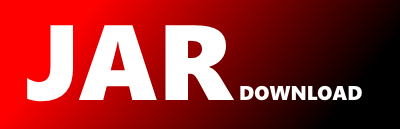
software.amazon.awssdk.services.kms.model.CreateKeyRequest Maven / Gradle / Ivy
Show all versions of kms Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kms.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateKeyRequest extends KmsRequest implements ToCopyableBuilder {
private static final SdkField POLICY_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Policy")
.getter(getter(CreateKeyRequest::policy)).setter(setter(Builder::policy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Policy").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Description").getter(getter(CreateKeyRequest::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField KEY_USAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KeyUsage").getter(getter(CreateKeyRequest::keyUsageAsString)).setter(setter(Builder::keyUsage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyUsage").build()).build();
private static final SdkField CUSTOMER_MASTER_KEY_SPEC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomerMasterKeySpec").getter(getter(CreateKeyRequest::customerMasterKeySpecAsString))
.setter(setter(Builder::customerMasterKeySpec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomerMasterKeySpec").build())
.build();
private static final SdkField KEY_SPEC_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("KeySpec").getter(getter(CreateKeyRequest::keySpecAsString)).setter(setter(Builder::keySpec))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeySpec").build()).build();
private static final SdkField ORIGIN_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Origin")
.getter(getter(CreateKeyRequest::originAsString)).setter(setter(Builder::origin))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Origin").build()).build();
private static final SdkField CUSTOM_KEY_STORE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CustomKeyStoreId").getter(getter(CreateKeyRequest::customKeyStoreId))
.setter(setter(Builder::customKeyStoreId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomKeyStoreId").build()).build();
private static final SdkField BYPASS_POLICY_LOCKOUT_SAFETY_CHECK_FIELD = SdkField
. builder(MarshallingType.BOOLEAN)
.memberName("BypassPolicyLockoutSafetyCheck")
.getter(getter(CreateKeyRequest::bypassPolicyLockoutSafetyCheck))
.setter(setter(Builder::bypassPolicyLockoutSafetyCheck))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("BypassPolicyLockoutSafetyCheck")
.build()).build();
private static final SdkField> TAGS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Tags")
.getter(getter(CreateKeyRequest::tags))
.setter(setter(Builder::tags))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Tags").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Tag::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField MULTI_REGION_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("MultiRegion").getter(getter(CreateKeyRequest::multiRegion)).setter(setter(Builder::multiRegion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("MultiRegion").build()).build();
private static final SdkField XKS_KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("XksKeyId").getter(getter(CreateKeyRequest::xksKeyId)).setter(setter(Builder::xksKeyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("XksKeyId").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays
.asList(POLICY_FIELD, DESCRIPTION_FIELD, KEY_USAGE_FIELD, CUSTOMER_MASTER_KEY_SPEC_FIELD, KEY_SPEC_FIELD,
ORIGIN_FIELD, CUSTOM_KEY_STORE_ID_FIELD, BYPASS_POLICY_LOCKOUT_SAFETY_CHECK_FIELD, TAGS_FIELD,
MULTI_REGION_FIELD, XKS_KEY_ID_FIELD));
private final String policy;
private final String description;
private final String keyUsage;
private final String customerMasterKeySpec;
private final String keySpec;
private final String origin;
private final String customKeyStoreId;
private final Boolean bypassPolicyLockoutSafetyCheck;
private final List tags;
private final Boolean multiRegion;
private final String xksKeyId;
private CreateKeyRequest(BuilderImpl builder) {
super(builder);
this.policy = builder.policy;
this.description = builder.description;
this.keyUsage = builder.keyUsage;
this.customerMasterKeySpec = builder.customerMasterKeySpec;
this.keySpec = builder.keySpec;
this.origin = builder.origin;
this.customKeyStoreId = builder.customKeyStoreId;
this.bypassPolicyLockoutSafetyCheck = builder.bypassPolicyLockoutSafetyCheck;
this.tags = builder.tags;
this.multiRegion = builder.multiRegion;
this.xksKeyId = builder.xksKeyId;
}
/**
*
* The key policy to attach to the KMS key.
*
*
* If you provide a key policy, it must meet the following criteria:
*
*
* -
*
* If you don't set BypassPolicyLockoutSafetyCheck
to true, the key policy must allow the principal
* that is making the CreateKey
request to make a subsequent PutKeyPolicy request on the KMS
* key. This reduces the risk that the KMS key becomes unmanageable. For more information, refer to the scenario in
* the Default Key Policy section of the Key Management Service Developer Guide .
*
*
* -
*
* Each statement in the key policy must contain one or more principals. The principals in the key policy must exist
* and be visible to KMS. When you create a new Amazon Web Services principal (for example, an IAM user or role),
* you might need to enforce a delay before including the new principal in a key policy because the new principal
* might not be immediately visible to KMS. For more information, see Changes that I make are not always immediately visible in the Amazon Web Services Identity and Access
* Management User Guide.
*
*
*
*
* If you do not provide a key policy, KMS attaches a default key policy to the KMS key. For more information, see
* Default Key
* Policy in the Key Management Service Developer Guide.
*
*
* The key policy size quota is 32 kilobytes (32768 bytes).
*
*
* For help writing and formatting a JSON policy document, see the IAM JSON Policy Reference in
* the Identity and Access Management User Guide .
*
*
* @return The key policy to attach to the KMS key.
*
* If you provide a key policy, it must meet the following criteria:
*
*
* -
*
* If you don't set BypassPolicyLockoutSafetyCheck
to true, the key policy must allow the
* principal that is making the CreateKey
request to make a subsequent PutKeyPolicy
* request on the KMS key. This reduces the risk that the KMS key becomes unmanageable. For more
* information, refer to the scenario in the Default Key Policy section of the Key Management Service Developer Guide .
*
*
* -
*
* Each statement in the key policy must contain one or more principals. The principals in the key policy
* must exist and be visible to KMS. When you create a new Amazon Web Services principal (for example, an
* IAM user or role), you might need to enforce a delay before including the new principal in a key policy
* because the new principal might not be immediately visible to KMS. For more information, see Changes that I make are not always immediately visible in the Amazon Web Services Identity and
* Access Management User Guide.
*
*
*
*
* If you do not provide a key policy, KMS attaches a default key policy to the KMS key. For more
* information, see Default
* Key Policy in the Key Management Service Developer Guide.
*
*
* The key policy size quota is 32 kilobytes (32768 bytes).
*
*
* For help writing and formatting a JSON policy document, see the IAM JSON Policy
* Reference in the Identity and Access Management User Guide .
*/
public final String policy() {
return policy;
}
/**
*
* A description of the KMS key.
*
*
* Use a description that helps you decide whether the KMS key is appropriate for a task. The default value is an
* empty string (no description).
*
*
* To set or change the description after the key is created, use UpdateKeyDescription.
*
*
* @return A description of the KMS key.
*
* Use a description that helps you decide whether the KMS key is appropriate for a task. The default value
* is an empty string (no description).
*
*
* To set or change the description after the key is created, use UpdateKeyDescription.
*/
public final String description() {
return description;
}
/**
*
* Determines the cryptographic
* operations for which you can use the KMS key. The default value is ENCRYPT_DECRYPT
. This
* parameter is optional when you are creating a symmetric encryption KMS key; otherwise, it is required. You can't
* change the KeyUsage
value after the KMS key is created.
*
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyUsage} will
* return {@link KeyUsageType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyUsageAsString}.
*
*
* @return Determines the cryptographic operations for which you can use the KMS key. The default value is
* ENCRYPT_DECRYPT
. This parameter is optional when you are creating a symmetric encryption KMS
* key; otherwise, it is required. You can't change the KeyUsage
value after the KMS key is
* created.
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify ENCRYPT_DECRYPT
* or SIGN_VERIFY
.
*
*
* @see KeyUsageType
*/
public final KeyUsageType keyUsage() {
return KeyUsageType.fromValue(keyUsage);
}
/**
*
* Determines the cryptographic
* operations for which you can use the KMS key. The default value is ENCRYPT_DECRYPT
. This
* parameter is optional when you are creating a symmetric encryption KMS key; otherwise, it is required. You can't
* change the KeyUsage
value after the KMS key is created.
*
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyUsage} will
* return {@link KeyUsageType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyUsageAsString}.
*
*
* @return Determines the cryptographic operations for which you can use the KMS key. The default value is
* ENCRYPT_DECRYPT
. This parameter is optional when you are creating a symmetric encryption KMS
* key; otherwise, it is required. You can't change the KeyUsage
value after the KMS key is
* created.
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify ENCRYPT_DECRYPT
* or SIGN_VERIFY
.
*
*
* @see KeyUsageType
*/
public final String keyUsageAsString() {
return keyUsage;
}
/**
*
* Instead, use the KeySpec
parameter.
*
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the names
* differ. We recommend that you use KeySpec
parameter in your code. However, to avoid breaking
* changes, KMS supports both parameters.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #customerMasterKeySpec} will return {@link CustomerMasterKeySpec#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #customerMasterKeySpecAsString}.
*
*
* @return Instead, use the KeySpec
parameter.
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the
* names differ. We recommend that you use KeySpec
parameter in your code. However, to avoid
* breaking changes, KMS supports both parameters.
* @see CustomerMasterKeySpec
* @deprecated This parameter has been deprecated. Instead, use the KeySpec parameter.
*/
@Deprecated
public final CustomerMasterKeySpec customerMasterKeySpec() {
return CustomerMasterKeySpec.fromValue(customerMasterKeySpec);
}
/**
*
* Instead, use the KeySpec
parameter.
*
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the names
* differ. We recommend that you use KeySpec
parameter in your code. However, to avoid breaking
* changes, KMS supports both parameters.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #customerMasterKeySpec} will return {@link CustomerMasterKeySpec#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #customerMasterKeySpecAsString}.
*
*
* @return Instead, use the KeySpec
parameter.
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the
* names differ. We recommend that you use KeySpec
parameter in your code. However, to avoid
* breaking changes, KMS supports both parameters.
* @see CustomerMasterKeySpec
* @deprecated This parameter has been deprecated. Instead, use the KeySpec parameter.
*/
@Deprecated
public final String customerMasterKeySpecAsString() {
return customerMasterKeySpec;
}
/**
*
* Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a KMS key
* with a 256-bit AES-GCM key that is used for encryption and decryption, except in China Regions, where it creates
* a 128-bit symmetric key that uses SM4 encryption. For help choosing a key spec for your KMS key, see Choosing a KMS key
* type in the Key Management Service Developer Guide .
*
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key pair. It
* also determines the algorithms that the KMS key supports. You can't change the KeySpec
after the KMS
* key is created. To further restrict the algorithms that can be used with the KMS key, use a condition key in its
* key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services that are
* integrated with KMS use symmetric encryption KMS keys to protect your data. These services do not support
* asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keySpec} will
* return {@link KeySpec#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keySpecAsString}.
*
*
* @return Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a KMS
* key with a 256-bit AES-GCM key that is used for encryption and decryption, except in China Regions, where
* it creates a 128-bit symmetric key that uses SM4 encryption. For help choosing a key spec for your KMS
* key, see Choosing a
* KMS key type in the Key Management Service Developer Guide .
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key
* pair. It also determines the algorithms that the KMS key supports. You can't change the
* KeySpec
after the KMS key is created. To further restrict the algorithms that can be used
* with the KMS key, use a condition key in its key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services that
* are integrated with KMS use symmetric encryption KMS keys to protect your data. These services do not
* support asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
* @see KeySpec
*/
public final KeySpec keySpec() {
return KeySpec.fromValue(keySpec);
}
/**
*
* Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a KMS key
* with a 256-bit AES-GCM key that is used for encryption and decryption, except in China Regions, where it creates
* a 128-bit symmetric key that uses SM4 encryption. For help choosing a key spec for your KMS key, see Choosing a KMS key
* type in the Key Management Service Developer Guide .
*
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key pair. It
* also determines the algorithms that the KMS key supports. You can't change the KeySpec
after the KMS
* key is created. To further restrict the algorithms that can be used with the KMS key, use a condition key in its
* key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services that are
* integrated with KMS use symmetric encryption KMS keys to protect your data. These services do not support
* asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keySpec} will
* return {@link KeySpec#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keySpecAsString}.
*
*
* @return Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a KMS
* key with a 256-bit AES-GCM key that is used for encryption and decryption, except in China Regions, where
* it creates a 128-bit symmetric key that uses SM4 encryption. For help choosing a key spec for your KMS
* key, see Choosing a
* KMS key type in the Key Management Service Developer Guide .
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key
* pair. It also determines the algorithms that the KMS key supports. You can't change the
* KeySpec
after the KMS key is created. To further restrict the algorithms that can be used
* with the KMS key, use a condition key in its key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services that
* are integrated with KMS use symmetric encryption KMS keys to protect your data. These services do not
* support asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
* @see KeySpec
*/
public final String keySpecAsString() {
return keySpec;
}
/**
*
* The source of the key material for the KMS key. You cannot change the origin after you create the KMS key. The
* default is AWS_KMS
, which means that KMS creates the key material.
*
*
* To create a KMS
* key with no key material (for imported key material), set this value to EXTERNAL
. For more
* information about importing key material into KMS, see Importing Key Material in
* the Key Management Service Developer Guide. The EXTERNAL
origin value is valid only for
* symmetric KMS keys.
*
*
* To create a KMS key in
* an CloudHSM key store and create its key material in the associated CloudHSM cluster, set this value to
* AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
parameter to identify the CloudHSM
* key store. The KeySpec
value must be SYMMETRIC_DEFAULT
.
*
*
* To create a KMS key in an
* external key store, set this value to EXTERNAL_KEY_STORE
. You must also use the
* CustomKeyStoreId
parameter to identify the external key store and the XksKeyId
* parameter to identify the associated external key. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #origin} will
* return {@link OriginType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #originAsString}.
*
*
* @return The source of the key material for the KMS key. You cannot change the origin after you create the KMS
* key. The default is AWS_KMS
, which means that KMS creates the key material.
*
* To create
* a KMS key with no key material (for imported key material), set this value to EXTERNAL
.
* For more information about importing key material into KMS, see Importing Key
* Material in the Key Management Service Developer Guide. The EXTERNAL
origin value
* is valid only for symmetric KMS keys.
*
*
* To create a KMS
* key in an CloudHSM key store and create its key material in the associated CloudHSM cluster, set this
* value to AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
parameter to
* identify the CloudHSM key store. The KeySpec
value must be SYMMETRIC_DEFAULT
.
*
*
* To create a KMS key
* in an external key store, set this value to EXTERNAL_KEY_STORE
. You must also use the
* CustomKeyStoreId
parameter to identify the external key store and the XksKeyId
* parameter to identify the associated external key. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
* @see OriginType
*/
public final OriginType origin() {
return OriginType.fromValue(origin);
}
/**
*
* The source of the key material for the KMS key. You cannot change the origin after you create the KMS key. The
* default is AWS_KMS
, which means that KMS creates the key material.
*
*
* To create a KMS
* key with no key material (for imported key material), set this value to EXTERNAL
. For more
* information about importing key material into KMS, see Importing Key Material in
* the Key Management Service Developer Guide. The EXTERNAL
origin value is valid only for
* symmetric KMS keys.
*
*
* To create a KMS key in
* an CloudHSM key store and create its key material in the associated CloudHSM cluster, set this value to
* AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
parameter to identify the CloudHSM
* key store. The KeySpec
value must be SYMMETRIC_DEFAULT
.
*
*
* To create a KMS key in an
* external key store, set this value to EXTERNAL_KEY_STORE
. You must also use the
* CustomKeyStoreId
parameter to identify the external key store and the XksKeyId
* parameter to identify the associated external key. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #origin} will
* return {@link OriginType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #originAsString}.
*
*
* @return The source of the key material for the KMS key. You cannot change the origin after you create the KMS
* key. The default is AWS_KMS
, which means that KMS creates the key material.
*
* To create
* a KMS key with no key material (for imported key material), set this value to EXTERNAL
.
* For more information about importing key material into KMS, see Importing Key
* Material in the Key Management Service Developer Guide. The EXTERNAL
origin value
* is valid only for symmetric KMS keys.
*
*
* To create a KMS
* key in an CloudHSM key store and create its key material in the associated CloudHSM cluster, set this
* value to AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
parameter to
* identify the CloudHSM key store. The KeySpec
value must be SYMMETRIC_DEFAULT
.
*
*
* To create a KMS key
* in an external key store, set this value to EXTERNAL_KEY_STORE
. You must also use the
* CustomKeyStoreId
parameter to identify the external key store and the XksKeyId
* parameter to identify the associated external key. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
* @see OriginType
*/
public final String originAsString() {
return origin;
}
/**
*
* Creates the KMS key in the specified custom key store.
* The ConnectionState
of the custom key store must be CONNECTED
. To find the
* CustomKeyStoreID and ConnectionState use the DescribeCustomKeyStores operation.
*
*
* This parameter is valid only for symmetric encryption KMS keys in a single Region. You cannot create any other
* type of KMS key in a custom key store.
*
*
* When you create a KMS key in an CloudHSM key store, KMS generates a non-exportable 256-bit symmetric key in its
* associated CloudHSM cluster and associates it with the KMS key. When you create a KMS key in an external key
* store, you must use the XksKeyId
parameter to specify an external key that serves as key material
* for the KMS key.
*
*
* @return Creates the KMS key in the specified custom key
* store. The ConnectionState
of the custom key store must be CONNECTED
. To
* find the CustomKeyStoreID and ConnectionState use the DescribeCustomKeyStores operation.
*
* This parameter is valid only for symmetric encryption KMS keys in a single Region. You cannot create any
* other type of KMS key in a custom key store.
*
*
* When you create a KMS key in an CloudHSM key store, KMS generates a non-exportable 256-bit symmetric key
* in its associated CloudHSM cluster and associates it with the KMS key. When you create a KMS key in an
* external key store, you must use the XksKeyId
parameter to specify an external key that
* serves as key material for the KMS key.
*/
public final String customKeyStoreId() {
return customKeyStoreId;
}
/**
*
* A flag to indicate whether to bypass the key policy lockout safety check.
*
*
*
* Setting this value to true increases the risk that the KMS key becomes unmanageable. Do not set this value to
* true indiscriminately.
*
*
* For more information, refer to the scenario in the Default Key Policy section in the Key Management Service Developer Guide .
*
*
*
* Use this parameter only when you include a policy in the request and you intend to prevent the principal that is
* making the request from making a subsequent PutKeyPolicy request on the KMS key.
*
*
* The default value is false.
*
*
* @return A flag to indicate whether to bypass the key policy lockout safety check.
*
* Setting this value to true increases the risk that the KMS key becomes unmanageable. Do not set this
* value to true indiscriminately.
*
*
* For more information, refer to the scenario in the Default Key Policy section in the Key Management Service Developer Guide .
*
*
*
* Use this parameter only when you include a policy in the request and you intend to prevent the principal
* that is making the request from making a subsequent PutKeyPolicy request on the KMS key.
*
*
* The default value is false.
*/
public final Boolean bypassPolicyLockoutSafetyCheck() {
return bypassPolicyLockoutSafetyCheck;
}
/**
* For responses, this returns true if the service returned a value for the Tags property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasTags() {
return tags != null && !(tags instanceof SdkAutoConstructList);
}
/**
*
* Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To tag an
* existing KMS key, use the TagResource operation.
*
*
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key Management
* Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but the tag
* value can be an empty (null) string. You cannot have more than one tag on a KMS key with the same tag key. If you
* specify an existing tag key with a different tag value, KMS replaces the current tag value with the specified
* one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation report with
* usage and costs aggregated by tags. Tags can also be used to control access to a KMS key. For details, see Tagging Keys.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasTags} method.
*
*
* @return Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To tag
* an existing KMS key, use the TagResource operation.
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key
* Management Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but the
* tag value can be an empty (null) string. You cannot have more than one tag on a KMS key with the same tag
* key. If you specify an existing tag key with a different tag value, KMS replaces the current tag value
* with the specified one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation
* report with usage and costs aggregated by tags. Tags can also be used to control access to a KMS key. For
* details, see Tagging
* Keys.
*/
public final List tags() {
return tags;
}
/**
*
* Creates a multi-Region primary key that you can replicate into other Amazon Web Services Regions. You cannot
* change this value after you create the KMS key.
*
*
* For a multi-Region key, set this parameter to True
. For a single-Region KMS key, omit this parameter
* or set it to False
. The default value is False
.
*
*
* This operation supports multi-Region keys, an KMS feature that lets you create multiple interoperable KMS
* keys in different Amazon Web Services Regions. Because these KMS keys have the same key ID, key material, and
* other metadata, you can use them interchangeably to encrypt data in one Amazon Web Services Region and decrypt it
* in a different Amazon Web Services Region without re-encrypting the data or making a cross-Region call. For more
* information about multi-Region keys, see Multi-Region keys in
* KMS in the Key Management Service Developer Guide.
*
*
* This value creates a primary key, not a replica. To create a replica key, use the
* ReplicateKey operation.
*
*
* You can create a symmetric or asymmetric multi-Region key, and you can create a multi-Region key with imported
* key material. However, you cannot create a multi-Region key in a custom key store.
*
*
* @return Creates a multi-Region primary key that you can replicate into other Amazon Web Services Regions. You
* cannot change this value after you create the KMS key.
*
* For a multi-Region key, set this parameter to True
. For a single-Region KMS key, omit this
* parameter or set it to False
. The default value is False
.
*
*
* This operation supports multi-Region keys, an KMS feature that lets you create multiple
* interoperable KMS keys in different Amazon Web Services Regions. Because these KMS keys have the same key
* ID, key material, and other metadata, you can use them interchangeably to encrypt data in one Amazon Web
* Services Region and decrypt it in a different Amazon Web Services Region without re-encrypting the data
* or making a cross-Region call. For more information about multi-Region keys, see Multi-Region
* keys in KMS in the Key Management Service Developer Guide.
*
*
* This value creates a primary key, not a replica. To create a replica key, use the
* ReplicateKey operation.
*
*
* You can create a symmetric or asymmetric multi-Region key, and you can create a multi-Region key with
* imported key material. However, you cannot create a multi-Region key in a custom key store.
*/
public final Boolean multiRegion() {
return multiRegion;
}
/**
*
* Identifies the external
* key that serves as key material for the KMS key in an external key store.
* Specify the ID that the external
* key store proxy uses to refer to the external key. For help, see the documentation for your external key
* store proxy.
*
*
* This parameter is required for a KMS key with an Origin
value of EXTERNAL_KEY_STORE
. It
* is not valid for KMS keys with any other Origin
value.
*
*
* The external key must be an existing 256-bit AES symmetric encryption key hosted outside of Amazon Web Services
* in an external key manager associated with the external key store specified by the CustomKeyStoreId
* parameter. This key must be enabled and configured to perform encryption and decryption. Each KMS key in an
* external key store must use a different external key. For details, see Requirements for a KMS key in an
* external key store in the Key Management Service Developer Guide.
*
*
* Each KMS key in an external key store is associated two backing keys. One is key material that KMS generates. The
* other is the external key specified by this parameter. When you use the KMS key in an external key store to
* encrypt data, the encryption operation is performed first by KMS using the KMS key material, and then by the
* external key manager using the specified external key, a process known as double encryption. For details,
* see Double encryption in the Key Management Service Developer Guide.
*
*
* @return Identifies the external key that serves as key material for the KMS key in an external key
* store. Specify the ID that the external key store proxy uses to refer to the external key. For help, see the documentation for your
* external key store proxy.
*
* This parameter is required for a KMS key with an Origin
value of
* EXTERNAL_KEY_STORE
. It is not valid for KMS keys with any other Origin
value.
*
*
* The external key must be an existing 256-bit AES symmetric encryption key hosted outside of Amazon Web
* Services in an external key manager associated with the external key store specified by the
* CustomKeyStoreId
parameter. This key must be enabled and configured to perform encryption
* and decryption. Each KMS key in an external key store must use a different external key. For details, see
* Requirements for a KMS
* key in an external key store in the Key Management Service Developer Guide.
*
*
* Each KMS key in an external key store is associated two backing keys. One is key material that KMS
* generates. The other is the external key specified by this parameter. When you use the KMS key in an
* external key store to encrypt data, the encryption operation is performed first by KMS using the KMS key
* material, and then by the external key manager using the specified external key, a process known as
* double encryption. For details, see Double encryption in the Key Management Service Developer Guide.
*/
public final String xksKeyId() {
return xksKeyId;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(policy());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(keyUsageAsString());
hashCode = 31 * hashCode + Objects.hashCode(customerMasterKeySpecAsString());
hashCode = 31 * hashCode + Objects.hashCode(keySpecAsString());
hashCode = 31 * hashCode + Objects.hashCode(originAsString());
hashCode = 31 * hashCode + Objects.hashCode(customKeyStoreId());
hashCode = 31 * hashCode + Objects.hashCode(bypassPolicyLockoutSafetyCheck());
hashCode = 31 * hashCode + Objects.hashCode(hasTags() ? tags() : null);
hashCode = 31 * hashCode + Objects.hashCode(multiRegion());
hashCode = 31 * hashCode + Objects.hashCode(xksKeyId());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateKeyRequest)) {
return false;
}
CreateKeyRequest other = (CreateKeyRequest) obj;
return Objects.equals(policy(), other.policy()) && Objects.equals(description(), other.description())
&& Objects.equals(keyUsageAsString(), other.keyUsageAsString())
&& Objects.equals(customerMasterKeySpecAsString(), other.customerMasterKeySpecAsString())
&& Objects.equals(keySpecAsString(), other.keySpecAsString())
&& Objects.equals(originAsString(), other.originAsString())
&& Objects.equals(customKeyStoreId(), other.customKeyStoreId())
&& Objects.equals(bypassPolicyLockoutSafetyCheck(), other.bypassPolicyLockoutSafetyCheck())
&& hasTags() == other.hasTags() && Objects.equals(tags(), other.tags())
&& Objects.equals(multiRegion(), other.multiRegion()) && Objects.equals(xksKeyId(), other.xksKeyId());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateKeyRequest").add("Policy", policy()).add("Description", description())
.add("KeyUsage", keyUsageAsString()).add("CustomerMasterKeySpec", customerMasterKeySpecAsString())
.add("KeySpec", keySpecAsString()).add("Origin", originAsString()).add("CustomKeyStoreId", customKeyStoreId())
.add("BypassPolicyLockoutSafetyCheck", bypassPolicyLockoutSafetyCheck()).add("Tags", hasTags() ? tags() : null)
.add("MultiRegion", multiRegion()).add("XksKeyId", xksKeyId()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Policy":
return Optional.ofNullable(clazz.cast(policy()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "KeyUsage":
return Optional.ofNullable(clazz.cast(keyUsageAsString()));
case "CustomerMasterKeySpec":
return Optional.ofNullable(clazz.cast(customerMasterKeySpecAsString()));
case "KeySpec":
return Optional.ofNullable(clazz.cast(keySpecAsString()));
case "Origin":
return Optional.ofNullable(clazz.cast(originAsString()));
case "CustomKeyStoreId":
return Optional.ofNullable(clazz.cast(customKeyStoreId()));
case "BypassPolicyLockoutSafetyCheck":
return Optional.ofNullable(clazz.cast(bypassPolicyLockoutSafetyCheck()));
case "Tags":
return Optional.ofNullable(clazz.cast(tags()));
case "MultiRegion":
return Optional.ofNullable(clazz.cast(multiRegion()));
case "XksKeyId":
return Optional.ofNullable(clazz.cast(xksKeyId()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If you provide a key policy, it must meet the following criteria:
*
*
* -
*
* If you don't set BypassPolicyLockoutSafetyCheck
to true, the key policy must allow the
* principal that is making the CreateKey
request to make a subsequent PutKeyPolicy
* request on the KMS key. This reduces the risk that the KMS key becomes unmanageable. For more
* information, refer to the scenario in the Default Key Policy section of the Key Management Service Developer Guide .
*
*
* -
*
* Each statement in the key policy must contain one or more principals. The principals in the key policy
* must exist and be visible to KMS. When you create a new Amazon Web Services principal (for example, an
* IAM user or role), you might need to enforce a delay before including the new principal in a key
* policy because the new principal might not be immediately visible to KMS. For more information, see Changes that I make are not always immediately visible in the Amazon Web Services Identity and
* Access Management User Guide.
*
*
*
*
* If you do not provide a key policy, KMS attaches a default key policy to the KMS key. For more
* information, see Default Key Policy in the Key Management Service Developer Guide.
*
*
* The key policy size quota is 32 kilobytes (32768 bytes).
*
*
* For help writing and formatting a JSON policy document, see the IAM JSON Policy
* Reference in the Identity and Access Management User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder policy(String policy);
/**
*
* A description of the KMS key.
*
*
* Use a description that helps you decide whether the KMS key is appropriate for a task. The default value is
* an empty string (no description).
*
*
* To set or change the description after the key is created, use UpdateKeyDescription.
*
*
* @param description
* A description of the KMS key.
*
* Use a description that helps you decide whether the KMS key is appropriate for a task. The default
* value is an empty string (no description).
*
*
* To set or change the description after the key is created, use UpdateKeyDescription.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder description(String description);
/**
*
* Determines the cryptographic operations for which you can use the KMS key. The default value is
* ENCRYPT_DECRYPT
. This parameter is optional when you are creating a symmetric encryption KMS
* key; otherwise, it is required. You can't change the KeyUsage
value after the KMS key is
* created.
*
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
*
*
* @param keyUsage
* Determines the cryptographic operations for which you can use the KMS key. The default value is
* ENCRYPT_DECRYPT
. This parameter is optional when you are creating a symmetric encryption
* KMS key; otherwise, it is required. You can't change the KeyUsage
value after the KMS key
* is created.
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify
* ENCRYPT_DECRYPT
or SIGN_VERIFY
.
*
*
* @see KeyUsageType
* @return Returns a reference to this object so that method calls can be chained together.
* @see KeyUsageType
*/
Builder keyUsage(String keyUsage);
/**
*
* Determines the cryptographic operations for which you can use the KMS key. The default value is
* ENCRYPT_DECRYPT
. This parameter is optional when you are creating a symmetric encryption KMS
* key; otherwise, it is required. You can't change the KeyUsage
value after the KMS key is
* created.
*
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
*
*
* @param keyUsage
* Determines the cryptographic operations for which you can use the KMS key. The default value is
* ENCRYPT_DECRYPT
. This parameter is optional when you are creating a symmetric encryption
* KMS key; otherwise, it is required. You can't change the KeyUsage
value after the KMS key
* is created.
*
* Select only one valid value.
*
*
* -
*
* For symmetric encryption KMS keys, omit the parameter or specify ENCRYPT_DECRYPT
.
*
*
* -
*
* For HMAC KMS keys (symmetric), specify GENERATE_VERIFY_MAC
.
*
*
* -
*
* For asymmetric KMS keys with RSA key material, specify ENCRYPT_DECRYPT
or
* SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with ECC key material, specify SIGN_VERIFY
.
*
*
* -
*
* For asymmetric KMS keys with SM2 key material (China Regions only), specify
* ENCRYPT_DECRYPT
or SIGN_VERIFY
.
*
*
* @see KeyUsageType
* @return Returns a reference to this object so that method calls can be chained together.
* @see KeyUsageType
*/
Builder keyUsage(KeyUsageType keyUsage);
/**
*
* Instead, use the KeySpec
parameter.
*
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the names
* differ. We recommend that you use KeySpec
parameter in your code. However, to avoid breaking
* changes, KMS supports both parameters.
*
*
* @param customerMasterKeySpec
* Instead, use the KeySpec
parameter.
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the
* names differ. We recommend that you use KeySpec
parameter in your code. However, to avoid
* breaking changes, KMS supports both parameters.
* @see CustomerMasterKeySpec
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomerMasterKeySpec
* @deprecated This parameter has been deprecated. Instead, use the KeySpec parameter.
*/
@Deprecated
Builder customerMasterKeySpec(String customerMasterKeySpec);
/**
*
* Instead, use the KeySpec
parameter.
*
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the names
* differ. We recommend that you use KeySpec
parameter in your code. However, to avoid breaking
* changes, KMS supports both parameters.
*
*
* @param customerMasterKeySpec
* Instead, use the KeySpec
parameter.
*
* The KeySpec
and CustomerMasterKeySpec
parameters work the same way. Only the
* names differ. We recommend that you use KeySpec
parameter in your code. However, to avoid
* breaking changes, KMS supports both parameters.
* @see CustomerMasterKeySpec
* @return Returns a reference to this object so that method calls can be chained together.
* @see CustomerMasterKeySpec
* @deprecated This parameter has been deprecated. Instead, use the KeySpec parameter.
*/
@Deprecated
Builder customerMasterKeySpec(CustomerMasterKeySpec customerMasterKeySpec);
/**
*
* Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a KMS key
* with a 256-bit AES-GCM key that is used for encryption and decryption, except in China Regions, where it
* creates a 128-bit symmetric key that uses SM4 encryption. For help choosing a key spec for your KMS key, see
* Choosing a
* KMS key type in the Key Management Service Developer Guide .
*
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key pair.
* It also determines the algorithms that the KMS key supports. You can't change the KeySpec
after
* the KMS key is created. To further restrict the algorithms that can be used with the KMS key, use a condition
* key in its key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services that are
* integrated with KMS use symmetric encryption KMS keys to protect your data. These services do not support
* asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
*
*
* @param keySpec
* Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a
* KMS key with a 256-bit AES-GCM key that is used for encryption and decryption, except in China
* Regions, where it creates a 128-bit symmetric key that uses SM4 encryption. For help choosing a key
* spec for your KMS key, see Choosing
* a KMS key type in the Key Management Service Developer Guide .
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key
* pair. It also determines the algorithms that the KMS key supports. You can't change the
* KeySpec
after the KMS key is created. To further restrict the algorithms that can be used
* with the KMS key, use a condition key in its key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services
* that are integrated with KMS use symmetric encryption KMS keys to protect your data. These
* services do not support asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
* @see KeySpec
* @return Returns a reference to this object so that method calls can be chained together.
* @see KeySpec
*/
Builder keySpec(String keySpec);
/**
*
* Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a KMS key
* with a 256-bit AES-GCM key that is used for encryption and decryption, except in China Regions, where it
* creates a 128-bit symmetric key that uses SM4 encryption. For help choosing a key spec for your KMS key, see
* Choosing a
* KMS key type in the Key Management Service Developer Guide .
*
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key pair.
* It also determines the algorithms that the KMS key supports. You can't change the KeySpec
after
* the KMS key is created. To further restrict the algorithms that can be used with the KMS key, use a condition
* key in its key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services that are
* integrated with KMS use symmetric encryption KMS keys to protect your data. These services do not support
* asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
*
*
* @param keySpec
* Specifies the type of KMS key to create. The default value, SYMMETRIC_DEFAULT
, creates a
* KMS key with a 256-bit AES-GCM key that is used for encryption and decryption, except in China
* Regions, where it creates a 128-bit symmetric key that uses SM4 encryption. For help choosing a key
* spec for your KMS key, see Choosing
* a KMS key type in the Key Management Service Developer Guide .
*
* The KeySpec
determines whether the KMS key contains a symmetric key or an asymmetric key
* pair. It also determines the algorithms that the KMS key supports. You can't change the
* KeySpec
after the KMS key is created. To further restrict the algorithms that can be used
* with the KMS key, use a condition key in its key policy or IAM policy. For more information, see kms:EncryptionAlgorithm, kms:MacAlgorithm or kms:Signing Algorithm in the Key Management Service Developer Guide .
*
*
*
* Amazon Web Services services
* that are integrated with KMS use symmetric encryption KMS keys to protect your data. These
* services do not support asymmetric KMS keys or HMAC KMS keys.
*
*
*
* KMS supports the following key specs for KMS keys:
*
*
* -
*
* Symmetric encryption key (default)
*
*
* -
*
* SYMMETRIC_DEFAULT
*
*
*
*
* -
*
* HMAC keys (symmetric)
*
*
* -
*
* HMAC_224
*
*
* -
*
* HMAC_256
*
*
* -
*
* HMAC_384
*
*
* -
*
* HMAC_512
*
*
*
*
* -
*
* Asymmetric RSA key pairs
*
*
* -
*
* RSA_2048
*
*
* -
*
* RSA_3072
*
*
* -
*
* RSA_4096
*
*
*
*
* -
*
* Asymmetric NIST-recommended elliptic curve key pairs
*
*
* -
*
* ECC_NIST_P256
(secp256r1)
*
*
* -
*
* ECC_NIST_P384
(secp384r1)
*
*
* -
*
* ECC_NIST_P521
(secp521r1)
*
*
*
*
* -
*
* Other asymmetric elliptic curve key pairs
*
*
* -
*
* ECC_SECG_P256K1
(secp256k1), commonly used for cryptocurrencies.
*
*
*
*
* -
*
* SM2 key pairs (China Regions only)
*
*
* -
*
* SM2
*
*
*
*
* @see KeySpec
* @return Returns a reference to this object so that method calls can be chained together.
* @see KeySpec
*/
Builder keySpec(KeySpec keySpec);
/**
*
* The source of the key material for the KMS key. You cannot change the origin after you create the KMS key.
* The default is AWS_KMS
, which means that KMS creates the key material.
*
*
* To create a
* KMS key with no key material (for imported key material), set this value to EXTERNAL
. For
* more information about importing key material into KMS, see Importing Key Material
* in the Key Management Service Developer Guide. The EXTERNAL
origin value is valid only
* for symmetric KMS keys.
*
*
* To create a KMS key
* in an CloudHSM key store and create its key material in the associated CloudHSM cluster, set this value
* to AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
parameter to identify the
* CloudHSM key store. The KeySpec
value must be SYMMETRIC_DEFAULT
.
*
*
* To create a KMS key in
* an external key store, set this value to EXTERNAL_KEY_STORE
. You must also use the
* CustomKeyStoreId
parameter to identify the external key store and the XksKeyId
* parameter to identify the associated external key. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
*
*
* @param origin
* The source of the key material for the KMS key. You cannot change the origin after you create the KMS
* key. The default is AWS_KMS
, which means that KMS creates the key material.
*
* To create a
* KMS key with no key material (for imported key material), set this value to EXTERNAL
.
* For more information about importing key material into KMS, see Importing Key
* Material in the Key Management Service Developer Guide. The EXTERNAL
origin
* value is valid only for symmetric KMS keys.
*
*
* To create a
* KMS key in an CloudHSM key store and create its key material in the associated CloudHSM cluster,
* set this value to AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
* parameter to identify the CloudHSM key store. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
*
*
* To create a KMS
* key in an external key store, set this value to EXTERNAL_KEY_STORE
. You must also use
* the CustomKeyStoreId
parameter to identify the external key store and the
* XksKeyId
parameter to identify the associated external key. The KeySpec
* value must be SYMMETRIC_DEFAULT
.
* @see OriginType
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginType
*/
Builder origin(String origin);
/**
*
* The source of the key material for the KMS key. You cannot change the origin after you create the KMS key.
* The default is AWS_KMS
, which means that KMS creates the key material.
*
*
* To create a
* KMS key with no key material (for imported key material), set this value to EXTERNAL
. For
* more information about importing key material into KMS, see Importing Key Material
* in the Key Management Service Developer Guide. The EXTERNAL
origin value is valid only
* for symmetric KMS keys.
*
*
* To create a KMS key
* in an CloudHSM key store and create its key material in the associated CloudHSM cluster, set this value
* to AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
parameter to identify the
* CloudHSM key store. The KeySpec
value must be SYMMETRIC_DEFAULT
.
*
*
* To create a KMS key in
* an external key store, set this value to EXTERNAL_KEY_STORE
. You must also use the
* CustomKeyStoreId
parameter to identify the external key store and the XksKeyId
* parameter to identify the associated external key. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
*
*
* @param origin
* The source of the key material for the KMS key. You cannot change the origin after you create the KMS
* key. The default is AWS_KMS
, which means that KMS creates the key material.
*
* To create a
* KMS key with no key material (for imported key material), set this value to EXTERNAL
.
* For more information about importing key material into KMS, see Importing Key
* Material in the Key Management Service Developer Guide. The EXTERNAL
origin
* value is valid only for symmetric KMS keys.
*
*
* To create a
* KMS key in an CloudHSM key store and create its key material in the associated CloudHSM cluster,
* set this value to AWS_CLOUDHSM
. You must also use the CustomKeyStoreId
* parameter to identify the CloudHSM key store. The KeySpec
value must be
* SYMMETRIC_DEFAULT
.
*
*
* To create a KMS
* key in an external key store, set this value to EXTERNAL_KEY_STORE
. You must also use
* the CustomKeyStoreId
parameter to identify the external key store and the
* XksKeyId
parameter to identify the associated external key. The KeySpec
* value must be SYMMETRIC_DEFAULT
.
* @see OriginType
* @return Returns a reference to this object so that method calls can be chained together.
* @see OriginType
*/
Builder origin(OriginType origin);
/**
*
* Creates the KMS key in the specified custom key
* store. The ConnectionState
of the custom key store must be CONNECTED
. To find
* the CustomKeyStoreID and ConnectionState use the DescribeCustomKeyStores operation.
*
*
* This parameter is valid only for symmetric encryption KMS keys in a single Region. You cannot create any
* other type of KMS key in a custom key store.
*
*
* When you create a KMS key in an CloudHSM key store, KMS generates a non-exportable 256-bit symmetric key in
* its associated CloudHSM cluster and associates it with the KMS key. When you create a KMS key in an external
* key store, you must use the XksKeyId
parameter to specify an external key that serves as key
* material for the KMS key.
*
*
* @param customKeyStoreId
* Creates the KMS key in the specified custom key
* store. The ConnectionState
of the custom key store must be CONNECTED
. To
* find the CustomKeyStoreID and ConnectionState use the DescribeCustomKeyStores operation.
*
* This parameter is valid only for symmetric encryption KMS keys in a single Region. You cannot create
* any other type of KMS key in a custom key store.
*
*
* When you create a KMS key in an CloudHSM key store, KMS generates a non-exportable 256-bit symmetric
* key in its associated CloudHSM cluster and associates it with the KMS key. When you create a KMS key
* in an external key store, you must use the XksKeyId
parameter to specify an external key
* that serves as key material for the KMS key.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder customKeyStoreId(String customKeyStoreId);
/**
*
* A flag to indicate whether to bypass the key policy lockout safety check.
*
*
*
* Setting this value to true increases the risk that the KMS key becomes unmanageable. Do not set this value to
* true indiscriminately.
*
*
* For more information, refer to the scenario in the Default Key Policy section in the Key Management Service Developer Guide .
*
*
*
* Use this parameter only when you include a policy in the request and you intend to prevent the principal that
* is making the request from making a subsequent PutKeyPolicy request on the KMS key.
*
*
* The default value is false.
*
*
* @param bypassPolicyLockoutSafetyCheck
* A flag to indicate whether to bypass the key policy lockout safety check.
*
* Setting this value to true increases the risk that the KMS key becomes unmanageable. Do not set this
* value to true indiscriminately.
*
*
* For more information, refer to the scenario in the Default Key Policy section in the Key Management Service Developer Guide .
*
*
*
* Use this parameter only when you include a policy in the request and you intend to prevent the
* principal that is making the request from making a subsequent PutKeyPolicy request on the KMS
* key.
*
*
* The default value is false.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder bypassPolicyLockoutSafetyCheck(Boolean bypassPolicyLockoutSafetyCheck);
/**
*
* Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To tag an
* existing KMS key, use the TagResource operation.
*
*
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key
* Management Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but the tag
* value can be an empty (null) string. You cannot have more than one tag on a KMS key with the same tag key. If
* you specify an existing tag key with a different tag value, KMS replaces the current tag value with the
* specified one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation report
* with usage and costs aggregated by tags. Tags can also be used to control access to a KMS key. For details,
* see Tagging Keys.
*
*
* @param tags
* Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To
* tag an existing KMS key, use the TagResource operation.
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key
* Management Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but
* the tag value can be an empty (null) string. You cannot have more than one tag on a KMS key with the
* same tag key. If you specify an existing tag key with a different tag value, KMS replaces the current
* tag value with the specified one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation
* report with usage and costs aggregated by tags. Tags can also be used to control access to a KMS key.
* For details, see Tagging Keys.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Collection tags);
/**
*
* Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To tag an
* existing KMS key, use the TagResource operation.
*
*
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key
* Management Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but the tag
* value can be an empty (null) string. You cannot have more than one tag on a KMS key with the same tag key. If
* you specify an existing tag key with a different tag value, KMS replaces the current tag value with the
* specified one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation report
* with usage and costs aggregated by tags. Tags can also be used to control access to a KMS key. For details,
* see Tagging Keys.
*
*
* @param tags
* Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To
* tag an existing KMS key, use the TagResource operation.
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key
* Management Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but
* the tag value can be an empty (null) string. You cannot have more than one tag on a KMS key with the
* same tag key. If you specify an existing tag key with a different tag value, KMS replaces the current
* tag value with the specified one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation
* report with usage and costs aggregated by tags. Tags can also be used to control access to a KMS key.
* For details, see Tagging Keys.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder tags(Tag... tags);
/**
*
* Assigns one or more tags to the KMS key. Use this parameter to tag the KMS key when it is created. To tag an
* existing KMS key, use the TagResource operation.
*
*
*
* Tagging or untagging a KMS key can allow or deny permission to the KMS key. For details, see ABAC for KMS in the Key
* Management Service Developer Guide.
*
*
*
* To use this parameter, you must have kms:TagResource permission in an IAM policy.
*
*
* Each tag consists of a tag key and a tag value. Both the tag key and the tag value are required, but the tag
* value can be an empty (null) string. You cannot have more than one tag on a KMS key with the same tag key. If
* you specify an existing tag key with a different tag value, KMS replaces the current tag value with the
* specified one.
*
*
* When you add tags to an Amazon Web Services resource, Amazon Web Services generates a cost allocation report
* with usage and costs aggregated by tags. Tags can also be used to control access to a KMS key. For details,
* see Tagging Keys.
*
* This is a convenience method that creates an instance of the
* {@link software.amazon.awssdk.services.kms.model.Tag.Builder} avoiding the need to create one manually via
* {@link software.amazon.awssdk.services.kms.model.Tag#builder()}.
*
*
* When the {@link Consumer} completes, {@link software.amazon.awssdk.services.kms.model.Tag.Builder#build()} is
* called immediately and its result is passed to {@link #tags(List)}.
*
* @param tags
* a consumer that will call methods on {@link software.amazon.awssdk.services.kms.model.Tag.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #tags(java.util.Collection)
*/
Builder tags(Consumer... tags);
/**
*
* Creates a multi-Region primary key that you can replicate into other Amazon Web Services Regions. You cannot
* change this value after you create the KMS key.
*
*
* For a multi-Region key, set this parameter to True
. For a single-Region KMS key, omit this
* parameter or set it to False
. The default value is False
.
*
*
* This operation supports multi-Region keys, an KMS feature that lets you create multiple interoperable
* KMS keys in different Amazon Web Services Regions. Because these KMS keys have the same key ID, key material,
* and other metadata, you can use them interchangeably to encrypt data in one Amazon Web Services Region and
* decrypt it in a different Amazon Web Services Region without re-encrypting the data or making a cross-Region
* call. For more information about multi-Region keys, see Multi-Region
* keys in KMS in the Key Management Service Developer Guide.
*
*
* This value creates a primary key, not a replica. To create a replica key, use the
* ReplicateKey operation.
*
*
* You can create a symmetric or asymmetric multi-Region key, and you can create a multi-Region key with
* imported key material. However, you cannot create a multi-Region key in a custom key store.
*
*
* @param multiRegion
* Creates a multi-Region primary key that you can replicate into other Amazon Web Services Regions. You
* cannot change this value after you create the KMS key.
*
* For a multi-Region key, set this parameter to True
. For a single-Region KMS key, omit
* this parameter or set it to False
. The default value is False
.
*
*
* This operation supports multi-Region keys, an KMS feature that lets you create multiple
* interoperable KMS keys in different Amazon Web Services Regions. Because these KMS keys have the same
* key ID, key material, and other metadata, you can use them interchangeably to encrypt data in one
* Amazon Web Services Region and decrypt it in a different Amazon Web Services Region without
* re-encrypting the data or making a cross-Region call. For more information about multi-Region keys,
* see Multi
* -Region keys in KMS in the Key Management Service Developer Guide.
*
*
* This value creates a primary key, not a replica. To create a replica key, use the
* ReplicateKey operation.
*
*
* You can create a symmetric or asymmetric multi-Region key, and you can create a multi-Region key with
* imported key material. However, you cannot create a multi-Region key in a custom key store.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder multiRegion(Boolean multiRegion);
/**
*
* Identifies the external key that serves as key material for the KMS key in an external key store.
* Specify the ID that the external key store proxy uses to refer to the external key. For help, see the documentation for your
* external key store proxy.
*
*
* This parameter is required for a KMS key with an Origin
value of EXTERNAL_KEY_STORE
* . It is not valid for KMS keys with any other Origin
value.
*
*
* The external key must be an existing 256-bit AES symmetric encryption key hosted outside of Amazon Web
* Services in an external key manager associated with the external key store specified by the
* CustomKeyStoreId
parameter. This key must be enabled and configured to perform encryption and
* decryption. Each KMS key in an external key store must use a different external key. For details, see Requirements for a KMS key in an
* external key store in the Key Management Service Developer Guide.
*
*
* Each KMS key in an external key store is associated two backing keys. One is key material that KMS generates.
* The other is the external key specified by this parameter. When you use the KMS key in an external key store
* to encrypt data, the encryption operation is performed first by KMS using the KMS key material, and then by
* the external key manager using the specified external key, a process known as double encryption. For
* details, see Double encryption in the Key Management Service Developer Guide.
*
*
* @param xksKeyId
* Identifies the external key that serves as key material for the KMS key in an external key
* store. Specify the ID that the external key store proxy uses to refer to the external key. For help, see the documentation for
* your external key store proxy.
*
* This parameter is required for a KMS key with an Origin
value of
* EXTERNAL_KEY_STORE
. It is not valid for KMS keys with any other Origin
* value.
*
*
* The external key must be an existing 256-bit AES symmetric encryption key hosted outside of Amazon Web
* Services in an external key manager associated with the external key store specified by the
* CustomKeyStoreId
parameter. This key must be enabled and configured to perform encryption
* and decryption. Each KMS key in an external key store must use a different external key. For details,
* see Requirements for a
* KMS key in an external key store in the Key Management Service Developer Guide.
*
*
* Each KMS key in an external key store is associated two backing keys. One is key material that KMS
* generates. The other is the external key specified by this parameter. When you use the KMS key in an
* external key store to encrypt data, the encryption operation is performed first by KMS using the KMS
* key material, and then by the external key manager using the specified external key, a process known
* as double encryption. For details, see Double encryption in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder xksKeyId(String xksKeyId);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends KmsRequest.BuilderImpl implements Builder {
private String policy;
private String description;
private String keyUsage;
private String customerMasterKeySpec;
private String keySpec;
private String origin;
private String customKeyStoreId;
private Boolean bypassPolicyLockoutSafetyCheck;
private List tags = DefaultSdkAutoConstructList.getInstance();
private Boolean multiRegion;
private String xksKeyId;
private BuilderImpl() {
}
private BuilderImpl(CreateKeyRequest model) {
super(model);
policy(model.policy);
description(model.description);
keyUsage(model.keyUsage);
customerMasterKeySpec(model.customerMasterKeySpec);
keySpec(model.keySpec);
origin(model.origin);
customKeyStoreId(model.customKeyStoreId);
bypassPolicyLockoutSafetyCheck(model.bypassPolicyLockoutSafetyCheck);
tags(model.tags);
multiRegion(model.multiRegion);
xksKeyId(model.xksKeyId);
}
public final String getPolicy() {
return policy;
}
public final void setPolicy(String policy) {
this.policy = policy;
}
@Override
public final Builder policy(String policy) {
this.policy = policy;
return this;
}
public final String getDescription() {
return description;
}
public final void setDescription(String description) {
this.description = description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final String getKeyUsage() {
return keyUsage;
}
public final void setKeyUsage(String keyUsage) {
this.keyUsage = keyUsage;
}
@Override
public final Builder keyUsage(String keyUsage) {
this.keyUsage = keyUsage;
return this;
}
@Override
public final Builder keyUsage(KeyUsageType keyUsage) {
this.keyUsage(keyUsage == null ? null : keyUsage.toString());
return this;
}
@Deprecated
public final String getCustomerMasterKeySpec() {
return customerMasterKeySpec;
}
@Deprecated
public final void setCustomerMasterKeySpec(String customerMasterKeySpec) {
this.customerMasterKeySpec = customerMasterKeySpec;
}
@Override
@Deprecated
public final Builder customerMasterKeySpec(String customerMasterKeySpec) {
this.customerMasterKeySpec = customerMasterKeySpec;
return this;
}
@Override
@Deprecated
public final Builder customerMasterKeySpec(CustomerMasterKeySpec customerMasterKeySpec) {
this.customerMasterKeySpec(customerMasterKeySpec == null ? null : customerMasterKeySpec.toString());
return this;
}
public final String getKeySpec() {
return keySpec;
}
public final void setKeySpec(String keySpec) {
this.keySpec = keySpec;
}
@Override
public final Builder keySpec(String keySpec) {
this.keySpec = keySpec;
return this;
}
@Override
public final Builder keySpec(KeySpec keySpec) {
this.keySpec(keySpec == null ? null : keySpec.toString());
return this;
}
public final String getOrigin() {
return origin;
}
public final void setOrigin(String origin) {
this.origin = origin;
}
@Override
public final Builder origin(String origin) {
this.origin = origin;
return this;
}
@Override
public final Builder origin(OriginType origin) {
this.origin(origin == null ? null : origin.toString());
return this;
}
public final String getCustomKeyStoreId() {
return customKeyStoreId;
}
public final void setCustomKeyStoreId(String customKeyStoreId) {
this.customKeyStoreId = customKeyStoreId;
}
@Override
public final Builder customKeyStoreId(String customKeyStoreId) {
this.customKeyStoreId = customKeyStoreId;
return this;
}
public final Boolean getBypassPolicyLockoutSafetyCheck() {
return bypassPolicyLockoutSafetyCheck;
}
public final void setBypassPolicyLockoutSafetyCheck(Boolean bypassPolicyLockoutSafetyCheck) {
this.bypassPolicyLockoutSafetyCheck = bypassPolicyLockoutSafetyCheck;
}
@Override
public final Builder bypassPolicyLockoutSafetyCheck(Boolean bypassPolicyLockoutSafetyCheck) {
this.bypassPolicyLockoutSafetyCheck = bypassPolicyLockoutSafetyCheck;
return this;
}
public final List getTags() {
List result = TagListCopier.copyToBuilder(this.tags);
if (result instanceof SdkAutoConstructList) {
return null;
}
return result;
}
public final void setTags(Collection tags) {
this.tags = TagListCopier.copyFromBuilder(tags);
}
@Override
public final Builder tags(Collection tags) {
this.tags = TagListCopier.copy(tags);
return this;
}
@Override
@SafeVarargs
public final Builder tags(Tag... tags) {
tags(Arrays.asList(tags));
return this;
}
@Override
@SafeVarargs
public final Builder tags(Consumer... tags) {
tags(Stream.of(tags).map(c -> Tag.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final Boolean getMultiRegion() {
return multiRegion;
}
public final void setMultiRegion(Boolean multiRegion) {
this.multiRegion = multiRegion;
}
@Override
public final Builder multiRegion(Boolean multiRegion) {
this.multiRegion = multiRegion;
return this;
}
public final String getXksKeyId() {
return xksKeyId;
}
public final void setXksKeyId(String xksKeyId) {
this.xksKeyId = xksKeyId;
}
@Override
public final Builder xksKeyId(String xksKeyId) {
this.xksKeyId = xksKeyId;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateKeyRequest build() {
return new CreateKeyRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}