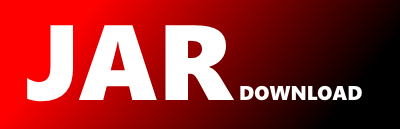
software.amazon.awssdk.services.lexmodelbuilding.model.GetImportResponse Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexmodelbuilding.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetImportResponse extends LexModelBuildingResponse implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING).memberName("name")
.getter(getter(GetImportResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField RESOURCE_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("resourceType").getter(getter(GetImportResponse::resourceTypeAsString))
.setter(setter(Builder::resourceType))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("resourceType").build()).build();
private static final SdkField MERGE_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("mergeStrategy").getter(getter(GetImportResponse::mergeStrategyAsString))
.setter(setter(Builder::mergeStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("mergeStrategy").build()).build();
private static final SdkField IMPORT_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("importId").getter(getter(GetImportResponse::importId)).setter(setter(Builder::importId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("importId").build()).build();
private static final SdkField IMPORT_STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("importStatus").getter(getter(GetImportResponse::importStatusAsString))
.setter(setter(Builder::importStatus))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("importStatus").build()).build();
private static final SdkField> FAILURE_REASON_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("failureReason")
.getter(getter(GetImportResponse::failureReason))
.setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failureReason").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.STRING)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CREATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.memberName("createdDate").getter(getter(GetImportResponse::createdDate)).setter(setter(Builder::createdDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdDate").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD,
RESOURCE_TYPE_FIELD, MERGE_STRATEGY_FIELD, IMPORT_ID_FIELD, IMPORT_STATUS_FIELD, FAILURE_REASON_FIELD,
CREATED_DATE_FIELD));
private final String name;
private final String resourceType;
private final String mergeStrategy;
private final String importId;
private final String importStatus;
private final List failureReason;
private final Instant createdDate;
private GetImportResponse(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.resourceType = builder.resourceType;
this.mergeStrategy = builder.mergeStrategy;
this.importId = builder.importId;
this.importStatus = builder.importStatus;
this.failureReason = builder.failureReason;
this.createdDate = builder.createdDate;
}
/**
*
* The name given to the import job.
*
*
* @return The name given to the import job.
*/
public final String name() {
return name;
}
/**
*
* The type of resource imported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resourceTypeAsString}.
*
*
* @return The type of resource imported.
* @see ResourceType
*/
public final ResourceType resourceType() {
return ResourceType.fromValue(resourceType);
}
/**
*
* The type of resource imported.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #resourceType} will
* return {@link ResourceType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #resourceTypeAsString}.
*
*
* @return The type of resource imported.
* @see ResourceType
*/
public final String resourceTypeAsString() {
return resourceType;
}
/**
*
* The action taken when there was a conflict between an existing resource and a resource in the import file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mergeStrategy}
* will return {@link MergeStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mergeStrategyAsString}.
*
*
* @return The action taken when there was a conflict between an existing resource and a resource in the import
* file.
* @see MergeStrategy
*/
public final MergeStrategy mergeStrategy() {
return MergeStrategy.fromValue(mergeStrategy);
}
/**
*
* The action taken when there was a conflict between an existing resource and a resource in the import file.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #mergeStrategy}
* will return {@link MergeStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #mergeStrategyAsString}.
*
*
* @return The action taken when there was a conflict between an existing resource and a resource in the import
* file.
* @see MergeStrategy
*/
public final String mergeStrategyAsString() {
return mergeStrategy;
}
/**
*
* The identifier for the specific import job.
*
*
* @return The identifier for the specific import job.
*/
public final String importId() {
return importId;
}
/**
*
* The status of the import job. If the status is FAILED
, you can get the reason for the failure from
* the failureReason
field.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #importStatus} will
* return {@link ImportStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #importStatusAsString}.
*
*
* @return The status of the import job. If the status is FAILED
, you can get the reason for the
* failure from the failureReason
field.
* @see ImportStatus
*/
public final ImportStatus importStatus() {
return ImportStatus.fromValue(importStatus);
}
/**
*
* The status of the import job. If the status is FAILED
, you can get the reason for the failure from
* the failureReason
field.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #importStatus} will
* return {@link ImportStatus#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #importStatusAsString}.
*
*
* @return The status of the import job. If the status is FAILED
, you can get the reason for the
* failure from the failureReason
field.
* @see ImportStatus
*/
public final String importStatusAsString() {
return importStatus;
}
/**
* For responses, this returns true if the service returned a value for the FailureReason property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasFailureReason() {
return failureReason != null && !(failureReason instanceof SdkAutoConstructList);
}
/**
*
* A string that describes why an import job failed to complete.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasFailureReason} method.
*
*
* @return A string that describes why an import job failed to complete.
*/
public final List failureReason() {
return failureReason;
}
/**
*
* A timestamp for the date and time that the import job was created.
*
*
* @return A timestamp for the date and time that the import job was created.
*/
public final Instant createdDate() {
return createdDate;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(resourceTypeAsString());
hashCode = 31 * hashCode + Objects.hashCode(mergeStrategyAsString());
hashCode = 31 * hashCode + Objects.hashCode(importId());
hashCode = 31 * hashCode + Objects.hashCode(importStatusAsString());
hashCode = 31 * hashCode + Objects.hashCode(hasFailureReason() ? failureReason() : null);
hashCode = 31 * hashCode + Objects.hashCode(createdDate());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetImportResponse)) {
return false;
}
GetImportResponse other = (GetImportResponse) obj;
return Objects.equals(name(), other.name()) && Objects.equals(resourceTypeAsString(), other.resourceTypeAsString())
&& Objects.equals(mergeStrategyAsString(), other.mergeStrategyAsString())
&& Objects.equals(importId(), other.importId())
&& Objects.equals(importStatusAsString(), other.importStatusAsString())
&& hasFailureReason() == other.hasFailureReason() && Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(createdDate(), other.createdDate());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("GetImportResponse").add("Name", name()).add("ResourceType", resourceTypeAsString())
.add("MergeStrategy", mergeStrategyAsString()).add("ImportId", importId())
.add("ImportStatus", importStatusAsString()).add("FailureReason", hasFailureReason() ? failureReason() : null)
.add("CreatedDate", createdDate()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "resourceType":
return Optional.ofNullable(clazz.cast(resourceTypeAsString()));
case "mergeStrategy":
return Optional.ofNullable(clazz.cast(mergeStrategyAsString()));
case "importId":
return Optional.ofNullable(clazz.cast(importId()));
case "importStatus":
return Optional.ofNullable(clazz.cast(importStatusAsString()));
case "failureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "createdDate":
return Optional.ofNullable(clazz.cast(createdDate()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function