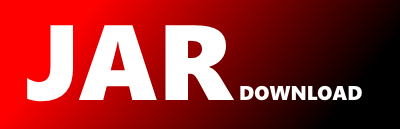
software.amazon.awssdk.services.lexmodelbuilding.model.GetBotResponse Maven / Gradle / Ivy
Show all versions of lexmodelbuilding Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexmodelbuilding.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class GetBotResponse extends LexModelBuildingResponse implements
ToCopyableBuilder {
private static final SdkField NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::name)).setter(setter(Builder::name))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("name").build()).build();
private static final SdkField DESCRIPTION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::description)).setter(setter(Builder::description))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("description").build()).build();
private static final SdkField> INTENTS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.getter(getter(GetBotResponse::intents))
.setter(setter(Builder::intents))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("intents").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Intent::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField CLARIFICATION_PROMPT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(GetBotResponse::clarificationPrompt)).setter(setter(Builder::clarificationPrompt))
.constructor(Prompt::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("clarificationPrompt").build())
.build();
private static final SdkField ABORT_STATEMENT_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.getter(getter(GetBotResponse::abortStatement)).setter(setter(Builder::abortStatement))
.constructor(Statement::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("abortStatement").build()).build();
private static final SdkField STATUS_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::statusAsString)).setter(setter(Builder::status))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("status").build()).build();
private static final SdkField FAILURE_REASON_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::failureReason)).setter(setter(Builder::failureReason))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("failureReason").build()).build();
private static final SdkField LAST_UPDATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetBotResponse::lastUpdatedDate)).setter(setter(Builder::lastUpdatedDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("lastUpdatedDate").build()).build();
private static final SdkField CREATED_DATE_FIELD = SdkField. builder(MarshallingType.INSTANT)
.getter(getter(GetBotResponse::createdDate)).setter(setter(Builder::createdDate))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("createdDate").build()).build();
private static final SdkField IDLE_SESSION_TTL_IN_SECONDS_FIELD = SdkField
. builder(MarshallingType.INTEGER).getter(getter(GetBotResponse::idleSessionTTLInSeconds))
.setter(setter(Builder::idleSessionTTLInSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("idleSessionTTLInSeconds").build())
.build();
private static final SdkField VOICE_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::voiceId)).setter(setter(Builder::voiceId))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("voiceId").build()).build();
private static final SdkField CHECKSUM_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::checksum)).setter(setter(Builder::checksum))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("checksum").build()).build();
private static final SdkField VERSION_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::version)).setter(setter(Builder::version))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("version").build()).build();
private static final SdkField LOCALE_FIELD = SdkField. builder(MarshallingType.STRING)
.getter(getter(GetBotResponse::localeAsString)).setter(setter(Builder::locale))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("locale").build()).build();
private static final SdkField CHILD_DIRECTED_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.getter(getter(GetBotResponse::childDirected)).setter(setter(Builder::childDirected))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("childDirected").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(NAME_FIELD, DESCRIPTION_FIELD,
INTENTS_FIELD, CLARIFICATION_PROMPT_FIELD, ABORT_STATEMENT_FIELD, STATUS_FIELD, FAILURE_REASON_FIELD,
LAST_UPDATED_DATE_FIELD, CREATED_DATE_FIELD, IDLE_SESSION_TTL_IN_SECONDS_FIELD, VOICE_ID_FIELD, CHECKSUM_FIELD,
VERSION_FIELD, LOCALE_FIELD, CHILD_DIRECTED_FIELD));
private final String name;
private final String description;
private final List intents;
private final Prompt clarificationPrompt;
private final Statement abortStatement;
private final String status;
private final String failureReason;
private final Instant lastUpdatedDate;
private final Instant createdDate;
private final Integer idleSessionTTLInSeconds;
private final String voiceId;
private final String checksum;
private final String version;
private final String locale;
private final Boolean childDirected;
private GetBotResponse(BuilderImpl builder) {
super(builder);
this.name = builder.name;
this.description = builder.description;
this.intents = builder.intents;
this.clarificationPrompt = builder.clarificationPrompt;
this.abortStatement = builder.abortStatement;
this.status = builder.status;
this.failureReason = builder.failureReason;
this.lastUpdatedDate = builder.lastUpdatedDate;
this.createdDate = builder.createdDate;
this.idleSessionTTLInSeconds = builder.idleSessionTTLInSeconds;
this.voiceId = builder.voiceId;
this.checksum = builder.checksum;
this.version = builder.version;
this.locale = builder.locale;
this.childDirected = builder.childDirected;
}
/**
*
* The name of the bot.
*
*
* @return The name of the bot.
*/
public String name() {
return name;
}
/**
*
* A description of the bot.
*
*
* @return A description of the bot.
*/
public String description() {
return description;
}
/**
*
* An array of intent
objects. For more information, see PutBot.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* @return An array of intent
objects. For more information, see PutBot.
*/
public List intents() {
return intents;
}
/**
*
* The message Amazon Lex uses when it doesn't understand the user's request. For more information, see
* PutBot.
*
*
* @return The message Amazon Lex uses when it doesn't understand the user's request. For more information, see
* PutBot.
*/
public Prompt clarificationPrompt() {
return clarificationPrompt;
}
/**
*
* The message that Amazon Lex returns when the user elects to end the conversation without completing it. For more
* information, see PutBot.
*
*
* @return The message that Amazon Lex returns when the user elects to end the conversation without completing it.
* For more information, see PutBot.
*/
public Statement abortStatement() {
return abortStatement;
}
/**
*
* The status of the bot. If the bot is ready to run, the status is READY
. If there was a problem with
* building the bot, the status is FAILED
and the failureReason
explains why the bot did
* not build. If the bot was saved but not built, the status is NOT BUILT
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the bot. If the bot is ready to run, the status is READY
. If there was a
* problem with building the bot, the status is FAILED
and the failureReason
* explains why the bot did not build. If the bot was saved but not built, the status is
* NOT BUILT
.
* @see Status
*/
public Status status() {
return Status.fromValue(status);
}
/**
*
* The status of the bot. If the bot is ready to run, the status is READY
. If there was a problem with
* building the bot, the status is FAILED
and the failureReason
explains why the bot did
* not build. If the bot was saved but not built, the status is NOT BUILT
.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #status} will
* return {@link Status#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #statusAsString}.
*
*
* @return The status of the bot. If the bot is ready to run, the status is READY
. If there was a
* problem with building the bot, the status is FAILED
and the failureReason
* explains why the bot did not build. If the bot was saved but not built, the status is
* NOT BUILT
.
* @see Status
*/
public String statusAsString() {
return status;
}
/**
*
* If status
is FAILED
, Amazon Lex explains why it failed to build the bot.
*
*
* @return If status
is FAILED
, Amazon Lex explains why it failed to build the bot.
*/
public String failureReason() {
return failureReason;
}
/**
*
* The date that the bot was updated. When you create a resource, the creation date and last updated date are the
* same.
*
*
* @return The date that the bot was updated. When you create a resource, the creation date and last updated date
* are the same.
*/
public Instant lastUpdatedDate() {
return lastUpdatedDate;
}
/**
*
* The date that the bot was created.
*
*
* @return The date that the bot was created.
*/
public Instant createdDate() {
return createdDate;
}
/**
*
* The maximum time in seconds that Amazon Lex retains the data gathered in a conversation. For more information,
* see PutBot.
*
*
* @return The maximum time in seconds that Amazon Lex retains the data gathered in a conversation. For more
* information, see PutBot.
*/
public Integer idleSessionTTLInSeconds() {
return idleSessionTTLInSeconds;
}
/**
*
* The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information, see
* PutBot.
*
*
* @return The Amazon Polly voice ID that Amazon Lex uses for voice interaction with the user. For more information,
* see PutBot.
*/
public String voiceId() {
return voiceId;
}
/**
*
* Checksum of the bot used to identify a specific revision of the bot's $LATEST
version.
*
*
* @return Checksum of the bot used to identify a specific revision of the bot's $LATEST
version.
*/
public String checksum() {
return checksum;
}
/**
*
* The version of the bot. For a new bot, the version is always $LATEST
.
*
*
* @return The version of the bot. For a new bot, the version is always $LATEST
.
*/
public String version() {
return version;
}
/**
*
* The target locale for the bot.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #locale} will
* return {@link Locale#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #localeAsString}.
*
*
* @return The target locale for the bot.
* @see Locale
*/
public Locale locale() {
return Locale.fromValue(locale);
}
/**
*
* The target locale for the bot.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #locale} will
* return {@link Locale#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #localeAsString}.
*
*
* @return The target locale for the bot.
* @see Locale
*/
public String localeAsString() {
return locale;
}
/**
*
* For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your use of
* Amazon Lex is related to a website, program, or other application that is directed or targeted, in whole or in
* part, to children under age 13 and subject to the Children's Online Privacy Protection Act (COPPA) by specifying
* true
or false
in the childDirected
field. By specifying true
* in the childDirected
field, you confirm that your use of Amazon Lex is related to a website,
* program, or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA. By specifying false
in the childDirected
field, you confirm that your
* use of Amazon Lex is not related to a website, program, or other application that is directed or targeted,
* in whole or in part, to children under age 13 and subject to COPPA. You may not specify a default value for the
* childDirected
field that does not accurately reflect whether your use of Amazon Lex is related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children under age
* 13 and subject to COPPA.
*
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole or in
* part, to children under age 13, you must obtain any required verifiable parental consent under COPPA. For
* information regarding the use of Amazon Lex in connection with websites, programs, or other applications that are
* directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*
*
* @return For each Amazon Lex bot created with the Amazon Lex Model Building Service, you must specify whether your
* use of Amazon Lex is related to a website, program, or other application that is directed or targeted, in
* whole or in part, to children under age 13 and subject to the Children's Online Privacy Protection Act
* (COPPA) by specifying true
or false
in the childDirected
field. By
* specifying true
in the childDirected
field, you confirm that your use of Amazon
* Lex is related to a website, program, or other application that is directed or targeted, in whole
* or in part, to children under age 13 and subject to COPPA. By specifying false
in the
* childDirected
field, you confirm that your use of Amazon Lex is not related to a
* website, program, or other application that is directed or targeted, in whole or in part, to children
* under age 13 and subject to COPPA. You may not specify a default value for the childDirected
* field that does not accurately reflect whether your use of Amazon Lex is related to a website, program,
* or other application that is directed or targeted, in whole or in part, to children under age 13 and
* subject to COPPA.
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in whole
* or in part, to children under age 13, you must obtain any required verifiable parental consent under
* COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or other
* applications that are directed or targeted, in whole or in part, to children under age 13, see the Amazon Lex FAQ.
*/
public Boolean childDirected() {
return childDirected;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(name());
hashCode = 31 * hashCode + Objects.hashCode(description());
hashCode = 31 * hashCode + Objects.hashCode(intents());
hashCode = 31 * hashCode + Objects.hashCode(clarificationPrompt());
hashCode = 31 * hashCode + Objects.hashCode(abortStatement());
hashCode = 31 * hashCode + Objects.hashCode(statusAsString());
hashCode = 31 * hashCode + Objects.hashCode(failureReason());
hashCode = 31 * hashCode + Objects.hashCode(lastUpdatedDate());
hashCode = 31 * hashCode + Objects.hashCode(createdDate());
hashCode = 31 * hashCode + Objects.hashCode(idleSessionTTLInSeconds());
hashCode = 31 * hashCode + Objects.hashCode(voiceId());
hashCode = 31 * hashCode + Objects.hashCode(checksum());
hashCode = 31 * hashCode + Objects.hashCode(version());
hashCode = 31 * hashCode + Objects.hashCode(localeAsString());
hashCode = 31 * hashCode + Objects.hashCode(childDirected());
return hashCode;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof GetBotResponse)) {
return false;
}
GetBotResponse other = (GetBotResponse) obj;
return Objects.equals(name(), other.name()) && Objects.equals(description(), other.description())
&& Objects.equals(intents(), other.intents())
&& Objects.equals(clarificationPrompt(), other.clarificationPrompt())
&& Objects.equals(abortStatement(), other.abortStatement())
&& Objects.equals(statusAsString(), other.statusAsString())
&& Objects.equals(failureReason(), other.failureReason())
&& Objects.equals(lastUpdatedDate(), other.lastUpdatedDate())
&& Objects.equals(createdDate(), other.createdDate())
&& Objects.equals(idleSessionTTLInSeconds(), other.idleSessionTTLInSeconds())
&& Objects.equals(voiceId(), other.voiceId()) && Objects.equals(checksum(), other.checksum())
&& Objects.equals(version(), other.version()) && Objects.equals(localeAsString(), other.localeAsString())
&& Objects.equals(childDirected(), other.childDirected());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public String toString() {
return ToString.builder("GetBotResponse").add("Name", name()).add("Description", description()).add("Intents", intents())
.add("ClarificationPrompt", clarificationPrompt()).add("AbortStatement", abortStatement())
.add("Status", statusAsString()).add("FailureReason", failureReason()).add("LastUpdatedDate", lastUpdatedDate())
.add("CreatedDate", createdDate()).add("IdleSessionTTLInSeconds", idleSessionTTLInSeconds())
.add("VoiceId", voiceId()).add("Checksum", checksum()).add("Version", version()).add("Locale", localeAsString())
.add("ChildDirected", childDirected()).build();
}
public Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "name":
return Optional.ofNullable(clazz.cast(name()));
case "description":
return Optional.ofNullable(clazz.cast(description()));
case "intents":
return Optional.ofNullable(clazz.cast(intents()));
case "clarificationPrompt":
return Optional.ofNullable(clazz.cast(clarificationPrompt()));
case "abortStatement":
return Optional.ofNullable(clazz.cast(abortStatement()));
case "status":
return Optional.ofNullable(clazz.cast(statusAsString()));
case "failureReason":
return Optional.ofNullable(clazz.cast(failureReason()));
case "lastUpdatedDate":
return Optional.ofNullable(clazz.cast(lastUpdatedDate()));
case "createdDate":
return Optional.ofNullable(clazz.cast(createdDate()));
case "idleSessionTTLInSeconds":
return Optional.ofNullable(clazz.cast(idleSessionTTLInSeconds()));
case "voiceId":
return Optional.ofNullable(clazz.cast(voiceId()));
case "checksum":
return Optional.ofNullable(clazz.cast(checksum()));
case "version":
return Optional.ofNullable(clazz.cast(version()));
case "locale":
return Optional.ofNullable(clazz.cast(localeAsString()));
case "childDirected":
return Optional.ofNullable(clazz.cast(childDirected()));
default:
return Optional.empty();
}
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* If your use of Amazon Lex relates to a website, program, or other application that is directed in
* whole or in part, to children under age 13, you must obtain any required verifiable parental consent
* under COPPA. For information regarding the use of Amazon Lex in connection with websites, programs, or
* other applications that are directed or targeted, in whole or in part, to children under age 13, see
* the Amazon Lex FAQ.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder childDirected(Boolean childDirected);
}
static final class BuilderImpl extends LexModelBuildingResponse.BuilderImpl implements Builder {
private String name;
private String description;
private List intents = DefaultSdkAutoConstructList.getInstance();
private Prompt clarificationPrompt;
private Statement abortStatement;
private String status;
private String failureReason;
private Instant lastUpdatedDate;
private Instant createdDate;
private Integer idleSessionTTLInSeconds;
private String voiceId;
private String checksum;
private String version;
private String locale;
private Boolean childDirected;
private BuilderImpl() {
}
private BuilderImpl(GetBotResponse model) {
super(model);
name(model.name);
description(model.description);
intents(model.intents);
clarificationPrompt(model.clarificationPrompt);
abortStatement(model.abortStatement);
status(model.status);
failureReason(model.failureReason);
lastUpdatedDate(model.lastUpdatedDate);
createdDate(model.createdDate);
idleSessionTTLInSeconds(model.idleSessionTTLInSeconds);
voiceId(model.voiceId);
checksum(model.checksum);
version(model.version);
locale(model.locale);
childDirected(model.childDirected);
}
public final String getName() {
return name;
}
@Override
public final Builder name(String name) {
this.name = name;
return this;
}
public final void setName(String name) {
this.name = name;
}
public final String getDescription() {
return description;
}
@Override
public final Builder description(String description) {
this.description = description;
return this;
}
public final void setDescription(String description) {
this.description = description;
}
public final Collection getIntents() {
return intents != null ? intents.stream().map(Intent::toBuilder).collect(Collectors.toList()) : null;
}
@Override
public final Builder intents(Collection intents) {
this.intents = IntentListCopier.copy(intents);
return this;
}
@Override
@SafeVarargs
public final Builder intents(Intent... intents) {
intents(Arrays.asList(intents));
return this;
}
@Override
@SafeVarargs
public final Builder intents(Consumer... intents) {
intents(Stream.of(intents).map(c -> Intent.builder().applyMutation(c).build()).collect(Collectors.toList()));
return this;
}
public final void setIntents(Collection intents) {
this.intents = IntentListCopier.copyFromBuilder(intents);
}
public final Prompt.Builder getClarificationPrompt() {
return clarificationPrompt != null ? clarificationPrompt.toBuilder() : null;
}
@Override
public final Builder clarificationPrompt(Prompt clarificationPrompt) {
this.clarificationPrompt = clarificationPrompt;
return this;
}
public final void setClarificationPrompt(Prompt.BuilderImpl clarificationPrompt) {
this.clarificationPrompt = clarificationPrompt != null ? clarificationPrompt.build() : null;
}
public final Statement.Builder getAbortStatement() {
return abortStatement != null ? abortStatement.toBuilder() : null;
}
@Override
public final Builder abortStatement(Statement abortStatement) {
this.abortStatement = abortStatement;
return this;
}
public final void setAbortStatement(Statement.BuilderImpl abortStatement) {
this.abortStatement = abortStatement != null ? abortStatement.build() : null;
}
public final String getStatusAsString() {
return status;
}
@Override
public final Builder status(String status) {
this.status = status;
return this;
}
@Override
public final Builder status(Status status) {
this.status(status.toString());
return this;
}
public final void setStatus(String status) {
this.status = status;
}
public final String getFailureReason() {
return failureReason;
}
@Override
public final Builder failureReason(String failureReason) {
this.failureReason = failureReason;
return this;
}
public final void setFailureReason(String failureReason) {
this.failureReason = failureReason;
}
public final Instant getLastUpdatedDate() {
return lastUpdatedDate;
}
@Override
public final Builder lastUpdatedDate(Instant lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
return this;
}
public final void setLastUpdatedDate(Instant lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
}
public final Instant getCreatedDate() {
return createdDate;
}
@Override
public final Builder createdDate(Instant createdDate) {
this.createdDate = createdDate;
return this;
}
public final void setCreatedDate(Instant createdDate) {
this.createdDate = createdDate;
}
public final Integer getIdleSessionTTLInSeconds() {
return idleSessionTTLInSeconds;
}
@Override
public final Builder idleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
this.idleSessionTTLInSeconds = idleSessionTTLInSeconds;
return this;
}
public final void setIdleSessionTTLInSeconds(Integer idleSessionTTLInSeconds) {
this.idleSessionTTLInSeconds = idleSessionTTLInSeconds;
}
public final String getVoiceId() {
return voiceId;
}
@Override
public final Builder voiceId(String voiceId) {
this.voiceId = voiceId;
return this;
}
public final void setVoiceId(String voiceId) {
this.voiceId = voiceId;
}
public final String getChecksum() {
return checksum;
}
@Override
public final Builder checksum(String checksum) {
this.checksum = checksum;
return this;
}
public final void setChecksum(String checksum) {
this.checksum = checksum;
}
public final String getVersion() {
return version;
}
@Override
public final Builder version(String version) {
this.version = version;
return this;
}
public final void setVersion(String version) {
this.version = version;
}
public final String getLocaleAsString() {
return locale;
}
@Override
public final Builder locale(String locale) {
this.locale = locale;
return this;
}
@Override
public final Builder locale(Locale locale) {
this.locale(locale.toString());
return this;
}
public final void setLocale(String locale) {
this.locale = locale;
}
public final Boolean getChildDirected() {
return childDirected;
}
@Override
public final Builder childDirected(Boolean childDirected) {
this.childDirected = childDirected;
return this;
}
public final void setChildDirected(Boolean childDirected) {
this.childDirected = childDirected;
}
@Override
public GetBotResponse build() {
return new GetBotResponse(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}