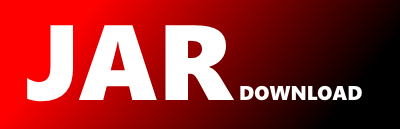
software.amazon.awssdk.services.lexruntime.model.PutSessionResponse Maven / Gradle / Ivy
Show all versions of lexruntime Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.lexruntime.model;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.JsonValueTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class PutSessionResponse extends LexRuntimeResponse implements
ToCopyableBuilder {
private static final SdkField CONTENT_TYPE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("contentType").getter(getter(PutSessionResponse::contentType)).setter(setter(Builder::contentType))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("Content-Type").build()).build();
private static final SdkField INTENT_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("intentName").getter(getter(PutSessionResponse::intentName)).setter(setter(Builder::intentName))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-intent-name").build())
.build();
private static final SdkField SLOTS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("slots")
.getter(getter(PutSessionResponse::slots))
.setter(setter(Builder::slots))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-slots").build(),
JsonValueTrait.create()).build();
private static final SdkField SESSION_ATTRIBUTES_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("sessionAttributes")
.getter(getter(PutSessionResponse::sessionAttributes))
.setter(setter(Builder::sessionAttributes))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-session-attributes")
.build(), JsonValueTrait.create()).build();
private static final SdkField MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING).memberName("message")
.getter(getter(PutSessionResponse::message)).setter(setter(Builder::message))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-message").build()).build();
private static final SdkField ENCODED_MESSAGE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("encodedMessage").getter(getter(PutSessionResponse::encodedMessage))
.setter(setter(Builder::encodedMessage))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-encoded-message").build())
.build();
private static final SdkField MESSAGE_FORMAT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("messageFormat").getter(getter(PutSessionResponse::messageFormatAsString))
.setter(setter(Builder::messageFormat))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-message-format").build())
.build();
private static final SdkField DIALOG_STATE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("dialogState").getter(getter(PutSessionResponse::dialogStateAsString))
.setter(setter(Builder::dialogState))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-dialog-state").build())
.build();
private static final SdkField SLOT_TO_ELICIT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("slotToElicit").getter(getter(PutSessionResponse::slotToElicit)).setter(setter(Builder::slotToElicit))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-slot-to-elicit").build())
.build();
private static final SdkField SESSION_ID_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("sessionId").getter(getter(PutSessionResponse::sessionId)).setter(setter(Builder::sessionId))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-session-id").build())
.build();
private static final SdkField ACTIVE_CONTEXTS_FIELD = SdkField
. builder(MarshallingType.STRING)
.memberName("activeContexts")
.getter(getter(PutSessionResponse::activeContexts))
.setter(setter(Builder::activeContexts))
.traits(LocationTrait.builder().location(MarshallLocation.HEADER).locationName("x-amz-lex-active-contexts").build(),
JsonValueTrait.create()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CONTENT_TYPE_FIELD,
INTENT_NAME_FIELD, SLOTS_FIELD, SESSION_ATTRIBUTES_FIELD, MESSAGE_FIELD, ENCODED_MESSAGE_FIELD, MESSAGE_FORMAT_FIELD,
DIALOG_STATE_FIELD, SLOT_TO_ELICIT_FIELD, SESSION_ID_FIELD, ACTIVE_CONTEXTS_FIELD));
private static final Map> SDK_NAME_TO_FIELD = memberNameToFieldInitializer();
private final String contentType;
private final String intentName;
private final String slots;
private final String sessionAttributes;
private final String message;
private final String encodedMessage;
private final String messageFormat;
private final String dialogState;
private final String slotToElicit;
private final String sessionId;
private final String activeContexts;
private PutSessionResponse(BuilderImpl builder) {
super(builder);
this.contentType = builder.contentType;
this.intentName = builder.intentName;
this.slots = builder.slots;
this.sessionAttributes = builder.sessionAttributes;
this.message = builder.message;
this.encodedMessage = builder.encodedMessage;
this.messageFormat = builder.messageFormat;
this.dialogState = builder.dialogState;
this.slotToElicit = builder.slotToElicit;
this.sessionId = builder.sessionId;
this.activeContexts = builder.activeContexts;
}
/**
*
* Content type as specified in the Accept
HTTP header in the request.
*
*
* @return Content type as specified in the Accept
HTTP header in the request.
*/
public final String contentType() {
return contentType;
}
/**
*
* The name of the current intent.
*
*
* @return The name of the current intent.
*/
public final String intentName() {
return intentName;
}
/**
*
* Map of zero or more intent slots Amazon Lex detected from the user input during the conversation.
*
*
* Amazon Lex creates a resolution list containing likely values for a slot. The value that it returns is determined
* by the valueSelectionStrategy
selected when the slot type was created or updated. If
* valueSelectionStrategy
is set to ORIGINAL_VALUE
, the value provided by the user is
* returned, if the user value is similar to the slot values. If valueSelectionStrategy
is set to
* TOP_RESOLUTION
Amazon Lex returns the first value in the resolution list or, if there is no
* resolution list, null. If you don't specify a valueSelectionStrategy
the default is
* ORIGINAL_VALUE
.
*
*
* @return Map of zero or more intent slots Amazon Lex detected from the user input during the conversation.
*
* Amazon Lex creates a resolution list containing likely values for a slot. The value that it returns is
* determined by the valueSelectionStrategy
selected when the slot type was created or updated.
* If valueSelectionStrategy
is set to ORIGINAL_VALUE
, the value provided by the
* user is returned, if the user value is similar to the slot values. If valueSelectionStrategy
* is set to TOP_RESOLUTION
Amazon Lex returns the first value in the resolution list or, if
* there is no resolution list, null. If you don't specify a valueSelectionStrategy
the default
* is ORIGINAL_VALUE
.
*/
public final String slots() {
return slots;
}
/**
*
* Map of key/value pairs representing session-specific context information.
*
*
* @return Map of key/value pairs representing session-specific context information.
*/
public final String sessionAttributes() {
return sessionAttributes;
}
/**
*
* The next message that should be presented to the user.
*
*
* You can only use this field in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR, and it-IT
* locales. In all other locales, the message
field is null. You should use the
* encodedMessage
field instead.
*
*
* @return The next message that should be presented to the user.
*
* You can only use this field in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR, and
* it-IT locales. In all other locales, the message
field is null. You should use the
* encodedMessage
field instead.
* @deprecated The message field is deprecated, use the encodedMessage field instead. The message field is available
* only in the de-DE, en-AU, en-GB, en-US, es-419, es-ES, es-US, fr-CA, fr-FR and it-IT locales.
*/
@Deprecated
public final String message() {
return message;
}
/**
*
* The next message that should be presented to the user.
*
*
* The encodedMessage
field is base-64 encoded. You must decode the field before you can use the value.
*
*
* @return The next message that should be presented to the user.
*
* The encodedMessage
field is base-64 encoded. You must decode the field before you can use
* the value.
*/
public final String encodedMessage() {
return encodedMessage;
}
/**
*
* The format of the response message. One of the following values:
*
*
* -
*
* PlainText
- The message contains plain UTF-8 text.
*
*
* -
*
* CustomPayload
- The message is a custom format for the client.
*
*
* -
*
* SSML
- The message contains text formatted for voice output.
*
*
* -
*
* Composite
- The message contains an escaped JSON object containing one or more messages from the
* groups that messages were assigned to when the intent was created.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #messageFormat}
* will return {@link MessageFormatType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #messageFormatAsString}.
*
*
* @return The format of the response message. One of the following values:
*
* -
*
* PlainText
- The message contains plain UTF-8 text.
*
*
* -
*
* CustomPayload
- The message is a custom format for the client.
*
*
* -
*
* SSML
- The message contains text formatted for voice output.
*
*
* -
*
* Composite
- The message contains an escaped JSON object containing one or more messages from
* the groups that messages were assigned to when the intent was created.
*
*
* @see MessageFormatType
*/
public final MessageFormatType messageFormat() {
return MessageFormatType.fromValue(messageFormat);
}
/**
*
* The format of the response message. One of the following values:
*
*
* -
*
* PlainText
- The message contains plain UTF-8 text.
*
*
* -
*
* CustomPayload
- The message is a custom format for the client.
*
*
* -
*
* SSML
- The message contains text formatted for voice output.
*
*
* -
*
* Composite
- The message contains an escaped JSON object containing one or more messages from the
* groups that messages were assigned to when the intent was created.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #messageFormat}
* will return {@link MessageFormatType#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available
* from {@link #messageFormatAsString}.
*
*
* @return The format of the response message. One of the following values:
*
* -
*
* PlainText
- The message contains plain UTF-8 text.
*
*
* -
*
* CustomPayload
- The message is a custom format for the client.
*
*
* -
*
* SSML
- The message contains text formatted for voice output.
*
*
* -
*
* Composite
- The message contains an escaped JSON object containing one or more messages from
* the groups that messages were assigned to when the intent was created.
*
*
* @see MessageFormatType
*/
public final String messageFormatAsString() {
return messageFormat;
}
/**
*
*
* -
*
* ConfirmIntent
- Amazon Lex is expecting a "yes" or "no" response to confirm the intent before
* fulfilling an intent.
*
*
* -
*
* ElicitIntent
- Amazon Lex wants to elicit the user's intent.
*
*
* -
*
* ElicitSlot
- Amazon Lex is expecting the value of a slot for the current intent.
*
*
* -
*
* Failed
- Conveys that the conversation with the user has failed. This can happen for various
* reasons, including the user does not provide an appropriate response to prompts from the service, or if the
* Lambda function fails to fulfill the intent.
*
*
* -
*
* Fulfilled
- Conveys that the Lambda function has sucessfully fulfilled the intent.
*
*
* -
*
* ReadyForFulfillment
- Conveys that the client has to fulfill the intent.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dialogState} will
* return {@link DialogState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dialogStateAsString}.
*
*
* @return
* -
*
* ConfirmIntent
- Amazon Lex is expecting a "yes" or "no" response to confirm the intent
* before fulfilling an intent.
*
*
* -
*
* ElicitIntent
- Amazon Lex wants to elicit the user's intent.
*
*
* -
*
* ElicitSlot
- Amazon Lex is expecting the value of a slot for the current intent.
*
*
* -
*
* Failed
- Conveys that the conversation with the user has failed. This can happen for various
* reasons, including the user does not provide an appropriate response to prompts from the service, or if
* the Lambda function fails to fulfill the intent.
*
*
* -
*
* Fulfilled
- Conveys that the Lambda function has sucessfully fulfilled the intent.
*
*
* -
*
* ReadyForFulfillment
- Conveys that the client has to fulfill the intent.
*
*
* @see DialogState
*/
public final DialogState dialogState() {
return DialogState.fromValue(dialogState);
}
/**
*
*
* -
*
* ConfirmIntent
- Amazon Lex is expecting a "yes" or "no" response to confirm the intent before
* fulfilling an intent.
*
*
* -
*
* ElicitIntent
- Amazon Lex wants to elicit the user's intent.
*
*
* -
*
* ElicitSlot
- Amazon Lex is expecting the value of a slot for the current intent.
*
*
* -
*
* Failed
- Conveys that the conversation with the user has failed. This can happen for various
* reasons, including the user does not provide an appropriate response to prompts from the service, or if the
* Lambda function fails to fulfill the intent.
*
*
* -
*
* Fulfilled
- Conveys that the Lambda function has sucessfully fulfilled the intent.
*
*
* -
*
* ReadyForFulfillment
- Conveys that the client has to fulfill the intent.
*
*
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #dialogState} will
* return {@link DialogState#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #dialogStateAsString}.
*
*
* @return
* -
*
* ConfirmIntent
- Amazon Lex is expecting a "yes" or "no" response to confirm the intent
* before fulfilling an intent.
*
*
* -
*
* ElicitIntent
- Amazon Lex wants to elicit the user's intent.
*
*
* -
*
* ElicitSlot
- Amazon Lex is expecting the value of a slot for the current intent.
*
*
* -
*
* Failed
- Conveys that the conversation with the user has failed. This can happen for various
* reasons, including the user does not provide an appropriate response to prompts from the service, or if
* the Lambda function fails to fulfill the intent.
*
*
* -
*
* Fulfilled
- Conveys that the Lambda function has sucessfully fulfilled the intent.
*
*
* -
*
* ReadyForFulfillment
- Conveys that the client has to fulfill the intent.
*
*
* @see DialogState
*/
public final String dialogStateAsString() {
return dialogState;
}
/**
*
* If the dialogState
is ElicitSlot
, returns the name of the slot for which Amazon Lex is
* eliciting a value.
*
*
* @return If the dialogState
is ElicitSlot
, returns the name of the slot for which Amazon
* Lex is eliciting a value.
*/
public final String slotToElicit() {
return slotToElicit;
}
/**
*
* A unique identifier for the session.
*
*
* @return A unique identifier for the session.
*/
public final String sessionId() {
return sessionId;
}
/**
*
* A list of active contexts for the session.
*
*
* @return A list of active contexts for the session.
*/
public final String activeContexts() {
return activeContexts;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(contentType());
hashCode = 31 * hashCode + Objects.hashCode(intentName());
hashCode = 31 * hashCode + Objects.hashCode(slots());
hashCode = 31 * hashCode + Objects.hashCode(sessionAttributes());
hashCode = 31 * hashCode + Objects.hashCode(message());
hashCode = 31 * hashCode + Objects.hashCode(encodedMessage());
hashCode = 31 * hashCode + Objects.hashCode(messageFormatAsString());
hashCode = 31 * hashCode + Objects.hashCode(dialogStateAsString());
hashCode = 31 * hashCode + Objects.hashCode(slotToElicit());
hashCode = 31 * hashCode + Objects.hashCode(sessionId());
hashCode = 31 * hashCode + Objects.hashCode(activeContexts());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof PutSessionResponse)) {
return false;
}
PutSessionResponse other = (PutSessionResponse) obj;
return Objects.equals(contentType(), other.contentType()) && Objects.equals(intentName(), other.intentName())
&& Objects.equals(slots(), other.slots()) && Objects.equals(sessionAttributes(), other.sessionAttributes())
&& Objects.equals(message(), other.message()) && Objects.equals(encodedMessage(), other.encodedMessage())
&& Objects.equals(messageFormatAsString(), other.messageFormatAsString())
&& Objects.equals(dialogStateAsString(), other.dialogStateAsString())
&& Objects.equals(slotToElicit(), other.slotToElicit()) && Objects.equals(sessionId(), other.sessionId())
&& Objects.equals(activeContexts(), other.activeContexts());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("PutSessionResponse").add("ContentType", contentType()).add("IntentName", intentName())
.add("Slots", slots()).add("SessionAttributes", sessionAttributes())
.add("Message", message() == null ? null : "*** Sensitive Data Redacted ***")
.add("EncodedMessage", encodedMessage() == null ? null : "*** Sensitive Data Redacted ***")
.add("MessageFormat", messageFormatAsString()).add("DialogState", dialogStateAsString())
.add("SlotToElicit", slotToElicit()).add("SessionId", sessionId())
.add("ActiveContexts", activeContexts() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "contentType":
return Optional.ofNullable(clazz.cast(contentType()));
case "intentName":
return Optional.ofNullable(clazz.cast(intentName()));
case "slots":
return Optional.ofNullable(clazz.cast(slots()));
case "sessionAttributes":
return Optional.ofNullable(clazz.cast(sessionAttributes()));
case "message":
return Optional.ofNullable(clazz.cast(message()));
case "encodedMessage":
return Optional.ofNullable(clazz.cast(encodedMessage()));
case "messageFormat":
return Optional.ofNullable(clazz.cast(messageFormatAsString()));
case "dialogState":
return Optional.ofNullable(clazz.cast(dialogStateAsString()));
case "slotToElicit":
return Optional.ofNullable(clazz.cast(slotToElicit()));
case "sessionId":
return Optional.ofNullable(clazz.cast(sessionId()));
case "activeContexts":
return Optional.ofNullable(clazz.cast(activeContexts()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
@Override
public final Map> sdkFieldNameToField() {
return SDK_NAME_TO_FIELD;
}
private static Map> memberNameToFieldInitializer() {
Map> map = new HashMap<>();
map.put("Content-Type", CONTENT_TYPE_FIELD);
map.put("x-amz-lex-intent-name", INTENT_NAME_FIELD);
map.put("x-amz-lex-slots", SLOTS_FIELD);
map.put("x-amz-lex-session-attributes", SESSION_ATTRIBUTES_FIELD);
map.put("x-amz-lex-message", MESSAGE_FIELD);
map.put("x-amz-lex-encoded-message", ENCODED_MESSAGE_FIELD);
map.put("x-amz-lex-message-format", MESSAGE_FORMAT_FIELD);
map.put("x-amz-lex-dialog-state", DIALOG_STATE_FIELD);
map.put("x-amz-lex-slot-to-elicit", SLOT_TO_ELICIT_FIELD);
map.put("x-amz-lex-session-id", SESSION_ID_FIELD);
map.put("x-amz-lex-active-contexts", ACTIVE_CONTEXTS_FIELD);
return Collections.unmodifiableMap(map);
}
private static Function