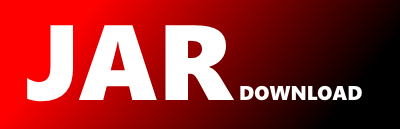
software.amazon.awssdk.services.kms.model.KeyMetadata Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.kms.model;
import java.io.Serializable;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains metadata about a customer master key (CMK).
*
*
* This data type is used as a response element for the CreateKey and DescribeKey operations.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class KeyMetadata implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField AWS_ACCOUNT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::awsAccountId)).setter(setter(Builder::awsAccountId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("AWSAccountId").build()).build();
private static final SdkField KEY_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::keyId)).setter(setter(Builder::keyId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyId").build()).build();
private static final SdkField ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::arn)).setter(setter(Builder::arn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Arn").build()).build();
private static final SdkField CREATION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(KeyMetadata::creationDate)).setter(setter(Builder::creationDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CreationDate").build()).build();
private static final SdkField ENABLED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(KeyMetadata::enabled)).setter(setter(Builder::enabled))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Enabled").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Description").build()).build();
private static final SdkField KEY_USAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::keyUsageAsString)).setter(setter(Builder::keyUsage))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyUsage").build()).build();
private static final SdkField KEY_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::keyStateAsString)).setter(setter(Builder::keyState))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyState").build()).build();
private static final SdkField DELETION_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(KeyMetadata::deletionDate)).setter(setter(Builder::deletionDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeletionDate").build()).build();
private static final SdkField VALID_TO_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(KeyMetadata::validTo)).setter(setter(Builder::validTo))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ValidTo").build()).build();
private static final SdkField ORIGIN_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::originAsString)).setter(setter(Builder::origin))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Origin").build()).build();
private static final SdkField CUSTOM_KEY_STORE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::customKeyStoreId)).setter(setter(Builder::customKeyStoreId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CustomKeyStoreId").build()).build();
private static final SdkField CLOUD_HSM_CLUSTER_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::cloudHsmClusterId)).setter(setter(Builder::cloudHsmClusterId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CloudHsmClusterId").build()).build();
private static final SdkField EXPIRATION_MODEL_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::expirationModelAsString)).setter(setter(Builder::expirationModel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ExpirationModel").build()).build();
private static final SdkField KEY_MANAGER_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(KeyMetadata::keyManagerAsString)).setter(setter(Builder::keyManager))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("KeyManager").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(AWS_ACCOUNT_ID_FIELD,
KEY_ID_FIELD, ARN_FIELD, CREATION_DATE_FIELD, ENABLED_FIELD, DESCRIPTION_FIELD, KEY_USAGE_FIELD, KEY_STATE_FIELD,
DELETION_DATE_FIELD, VALID_TO_FIELD, ORIGIN_FIELD, CUSTOM_KEY_STORE_ID_FIELD, CLOUD_HSM_CLUSTER_ID_FIELD,
EXPIRATION_MODEL_FIELD, KEY_MANAGER_FIELD));
private static final long serialVersionUID = 1L;
private final String awsAccountId;
private final String keyId;
private final String arn;
private final Instant creationDate;
private final Boolean enabled;
private final String description;
private final String keyUsage;
private final String keyState;
private final Instant deletionDate;
private final Instant validTo;
private final String origin;
private final String customKeyStoreId;
private final String cloudHsmClusterId;
private final String expirationModel;
private final String keyManager;
private KeyMetadata(BuilderImpl builder) {
this.awsAccountId = builder.awsAccountId;
this.keyId = builder.keyId;
this.arn = builder.arn;
this.creationDate = builder.creationDate;
this.enabled = builder.enabled;
this.description = builder.description;
this.keyUsage = builder.keyUsage;
this.keyState = builder.keyState;
this.deletionDate = builder.deletionDate;
this.validTo = builder.validTo;
this.origin = builder.origin;
this.customKeyStoreId = builder.customKeyStoreId;
this.cloudHsmClusterId = builder.cloudHsmClusterId;
this.expirationModel = builder.expirationModel;
this.keyManager = builder.keyManager;
}
/**
*
* The twelve-digit account ID of the AWS account that owns the CMK.
*
*
* @return The twelve-digit account ID of the AWS account that owns the CMK.
*/
public String awsAccountId() {
return awsAccountId;
}
/**
*
* The globally unique identifier for the CMK.
*
*
* @return The globally unique identifier for the CMK.
*/
public String keyId() {
return keyId;
}
/**
*
* The Amazon Resource Name (ARN) of the CMK. For examples, see AWS Key
* Management Service (AWS KMS) in the Example ARNs section of the AWS General Reference.
*
*
* @return The Amazon Resource Name (ARN) of the CMK. For examples, see AWS Key
* Management Service (AWS KMS) in the Example ARNs section of the AWS General Reference.
*/
public String arn() {
return arn;
}
/**
*
* The date and time when the CMK was created.
*
*
* @return The date and time when the CMK was created.
*/
public Instant creationDate() {
return creationDate;
}
/**
*
* Specifies whether the CMK is enabled. When KeyState
is Enabled
this value is true,
* otherwise it is false.
*
*
* @return Specifies whether the CMK is enabled. When KeyState
is Enabled
this value is
* true, otherwise it is false.
*/
public Boolean enabled() {
return enabled;
}
/**
*
* The description of the CMK.
*
*
* @return The description of the CMK.
*/
public String description() {
return description;
}
/**
*
* The cryptographic operations for which you can use the CMK. The only valid value is ENCRYPT_DECRYPT
,
* which means you can use the CMK to encrypt and decrypt data.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyUsage} will
* return {@link KeyUsageType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyUsageAsString}.
*
*
* @return The cryptographic operations for which you can use the CMK. The only valid value is
* ENCRYPT_DECRYPT
, which means you can use the CMK to encrypt and decrypt data.
* @see KeyUsageType
*/
public KeyUsageType keyUsage() {
return KeyUsageType.fromValue(keyUsage);
}
/**
*
* The cryptographic operations for which you can use the CMK. The only valid value is ENCRYPT_DECRYPT
,
* which means you can use the CMK to encrypt and decrypt data.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyUsage} will
* return {@link KeyUsageType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyUsageAsString}.
*
*
* @return The cryptographic operations for which you can use the CMK. The only valid value is
* ENCRYPT_DECRYPT
, which means you can use the CMK to encrypt and decrypt data.
* @see KeyUsageType
*/
public String keyUsageAsString() {
return keyUsage;
}
/**
*
* The state of the CMK.
*
*
* For more information about how key state affects the use of a CMK, see How Key State Affects the Use of a
* Customer Master Key in the AWS Key Management Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyState} will
* return {@link KeyState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyStateAsString}.
*
*
* @return The state of the CMK.
*
* For more information about how key state affects the use of a CMK, see How Key State Affects the Use
* of a Customer Master Key in the AWS Key Management Service Developer Guide.
* @see KeyState
*/
public KeyState keyState() {
return KeyState.fromValue(keyState);
}
/**
*
* The state of the CMK.
*
*
* For more information about how key state affects the use of a CMK, see How Key State Affects the Use of a
* Customer Master Key in the AWS Key Management Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyState} will
* return {@link KeyState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyStateAsString}.
*
*
* @return The state of the CMK.
*
* For more information about how key state affects the use of a CMK, see How Key State Affects the Use
* of a Customer Master Key in the AWS Key Management Service Developer Guide.
* @see KeyState
*/
public String keyStateAsString() {
return keyState;
}
/**
*
* The date and time after which AWS KMS deletes the CMK. This value is present only when KeyState
is
* PendingDeletion
.
*
*
* @return The date and time after which AWS KMS deletes the CMK. This value is present only when
* KeyState
is PendingDeletion
.
*/
public Instant deletionDate() {
return deletionDate;
}
/**
*
* The time at which the imported key material expires. When the key material expires, AWS KMS deletes the key
* material and the CMK becomes unusable. This value is present only for CMKs whose Origin
is
* EXTERNAL
and whose ExpirationModel
is KEY_MATERIAL_EXPIRES
, otherwise this
* value is omitted.
*
*
* @return The time at which the imported key material expires. When the key material expires, AWS KMS deletes the
* key material and the CMK becomes unusable. This value is present only for CMKs whose Origin
* is EXTERNAL
and whose ExpirationModel
is KEY_MATERIAL_EXPIRES
,
* otherwise this value is omitted.
*/
public Instant validTo() {
return validTo;
}
/**
*
* The source of the CMK's key material. When this value is AWS_KMS
, AWS KMS created the key material.
* When this value is EXTERNAL
, the key material was imported from your existing key management
* infrastructure or the CMK lacks key material. When this value is AWS_CLOUDHSM
, the key material was
* created in the AWS CloudHSM cluster associated with a custom key store.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #origin} will
* return {@link OriginType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #originAsString}.
*
*
* @return The source of the CMK's key material. When this value is AWS_KMS
, AWS KMS created the key
* material. When this value is EXTERNAL
, the key material was imported from your existing key
* management infrastructure or the CMK lacks key material. When this value is AWS_CLOUDHSM
,
* the key material was created in the AWS CloudHSM cluster associated with a custom key store.
* @see OriginType
*/
public OriginType origin() {
return OriginType.fromValue(origin);
}
/**
*
* The source of the CMK's key material. When this value is AWS_KMS
, AWS KMS created the key material.
* When this value is EXTERNAL
, the key material was imported from your existing key management
* infrastructure or the CMK lacks key material. When this value is AWS_CLOUDHSM
, the key material was
* created in the AWS CloudHSM cluster associated with a custom key store.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #origin} will
* return {@link OriginType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #originAsString}.
*
*
* @return The source of the CMK's key material. When this value is AWS_KMS
, AWS KMS created the key
* material. When this value is EXTERNAL
, the key material was imported from your existing key
* management infrastructure or the CMK lacks key material. When this value is AWS_CLOUDHSM
,
* the key material was created in the AWS CloudHSM cluster associated with a custom key store.
* @see OriginType
*/
public String originAsString() {
return origin;
}
/**
*
* A unique identifier for the custom key store
* that contains the CMK. This value is present only when the CMK is created in a custom key store.
*
*
* @return A unique identifier for the custom key
* store that contains the CMK. This value is present only when the CMK is created in a custom key
* store.
*/
public String customKeyStoreId() {
return customKeyStoreId;
}
/**
*
* The cluster ID of the AWS CloudHSM cluster that contains the key material for the CMK. When you create a CMK in a
* custom key
* store, AWS KMS creates the key material for the CMK in the associated AWS CloudHSM cluster. This value is
* present only when the CMK is created in a custom key store.
*
*
* @return The cluster ID of the AWS CloudHSM cluster that contains the key material for the CMK. When you create a
* CMK in a custom key
* store, AWS KMS creates the key material for the CMK in the associated AWS CloudHSM cluster. This
* value is present only when the CMK is created in a custom key store.
*/
public String cloudHsmClusterId() {
return cloudHsmClusterId;
}
/**
*
* Specifies whether the CMK's key material expires. This value is present only when Origin
is
* EXTERNAL
, otherwise this value is omitted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #expirationModel}
* will return {@link ExpirationModelType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #expirationModelAsString}.
*
*
* @return Specifies whether the CMK's key material expires. This value is present only when Origin
is
* EXTERNAL
, otherwise this value is omitted.
* @see ExpirationModelType
*/
public ExpirationModelType expirationModel() {
return ExpirationModelType.fromValue(expirationModel);
}
/**
*
* Specifies whether the CMK's key material expires. This value is present only when Origin
is
* EXTERNAL
, otherwise this value is omitted.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #expirationModel}
* will return {@link ExpirationModelType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is
* available from {@link #expirationModelAsString}.
*
*
* @return Specifies whether the CMK's key material expires. This value is present only when Origin
is
* EXTERNAL
, otherwise this value is omitted.
* @see ExpirationModelType
*/
public String expirationModelAsString() {
return expirationModel;
}
/**
*
* The manager of the CMK. CMKs in your AWS account are either customer managed or AWS managed. For more information
* about the difference, see Customer Master Keys
* in the AWS Key Management Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyManager} will
* return {@link KeyManagerType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyManagerAsString}.
*
*
* @return The manager of the CMK. CMKs in your AWS account are either customer managed or AWS managed. For more
* information about the difference, see Customer Master
* Keys in the AWS Key Management Service Developer Guide.
* @see KeyManagerType
*/
public KeyManagerType keyManager() {
return KeyManagerType.fromValue(keyManager);
}
/**
*
* The manager of the CMK. CMKs in your AWS account are either customer managed or AWS managed. For more information
* about the difference, see Customer Master Keys
* in the AWS Key Management Service Developer Guide.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #keyManager} will
* return {@link KeyManagerType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #keyManagerAsString}.
*
*
* @return The manager of the CMK. CMKs in your AWS account are either customer managed or AWS managed. For more
* information about the difference, see Customer Master
* Keys in the AWS Key Management Service Developer Guide.
* @see KeyManagerType
*/
public String keyManagerAsString() {
return keyManager;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(awsAccountId());
hashCode = 31 * hashCode + Objects.hashCode(keyId());
hashCode = 31 * hashCode + Objects.hashCode(arn());
hashCode = 31 * hashCode + Objects.hashCode(creationDate());
hashCode = 31 * hashCode + Objects.hashCode(enabled());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(keyUsageAsString());
hashCode = 31 * hashCode + Objects.hashCode(keyStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(deletionDate());
hashCode = 31 * hashCode + Objects.hashCode(validTo());
hashCode = 31 * hashCode + Objects.hashCode(originAsString());
hashCode = 31 * hashCode + Objects.hashCode(customKeyStoreId());
hashCode = 31 * hashCode + Objects.hashCode(cloudHsmClusterId());
hashCode = 31 * hashCode + Objects.hashCode(expirationModelAsString());
hashCode = 31 * hashCode + Objects.hashCode(keyManagerAsString());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof KeyMetadata)) {
return false;
}
KeyMetadata other = (KeyMetadata) obj;
return Objects.equals(awsAccountId(), other.awsAccountId()) && Objects.equals(keyId(), other.keyId())
&& Objects.equals(arn(), other.arn()) && Objects.equals(creationDate(), other.creationDate())
&& Objects.equals(enabled(), other.enabled()) && Objects.equals(description(), other.description())
&& Objects.equals(keyUsageAsString(), other.keyUsageAsString())
&& Objects.equals(keyStateAsString(), other.keyStateAsString())
&& Objects.equals(deletionDate(), other.deletionDate()) && Objects.equals(validTo(), other.validTo())
&& Objects.equals(originAsString(), other.originAsString())
&& Objects.equals(customKeyStoreId(), other.customKeyStoreId())
&& Objects.equals(cloudHsmClusterId(), other.cloudHsmClusterId())
&& Objects.equals(expirationModelAsString(), other.expirationModelAsString())
&& Objects.equals(keyManagerAsString(), other.keyManagerAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("KeyMetadata").add("AWSAccountId", awsAccountId()).add("KeyId", keyId()).add("Arn", arn())
.add("CreationDate", creationDate()).add("Enabled", enabled()).add("Description", description())
.add("KeyUsage", keyUsageAsString()).add("KeyState", keyStateAsString()).add("DeletionDate", deletionDate())
.add("ValidTo", validTo()).add("Origin", originAsString()).add("CustomKeyStoreId", customKeyStoreId())
.add("CloudHsmClusterId", cloudHsmClusterId()).add("ExpirationModel", expirationModelAsString())
.add("KeyManager", keyManagerAsString()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "AWSAccountId":
return Optional.ofNullable(clazz.cast(awsAccountId()));
case "KeyId":
return Optional.ofNullable(clazz.cast(keyId()));
case "Arn":
return Optional.ofNullable(clazz.cast(arn()));
case "CreationDate":
return Optional.ofNullable(clazz.cast(creationDate()));
case "Enabled":
return Optional.ofNullable(clazz.cast(enabled()));
case "Description":
return Optional.ofNullable(clazz.cast(description()));
case "KeyUsage":
return Optional.ofNullable(clazz.cast(keyUsageAsString()));
case "KeyState":
return Optional.ofNullable(clazz.cast(keyStateAsString()));
case "DeletionDate":
return Optional.ofNullable(clazz.cast(deletionDate()));
case "ValidTo":
return Optional.ofNullable(clazz.cast(validTo()));
case "Origin":
return Optional.ofNullable(clazz.cast(originAsString()));
case "CustomKeyStoreId":
return Optional.ofNullable(clazz.cast(customKeyStoreId()));
case "CloudHsmClusterId":
return Optional.ofNullable(clazz.cast(cloudHsmClusterId()));
case "ExpirationModel":
return Optional.ofNullable(clazz.cast(expirationModelAsString()));
case "KeyManager":
return Optional.ofNullable(clazz.cast(keyManagerAsString()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function