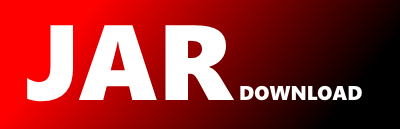
software.amazon.awssdk.services.location.model.Leg Maven / Gradle / Ivy
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.location.model;
import java.beans.Transient;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* Contains the calculated route's details for each path between a pair of positions. The number of legs returned
* corresponds to one fewer than the total number of positions in the request.
*
*
* For example, a route with a departure position and destination position returns one leg with the positions snapped to a nearby
* road:
*
*
* -
*
* The StartPosition
is the departure position.
*
*
* -
*
* The EndPosition
is the destination position.
*
*
*
*
* A route with a waypoint between the departure and destination position returns two legs with the positions snapped to
* a nearby road:
*
*
* -
*
* Leg 1: The StartPosition
is the departure position . The EndPosition
is the waypoint
* positon.
*
*
* -
*
* Leg 2: The StartPosition
is the waypoint position. The EndPosition
is the destination
* position.
*
*
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class Leg implements SdkPojo, Serializable, ToCopyableBuilder {
private static final SdkField DISTANCE_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("Distance").getter(getter(Leg::distance)).setter(setter(Builder::distance))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Distance").build()).build();
private static final SdkField DURATION_SECONDS_FIELD = SdkField. builder(MarshallingType.DOUBLE)
.memberName("DurationSeconds").getter(getter(Leg::durationSeconds)).setter(setter(Builder::durationSeconds))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DurationSeconds").build()).build();
private static final SdkField> END_POSITION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("EndPosition")
.getter(getter(Leg::endPosition))
.setter(setter(Builder::endPosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("EndPosition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField GEOMETRY_FIELD = SdkField. builder(MarshallingType.SDK_POJO)
.memberName("Geometry").getter(getter(Leg::geometry)).setter(setter(Builder::geometry))
.constructor(LegGeometry::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Geometry").build()).build();
private static final SdkField> START_POSITION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("StartPosition")
.getter(getter(Leg::startPosition))
.setter(setter(Builder::startPosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("StartPosition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField> STEPS_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("Steps")
.getter(getter(Leg::steps))
.setter(setter(Builder::steps))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("Steps").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.SDK_POJO)
.constructor(Step::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DISTANCE_FIELD,
DURATION_SECONDS_FIELD, END_POSITION_FIELD, GEOMETRY_FIELD, START_POSITION_FIELD, STEPS_FIELD));
private static final long serialVersionUID = 1L;
private final Double distance;
private final Double durationSeconds;
private final List endPosition;
private final LegGeometry geometry;
private final List startPosition;
private final List steps;
private Leg(BuilderImpl builder) {
this.distance = builder.distance;
this.durationSeconds = builder.durationSeconds;
this.endPosition = builder.endPosition;
this.geometry = builder.geometry;
this.startPosition = builder.startPosition;
this.steps = builder.steps;
}
/**
*
* The distance between the leg's StartPosition
and EndPosition
along a calculated route.
*
*
* -
*
* The default measurement is Kilometers
unless the request specifies a DistanceUnit
of
* Miles
.
*
*
*
*
* @return The distance between the leg's StartPosition
and EndPosition
along a calculated
* route.
*
* -
*
* The default measurement is Kilometers
unless the request specifies a
* DistanceUnit
of Miles
.
*
*
*/
public final Double distance() {
return distance;
}
/**
*
* The estimated travel time between the leg's StartPosition
and EndPosition
. The travel
* mode and departure time that you specify in the request determines the calculated time.
*
*
* @return The estimated travel time between the leg's StartPosition
and EndPosition
. The
* travel mode and departure time that you specify in the request determines the calculated time.
*/
public final Double durationSeconds() {
return durationSeconds;
}
/**
* For responses, this returns true if the service returned a value for the EndPosition property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasEndPosition() {
return endPosition != null && !(endPosition instanceof SdkAutoConstructList);
}
/**
*
* The terminating position of the leg. Follows the format [longitude,latitude]
.
*
*
*
* If the EndPosition
isn't located on a road, it's snapped to a nearby
* road.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasEndPosition} method.
*
*
* @return The terminating position of the leg. Follows the format [longitude,latitude]
.
*
* If the EndPosition
isn't located on a road, it's snapped to a
* nearby road.
*
*/
public final List endPosition() {
return endPosition;
}
/**
*
* Contains the calculated route's path as a linestring geometry.
*
*
* @return Contains the calculated route's path as a linestring geometry.
*/
public final LegGeometry geometry() {
return geometry;
}
/**
* For responses, this returns true if the service returned a value for the StartPosition property. This DOES NOT
* check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasStartPosition() {
return startPosition != null && !(startPosition instanceof SdkAutoConstructList);
}
/**
*
* The starting position of the leg. Follows the format [longitude,latitude]
.
*
*
*
* If the StartPosition
isn't located on a road, it's snapped to a nearby
* road.
*
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasStartPosition} method.
*
*
* @return The starting position of the leg. Follows the format [longitude,latitude]
.
*
* If the StartPosition
isn't located on a road, it's snapped to a
* nearby road.
*
*/
public final List startPosition() {
return startPosition;
}
/**
* For responses, this returns true if the service returned a value for the Steps property. This DOES NOT check that
* the value is non-empty (for which, you should check the {@code isEmpty()} method on the property). This is useful
* because the SDK will never return a null collection or map, but you may need to differentiate between the service
* returning nothing (or null) and the service returning an empty collection or map. For requests, this returns true
* if a value for the property was specified in the request builder, and false if a value was not specified.
*/
public final boolean hasSteps() {
return steps != null && !(steps instanceof SdkAutoConstructList);
}
/**
*
* Contains a list of steps, which represent subsections of a leg. Each step provides instructions for how to move
* to the next step in the leg such as the step's start position, end position, travel distance, travel duration,
* and geometry offset.
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasSteps} method.
*
*
* @return Contains a list of steps, which represent subsections of a leg. Each step provides instructions for how
* to move to the next step in the leg such as the step's start position, end position, travel distance,
* travel duration, and geometry offset.
*/
public final List steps() {
return steps;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(distance());
hashCode = 31 * hashCode + Objects.hashCode(durationSeconds());
hashCode = 31 * hashCode + Objects.hashCode(hasEndPosition() ? endPosition() : null);
hashCode = 31 * hashCode + Objects.hashCode(geometry());
hashCode = 31 * hashCode + Objects.hashCode(hasStartPosition() ? startPosition() : null);
hashCode = 31 * hashCode + Objects.hashCode(hasSteps() ? steps() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof Leg)) {
return false;
}
Leg other = (Leg) obj;
return Objects.equals(distance(), other.distance()) && Objects.equals(durationSeconds(), other.durationSeconds())
&& hasEndPosition() == other.hasEndPosition() && Objects.equals(endPosition(), other.endPosition())
&& Objects.equals(geometry(), other.geometry()) && hasStartPosition() == other.hasStartPosition()
&& Objects.equals(startPosition(), other.startPosition()) && hasSteps() == other.hasSteps()
&& Objects.equals(steps(), other.steps());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("Leg").add("Distance", distance()).add("DurationSeconds", durationSeconds())
.add("EndPosition", endPosition() == null ? null : "*** Sensitive Data Redacted ***").add("Geometry", geometry())
.add("StartPosition", startPosition() == null ? null : "*** Sensitive Data Redacted ***")
.add("Steps", hasSteps() ? steps() : null).build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "Distance":
return Optional.ofNullable(clazz.cast(distance()));
case "DurationSeconds":
return Optional.ofNullable(clazz.cast(durationSeconds()));
case "EndPosition":
return Optional.ofNullable(clazz.cast(endPosition()));
case "Geometry":
return Optional.ofNullable(clazz.cast(geometry()));
case "StartPosition":
return Optional.ofNullable(clazz.cast(startPosition()));
case "Steps":
return Optional.ofNullable(clazz.cast(steps()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function