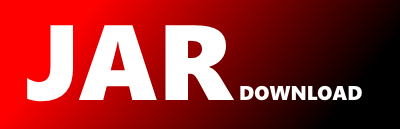
software.amazon.awssdk.services.lexmodelbuilding.model.StartMigrationRequest Maven / Gradle / Ivy
Show all versions of lexmodelbuilding Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexmodelbuilding.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class StartMigrationRequest extends LexModelBuildingRequest implements
ToCopyableBuilder {
private static final SdkField V1_BOT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("v1BotName").getter(getter(StartMigrationRequest::v1BotName)).setter(setter(Builder::v1BotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("v1BotName").build()).build();
private static final SdkField V1_BOT_VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("v1BotVersion").getter(getter(StartMigrationRequest::v1BotVersion)).setter(setter(Builder::v1BotVersion))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("v1BotVersion").build()).build();
private static final SdkField V2_BOT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("v2BotName").getter(getter(StartMigrationRequest::v2BotName)).setter(setter(Builder::v2BotName))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("v2BotName").build()).build();
private static final SdkField V2_BOT_ROLE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("v2BotRole").getter(getter(StartMigrationRequest::v2BotRole)).setter(setter(Builder::v2BotRole))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("v2BotRole").build()).build();
private static final SdkField MIGRATION_STRATEGY_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("migrationStrategy").getter(getter(StartMigrationRequest::migrationStrategyAsString))
.setter(setter(Builder::migrationStrategy))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("migrationStrategy").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(V1_BOT_NAME_FIELD,
V1_BOT_VERSION_FIELD, V2_BOT_NAME_FIELD, V2_BOT_ROLE_FIELD, MIGRATION_STRATEGY_FIELD));
private static final Map> SDK_NAME_TO_FIELD = Collections
.unmodifiableMap(new HashMap>() {
{
put("v1BotName", V1_BOT_NAME_FIELD);
put("v1BotVersion", V1_BOT_VERSION_FIELD);
put("v2BotName", V2_BOT_NAME_FIELD);
put("v2BotRole", V2_BOT_ROLE_FIELD);
put("migrationStrategy", MIGRATION_STRATEGY_FIELD);
}
});
private final String v1BotName;
private final String v1BotVersion;
private final String v2BotName;
private final String v2BotRole;
private final String migrationStrategy;
private StartMigrationRequest(BuilderImpl builder) {
super(builder);
this.v1BotName = builder.v1BotName;
this.v1BotVersion = builder.v1BotVersion;
this.v2BotName = builder.v2BotName;
this.v2BotRole = builder.v2BotRole;
this.migrationStrategy = builder.migrationStrategy;
}
/**
*
* The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*
*
* @return The name of the Amazon Lex V1 bot that you are migrating to Amazon Lex V2.
*/
public final String v1BotName() {
return v1BotName;
}
/**
*
* The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as well as
* any numbered version.
*
*
* @return The version of the bot to migrate to Amazon Lex V2. You can migrate the $LATEST
version as
* well as any numbered version.
*/
public final String v1BotVersion() {
return v1BotVersion;
}
/**
*
* The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to change the
* contents of the Amazon Lex V2 bot.
*
*
*
*
* @return The name of the Amazon Lex V2 bot that you are migrating the Amazon Lex V1 bot to.
*
* -
*
* If the Amazon Lex V2 bot doesn't exist, you must use the CREATE_NEW
migration strategy.
*
*
* -
*
* If the Amazon Lex V2 bot exists, you must use the UPDATE_EXISTING
migration strategy to
* change the contents of the Amazon Lex V2 bot.
*
*
*/
public final String v2BotName() {
return v2BotName;
}
/**
*
* The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*
*
* @return The IAM role that Amazon Lex uses to run the Amazon Lex V2 bot.
*/
public final String v2BotRole() {
return v2BotRole;
}
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #migrationStrategy}
* will return {@link MigrationStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #migrationStrategyAsString}.
*
*
* @return The strategy used to conduct the migration.
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new
* bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being
* migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a
* new locale is created in the Amazon Lex V2 bot.
*
*
* @see MigrationStrategy
*/
public final MigrationStrategy migrationStrategy() {
return MigrationStrategy.fromValue(migrationStrategy);
}
/**
*
* The strategy used to conduct the migration.
*
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being migrated.
* It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a new locale is
* created in the Amazon Lex V2 bot.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #migrationStrategy}
* will return {@link MigrationStrategy#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #migrationStrategyAsString}.
*
*
* @return The strategy used to conduct the migration.
*
* -
*
* CREATE_NEW
- Creates a new Amazon Lex V2 bot and migrates the Amazon Lex V1 bot to the new
* bot.
*
*
* -
*
* UPDATE_EXISTING
- Overwrites the existing Amazon Lex V2 bot metadata and the locale being
* migrated. It doesn't change any other locales in the Amazon Lex V2 bot. If the locale doesn't exist, a
* new locale is created in the Amazon Lex V2 bot.
*
*
* @see MigrationStrategy
*/
public final String migrationStrategyAsString() {
return migrationStrategy;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(v1BotName());
hashCode = 31 * hashCode + Objects.hashCode(v1BotVersion());
hashCode = 31 * hashCode + Objects.hashCode(v2BotName());
hashCode = 31 * hashCode + Objects.hashCode(v2BotRole());
hashCode = 31 * hashCode + Objects.hashCode(migrationStrategyAsString());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof StartMigrationRequest)) {
return false;
}
StartMigrationRequest other = (StartMigrationRequest) obj;
return Objects.equals(v1BotName(), other.v1BotName()) && Objects.equals(v1BotVersion(), other.v1BotVersion())
&& Objects.equals(v2BotName(), other.v2BotName()) && Objects.equals(v2BotRole(), other.v2BotRole())
&& Objects.equals(migrationStrategyAsString(), other.migrationStrategyAsString());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("StartMigrationRequest").add("V1BotName", v1BotName()).add("V1BotVersion", v1BotVersion())
.add("V2BotName", v2BotName()).add("V2BotRole", v2BotRole())
.add("MigrationStrategy", migrationStrategyAsString()).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "v1BotName":
return Optional.ofNullable(clazz.cast(v1BotName()));
case "v1BotVersion":
return Optional.ofNullable(clazz.cast(v1BotVersion()));
case "v2BotName":
return Optional.ofNullable(clazz.cast(v2BotName()));
case "v2BotRole":
return Optional.ofNullable(clazz.cast(v2BotRole()));
case "migrationStrategy":
return Optional.ofNullable(clazz.cast(migrationStrategyAsString()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Function