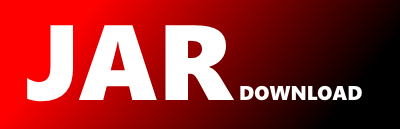
software.amazon.awssdk.services.kms.model.CreateGrantRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kms.model;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateGrantRequest extends KmsRequest implements
ToCopyableBuilder {
private static final SdkField KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING).memberName("KeyId")
.getter(getter(CreateGrantRequest::keyId)).setter(setter(Builder::keyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyId").build()).build();
private static final SdkField GRANTEE_PRINCIPAL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("GranteePrincipal").getter(getter(CreateGrantRequest::granteePrincipal))
.setter(setter(Builder::granteePrincipal))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GranteePrincipal").build()).build();
private static final SdkField RETIRING_PRINCIPAL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RetiringPrincipal").getter(getter(CreateGrantRequest::retiringPrincipal))
.setter(setter(Builder::retiringPrincipal))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RetiringPrincipal").build()).build();
private static final SdkField> OPERATIONS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Operations")
.getter(getter(CreateGrantRequest::operationsAsStrings))
.setter(setter(Builder::operationsWithStrings))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Operations").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONSTRAINTS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("Constraints")
.getter(getter(CreateGrantRequest::constraints)).setter(setter(Builder::constraints))
.constructor(GrantConstraints::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Constraints").build()).build();
private static final SdkField> GRANT_TOKENS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("GrantTokens")
.getter(getter(CreateGrantRequest::grantTokens))
.setter(setter(Builder::grantTokens))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("GrantTokens").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Name")
.getter(getter(CreateGrantRequest::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Name").build()).build();
private static final SdkField DRY_RUN_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DryRun").getter(getter(CreateGrantRequest::dryRun)).setter(setter(Builder::dryRun))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DryRun").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(KEY_ID_FIELD,
GRANTEE_PRINCIPAL_FIELD, RETIRING_PRINCIPAL_FIELD, OPERATIONS_FIELD, CONSTRAINTS_FIELD, GRANT_TOKENS_FIELD,
NAME_FIELD, DRY_RUN_FIELD));
private final String keyId;
private final String granteePrincipal;
private final String retiringPrincipal;
private final List operations;
private final GrantConstraints constraints;
private final List grantTokens;
private final String name;
private final Boolean dryRun;
private CreateGrantRequest(BuilderImpl builder) {
super(builder);
this.keyId = builder.keyId;
this.granteePrincipal = builder.granteePrincipal;
this.retiringPrincipal = builder.retiringPrincipal;
this.operations = builder.operations;
this.constraints = builder.constraints;
this.grantTokens = builder.grantTokens;
this.name = builder.name;
this.dryRun = builder.dryRun;
}
/**
*
* Identifies the KMS key for the grant. The grant gives principals permission to use this KMS key.
*
*
* Specify the key ID or key ARN of the KMS key. To specify a KMS key in a different Amazon Web Services account,
* you must use the key ARN.
*
*
* For example:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-east-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
*
*
* To get the key ID and key ARN for a KMS key, use ListKeys or DescribeKey.
*
*
* @return Identifies the KMS key for the grant. The grant gives principals permission to use this KMS key.
*
* Specify the key ID or key ARN of the KMS key. To specify a KMS key in a different Amazon Web Services
* account, you must use the key ARN.
*
*
* For example:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-east-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
*
*
* To get the key ID and key ARN for a KMS key, use ListKeys or DescribeKey.
*/
public final String keyId() {
return keyId;
}
/**
*
* The identity that gets the permissions specified in the grant.
*
*
* To specify the grantee principal, use the Amazon Resource Name (ARN) of an Amazon Web Services principal. Valid
* principals include Amazon Web Services accounts, IAM users, IAM roles, federated users, and assumed role users.
* For help with the ARN syntax for a principal, see IAM ARNs
* in the Identity and Access Management User Guide .
*
*
* @return The identity that gets the permissions specified in the grant.
*
* To specify the grantee principal, use the Amazon Resource Name (ARN) of an Amazon Web Services principal.
* Valid principals include Amazon Web Services accounts, IAM users, IAM roles, federated users, and assumed
* role users. For help with the ARN syntax for a principal, see IAM
* ARNs in the Identity and Access Management User Guide .
*/
public final String granteePrincipal() {
return granteePrincipal;
}
/**
*
* The principal that has permission to use the RetireGrant operation to retire the grant.
*
*
* To specify the principal, use the Amazon Resource Name (ARN)
* of an Amazon Web Services principal. Valid principals include Amazon Web Services accounts, IAM users, IAM roles,
* federated users, and assumed role users. For help with the ARN syntax for a principal, see IAM ARNs
* in the Identity and Access Management User Guide .
*
*
* The grant determines the retiring principal. Other principals might have permission to retire the grant or revoke
* the grant. For details, see RevokeGrant and Retiring and revoking
* grants in the Key Management Service Developer Guide.
*
*
* @return The principal that has permission to use the RetireGrant operation to retire the grant.
*
* To specify the principal, use the Amazon Resource Name
* (ARN) of an Amazon Web Services principal. Valid principals include Amazon Web Services accounts, IAM
* users, IAM roles, federated users, and assumed role users. For help with the ARN syntax for a principal,
* see IAM
* ARNs in the Identity and Access Management User Guide .
*
*
* The grant determines the retiring principal. Other principals might have permission to retire the grant
* or revoke the grant. For details, see RevokeGrant and Retiring and
* revoking grants in the Key Management Service Developer Guide.
*/
public final String retiringPrincipal() {
return retiringPrincipal;
}
/**
*
* A list of operations that the grant permits.
*
*
* This list must include only operations that are permitted in a grant. Also, the operation must be supported on
* the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that allows the
* Sign operation, or a grant for an asymmetric KMS key that allows the GenerateDataKey operation. If
* you try, KMS returns a ValidationError
exception. For details, see Grant
* operations in the Key Management Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOperations} method.
*
*
* @return A list of operations that the grant permits.
*
* This list must include only operations that are permitted in a grant. Also, the operation must be
* supported on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that
* allows the Sign operation, or a grant for an asymmetric KMS key that allows the
* GenerateDataKey operation. If you try, KMS returns a ValidationError
exception. For
* details, see Grant
* operations in the Key Management Service Developer Guide.
*/
public final List operations() {
return GrantOperationListCopier.copyStringToEnum(operations);
}
/**
* For responses, this returns true if the service returned a value for the Operations property. This DOES NOT check
* that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is
* useful because the SDK will never return a null collection or map, but you may need to differentiate between the
* service returning nothing (or null) and the service returning an empty collection or map. For requests, this
* returns true if a value for the property was specified in the request builder, and false if a value was not
* specified.
*/
public final boolean hasOperations() {
return operations != null && !(operations instanceof SdkAutoConstructList);
}
/**
*
* A list of operations that the grant permits.
*
*
* This list must include only operations that are permitted in a grant. Also, the operation must be supported on
* the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that allows the
* Sign operation, or a grant for an asymmetric KMS key that allows the GenerateDataKey operation. If
* you try, KMS returns a ValidationError
exception. For details, see Grant
* operations in the Key Management Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasOperations} method.
*
*
* @return A list of operations that the grant permits.
*
* This list must include only operations that are permitted in a grant. Also, the operation must be
* supported on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that
* allows the Sign operation, or a grant for an asymmetric KMS key that allows the
* GenerateDataKey operation. If you try, KMS returns a ValidationError
exception. For
* details, see Grant
* operations in the Key Management Service Developer Guide.
*/
public final List operationsAsStrings() {
return operations;
}
/**
*
* Specifies a grant constraint.
*
*
*
* Do not include confidential or sensitive information in this field. This field may be displayed in plaintext in
* CloudTrail logs and other output.
*
*
*
* KMS supports the EncryptionContextEquals
and EncryptionContextSubset
grant constraints,
* which allow the permissions in the grant only when the encryption context in the request matches (
* EncryptionContextEquals
) or includes (EncryptionContextSubset
) the encryption context
* specified in the constraint.
*
*
* The encryption context grant constraints are supported only on grant
* operations that include an EncryptionContext
parameter, such as cryptographic operations on
* symmetric encryption KMS keys. Grants with grant constraints can include the DescribeKey and
* RetireGrant operations, but the constraint doesn't apply to these operations. If a grant with a grant
* constraint includes the CreateGrant
operation, the constraint requires that any grants created with
* the CreateGrant
permission have an equally strict or stricter encryption context constraint.
*
*
* You cannot use an encryption context grant constraint for cryptographic operations with asymmetric KMS keys or
* HMAC KMS keys. Operations with these keys don't support an encryption context.
*
*
* Each constraint value can include up to 8 encryption context pairs. The encryption context value in each
* constraint cannot exceed 384 characters. For information about grant constraints, see Using
* grant constraints in the Key Management Service Developer Guide. For more information about encryption
* context, see Encryption context
* in the Key Management Service Developer Guide .
*
*
* @return Specifies a grant constraint.
*
* Do not include confidential or sensitive information in this field. This field may be displayed in
* plaintext in CloudTrail logs and other output.
*
*
*
* KMS supports the EncryptionContextEquals
and EncryptionContextSubset
grant
* constraints, which allow the permissions in the grant only when the encryption context in the request
* matches (EncryptionContextEquals
) or includes (EncryptionContextSubset
) the
* encryption context specified in the constraint.
*
*
* The encryption context grant constraints are supported only on grant
* operations that include an EncryptionContext
parameter, such as cryptographic operations
* on symmetric encryption KMS keys. Grants with grant constraints can include the DescribeKey and
* RetireGrant operations, but the constraint doesn't apply to these operations. If a grant with a
* grant constraint includes the CreateGrant
operation, the constraint requires that any grants
* created with the CreateGrant
permission have an equally strict or stricter encryption
* context constraint.
*
*
* You cannot use an encryption context grant constraint for cryptographic operations with asymmetric KMS
* keys or HMAC KMS keys. Operations with these keys don't support an encryption context.
*
*
* Each constraint value can include up to 8 encryption context pairs. The encryption context value in each
* constraint cannot exceed 384 characters. For information about grant constraints, see Using grant constraints in the Key Management Service Developer Guide. For more information
* about encryption context, see Encryption
* context in the Key Management Service Developer Guide .
*/
public final GrantConstraints constraints() {
return constraints;
}
/**
* For responses, this returns true if the service returned a value for the GrantTokens property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasGrantTokens() {
return grantTokens != null && !(grantTokens instanceof SdkAutoConstructList);
}
/**
*
* A list of grant tokens.
*
*
* Use a grant token when your permission to call this operation comes from a new grant that has not yet achieved
* eventual consistency. For more information, see Grant token and Using a grant
* token in the Key Management Service Developer Guide.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasGrantTokens} method.
*
*
* @return A list of grant tokens.
*
* Use a grant token when your permission to call this operation comes from a new grant that has not yet
* achieved eventual consistency. For more information, see Grant token and
* Using
* a grant token in the Key Management Service Developer Guide.
*/
public final List grantTokens() {
return grantTokens;
}
/**
*
* A friendly name for the grant. Use this value to prevent the unintended creation of duplicate grants when
* retrying this request.
*
*
*
* Do not include confidential or sensitive information in this field. This field may be displayed in plaintext in
* CloudTrail logs and other output.
*
*
*
* When this value is absent, all CreateGrant
requests result in a new grant with a unique
* GrantId
even if all the supplied parameters are identical. This can result in unintended duplicates
* when you retry the CreateGrant
request.
*
*
* When this value is present, you can retry a CreateGrant
request with identical parameters; if the
* grant already exists, the original GrantId
is returned without creating a new grant. Note that the
* returned grant token is unique with every CreateGrant
request, even when a duplicate
* GrantId
is returned. All grant tokens for the same grant ID can be used interchangeably.
*
*
* @return A friendly name for the grant. Use this value to prevent the unintended creation of duplicate grants when
* retrying this request.
*
* Do not include confidential or sensitive information in this field. This field may be displayed in
* plaintext in CloudTrail logs and other output.
*
*
*
* When this value is absent, all CreateGrant
requests result in a new grant with a unique
* GrantId
even if all the supplied parameters are identical. This can result in unintended
* duplicates when you retry the CreateGrant
request.
*
*
* When this value is present, you can retry a CreateGrant
request with identical parameters;
* if the grant already exists, the original GrantId
is returned without creating a new grant.
* Note that the returned grant token is unique with every CreateGrant
request, even when a
* duplicate GrantId
is returned. All grant tokens for the same grant ID can be used
* interchangeably.
*/
public final String name() {
return name;
}
/**
*
* Checks if your request will succeed. DryRun
is an optional parameter.
*
*
* To learn more about how to use this parameter, see Testing your KMS API
* calls in the Key Management Service Developer Guide.
*
*
* @return Checks if your request will succeed. DryRun
is an optional parameter.
*
* To learn more about how to use this parameter, see Testing your KMS API
* calls in the Key Management Service Developer Guide.
*/
public final Boolean dryRun() {
return dryRun;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(keyId());
hashCode = 31 * hashCode + Objects.hashCode(granteePrincipal());
hashCode = 31 * hashCode + Objects.hashCode(retiringPrincipal());
hashCode = 31 * hashCode + Objects.hashCode(hasOperations() ? operationsAsStrings() : null);
hashCode = 31 * hashCode + Objects.hashCode(constraints());
hashCode = 31 * hashCode + Objects.hashCode(hasGrantTokens() ? grantTokens() : null);
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(dryRun());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateGrantRequest)) {
return false;
}
CreateGrantRequest other = (CreateGrantRequest) obj;
return Objects.equals(keyId(), other.keyId()) && Objects.equals(granteePrincipal(), other.granteePrincipal())
&& Objects.equals(retiringPrincipal(), other.retiringPrincipal()) && hasOperations() == other.hasOperations()
&& Objects.equals(operationsAsStrings(), other.operationsAsStrings())
&& Objects.equals(constraints(), other.constraints()) && hasGrantTokens() == other.hasGrantTokens()
&& Objects.equals(grantTokens(), other.grantTokens()) && Objects.equals(name(), other.name())
&& Objects.equals(dryRun(), other.dryRun());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CreateGrantRequest").add("KeyId", keyId()).add("GranteePrincipal", granteePrincipal())
.add("RetiringPrincipal", retiringPrincipal()).add("Operations", hasOperations() ? operationsAsStrings() : null)
.add("Constraints", constraints()).add("GrantTokens", hasGrantTokens() ? grantTokens() : null)
.add("Name", name()).add("DryRun", dryRun()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "KeyId":
return Optional.ofNullable(clazz.cast(keyId()));
case "GranteePrincipal":
return Optional.ofNullable(clazz.cast(granteePrincipal()));
case "RetiringPrincipal":
return Optional.ofNullable(clazz.cast(retiringPrincipal()));
case "Operations":
return Optional.ofNullable(clazz.cast(operationsAsStrings()));
case "Constraints":
return Optional.ofNullable(clazz.cast(constraints()));
case "GrantTokens":
return Optional.ofNullable(clazz.cast(grantTokens()));
case "Name":
return Optional.ofNullable(clazz.cast(name()));
case "DryRun":
return Optional.ofNullable(clazz.cast(dryRun()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Specify the key ID or key ARN of the KMS key. To specify a KMS key in a different Amazon Web Services
* account, you must use the key ARN.
*
*
* For example:
*
*
* -
*
* Key ID: 1234abcd-12ab-34cd-56ef-1234567890ab
*
*
* -
*
* Key ARN: arn:aws:kms:us-east-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab
*
*
*
*
* To get the key ID and key ARN for a KMS key, use ListKeys or DescribeKey.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder keyId(String keyId);
/**
*
* The identity that gets the permissions specified in the grant.
*
*
* To specify the grantee principal, use the Amazon Resource Name (ARN) of an Amazon Web Services principal.
* Valid principals include Amazon Web Services accounts, IAM users, IAM roles, federated users, and assumed
* role users. For help with the ARN syntax for a principal, see IAM
* ARNs in the Identity and Access Management User Guide .
*
*
* @param granteePrincipal
* The identity that gets the permissions specified in the grant.
*
* To specify the grantee principal, use the Amazon Resource Name (ARN) of an Amazon Web Services
* principal. Valid principals include Amazon Web Services accounts, IAM users, IAM roles, federated
* users, and assumed role users. For help with the ARN syntax for a principal, see IAM ARNs in the Identity and Access Management User Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder granteePrincipal(String granteePrincipal);
/**
*
* The principal that has permission to use the RetireGrant operation to retire the grant.
*
*
* To specify the principal, use the Amazon Resource Name
* (ARN) of an Amazon Web Services principal. Valid principals include Amazon Web Services accounts, IAM
* users, IAM roles, federated users, and assumed role users. For help with the ARN syntax for a principal, see
* IAM
* ARNs in the Identity and Access Management User Guide .
*
*
* The grant determines the retiring principal. Other principals might have permission to retire the grant or
* revoke the grant. For details, see RevokeGrant and Retiring and
* revoking grants in the Key Management Service Developer Guide.
*
*
* @param retiringPrincipal
* The principal that has permission to use the RetireGrant operation to retire the grant.
*
* To specify the principal, use the Amazon Resource Name
* (ARN) of an Amazon Web Services principal. Valid principals include Amazon Web Services accounts,
* IAM users, IAM roles, federated users, and assumed role users. For help with the ARN syntax for a
* principal, see IAM ARNs in the Identity and Access Management User Guide .
*
*
* The grant determines the retiring principal. Other principals might have permission to retire the
* grant or revoke the grant. For details, see RevokeGrant and Retiring
* and revoking grants in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder retiringPrincipal(String retiringPrincipal);
/**
*
* A list of operations that the grant permits.
*
*
* This list must include only operations that are permitted in a grant. Also, the operation must be supported
* on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that allows the
* Sign operation, or a grant for an asymmetric KMS key that allows the GenerateDataKey operation.
* If you try, KMS returns a ValidationError
exception. For details, see Grant
* operations in the Key Management Service Developer Guide.
*
*
* @param operations
* A list of operations that the grant permits.
*
* This list must include only operations that are permitted in a grant. Also, the operation must be
* supported on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key
* that allows the Sign operation, or a grant for an asymmetric KMS key that allows the
* GenerateDataKey operation. If you try, KMS returns a ValidationError
exception.
* For details, see Grant
* operations in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operationsWithStrings(Collection operations);
/**
*
* A list of operations that the grant permits.
*
*
* This list must include only operations that are permitted in a grant. Also, the operation must be supported
* on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that allows the
* Sign operation, or a grant for an asymmetric KMS key that allows the GenerateDataKey operation.
* If you try, KMS returns a ValidationError
exception. For details, see Grant
* operations in the Key Management Service Developer Guide.
*
*
* @param operations
* A list of operations that the grant permits.
*
* This list must include only operations that are permitted in a grant. Also, the operation must be
* supported on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key
* that allows the Sign operation, or a grant for an asymmetric KMS key that allows the
* GenerateDataKey operation. If you try, KMS returns a ValidationError
exception.
* For details, see Grant
* operations in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operationsWithStrings(String... operations);
/**
*
* A list of operations that the grant permits.
*
*
* This list must include only operations that are permitted in a grant. Also, the operation must be supported
* on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that allows the
* Sign operation, or a grant for an asymmetric KMS key that allows the GenerateDataKey operation.
* If you try, KMS returns a ValidationError
exception. For details, see Grant
* operations in the Key Management Service Developer Guide.
*
*
* @param operations
* A list of operations that the grant permits.
*
* This list must include only operations that are permitted in a grant. Also, the operation must be
* supported on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key
* that allows the Sign operation, or a grant for an asymmetric KMS key that allows the
* GenerateDataKey operation. If you try, KMS returns a ValidationError
exception.
* For details, see Grant
* operations in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operations(Collection operations);
/**
*
* A list of operations that the grant permits.
*
*
* This list must include only operations that are permitted in a grant. Also, the operation must be supported
* on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key that allows the
* Sign operation, or a grant for an asymmetric KMS key that allows the GenerateDataKey operation.
* If you try, KMS returns a ValidationError
exception. For details, see Grant
* operations in the Key Management Service Developer Guide.
*
*
* @param operations
* A list of operations that the grant permits.
*
* This list must include only operations that are permitted in a grant. Also, the operation must be
* supported on the KMS key. For example, you cannot create a grant for a symmetric encryption KMS key
* that allows the Sign operation, or a grant for an asymmetric KMS key that allows the
* GenerateDataKey operation. If you try, KMS returns a ValidationError
exception.
* For details, see Grant
* operations in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder operations(GrantOperation... operations);
/**
*
* Specifies a grant constraint.
*
*
*
* Do not include confidential or sensitive information in this field. This field may be displayed in plaintext
* in CloudTrail logs and other output.
*
*
*
* KMS supports the EncryptionContextEquals
and EncryptionContextSubset
grant
* constraints, which allow the permissions in the grant only when the encryption context in the request matches
* (EncryptionContextEquals
) or includes (EncryptionContextSubset
) the encryption
* context specified in the constraint.
*
*
* The encryption context grant constraints are supported only on grant
* operations that include an EncryptionContext
parameter, such as cryptographic operations on
* symmetric encryption KMS keys. Grants with grant constraints can include the DescribeKey and
* RetireGrant operations, but the constraint doesn't apply to these operations. If a grant with a grant
* constraint includes the CreateGrant
operation, the constraint requires that any grants created
* with the CreateGrant
permission have an equally strict or stricter encryption context
* constraint.
*
*
* You cannot use an encryption context grant constraint for cryptographic operations with asymmetric KMS keys
* or HMAC KMS keys. Operations with these keys don't support an encryption context.
*
*
* Each constraint value can include up to 8 encryption context pairs. The encryption context value in each
* constraint cannot exceed 384 characters. For information about grant constraints, see Using grant constraints in the Key Management Service Developer Guide. For more information about
* encryption context, see Encryption
* context in the Key Management Service Developer Guide .
*
*
* @param constraints
* Specifies a grant constraint.
*
* Do not include confidential or sensitive information in this field. This field may be displayed in
* plaintext in CloudTrail logs and other output.
*
*
*
* KMS supports the EncryptionContextEquals
and EncryptionContextSubset
grant
* constraints, which allow the permissions in the grant only when the encryption context in the request
* matches (EncryptionContextEquals
) or includes (EncryptionContextSubset
) the
* encryption context specified in the constraint.
*
*
* The encryption context grant constraints are supported only on grant
* operations that include an EncryptionContext
parameter, such as cryptographic
* operations on symmetric encryption KMS keys. Grants with grant constraints can include the
* DescribeKey and RetireGrant operations, but the constraint doesn't apply to these
* operations. If a grant with a grant constraint includes the CreateGrant
operation, the
* constraint requires that any grants created with the CreateGrant
permission have an
* equally strict or stricter encryption context constraint.
*
*
* You cannot use an encryption context grant constraint for cryptographic operations with asymmetric KMS
* keys or HMAC KMS keys. Operations with these keys don't support an encryption context.
*
*
* Each constraint value can include up to 8 encryption context pairs. The encryption context value in
* each constraint cannot exceed 384 characters. For information about grant constraints, see Using grant constraints in the Key Management Service Developer Guide. For more
* information about encryption context, see Encryption
* context in the Key Management Service Developer Guide .
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder constraints(GrantConstraints constraints);
/**
*
* Specifies a grant constraint.
*
*
*
* Do not include confidential or sensitive information in this field. This field may be displayed in plaintext
* in CloudTrail logs and other output.
*
*
*
* KMS supports the EncryptionContextEquals
and EncryptionContextSubset
grant
* constraints, which allow the permissions in the grant only when the encryption context in the request matches
* (EncryptionContextEquals
) or includes (EncryptionContextSubset
) the encryption
* context specified in the constraint.
*
*
* The encryption context grant constraints are supported only on grant
* operations that include an EncryptionContext
parameter, such as cryptographic operations on
* symmetric encryption KMS keys. Grants with grant constraints can include the DescribeKey and
* RetireGrant operations, but the constraint doesn't apply to these operations. If a grant with a grant
* constraint includes the CreateGrant
operation, the constraint requires that any grants created
* with the CreateGrant
permission have an equally strict or stricter encryption context
* constraint.
*
*
* You cannot use an encryption context grant constraint for cryptographic operations with asymmetric KMS keys
* or HMAC KMS keys. Operations with these keys don't support an encryption context.
*
*
* Each constraint value can include up to 8 encryption context pairs. The encryption context value in each
* constraint cannot exceed 384 characters. For information about grant constraints, see Using grant constraints in the Key Management Service Developer Guide. For more information about
* encryption context, see Encryption
* context in the Key Management Service Developer Guide .
*
* This is a convenience method that creates an instance of the {@link GrantConstraints.Builder} avoiding the
* need to create one manually via {@link GrantConstraints#builder()}.
*
*
* When the {@link Consumer} completes, {@link GrantConstraints.Builder#build()} is called immediately and its
* result is passed to {@link #constraints(GrantConstraints)}.
*
* @param constraints
* a consumer that will call methods on {@link GrantConstraints.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #constraints(GrantConstraints)
*/
default Builder constraints(Consumer constraints) {
return constraints(GrantConstraints.builder().applyMutation(constraints).build());
}
/**
*
* A list of grant tokens.
*
*
* Use a grant token when your permission to call this operation comes from a new grant that has not yet
* achieved eventual consistency. For more information, see Grant token and Using a
* grant token in the Key Management Service Developer Guide.
*
*
* @param grantTokens
* A list of grant tokens.
*
* Use a grant token when your permission to call this operation comes from a new grant that has not yet
* achieved eventual consistency. For more information, see Grant token
* and Using
* a grant token in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder grantTokens(Collection grantTokens);
/**
*
* A list of grant tokens.
*
*
* Use a grant token when your permission to call this operation comes from a new grant that has not yet
* achieved eventual consistency. For more information, see Grant token and Using a
* grant token in the Key Management Service Developer Guide.
*
*
* @param grantTokens
* A list of grant tokens.
*
* Use a grant token when your permission to call this operation comes from a new grant that has not yet
* achieved eventual consistency. For more information, see Grant token
* and Using
* a grant token in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder grantTokens(String... grantTokens);
/**
*
* A friendly name for the grant. Use this value to prevent the unintended creation of duplicate grants when
* retrying this request.
*
*
*
* Do not include confidential or sensitive information in this field. This field may be displayed in plaintext
* in CloudTrail logs and other output.
*
*
*
* When this value is absent, all CreateGrant
requests result in a new grant with a unique
* GrantId
even if all the supplied parameters are identical. This can result in unintended
* duplicates when you retry the CreateGrant
request.
*
*
* When this value is present, you can retry a CreateGrant
request with identical parameters; if
* the grant already exists, the original GrantId
is returned without creating a new grant. Note
* that the returned grant token is unique with every CreateGrant
request, even when a duplicate
* GrantId
is returned. All grant tokens for the same grant ID can be used interchangeably.
*
*
* @param name
* A friendly name for the grant. Use this value to prevent the unintended creation of duplicate grants
* when retrying this request.
*
* Do not include confidential or sensitive information in this field. This field may be displayed in
* plaintext in CloudTrail logs and other output.
*
*
*
* When this value is absent, all CreateGrant
requests result in a new grant with a unique
* GrantId
even if all the supplied parameters are identical. This can result in unintended
* duplicates when you retry the CreateGrant
request.
*
*
* When this value is present, you can retry a CreateGrant
request with identical
* parameters; if the grant already exists, the original GrantId
is returned without
* creating a new grant. Note that the returned grant token is unique with every CreateGrant
* request, even when a duplicate GrantId
is returned. All grant tokens for the same grant
* ID can be used interchangeably.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder name(String name);
/**
*
* Checks if your request will succeed. DryRun
is an optional parameter.
*
*
* To learn more about how to use this parameter, see Testing your KMS API
* calls in the Key Management Service Developer Guide.
*
*
* @param dryRun
* Checks if your request will succeed. DryRun
is an optional parameter.
*
* To learn more about how to use this parameter, see Testing your KMS
* API calls in the Key Management Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder dryRun(Boolean dryRun);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends KmsRequest.BuilderImpl implements Builder {
private String keyId;
private String granteePrincipal;
private String retiringPrincipal;
private List operations = DefaultSdkAutoConstructList.getInstance();
private GrantConstraints constraints;
private List grantTokens = DefaultSdkAutoConstructList.getInstance();
private String name;
private Boolean dryRun;
private BuilderImpl() {
}
private BuilderImpl(CreateGrantRequest model) {
super(model);
keyId(model.keyId);
granteePrincipal(model.granteePrincipal);
retiringPrincipal(model.retiringPrincipal);
operationsWithStrings(model.operations);
constraints(model.constraints);
grantTokens(model.grantTokens);
name(model.name);
dryRun(model.dryRun);
}
public final String getKeyId() {
return keyId;
}
public final void setKeyId(String keyId) {
this.keyId = keyId;
}
@Override
public final Builder keyId(String keyId) {
this.keyId = keyId;
return this;
}
public final String getGranteePrincipal() {
return granteePrincipal;
}
public final void setGranteePrincipal(String granteePrincipal) {
this.granteePrincipal = granteePrincipal;
}
@Override
public final Builder granteePrincipal(String granteePrincipal) {
this.granteePrincipal = granteePrincipal;
return this;
}
public final String getRetiringPrincipal() {
return retiringPrincipal;
}
public final void setRetiringPrincipal(String retiringPrincipal) {
this.retiringPrincipal = retiringPrincipal;
}
@Override
public final Builder retiringPrincipal(String retiringPrincipal) {
this.retiringPrincipal = retiringPrincipal;
return this;
}
public final Collection getOperations() {
if (operations instanceof SdkAutoConstructList) {
return null;
}
return operations;
}
public final void setOperations(Collection operations) {
this.operations = GrantOperationListCopier.copy(operations);
}
@Override
public final Builder operationsWithStrings(Collection operations) {
this.operations = GrantOperationListCopier.copy(operations);
return this;
}
@Override
@SafeVarargs
public final Builder operationsWithStrings(String... operations) {
operationsWithStrings(Arrays.asList(operations));
return this;
}
@Override
public final Builder operations(Collection operations) {
this.operations = GrantOperationListCopier.copyEnumToString(operations);
return this;
}
@Override
@SafeVarargs
public final Builder operations(GrantOperation... operations) {
operations(Arrays.asList(operations));
return this;
}
public final GrantConstraints.Builder getConstraints() {
return constraints != null ? constraints.toBuilder() : null;
}
public final void setConstraints(GrantConstraints.BuilderImpl constraints) {
this.constraints = constraints != null ? constraints.build() : null;
}
@Override
public final Builder constraints(GrantConstraints constraints) {
this.constraints = constraints;
return this;
}
public final Collection getGrantTokens() {
if (grantTokens instanceof SdkAutoConstructList) {
return null;
}
return grantTokens;
}
public final void setGrantTokens(Collection grantTokens) {
this.grantTokens = GrantTokenListCopier.copy(grantTokens);
}
@Override
public final Builder grantTokens(Collection grantTokens) {
this.grantTokens = GrantTokenListCopier.copy(grantTokens);
return this;
}
@Override
@SafeVarargs
public final Builder grantTokens(String... grantTokens) {
grantTokens(Arrays.asList(grantTokens));
return this;
}
public final String getName() {
return name;
}
public final void setName(String name) {
this.name = name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final Boolean getDryRun() {
return dryRun;
}
public final void setDryRun(Boolean dryRun) {
this.dryRun = dryRun;
}
@Override
public final Builder dryRun(Boolean dryRun) {
this.dryRun = dryRun;
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CreateGrantRequest build() {
return new CreateGrantRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}