediaType());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("UpdateStreamRequest").add("StreamName", streamName()).add("StreamARN", streamARN())
.add("CurrentVersion", currentVersion()).add("DeviceName", deviceName()).add("MediaType", mediaType()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "StreamName":
return Optional.ofNullable(clazz.cast(streamName()));
case "StreamARN":
return Optional.ofNullable(clazz.cast(streamARN()));
case "CurrentVersion":
return Optional.ofNullable(clazz.cast(currentVersion()));
case "DeviceName":
return Optional.ofNullable(clazz.cast(deviceName()));
case "MediaType":
return Optional.ofNullable(clazz.cast(mediaType()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("StreamName", STREAM_NAME_FIELD);
map.put("StreamARN", STREAM_ARN_FIELD);
map.put("CurrentVersion", CURRENT_VERSION_FIELD);
map.put("DeviceName", DEVICE_NAME_FIELD);
map.put("MediaType", MEDIA_TYPE_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function
*
* The stream name is an identifier for the stream, and must be unique for each account and region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder streamName(String streamName);
/**
*
* The ARN of the stream whose metadata you want to update.
*
*
* @param streamARN
* The ARN of the stream whose metadata you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder streamARN(String streamARN);
/**
*
* The version of the stream whose metadata you want to update.
*
*
* @param currentVersion
* The version of the stream whose metadata you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder currentVersion(String currentVersion);
/**
*
* The name of the device that is writing to the stream.
*
*
*
* In the current implementation, Kinesis Video Streams does not use this name.
*
*
*
* @param deviceName
* The name of the device that is writing to the stream.
*
* In the current implementation, Kinesis Video Streams does not use this name.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder deviceName(String deviceName);
/**
*
* The stream's media type. Use MediaType
to specify the type of content that the stream contains
* to the consumers of the stream. For more information about media types, see Media Types. If you choose to
* specify the MediaType
, see Naming
* Requirements.
*
*
* To play video on the console, you must specify the correct video type. For example, if the video in the
* stream is H.264, specify video/h264
as the MediaType
.
*
*
* @param mediaType
* The stream's media type. Use MediaType
to specify the type of content that the stream
* contains to the consumers of the stream. For more information about media types, see Media Types. If you choose to
* specify the MediaType
, see Naming Requirements.
*
* To play video on the console, you must specify the correct video type. For example, if the video in
* the stream is H.264, specify video/h264
as the MediaType
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder mediaType(String mediaType);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends KinesisVideoRequest.BuilderImpl implements Builder {
private String streamName;
private String streamARN;
private String currentVersion;
private String deviceName;
private String mediaType;
private BuilderImpl() {
}
private BuilderImpl(UpdateStreamRequest model) {
super(model);
streamName(model.streamName);
streamARN(model.streamARN);
currentVersion(model.currentVersion);
deviceName(model.deviceName);
mediaType(model.mediaType);
}
public final String getStreamName() {
return streamName;
}
public final void setStreamName(String streamName) {
this.streamName = streamName;
}
@Override
public final Builder streamName(String streamName) {
this.streamName = streamName;
return this;
}
public final String getStreamARN() {
return streamARN;
}
public final void setStreamARN(String streamARN) {
this.streamARN = streamARN;
}
@Override
public final Builder streamARN(String streamARN) {
this.streamARN = streamARN;
return this;
}
public final String getCurrentVersion() {
return currentVersion;
}
public final void setCurrentVersion(String currentVersion) {
this.currentVersion = currentVersion;
}
@Override
public final Builder currentVersion(String currentVersion) {
this.currentVersion = currentVersion;
return this;
}
public final String getDeviceName() {
return deviceName;
}
public final void setDeviceName(String deviceName) {
this.deviceName = deviceName;
}
@Override
public final Builder deviceName(String deviceName) {
this.deviceName = deviceName;
return this;
}
public final String getMediaType() {
return mediaType;
}
public final void setMediaType(String mediaType) {
this.mediaType = mediaType;
}
@Override
public final Builder mediaType(String mediaType) {
this.mediaType = mediaType;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public UpdateStreamRequest build() {
return new UpdateStreamRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
@Override
public Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
}
}