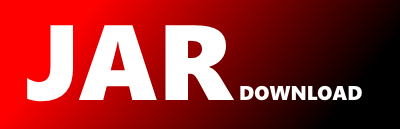
software.amazon.awssdk.services.lambda.model.ListLayerVersionsRequest Maven / Gradle / Ivy
Show all versions of lambda Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lambda.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class ListLayerVersionsRequest extends LambdaRequest implements
ToCopyableBuilder {
private static final SdkField COMPATIBLE_RUNTIME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CompatibleRuntime").getter(getter(ListLayerVersionsRequest::compatibleRuntimeAsString))
.setter(setter(Builder::compatibleRuntime))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("CompatibleRuntime").build())
.build();
private static final SdkField LAYER_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LayerName").getter(getter(ListLayerVersionsRequest::layerName)).setter(setter(Builder::layerName))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("LayerName").build()).build();
private static final SdkField MARKER_FIELD = SdkField. builder(MarshallingType.STRING).memberName("Marker")
.getter(getter(ListLayerVersionsRequest::marker)).setter(setter(Builder::marker))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("Marker").build()).build();
private static final SdkField MAX_ITEMS_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("MaxItems").getter(getter(ListLayerVersionsRequest::maxItems)).setter(setter(Builder::maxItems))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("MaxItems").build()).build();
private static final SdkField COMPATIBLE_ARCHITECTURE_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("CompatibleArchitecture")
.getter(getter(ListLayerVersionsRequest::compatibleArchitectureAsString))
.setter(setter(Builder::compatibleArchitecture))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("CompatibleArchitecture").build())
.build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(COMPATIBLE_RUNTIME_FIELD,
LAYER_NAME_FIELD, MARKER_FIELD, MAX_ITEMS_FIELD, COMPATIBLE_ARCHITECTURE_FIELD));
private final String compatibleRuntime;
private final String layerName;
private final String marker;
private final Integer maxItems;
private final String compatibleArchitecture;
private ListLayerVersionsRequest(BuilderImpl builder) {
super(builder);
this.compatibleRuntime = builder.compatibleRuntime;
this.layerName = builder.layerName;
this.marker = builder.marker;
this.maxItems = builder.maxItems;
this.compatibleArchitecture = builder.compatibleArchitecture;
}
/**
*
* A runtime identifier.
*
*
* The following list includes deprecated runtimes. For more information, see Runtime use
* after deprecation.
*
*
* For a list of all currently supported runtimes, see Supported
* runtimes.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compatibleRuntime}
* will return {@link Runtime#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compatibleRuntimeAsString}.
*
*
* @return A runtime identifier.
*
* The following list includes deprecated runtimes. For more information, see Runtime use after deprecation.
*
*
* For a list of all currently supported runtimes, see Supported
* runtimes.
* @see Runtime
*/
public final Runtime compatibleRuntime() {
return Runtime.fromValue(compatibleRuntime);
}
/**
*
* A runtime identifier.
*
*
* The following list includes deprecated runtimes. For more information, see Runtime use
* after deprecation.
*
*
* For a list of all currently supported runtimes, see Supported
* runtimes.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #compatibleRuntime}
* will return {@link Runtime#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #compatibleRuntimeAsString}.
*
*
* @return A runtime identifier.
*
* The following list includes deprecated runtimes. For more information, see Runtime use after deprecation.
*
*
* For a list of all currently supported runtimes, see Supported
* runtimes.
* @see Runtime
*/
public final String compatibleRuntimeAsString() {
return compatibleRuntime;
}
/**
*
* The name or Amazon Resource Name (ARN) of the layer.
*
*
* @return The name or Amazon Resource Name (ARN) of the layer.
*/
public final String layerName() {
return layerName;
}
/**
*
* A pagination token returned by a previous call.
*
*
* @return A pagination token returned by a previous call.
*/
public final String marker() {
return marker;
}
/**
*
* The maximum number of versions to return.
*
*
* @return The maximum number of versions to return.
*/
public final Integer maxItems() {
return maxItems;
}
/**
*
* The compatible instruction set
* architecture.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compatibleArchitecture} will return {@link Architecture#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #compatibleArchitectureAsString}.
*
*
* @return The compatible instruction
* set architecture.
* @see Architecture
*/
public final Architecture compatibleArchitecture() {
return Architecture.fromValue(compatibleArchitecture);
}
/**
*
* The compatible instruction set
* architecture.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #compatibleArchitecture} will return {@link Architecture#UNKNOWN_TO_SDK_VERSION}. The raw value returned
* by the service is available from {@link #compatibleArchitectureAsString}.
*
*
* @return The compatible instruction
* set architecture.
* @see Architecture
*/
public final String compatibleArchitectureAsString() {
return compatibleArchitecture;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(compatibleRuntimeAsString());
hashCode = 31 * hashCode + Objects.hashCode(layerName());
hashCode = 31 * hashCode + Objects.hashCode(marker());
hashCode = 31 * hashCode + Objects.hashCode(maxItems());
hashCode = 31 * hashCode + Objects.hashCode(compatibleArchitectureAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof ListLayerVersionsRequest)) {
return false;
}
ListLayerVersionsRequest other = (ListLayerVersionsRequest) obj;
return Objects.equals(compatibleRuntimeAsString(), other.compatibleRuntimeAsString())
&& Objects.equals(layerName(), other.layerName()) && Objects.equals(marker(), other.marker())
&& Objects.equals(maxItems(), other.maxItems())
&& Objects.equals(compatibleArchitectureAsString(), other.compatibleArchitectureAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("ListLayerVersionsRequest").add("CompatibleRuntime", compatibleRuntimeAsString())
.add("LayerName", layerName()).add("Marker", marker()).add("MaxItems", maxItems())
.add("CompatibleArchitecture", compatibleArchitectureAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CompatibleRuntime":
return Optional.ofNullable(clazz.cast(compatibleRuntimeAsString()));
case "LayerName":
return Optional.ofNullable(clazz.cast(layerName()));
case "Marker":
return Optional.ofNullable(clazz.cast(marker()));
case "MaxItems":
return Optional.ofNullable(clazz.cast(maxItems()));
case "CompatibleArchitecture":
return Optional.ofNullable(clazz.cast(compatibleArchitectureAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function