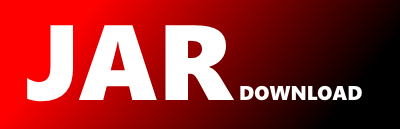
software.amazon.awssdk.services.lexmodelbuilding.model.GetExportResponse Maven / Gradle / Ivy
Show all versions of lexmodelbuilding Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexmodelbuilding.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetExportResponse extends LexModelBuildingResponse implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(GetExportResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.STRING).memberName("version")
.getter(getter(GetExportResponse::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceType").getter(getter(GetExportResponse::resourceTypeAsString))
.setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceType").build()).build();
private static final SdkField EXPORT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("exportType").getter(getter(GetExportResponse::exportTypeAsString)).setter(setter(Builder::exportType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("exportType").build()).build();
private static final SdkField EXPORT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("exportStatus").getter(getter(GetExportResponse::exportStatusAsString))
.setter(setter(Builder::exportStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("exportStatus").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("failureReason").getter(getter(GetExportResponse::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failureReason").build()).build();
private static final SdkField URL_FIELD = SdkField. builder(MarshallingType.STRING).memberName("url")
.getter(getter(GetExportResponse::url)).setter(setter(Builder::url))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("url").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, VERSION_FIELD,
RESOURCE_TYPE_FIELD, EXPORT_TYPE_FIELD, EXPORT_STATUS_FIELD, FAILURE_REASON_FIELD, URL_FIELD));
private final String name;
private final String version;
private final String resourceType;
private final String exportType;
private final String exportStatus;
private final String failureReason;
private final String url;
private GetExportResponse(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.version = builder.version;
this.resourceType = builder.resourceType;
this.exportType = builder.exportType;
this.exportStatus = builder.exportStatus;
this.failureReason = builder.failureReason;
this.url = builder.url;
}
/**
*
* The name of the bot being exported.
*
*
* @return The name of the bot being exported.
*/
public final String name() {
return name;
}
/**
*
* The version of the bot being exported.
*
*
* @return The version of the bot being exported.
*/
public final String version() {
return version;
}
/**
*
* The type of the exported resource.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resourceTypeAsString}.
*
*
* @return The type of the exported resource.
* @see ResourceType
*/
public final ResourceType resourceType() {
return ResourceType.fromValue(resourceType);
}
/**
*
* The type of the exported resource.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resourceTypeAsString}.
*
*
* @return The type of the exported resource.
* @see ResourceType
*/
public final String resourceTypeAsString() {
return resourceType;
}
/**
*
* The format of the exported data.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportType} will
* return {@link ExportType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportTypeAsString}.
*
*
* @return The format of the exported data.
* @see ExportType
*/
public final ExportType exportType() {
return ExportType.fromValue(exportType);
}
/**
*
* The format of the exported data.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportType} will
* return {@link ExportType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportTypeAsString}.
*
*
* @return The format of the exported data.
* @see ExportType
*/
public final String exportTypeAsString() {
return exportType;
}
/**
*
* The status of the export.
*
*
* -
*
* IN_PROGRESS
- The export is in progress.
*
*
* -
*
* READY
- The export is complete.
*
*
* -
*
* FAILED
- The export could not be completed.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportStatus} will
* return {@link ExportStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportStatusAsString}.
*
*
* @return The status of the export.
*
* -
*
* IN_PROGRESS
- The export is in progress.
*
*
* -
*
* READY
- The export is complete.
*
*
* -
*
* FAILED
- The export could not be completed.
*
*
* @see ExportStatus
*/
public final ExportStatus exportStatus() {
return ExportStatus.fromValue(exportStatus);
}
/**
*
* The status of the export.
*
*
* -
*
* IN_PROGRESS
- The export is in progress.
*
*
* -
*
* READY
- The export is complete.
*
*
* -
*
* FAILED
- The export could not be completed.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #exportStatus} will
* return {@link ExportStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #exportStatusAsString}.
*
*
* @return The status of the export.
*
* -
*
* IN_PROGRESS
- The export is in progress.
*
*
* -
*
* READY
- The export is complete.
*
*
* -
*
* FAILED
- The export could not be completed.
*
*
* @see ExportStatus
*/
public final String exportStatusAsString() {
return exportStatus;
}
/**
*
* If status
is FAILED
, Amazon Lex provides the reason that it failed to export the
* resource.
*
*
* @return If status
is FAILED
, Amazon Lex provides the reason that it failed to export
* the resource.
*/
public final String failureReason() {
return failureReason;
}
/**
*
* An S3 pre-signed URL that provides the location of the exported resource. The exported resource is a ZIP archive
* that contains the exported resource in JSON format. The structure of the archive may change. Your code should not
* rely on the archive structure.
*
*
* @return An S3 pre-signed URL that provides the location of the exported resource. The exported resource is a ZIP
* archive that contains the exported resource in JSON format. The structure of the archive may change. Your
* code should not rely on the archive structure.
*/
public final String url() {
return url;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(resourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(exportTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(exportStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(url());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetExportResponse)) {
return false;
}
GetExportResponse other = (GetExportResponse) obj;
return Objects.equals(name(), other.name()) && Objects.equals(version(), other.version())
&& Objects.equals(resourceTypeAsString(), other.resourceTypeAsString())
&& Objects.equals(exportTypeAsString(), other.exportTypeAsString())
&& Objects.equals(exportStatusAsString(), other.exportStatusAsString())
&& Objects.equals(failureReason(), other.failureReason()) && Objects.equals(url(), other.url());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetExportResponse").add("Name", name()).add("Version", version())
.add("ResourceType", resourceTypeAsString()).add("ExportType", exportTypeAsString())
.add("ExportStatus", exportStatusAsString()).add("FailureReason", failureReason()).add("Url", url()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "resourceType":
return Optional.ofNullable(clazz.cast(resourceTypeAsString()));
case "exportType":
return Optional.ofNullable(clazz.cast(exportTypeAsString()));
case "exportStatus":
return Optional.ofNullable(clazz.cast(exportStatusAsString()));
case "failureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "url":
return Optional.ofNullable(clazz.cast(url()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function