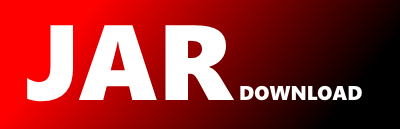
software.amazon.awssdk.services.lambda.model.PutRuntimeManagementConfigRequest Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lambda.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutRuntimeManagementConfigRequest extends LambdaRequest implements
ToCopyableBuilder {
private static final SdkField FUNCTION_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("FunctionName").getter(getter(PutRuntimeManagementConfigRequest::functionName))
.setter(setter(Builder::functionName))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("FunctionName").build()).build();
private static final SdkField QUALIFIER_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("Qualifier").getter(getter(PutRuntimeManagementConfigRequest::qualifier))
.setter(setter(Builder::qualifier))
.traits(LocationTrait.builder().location(MarshallLocation.QUERY_PARAM).locationName("Qualifier").build()).build();
private static final SdkField UPDATE_RUNTIME_ON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("UpdateRuntimeOn").getter(getter(PutRuntimeManagementConfigRequest::updateRuntimeOnAsString))
.setter(setter(Builder::updateRuntimeOn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("UpdateRuntimeOn").build()).build();
private static final SdkField RUNTIME_VERSION_ARN_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("RuntimeVersionArn").getter(getter(PutRuntimeManagementConfigRequest::runtimeVersionArn))
.setter(setter(Builder::runtimeVersionArn))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RuntimeVersionArn").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(FUNCTION_NAME_FIELD,
QUALIFIER_FIELD, UPDATE_RUNTIME_ON_FIELD, RUNTIME_VERSION_ARN_FIELD));
private final String functionName;
private final String qualifier;
private final String updateRuntimeOn;
private final String runtimeVersionArn;
private PutRuntimeManagementConfigRequest(BuilderImpl builder) {
super(builder);
this.functionName = builder.functionName;
this.qualifier = builder.qualifier;
this.updateRuntimeOn = builder.updateRuntimeOn;
this.runtimeVersionArn = builder.runtimeVersionArn;
}
/**
*
* The name or ARN of the Lambda function.
*
*
* Name formats
*
*
* -
*
* Function name – my-function
.
*
*
* -
*
* Function ARN – arn:aws:lambda:us-west-2:123456789012:function:my-function
.
*
*
* -
*
* Partial ARN – 123456789012:function:my-function
.
*
*
*
*
* The length constraint applies only to the full ARN. If you specify only the function name, it is limited to 64
* characters in length.
*
*
* @return The name or ARN of the Lambda function.
*
* Name formats
*
*
* -
*
* Function name – my-function
.
*
*
* -
*
* Function ARN – arn:aws:lambda:us-west-2:123456789012:function:my-function
.
*
*
* -
*
* Partial ARN – 123456789012:function:my-function
.
*
*
*
*
* The length constraint applies only to the full ARN. If you specify only the function name, it is limited
* to 64 characters in length.
*/
public final String functionName() {
return functionName;
}
/**
*
* Specify a version of the function. This can be $LATEST
or a published version number. If no value is
* specified, the configuration for the $LATEST
version is returned.
*
*
* @return Specify a version of the function. This can be $LATEST
or a published version number. If no
* value is specified, the configuration for the $LATEST
version is returned.
*/
public final String qualifier() {
return qualifier;
}
/**
*
* Specify the runtime update mode.
*
*
* -
*
* Auto (default) - Automatically update to the most recent and secure runtime version using a Two-phase
* runtime version rollout. This is the best choice for most customers to ensure they always benefit from
* runtime updates.
*
*
* -
*
* Function update - Lambda updates the runtime of your function to the most recent and secure runtime
* version when you update your function. This approach synchronizes runtime updates with function deployments,
* giving you control over when runtime updates are applied and allowing you to detect and mitigate rare runtime
* update incompatibilities early. When using this setting, you need to regularly update your functions to keep
* their runtime up-to-date.
*
*
* -
*
* Manual - You specify a runtime version in your function configuration. The function will use this runtime
* version indefinitely. In the rare case where a new runtime version is incompatible with an existing function,
* this allows you to roll back your function to an earlier runtime version. For more information, see Roll back a
* runtime version.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #updateRuntimeOn}
* will return {@link UpdateRuntimeOn#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #updateRuntimeOnAsString}.
*
*
* @return Specify the runtime update mode.
*
* -
*
* Auto (default) - Automatically update to the most recent and secure runtime version using a Two-
* phase runtime version rollout. This is the best choice for most customers to ensure they always
* benefit from runtime updates.
*
*
* -
*
* Function update - Lambda updates the runtime of your function to the most recent and secure
* runtime version when you update your function. This approach synchronizes runtime updates with function
* deployments, giving you control over when runtime updates are applied and allowing you to detect and
* mitigate rare runtime update incompatibilities early. When using this setting, you need to regularly
* update your functions to keep their runtime up-to-date.
*
*
* -
*
* Manual - You specify a runtime version in your function configuration. The function will use this
* runtime version indefinitely. In the rare case where a new runtime version is incompatible with an
* existing function, this allows you to roll back your function to an earlier runtime version. For more
* information, see Roll
* back a runtime version.
*
*
* @see UpdateRuntimeOn
*/
public final UpdateRuntimeOn updateRuntimeOn() {
return UpdateRuntimeOn.fromValue(updateRuntimeOn);
}
/**
*
* Specify the runtime update mode.
*
*
* -
*
* Auto (default) - Automatically update to the most recent and secure runtime version using a Two-phase
* runtime version rollout. This is the best choice for most customers to ensure they always benefit from
* runtime updates.
*
*
* -
*
* Function update - Lambda updates the runtime of your function to the most recent and secure runtime
* version when you update your function. This approach synchronizes runtime updates with function deployments,
* giving you control over when runtime updates are applied and allowing you to detect and mitigate rare runtime
* update incompatibilities early. When using this setting, you need to regularly update your functions to keep
* their runtime up-to-date.
*
*
* -
*
* Manual - You specify a runtime version in your function configuration. The function will use this runtime
* version indefinitely. In the rare case where a new runtime version is incompatible with an existing function,
* this allows you to roll back your function to an earlier runtime version. For more information, see Roll back a
* runtime version.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #updateRuntimeOn}
* will return {@link UpdateRuntimeOn#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #updateRuntimeOnAsString}.
*
*
* @return Specify the runtime update mode.
*
* -
*
* Auto (default) - Automatically update to the most recent and secure runtime version using a Two-
* phase runtime version rollout. This is the best choice for most customers to ensure they always
* benefit from runtime updates.
*
*
* -
*
* Function update - Lambda updates the runtime of your function to the most recent and secure
* runtime version when you update your function. This approach synchronizes runtime updates with function
* deployments, giving you control over when runtime updates are applied and allowing you to detect and
* mitigate rare runtime update incompatibilities early. When using this setting, you need to regularly
* update your functions to keep their runtime up-to-date.
*
*
* -
*
* Manual - You specify a runtime version in your function configuration. The function will use this
* runtime version indefinitely. In the rare case where a new runtime version is incompatible with an
* existing function, this allows you to roll back your function to an earlier runtime version. For more
* information, see Roll
* back a runtime version.
*
*
* @see UpdateRuntimeOn
*/
public final String updateRuntimeOnAsString() {
return updateRuntimeOn;
}
/**
*
* The ARN of the runtime version you want the function to use.
*
*
*
* This is only required if you're using the Manual runtime update mode.
*
*
*
* @return The ARN of the runtime version you want the function to use.
*
* This is only required if you're using the Manual runtime update mode.
*
*/
public final String runtimeVersionArn() {
return runtimeVersionArn;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(functionName());
hashCode = 31 * hashCode + Objects.hashCode(qualifier());
hashCode = 31 * hashCode + Objects.hashCode(updateRuntimeOnAsString());
hashCode = 31 * hashCode + Objects.hashCode(runtimeVersionArn());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutRuntimeManagementConfigRequest)) {
return false;
}
PutRuntimeManagementConfigRequest other = (PutRuntimeManagementConfigRequest) obj;
return Objects.equals(functionName(), other.functionName()) && Objects.equals(qualifier(), other.qualifier())
&& Objects.equals(updateRuntimeOnAsString(), other.updateRuntimeOnAsString())
&& Objects.equals(runtimeVersionArn(), other.runtimeVersionArn());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutRuntimeManagementConfigRequest").add("FunctionName", functionName())
.add("Qualifier", qualifier()).add("UpdateRuntimeOn", updateRuntimeOnAsString())
.add("RuntimeVersionArn", runtimeVersionArn()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "FunctionName":
return Optional.ofNullable(clazz.cast(functionName()));
case "Qualifier":
return Optional.ofNullable(clazz.cast(qualifier()));
case "UpdateRuntimeOn":
return Optional.ofNullable(clazz.cast(updateRuntimeOnAsString()));
case "RuntimeVersionArn":
return Optional.ofNullable(clazz.cast(runtimeVersionArn()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function