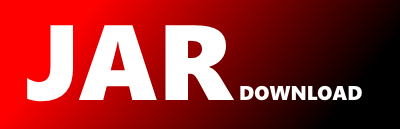
software.amazon.awssdk.services.lambda.model.LoggingConfig Maven / Gradle / Ivy
Show all versions of lambda Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lambda.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* The function's Amazon CloudWatch Logs configuration settings.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class LoggingConfig implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField LOG_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogFormat").getter(getter(LoggingConfig::logFormatAsString)).setter(setter(Builder::logFormat))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogFormat").build()).build();
private static final SdkField APPLICATION_LOG_LEVEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("ApplicationLogLevel").getter(getter(LoggingConfig::applicationLogLevelAsString))
.setter(setter(Builder::applicationLogLevel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ApplicationLogLevel").build())
.build();
private static final SdkField SYSTEM_LOG_LEVEL_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("SystemLogLevel").getter(getter(LoggingConfig::systemLogLevelAsString))
.setter(setter(Builder::systemLogLevel))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("SystemLogLevel").build()).build();
private static final SdkField LOG_GROUP_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("LogGroup").getter(getter(LoggingConfig::logGroup)).setter(setter(Builder::logGroup))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("LogGroup").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(LOG_FORMAT_FIELD,
APPLICATION_LOG_LEVEL_FIELD, SYSTEM_LOG_LEVEL_FIELD, LOG_GROUP_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("LogFormat", LOG_FORMAT_FIELD);
put("ApplicationLogLevel", APPLICATION_LOG_LEVEL_FIELD);
put("SystemLogLevel", SYSTEM_LOG_LEVEL_FIELD);
put("LogGroup", LOG_GROUP_FIELD);
}
});
private static final long serialVersionUID = 1L;
private final String logFormat;
private final String applicationLogLevel;
private final String systemLogLevel;
private final String logGroup;
private LoggingConfig(BuilderImpl builder) {
this.logFormat = builder.logFormat;
this.applicationLogLevel = builder.applicationLogLevel;
this.systemLogLevel = builder.systemLogLevel;
this.logGroup = builder.logGroup;
}
/**
*
* The format in which Lambda sends your function's application and system logs to CloudWatch. Select between plain
* text and structured JSON.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #logFormat} will
* return {@link LogFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #logFormatAsString}.
*
*
* @return The format in which Lambda sends your function's application and system logs to CloudWatch. Select
* between plain text and structured JSON.
* @see LogFormat
*/
public final LogFormat logFormat() {
return LogFormat.fromValue(logFormat);
}
/**
*
* The format in which Lambda sends your function's application and system logs to CloudWatch. Select between plain
* text and structured JSON.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #logFormat} will
* return {@link LogFormat#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #logFormatAsString}.
*
*
* @return The format in which Lambda sends your function's application and system logs to CloudWatch. Select
* between plain text and structured JSON.
* @see LogFormat
*/
public final String logFormatAsString() {
return logFormat;
}
/**
*
* Set this property to filter the application logs for your function that Lambda sends to CloudWatch. Lambda only
* sends application logs at the selected level of detail and lower, where TRACE
is the highest level
* and FATAL
is the lowest.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #applicationLogLevel} will return {@link ApplicationLogLevel#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #applicationLogLevelAsString}.
*
*
* @return Set this property to filter the application logs for your function that Lambda sends to CloudWatch.
* Lambda only sends application logs at the selected level of detail and lower, where TRACE
is
* the highest level and FATAL
is the lowest.
* @see ApplicationLogLevel
*/
public final ApplicationLogLevel applicationLogLevel() {
return ApplicationLogLevel.fromValue(applicationLogLevel);
}
/**
*
* Set this property to filter the application logs for your function that Lambda sends to CloudWatch. Lambda only
* sends application logs at the selected level of detail and lower, where TRACE
is the highest level
* and FATAL
is the lowest.
*
*
* If the service returns an enum value that is not available in the current SDK version,
* {@link #applicationLogLevel} will return {@link ApplicationLogLevel#UNKNOWN_TO_SDK_VERSION}. The raw value
* returned by the service is available from {@link #applicationLogLevelAsString}.
*
*
* @return Set this property to filter the application logs for your function that Lambda sends to CloudWatch.
* Lambda only sends application logs at the selected level of detail and lower, where TRACE
is
* the highest level and FATAL
is the lowest.
* @see ApplicationLogLevel
*/
public final String applicationLogLevelAsString() {
return applicationLogLevel;
}
/**
*
* Set this property to filter the system logs for your function that Lambda sends to CloudWatch. Lambda only sends
* system logs at the selected level of detail and lower, where DEBUG
is the highest level and
* WARN
is the lowest.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #systemLogLevel}
* will return {@link SystemLogLevel#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #systemLogLevelAsString}.
*
*
* @return Set this property to filter the system logs for your function that Lambda sends to CloudWatch. Lambda
* only sends system logs at the selected level of detail and lower, where DEBUG
is the highest
* level and WARN
is the lowest.
* @see SystemLogLevel
*/
public final SystemLogLevel systemLogLevel() {
return SystemLogLevel.fromValue(systemLogLevel);
}
/**
*
* Set this property to filter the system logs for your function that Lambda sends to CloudWatch. Lambda only sends
* system logs at the selected level of detail and lower, where DEBUG
is the highest level and
* WARN
is the lowest.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #systemLogLevel}
* will return {@link SystemLogLevel#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #systemLogLevelAsString}.
*
*
* @return Set this property to filter the system logs for your function that Lambda sends to CloudWatch. Lambda
* only sends system logs at the selected level of detail and lower, where DEBUG
is the highest
* level and WARN
is the lowest.
* @see SystemLogLevel
*/
public final String systemLogLevelAsString() {
return systemLogLevel;
}
/**
*
* The name of the Amazon CloudWatch log group the function sends logs to. By default, Lambda functions send logs to
* a default log group named /aws/lambda/<function name>
. To use a different log group, enter an
* existing log group or enter a new log group name.
*
*
* @return The name of the Amazon CloudWatch log group the function sends logs to. By default, Lambda functions send
* logs to a default log group named /aws/lambda/<function name>
. To use a different log
* group, enter an existing log group or enter a new log group name.
*/
public final String logGroup() {
return logGroup;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(logFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(applicationLogLevelAsString());
hashCode = 31 * hashCode + Objects.hashCode(systemLogLevelAsString());
hashCode = 31 * hashCode + Objects.hashCode(logGroup());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof LoggingConfig)) {
return false;
}
LoggingConfig other = (LoggingConfig) obj;
return Objects.equals(logFormatAsString(), other.logFormatAsString())
&& Objects.equals(applicationLogLevelAsString(), other.applicationLogLevelAsString())
&& Objects.equals(systemLogLevelAsString(), other.systemLogLevelAsString())
&& Objects.equals(logGroup(), other.logGroup());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("LoggingConfig").add("LogFormat", logFormatAsString())
.add("ApplicationLogLevel", applicationLogLevelAsString()).add("SystemLogLevel", systemLogLevelAsString())
.add("LogGroup", logGroup()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "LogFormat":
return Optional.ofNullable(clazz.cast(logFormatAsString()));
case "ApplicationLogLevel":
return Optional.ofNullable(clazz.cast(applicationLogLevelAsString()));
case "SystemLogLevel":
return Optional.ofNullable(clazz.cast(systemLogLevelAsString()));
case "LogGroup":
return Optional.ofNullable(clazz.cast(logGroup()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function