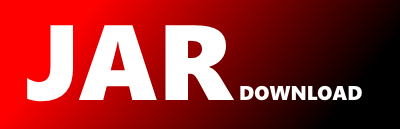
software.amazon.awssdk.services.lexruntime.LexRuntimeAsyncClient Maven / Gradle / Ivy
Show all versions of lexruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexruntime;
import java.nio.file.Path;
import java.util.concurrent.CompletableFuture;
import java.util.function.Consumer;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.annotations.SdkPublicApi;
import software.amazon.awssdk.annotations.ThreadSafe;
import software.amazon.awssdk.awscore.AwsClient;
import software.amazon.awssdk.core.async.AsyncRequestBody;
import software.amazon.awssdk.core.async.AsyncResponseTransformer;
import software.amazon.awssdk.services.lexruntime.model.DeleteSessionRequest;
import software.amazon.awssdk.services.lexruntime.model.DeleteSessionResponse;
import software.amazon.awssdk.services.lexruntime.model.GetSessionRequest;
import software.amazon.awssdk.services.lexruntime.model.GetSessionResponse;
import software.amazon.awssdk.services.lexruntime.model.PostContentRequest;
import software.amazon.awssdk.services.lexruntime.model.PostContentResponse;
import software.amazon.awssdk.services.lexruntime.model.PostTextRequest;
import software.amazon.awssdk.services.lexruntime.model.PostTextResponse;
import software.amazon.awssdk.services.lexruntime.model.PutSessionRequest;
import software.amazon.awssdk.services.lexruntime.model.PutSessionResponse;
/**
* Service client for accessing Amazon Lex Runtime Service asynchronously. This can be created using the static
* {@link #builder()} method.The asynchronous client performs non-blocking I/O when configured with any
* {@code SdkAsyncHttpClient} supported in the SDK. However, full non-blocking is not guaranteed as the async client may
* perform blocking calls in some cases such as credentials retrieval and endpoint discovery as part of the async API
* call.
*
*
* Amazon Lex provides both build and runtime endpoints. Each endpoint provides a set of operations (API). Your
* conversational bot uses the runtime API to understand user utterances (user input text or voice). For example,
* suppose a user says "I want pizza", your bot sends this input to Amazon Lex using the runtime API. Amazon Lex
* recognizes that the user request is for the OrderPizza intent (one of the intents defined in the bot). Then Amazon
* Lex engages in user conversation on behalf of the bot to elicit required information (slot values, such as pizza size
* and crust type), and then performs fulfillment activity (that you configured when you created the bot). You use the
* build-time API to create and manage your Amazon Lex bot. For a list of build-time operations, see the build-time API,
* .
*
*/
@Generated("software.amazon.awssdk:codegen")
@SdkPublicApi
@ThreadSafe
public interface LexRuntimeAsyncClient extends AwsClient {
String SERVICE_NAME = "lex";
/**
* Value for looking up the service's metadata from the
* {@link software.amazon.awssdk.regions.ServiceMetadataProvider}.
*/
String SERVICE_METADATA_ID = "runtime.lex";
/**
*
* Removes session information for a specified bot, alias, and user ID.
*
*
* @param deleteSessionRequest
* @return A Java Future containing the result of the DeleteSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.DeleteSession
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSession(DeleteSessionRequest deleteSessionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Removes session information for a specified bot, alias, and user ID.
*
*
*
* This is a convenience which creates an instance of the {@link DeleteSessionRequest.Builder} avoiding the need to
* create one manually via {@link DeleteSessionRequest#builder()}
*
*
* @param deleteSessionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.DeleteSessionRequest.Builder} to create a request.
* @return A Java Future containing the result of the DeleteSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.DeleteSession
* @see AWS API
* Documentation
*/
default CompletableFuture deleteSession(Consumer deleteSessionRequest) {
return deleteSession(DeleteSessionRequest.builder().applyMutation(deleteSessionRequest).build());
}
/**
*
* Returns session information for a specified bot, alias, and user ID.
*
*
* @param getSessionRequest
* @return A Java Future containing the result of the GetSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.GetSession
* @see AWS API
* Documentation
*/
default CompletableFuture getSession(GetSessionRequest getSessionRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Returns session information for a specified bot, alias, and user ID.
*
*
*
* This is a convenience which creates an instance of the {@link GetSessionRequest.Builder} avoiding the need to
* create one manually via {@link GetSessionRequest#builder()}
*
*
* @param getSessionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.GetSessionRequest.Builder} to create a request.
* @return A Java Future containing the result of the GetSession operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.GetSession
* @see AWS API
* Documentation
*/
default CompletableFuture getSession(Consumer getSessionRequest) {
return getSession(GetSessionRequest.builder().applyMutation(getSessionRequest).build());
}
/**
*
* Sends user input (text or speech) to Amazon Lex. Clients use this API to send text and audio requests to Amazon
* Lex at runtime. Amazon Lex interprets the user input using the machine learning model that it built for the bot.
*
*
* The PostContent
operation supports audio input at 8kHz and 16kHz. You can use 8kHz audio to achieve
* higher speech recognition accuracy in telephone audio applications.
*
*
* In response, Amazon Lex returns the next message to convey to the user. Consider the following example messages:
*
*
* -
*
* For a user input "I would like a pizza," Amazon Lex might return a response with a message eliciting slot data
* (for example, PizzaSize
): "What size pizza would you like?".
*
*
* -
*
* After the user provides all of the pizza order information, Amazon Lex might return a response with a message to
* get user confirmation: "Order the pizza?".
*
*
* -
*
* After the user replies "Yes" to the confirmation prompt, Amazon Lex might return a conclusion statement:
* "Thank you, your cheese pizza has been ordered.".
*
*
*
*
* Not all Amazon Lex messages require a response from the user. For example, conclusion statements do not require a
* response. Some messages require only a yes or no response. In addition to the message
, Amazon Lex
* provides additional context about the message in the response that you can use to enhance client behavior, such
* as displaying the appropriate client user interface. Consider the following examples:
*
*
* -
*
* If the message is to elicit slot data, Amazon Lex returns the following context information:
*
*
* -
*
* x-amz-lex-dialog-state
header set to ElicitSlot
*
*
* -
*
* x-amz-lex-intent-name
header set to the intent name in the current context
*
*
* -
*
* x-amz-lex-slot-to-elicit
header set to the slot name for which the message
is eliciting
* information
*
*
* -
*
* x-amz-lex-slots
header set to a map of slots configured for the intent with their current values
*
*
*
*
* -
*
* If the message is a confirmation prompt, the x-amz-lex-dialog-state
header is set to
* Confirmation
and the x-amz-lex-slot-to-elicit
header is omitted.
*
*
* -
*
* If the message is a clarification prompt configured for the intent, indicating that the user intent is not
* understood, the x-amz-dialog-state
header is set to ElicitIntent
and the
* x-amz-slot-to-elicit
header is omitted.
*
*
*
*
* In addition, Amazon Lex also returns your application-specific sessionAttributes
. For more
* information, see Managing Conversation
* Context.
*
*
* @param postContentRequest
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* User input in PCM or Opus audio format or text format as described in the Content-Type
HTTP
* header.
*
*
* You can stream audio data to Amazon Lex or you can create a local buffer that captures all of the audio
* data before sending. In general, you get better performance if you stream audio data rather than buffering
* the data locally.
*
* '
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The prompt (or statement) to convey to the user. This is based on the bot configuration and context. For
* example, if Amazon Lex did not understand the user intent, it sends the clarificationPrompt
* configured for the bot. If the intent requires confirmation before taking the fulfillment action, it sends
* the confirmationPrompt
. Another example: Suppose that the Lambda function successfully
* fulfilled the intent, and sent a message to convey to the user. Then Amazon Lex sends that message in the
* response.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - UnsupportedMediaTypeException The Content-Type header (
PostContent
API) has an invalid
* value.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - RequestTimeoutException The input speech is too long.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - LoopDetectedException This exception is not used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PostContent
* @see AWS API
* Documentation
*/
default CompletableFuture postContent(PostContentRequest postContentRequest, AsyncRequestBody requestBody,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Sends user input (text or speech) to Amazon Lex. Clients use this API to send text and audio requests to Amazon
* Lex at runtime. Amazon Lex interprets the user input using the machine learning model that it built for the bot.
*
*
* The PostContent
operation supports audio input at 8kHz and 16kHz. You can use 8kHz audio to achieve
* higher speech recognition accuracy in telephone audio applications.
*
*
* In response, Amazon Lex returns the next message to convey to the user. Consider the following example messages:
*
*
* -
*
* For a user input "I would like a pizza," Amazon Lex might return a response with a message eliciting slot data
* (for example, PizzaSize
): "What size pizza would you like?".
*
*
* -
*
* After the user provides all of the pizza order information, Amazon Lex might return a response with a message to
* get user confirmation: "Order the pizza?".
*
*
* -
*
* After the user replies "Yes" to the confirmation prompt, Amazon Lex might return a conclusion statement:
* "Thank you, your cheese pizza has been ordered.".
*
*
*
*
* Not all Amazon Lex messages require a response from the user. For example, conclusion statements do not require a
* response. Some messages require only a yes or no response. In addition to the message
, Amazon Lex
* provides additional context about the message in the response that you can use to enhance client behavior, such
* as displaying the appropriate client user interface. Consider the following examples:
*
*
* -
*
* If the message is to elicit slot data, Amazon Lex returns the following context information:
*
*
* -
*
* x-amz-lex-dialog-state
header set to ElicitSlot
*
*
* -
*
* x-amz-lex-intent-name
header set to the intent name in the current context
*
*
* -
*
* x-amz-lex-slot-to-elicit
header set to the slot name for which the message
is eliciting
* information
*
*
* -
*
* x-amz-lex-slots
header set to a map of slots configured for the intent with their current values
*
*
*
*
* -
*
* If the message is a confirmation prompt, the x-amz-lex-dialog-state
header is set to
* Confirmation
and the x-amz-lex-slot-to-elicit
header is omitted.
*
*
* -
*
* If the message is a clarification prompt configured for the intent, indicating that the user intent is not
* understood, the x-amz-dialog-state
header is set to ElicitIntent
and the
* x-amz-slot-to-elicit
header is omitted.
*
*
*
*
* In addition, Amazon Lex also returns your application-specific sessionAttributes
. For more
* information, see Managing Conversation
* Context.
*
*
*
* This is a convenience which creates an instance of the {@link PostContentRequest.Builder} avoiding the need to
* create one manually via {@link PostContentRequest#builder()}
*
*
* @param postContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.PostContentRequest.Builder} to create a request.
* @param requestBody
* Functional interface that can be implemented to produce the request content in a non-blocking manner. The
* size of the content is expected to be known up front. See {@link AsyncRequestBody} for specific details on
* implementing this interface as well as links to precanned implementations for common scenarios like
* uploading from a file. The service documentation for the request content is as follows '
*
* User input in PCM or Opus audio format or text format as described in the Content-Type
HTTP
* header.
*
*
* You can stream audio data to Amazon Lex or you can create a local buffer that captures all of the audio
* data before sending. In general, you get better performance if you stream audio data rather than buffering
* the data locally.
*
* '
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The prompt (or statement) to convey to the user. This is based on the bot configuration and context. For
* example, if Amazon Lex did not understand the user intent, it sends the clarificationPrompt
* configured for the bot. If the intent requires confirmation before taking the fulfillment action, it sends
* the confirmationPrompt
. Another example: Suppose that the Lambda function successfully
* fulfilled the intent, and sent a message to convey to the user. Then Amazon Lex sends that message in the
* response.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - UnsupportedMediaTypeException The Content-Type header (
PostContent
API) has an invalid
* value.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - RequestTimeoutException The input speech is too long.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - LoopDetectedException This exception is not used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PostContent
* @see AWS API
* Documentation
*/
default CompletableFuture postContent(Consumer postContentRequest,
AsyncRequestBody requestBody, AsyncResponseTransformer asyncResponseTransformer) {
return postContent(PostContentRequest.builder().applyMutation(postContentRequest).build(), requestBody,
asyncResponseTransformer);
}
/**
*
* Sends user input (text or speech) to Amazon Lex. Clients use this API to send text and audio requests to Amazon
* Lex at runtime. Amazon Lex interprets the user input using the machine learning model that it built for the bot.
*
*
* The PostContent
operation supports audio input at 8kHz and 16kHz. You can use 8kHz audio to achieve
* higher speech recognition accuracy in telephone audio applications.
*
*
* In response, Amazon Lex returns the next message to convey to the user. Consider the following example messages:
*
*
* -
*
* For a user input "I would like a pizza," Amazon Lex might return a response with a message eliciting slot data
* (for example, PizzaSize
): "What size pizza would you like?".
*
*
* -
*
* After the user provides all of the pizza order information, Amazon Lex might return a response with a message to
* get user confirmation: "Order the pizza?".
*
*
* -
*
* After the user replies "Yes" to the confirmation prompt, Amazon Lex might return a conclusion statement:
* "Thank you, your cheese pizza has been ordered.".
*
*
*
*
* Not all Amazon Lex messages require a response from the user. For example, conclusion statements do not require a
* response. Some messages require only a yes or no response. In addition to the message
, Amazon Lex
* provides additional context about the message in the response that you can use to enhance client behavior, such
* as displaying the appropriate client user interface. Consider the following examples:
*
*
* -
*
* If the message is to elicit slot data, Amazon Lex returns the following context information:
*
*
* -
*
* x-amz-lex-dialog-state
header set to ElicitSlot
*
*
* -
*
* x-amz-lex-intent-name
header set to the intent name in the current context
*
*
* -
*
* x-amz-lex-slot-to-elicit
header set to the slot name for which the message
is eliciting
* information
*
*
* -
*
* x-amz-lex-slots
header set to a map of slots configured for the intent with their current values
*
*
*
*
* -
*
* If the message is a confirmation prompt, the x-amz-lex-dialog-state
header is set to
* Confirmation
and the x-amz-lex-slot-to-elicit
header is omitted.
*
*
* -
*
* If the message is a clarification prompt configured for the intent, indicating that the user intent is not
* understood, the x-amz-dialog-state
header is set to ElicitIntent
and the
* x-amz-slot-to-elicit
header is omitted.
*
*
*
*
* In addition, Amazon Lex also returns your application-specific sessionAttributes
. For more
* information, see Managing Conversation
* Context.
*
*
* @param postContentRequest
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* User input in PCM or Opus audio format or text format as described in the Content-Type
HTTP
* header.
*
*
* You can stream audio data to Amazon Lex or you can create a local buffer that captures all of the audio
* data before sending. In general, you get better performance if you stream audio data rather than buffering
* the data locally.
*
* '
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The prompt (or statement) to convey to the user. This is based on the bot configuration and context. For
* example, if Amazon Lex did not understand the user intent, it sends the clarificationPrompt
* configured for the bot. If the intent requires confirmation before taking the fulfillment action, it sends
* the confirmationPrompt
. Another example: Suppose that the Lambda function successfully
* fulfilled the intent, and sent a message to convey to the user. Then Amazon Lex sends that message in the
* response.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - UnsupportedMediaTypeException The Content-Type header (
PostContent
API) has an invalid
* value.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - RequestTimeoutException The input speech is too long.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - LoopDetectedException This exception is not used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PostContent
* @see AWS API
* Documentation
*/
default CompletableFuture postContent(PostContentRequest postContentRequest, Path sourcePath,
Path destinationPath) {
return postContent(postContentRequest, AsyncRequestBody.fromFile(sourcePath),
AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Sends user input (text or speech) to Amazon Lex. Clients use this API to send text and audio requests to Amazon
* Lex at runtime. Amazon Lex interprets the user input using the machine learning model that it built for the bot.
*
*
* The PostContent
operation supports audio input at 8kHz and 16kHz. You can use 8kHz audio to achieve
* higher speech recognition accuracy in telephone audio applications.
*
*
* In response, Amazon Lex returns the next message to convey to the user. Consider the following example messages:
*
*
* -
*
* For a user input "I would like a pizza," Amazon Lex might return a response with a message eliciting slot data
* (for example, PizzaSize
): "What size pizza would you like?".
*
*
* -
*
* After the user provides all of the pizza order information, Amazon Lex might return a response with a message to
* get user confirmation: "Order the pizza?".
*
*
* -
*
* After the user replies "Yes" to the confirmation prompt, Amazon Lex might return a conclusion statement:
* "Thank you, your cheese pizza has been ordered.".
*
*
*
*
* Not all Amazon Lex messages require a response from the user. For example, conclusion statements do not require a
* response. Some messages require only a yes or no response. In addition to the message
, Amazon Lex
* provides additional context about the message in the response that you can use to enhance client behavior, such
* as displaying the appropriate client user interface. Consider the following examples:
*
*
* -
*
* If the message is to elicit slot data, Amazon Lex returns the following context information:
*
*
* -
*
* x-amz-lex-dialog-state
header set to ElicitSlot
*
*
* -
*
* x-amz-lex-intent-name
header set to the intent name in the current context
*
*
* -
*
* x-amz-lex-slot-to-elicit
header set to the slot name for which the message
is eliciting
* information
*
*
* -
*
* x-amz-lex-slots
header set to a map of slots configured for the intent with their current values
*
*
*
*
* -
*
* If the message is a confirmation prompt, the x-amz-lex-dialog-state
header is set to
* Confirmation
and the x-amz-lex-slot-to-elicit
header is omitted.
*
*
* -
*
* If the message is a clarification prompt configured for the intent, indicating that the user intent is not
* understood, the x-amz-dialog-state
header is set to ElicitIntent
and the
* x-amz-slot-to-elicit
header is omitted.
*
*
*
*
* In addition, Amazon Lex also returns your application-specific sessionAttributes
. For more
* information, see Managing Conversation
* Context.
*
*
*
* This is a convenience which creates an instance of the {@link PostContentRequest.Builder} avoiding the need to
* create one manually via {@link PostContentRequest#builder()}
*
*
* @param postContentRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.PostContentRequest.Builder} to create a request.
* @param sourcePath
* {@link Path} to file containing data to send to the service. File will be read entirely and may be read
* multiple times in the event of a retry. If the file does not exist or the current user does not have
* access to read it then an exception will be thrown. The service documentation for the request content is
* as follows '
*
* User input in PCM or Opus audio format or text format as described in the Content-Type
HTTP
* header.
*
*
* You can stream audio data to Amazon Lex or you can create a local buffer that captures all of the audio
* data before sending. In general, you get better performance if you stream audio data rather than buffering
* the data locally.
*
* '
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The prompt (or statement) to convey to the user. This is based on the bot configuration and context. For
* example, if Amazon Lex did not understand the user intent, it sends the clarificationPrompt
* configured for the bot. If the intent requires confirmation before taking the fulfillment action, it sends
* the confirmationPrompt
. Another example: Suppose that the Lambda function successfully
* fulfilled the intent, and sent a message to convey to the user. Then Amazon Lex sends that message in the
* response.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - UnsupportedMediaTypeException The Content-Type header (
PostContent
API) has an invalid
* value.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - RequestTimeoutException The input speech is too long.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - LoopDetectedException This exception is not used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PostContent
* @see AWS API
* Documentation
*/
default CompletableFuture postContent(Consumer postContentRequest,
Path sourcePath, Path destinationPath) {
return postContent(PostContentRequest.builder().applyMutation(postContentRequest).build(), sourcePath, destinationPath);
}
/**
*
* Sends user input to Amazon Lex. Client applications can use this API to send requests to Amazon Lex at runtime.
* Amazon Lex then interprets the user input using the machine learning model it built for the bot.
*
*
* In response, Amazon Lex returns the next message
to convey to the user an optional
* responseCard
to display. Consider the following example messages:
*
*
* -
*
* For a user input "I would like a pizza", Amazon Lex might return a response with a message eliciting slot data
* (for example, PizzaSize): "What size pizza would you like?"
*
*
* -
*
* After the user provides all of the pizza order information, Amazon Lex might return a response with a message to
* obtain user confirmation "Proceed with the pizza order?".
*
*
* -
*
* After the user replies to a confirmation prompt with a "yes", Amazon Lex might return a conclusion statement:
* "Thank you, your cheese pizza has been ordered.".
*
*
*
*
* Not all Amazon Lex messages require a user response. For example, a conclusion statement does not require a
* response. Some messages require only a "yes" or "no" user response. In addition to the message
,
* Amazon Lex provides additional context about the message in the response that you might use to enhance client
* behavior, for example, to display the appropriate client user interface. These are the slotToElicit
,
* dialogState
, intentName
, and slots
fields in the response. Consider the
* following examples:
*
*
* -
*
* If the message is to elicit slot data, Amazon Lex returns the following context information:
*
*
* -
*
* dialogState
set to ElicitSlot
*
*
* -
*
* intentName
set to the intent name in the current context
*
*
* -
*
* slotToElicit
set to the slot name for which the message
is eliciting information
*
*
* -
*
* slots
set to a map of slots, configured for the intent, with currently known values
*
*
*
*
* -
*
* If the message is a confirmation prompt, the dialogState
is set to ConfirmIntent and
* SlotToElicit
is set to null.
*
*
* -
*
* If the message is a clarification prompt (configured for the intent) that indicates that user intent is not
* understood, the dialogState
is set to ElicitIntent and slotToElicit
is set to null.
*
*
*
*
* In addition, Amazon Lex also returns your application-specific sessionAttributes
. For more
* information, see Managing Conversation
* Context.
*
*
* @param postTextRequest
* @return A Java Future containing the result of the PostText operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - LoopDetectedException This exception is not used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PostText
* @see AWS API
* Documentation
*/
default CompletableFuture postText(PostTextRequest postTextRequest) {
throw new UnsupportedOperationException();
}
/**
*
* Sends user input to Amazon Lex. Client applications can use this API to send requests to Amazon Lex at runtime.
* Amazon Lex then interprets the user input using the machine learning model it built for the bot.
*
*
* In response, Amazon Lex returns the next message
to convey to the user an optional
* responseCard
to display. Consider the following example messages:
*
*
* -
*
* For a user input "I would like a pizza", Amazon Lex might return a response with a message eliciting slot data
* (for example, PizzaSize): "What size pizza would you like?"
*
*
* -
*
* After the user provides all of the pizza order information, Amazon Lex might return a response with a message to
* obtain user confirmation "Proceed with the pizza order?".
*
*
* -
*
* After the user replies to a confirmation prompt with a "yes", Amazon Lex might return a conclusion statement:
* "Thank you, your cheese pizza has been ordered.".
*
*
*
*
* Not all Amazon Lex messages require a user response. For example, a conclusion statement does not require a
* response. Some messages require only a "yes" or "no" user response. In addition to the message
,
* Amazon Lex provides additional context about the message in the response that you might use to enhance client
* behavior, for example, to display the appropriate client user interface. These are the slotToElicit
,
* dialogState
, intentName
, and slots
fields in the response. Consider the
* following examples:
*
*
* -
*
* If the message is to elicit slot data, Amazon Lex returns the following context information:
*
*
* -
*
* dialogState
set to ElicitSlot
*
*
* -
*
* intentName
set to the intent name in the current context
*
*
* -
*
* slotToElicit
set to the slot name for which the message
is eliciting information
*
*
* -
*
* slots
set to a map of slots, configured for the intent, with currently known values
*
*
*
*
* -
*
* If the message is a confirmation prompt, the dialogState
is set to ConfirmIntent and
* SlotToElicit
is set to null.
*
*
* -
*
* If the message is a clarification prompt (configured for the intent) that indicates that user intent is not
* understood, the dialogState
is set to ElicitIntent and slotToElicit
is set to null.
*
*
*
*
* In addition, Amazon Lex also returns your application-specific sessionAttributes
. For more
* information, see Managing Conversation
* Context.
*
*
*
* This is a convenience which creates an instance of the {@link PostTextRequest.Builder} avoiding the need to
* create one manually via {@link PostTextRequest#builder()}
*
*
* @param postTextRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.PostTextRequest.Builder} to create a request.
* @return A Java Future containing the result of the PostText operation returned by the service.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - LoopDetectedException This exception is not used.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PostText
* @see AWS API
* Documentation
*/
default CompletableFuture postText(Consumer postTextRequest) {
return postText(PostTextRequest.builder().applyMutation(postTextRequest).build());
}
/**
*
* Creates a new session or modifies an existing session with an Amazon Lex bot. Use this operation to enable your
* application to set the state of the bot.
*
*
* For more information, see Managing
* Sessions.
*
*
* @param putSessionRequest
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The audio version of the message to convey to the user.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PutSession
* @see AWS API
* Documentation
*/
default CompletableFuture putSession(PutSessionRequest putSessionRequest,
AsyncResponseTransformer asyncResponseTransformer) {
throw new UnsupportedOperationException();
}
/**
*
* Creates a new session or modifies an existing session with an Amazon Lex bot. Use this operation to enable your
* application to set the state of the bot.
*
*
* For more information, see Managing
* Sessions.
*
*
*
* This is a convenience which creates an instance of the {@link PutSessionRequest.Builder} avoiding the need to
* create one manually via {@link PutSessionRequest#builder()}
*
*
* @param putSessionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.PutSessionRequest.Builder} to create a request.
* @param asyncResponseTransformer
* The response transformer for processing the streaming response in a non-blocking manner. See
* {@link AsyncResponseTransformer} for details on how this callback should be implemented and for links to
* precanned implementations for common scenarios like downloading to a file. The service documentation for
* the response content is as follows '
*
* The audio version of the message to convey to the user.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PutSession
* @see AWS API
* Documentation
*/
default CompletableFuture putSession(Consumer putSessionRequest,
AsyncResponseTransformer asyncResponseTransformer) {
return putSession(PutSessionRequest.builder().applyMutation(putSessionRequest).build(), asyncResponseTransformer);
}
/**
*
* Creates a new session or modifies an existing session with an Amazon Lex bot. Use this operation to enable your
* application to set the state of the bot.
*
*
* For more information, see Managing
* Sessions.
*
*
* @param putSessionRequest
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The audio version of the message to convey to the user.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PutSession
* @see AWS API
* Documentation
*/
default CompletableFuture putSession(PutSessionRequest putSessionRequest, Path destinationPath) {
return putSession(putSessionRequest, AsyncResponseTransformer.toFile(destinationPath));
}
/**
*
* Creates a new session or modifies an existing session with an Amazon Lex bot. Use this operation to enable your
* application to set the state of the bot.
*
*
* For more information, see Managing
* Sessions.
*
*
*
* This is a convenience which creates an instance of the {@link PutSessionRequest.Builder} avoiding the need to
* create one manually via {@link PutSessionRequest#builder()}
*
*
* @param putSessionRequest
* A {@link Consumer} that will call methods on
* {@link software.amazon.awssdk.services.lexruntime.model.PutSessionRequest.Builder} to create a request.
* @param destinationPath
* {@link Path} to file that response contents will be written to. The file must not exist or this method
* will throw an exception. If the file is not writable by the current user then an exception will be thrown.
* The service documentation for the response content is as follows '
*
* The audio version of the message to convey to the user.
*
* '.
* @return A future to the transformed result of the AsyncResponseTransformer.
* The CompletableFuture returned by this method can be completed exceptionally with the following
* exceptions. The exception returned is wrapped with CompletionException, so you need to invoke
* {@link Throwable#getCause} to retrieve the underlying exception.
*
* - NotFoundException The resource (such as the Amazon Lex bot or an alias) that is referred to is not
* found.
* - BadRequestException Request validation failed, there is no usable message in the context, or the bot
* build failed, is still in progress, or contains unbuilt changes.
* - LimitExceededException Exceeded a limit.
* - InternalFailureException Internal service error. Retry the call.
* - ConflictException Two clients are using the same AWS account, Amazon Lex bot, and user ID.
* - NotAcceptableException The accept header in the request does not have a valid value.
* - DependencyFailedException One of the dependencies, such as AWS Lambda or Amazon Polly, threw an
* exception. For example,
*
* -
*
* If Amazon Lex does not have sufficient permissions to call a Lambda function.
*
*
* -
*
* If a Lambda function takes longer than 30 seconds to execute.
*
*
* -
*
* If a fulfillment Lambda function returns a Delegate
dialog action without removing any slot
* values.
*
*
* - BadGatewayException Either the Amazon Lex bot is still building, or one of the dependent services
* (Amazon Polly, AWS Lambda) failed with an internal service error.
* - SdkException Base class for all exceptions that can be thrown by the SDK (both service and client).
* Can be used for catch all scenarios.
* - SdkClientException If any client side error occurs such as an IO related failure, failure to get
* credentials, etc.
* - LexRuntimeException Base class for all service exceptions. Unknown exceptions will be thrown as an
* instance of this type.
*
* @sample LexRuntimeAsyncClient.PutSession
* @see AWS API
* Documentation
*/
default CompletableFuture putSession(Consumer putSessionRequest,
Path destinationPath) {
return putSession(PutSessionRequest.builder().applyMutation(putSessionRequest).build(), destinationPath);
}
@Override
default LexRuntimeServiceClientConfiguration serviceClientConfiguration() {
throw new UnsupportedOperationException();
}
/**
* Create a {@link LexRuntimeAsyncClient} with the region loaded from the
* {@link software.amazon.awssdk.regions.providers.DefaultAwsRegionProviderChain} and credentials loaded from the
* {@link software.amazon.awssdk.auth.credentials.DefaultCredentialsProvider}.
*/
static LexRuntimeAsyncClient create() {
return builder().build();
}
/**
* Create a builder that can be used to configure and create a {@link LexRuntimeAsyncClient}.
*/
static LexRuntimeAsyncClientBuilder builder() {
return new DefaultLexRuntimeAsyncClientBuilder();
}
}