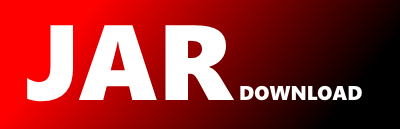
software.amazon.awssdk.services.lexmodelbuilding.model.CreateIntentVersionResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexmodelbuilding.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CreateIntentVersionResponse extends LexModelBuildingResponse implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateIntentVersionResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateIntentVersionResponse::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField> SLOTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateIntentVersionResponse::slots))
.setter(setter(Builder::slots))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("slots").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Slot::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> SAMPLE_UTTERANCES_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(CreateIntentVersionResponse::sampleUtterances))
.setter(setter(Builder::sampleUtterances))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("sampleUtterances").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CONFIRMATION_PROMPT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(CreateIntentVersionResponse::confirmationPrompt)).setter(setter(Builder::confirmationPrompt))
.constructor(Prompt::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("confirmationPrompt").build())
.build();
private static final SdkField REJECTION_STATEMENT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(CreateIntentVersionResponse::rejectionStatement)).setter(setter(Builder::rejectionStatement))
.constructor(Statement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("rejectionStatement").build())
.build();
private static final SdkField FOLLOW_UP_PROMPT_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).getter(getter(CreateIntentVersionResponse::followUpPrompt))
.setter(setter(Builder::followUpPrompt)).constructor(FollowUpPrompt::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("followUpPrompt").build()).build();
private static final SdkField CONCLUSION_STATEMENT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(CreateIntentVersionResponse::conclusionStatement)).setter(setter(Builder::conclusionStatement))
.constructor(Statement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("conclusionStatement").build())
.build();
private static final SdkField DIALOG_CODE_HOOK_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(CreateIntentVersionResponse::dialogCodeHook)).setter(setter(Builder::dialogCodeHook))
.constructor(CodeHook::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("dialogCodeHook").build()).build();
private static final SdkField FULFILLMENT_ACTIVITY_FIELD = SdkField
. builder(MarshallingType.SDK_POJO)
.getter(getter(CreateIntentVersionResponse::fulfillmentActivity)).setter(setter(Builder::fulfillmentActivity))
.constructor(FulfillmentActivity::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("fulfillmentActivity").build())
.build();
private static final SdkField PARENT_INTENT_SIGNATURE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateIntentVersionResponse::parentIntentSignature)).setter(setter(Builder::parentIntentSignature))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("parentIntentSignature").build())
.build();
private static final SdkField LAST_UPDATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(CreateIntentVersionResponse::lastUpdatedDate)).setter(setter(Builder::lastUpdatedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdatedDate").build()).build();
private static final SdkField CREATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(CreateIntentVersionResponse::createdDate)).setter(setter(Builder::createdDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdDate").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateIntentVersionResponse::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField CHECKSUM_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(CreateIntentVersionResponse::checksum)).setter(setter(Builder::checksum))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("checksum").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, DESCRIPTION_FIELD,
SLOTS_FIELD, SAMPLE_UTTERANCES_FIELD, CONFIRMATION_PROMPT_FIELD, REJECTION_STATEMENT_FIELD, FOLLOW_UP_PROMPT_FIELD,
CONCLUSION_STATEMENT_FIELD, DIALOG_CODE_HOOK_FIELD, FULFILLMENT_ACTIVITY_FIELD, PARENT_INTENT_SIGNATURE_FIELD,
LAST_UPDATED_DATE_FIELD, CREATED_DATE_FIELD, VERSION_FIELD, CHECKSUM_FIELD));
private final String name;
private final String description;
private final List slots;
private final List sampleUtterances;
private final Prompt confirmationPrompt;
private final Statement rejectionStatement;
private final FollowUpPrompt followUpPrompt;
private final Statement conclusionStatement;
private final CodeHook dialogCodeHook;
private final FulfillmentActivity fulfillmentActivity;
private final String parentIntentSignature;
private final Instant lastUpdatedDate;
private final Instant createdDate;
private final String version;
private final String checksum;
private CreateIntentVersionResponse(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.description = builder.description;
this.slots = builder.slots;
this.sampleUtterances = builder.sampleUtterances;
this.confirmationPrompt = builder.confirmationPrompt;
this.rejectionStatement = builder.rejectionStatement;
this.followUpPrompt = builder.followUpPrompt;
this.conclusionStatement = builder.conclusionStatement;
this.dialogCodeHook = builder.dialogCodeHook;
this.fulfillmentActivity = builder.fulfillmentActivity;
this.parentIntentSignature = builder.parentIntentSignature;
this.lastUpdatedDate = builder.lastUpdatedDate;
this.createdDate = builder.createdDate;
this.version = builder.version;
this.checksum = builder.checksum;
}
/**
*
* The name of the intent.
*
*
* @return The name of the intent.
*/
public String name() {
return name;
}
/**
*
* A description of the intent.
*
*
* @return A description of the intent.
*/
public String description() {
return description;
}
/**
* Returns true if the Slots property was specified by the sender (it may be empty), or false if the sender did not
* specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS service.
*/
public boolean hasSlots() {
return slots != null && !(slots instanceof SdkAutoConstructList);
}
/**
*
* An array of slot types that defines the information required to fulfill the intent.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSlots()} to see if a value was sent in this field.
*
*
* @return An array of slot types that defines the information required to fulfill the intent.
*/
public List slots() {
return slots;
}
/**
* Returns true if the SampleUtterances property was specified by the sender (it may be empty), or false if the
* sender did not specify the value (it will be empty). For responses returned by the SDK, the sender is the AWS
* service.
*/
public boolean hasSampleUtterances() {
return sampleUtterances != null && !(sampleUtterances instanceof SdkAutoConstructList);
}
/**
*
* An array of sample utterances configured for the intent.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* You can use {@link #hasSampleUtterances()} to see if a value was sent in this field.
*
*
* @return An array of sample utterances configured for the intent.
*/
public List sampleUtterances() {
return sampleUtterances;
}
/**
*
* If defined, the prompt that Amazon Lex uses to confirm the user's intent before fulfilling it.
*
*
* @return If defined, the prompt that Amazon Lex uses to confirm the user's intent before fulfilling it.
*/
public Prompt confirmationPrompt() {
return confirmationPrompt;
}
/**
*
* If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds with
* this statement to acknowledge that the intent was canceled.
*
*
* @return If the user answers "no" to the question defined in confirmationPrompt
, Amazon Lex responds
* with this statement to acknowledge that the intent was canceled.
*/
public Statement rejectionStatement() {
return rejectionStatement;
}
/**
*
* If defined, Amazon Lex uses this prompt to solicit additional user activity after the intent is fulfilled.
*
*
* @return If defined, Amazon Lex uses this prompt to solicit additional user activity after the intent is
* fulfilled.
*/
public FollowUpPrompt followUpPrompt() {
return followUpPrompt;
}
/**
*
* After the Lambda function specified in the fulfillmentActivity
field fulfills the intent, Amazon Lex
* conveys this statement to the user.
*
*
* @return After the Lambda function specified in the fulfillmentActivity
field fulfills the intent,
* Amazon Lex conveys this statement to the user.
*/
public Statement conclusionStatement() {
return conclusionStatement;
}
/**
*
* If defined, Amazon Lex invokes this Lambda function for each user input.
*
*
* @return If defined, Amazon Lex invokes this Lambda function for each user input.
*/
public CodeHook dialogCodeHook() {
return dialogCodeHook;
}
/**
*
* Describes how the intent is fulfilled.
*
*
* @return Describes how the intent is fulfilled.
*/
public FulfillmentActivity fulfillmentActivity() {
return fulfillmentActivity;
}
/**
*
* A unique identifier for a built-in intent.
*
*
* @return A unique identifier for a built-in intent.
*/
public String parentIntentSignature() {
return parentIntentSignature;
}
/**
*
* The date that the intent was updated.
*
*
* @return The date that the intent was updated.
*/
public Instant lastUpdatedDate() {
return lastUpdatedDate;
}
/**
*
* The date that the intent was created.
*
*
* @return The date that the intent was created.
*/
public Instant createdDate() {
return createdDate;
}
/**
*
* The version number assigned to the new version of the intent.
*
*
* @return The version number assigned to the new version of the intent.
*/
public String version() {
return version;
}
/**
*
* Checksum of the intent version created.
*
*
* @return Checksum of the intent version created.
*/
public String checksum() {
return checksum;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(slots());
hashCode = 31 * hashCode + Objects.hashCode(sampleUtterances());
hashCode = 31 * hashCode + Objects.hashCode(confirmationPrompt());
hashCode = 31 * hashCode + Objects.hashCode(rejectionStatement());
hashCode = 31 * hashCode + Objects.hashCode(followUpPrompt());
hashCode = 31 * hashCode + Objects.hashCode(conclusionStatement());
hashCode = 31 * hashCode + Objects.hashCode(dialogCodeHook());
hashCode = 31 * hashCode + Objects.hashCode(fulfillmentActivity());
hashCode = 31 * hashCode + Objects.hashCode(parentIntentSignature());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedDate());
hashCode = 31 * hashCode + Objects.hashCode(createdDate());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(checksum());
return hashCode;
}
@Override
public boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CreateIntentVersionResponse)) {
return false;
}
CreateIntentVersionResponse other = (CreateIntentVersionResponse) obj;
return Objects.equals(name(), other.name()) && Objects.equals(description(), other.description())
&& Objects.equals(slots(), other.slots()) && Objects.equals(sampleUtterances(), other.sampleUtterances())
&& Objects.equals(confirmationPrompt(), other.confirmationPrompt())
&& Objects.equals(rejectionStatement(), other.rejectionStatement())
&& Objects.equals(followUpPrompt(), other.followUpPrompt())
&& Objects.equals(conclusionStatement(), other.conclusionStatement())
&& Objects.equals(dialogCodeHook(), other.dialogCodeHook())
&& Objects.equals(fulfillmentActivity(), other.fulfillmentActivity())
&& Objects.equals(parentIntentSignature(), other.parentIntentSignature())
&& Objects.equals(lastUpdatedDate(), other.lastUpdatedDate())
&& Objects.equals(createdDate(), other.createdDate()) && Objects.equals(version(), other.version())
&& Objects.equals(checksum(), other.checksum());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("CreateIntentVersionResponse").add("Name", name()).add("Description", description())
.add("Slots", slots()).add("SampleUtterances", sampleUtterances())
.add("ConfirmationPrompt", confirmationPrompt()).add("RejectionStatement", rejectionStatement())
.add("FollowUpPrompt", followUpPrompt()).add("ConclusionStatement", conclusionStatement())
.add("DialogCodeHook", dialogCodeHook()).add("FulfillmentActivity", fulfillmentActivity())
.add("ParentIntentSignature", parentIntentSignature()).add("LastUpdatedDate", lastUpdatedDate())
.add("CreatedDate", createdDate()).add("Version", version()).add("Checksum", checksum()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "slots":
return Optional.ofNullable(clazz.cast(slots()));
case "sampleUtterances":
return Optional.ofNullable(clazz.cast(sampleUtterances()));
case "confirmationPrompt":
return Optional.ofNullable(clazz.cast(confirmationPrompt()));
case "rejectionStatement":
return Optional.ofNullable(clazz.cast(rejectionStatement()));
case "followUpPrompt":
return Optional.ofNullable(clazz.cast(followUpPrompt()));
case "conclusionStatement":
return Optional.ofNullable(clazz.cast(conclusionStatement()));
case "dialogCodeHook":
return Optional.ofNullable(clazz.cast(dialogCodeHook()));
case "fulfillmentActivity":
return Optional.ofNullable(clazz.cast(fulfillmentActivity()));
case "parentIntentSignature":
return Optional.ofNullable(clazz.cast(parentIntentSignature()));
case "lastUpdatedDate":
return Optional.ofNullable(clazz.cast(lastUpdatedDate()));
case "createdDate":
return Optional.ofNullable(clazz.cast(createdDate()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "checksum":
return Optional.ofNullable(clazz.cast(checksum()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function