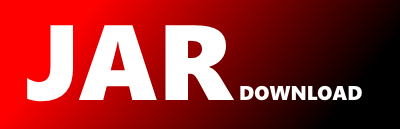
software.amazon.awssdk.services.location.model.CalculateRouteMatrixSummary Maven / Gradle / Ivy
Show all versions of location Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.location.model;
import java.io.Serializable;
import java.util.Arrays;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*
* A summary of the calculated route matrix.
*
*/
@Generated("software.amazon.awssdk:codegen")
public final class CalculateRouteMatrixSummary implements SdkPojo, Serializable,
ToCopyableBuilder {
private static final SdkField DATA_SOURCE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DataSource").getter(getter(CalculateRouteMatrixSummary::dataSource)).setter(setter(Builder::dataSource))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DataSource").build()).build();
private static final SdkField DISTANCE_UNIT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DistanceUnit").getter(getter(CalculateRouteMatrixSummary::distanceUnitAsString))
.setter(setter(Builder::distanceUnit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DistanceUnit").build()).build();
private static final SdkField ERROR_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("ErrorCount").getter(getter(CalculateRouteMatrixSummary::errorCount)).setter(setter(Builder::errorCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("ErrorCount").build()).build();
private static final SdkField ROUTE_COUNT_FIELD = SdkField. builder(MarshallingType.INTEGER)
.memberName("RouteCount").getter(getter(CalculateRouteMatrixSummary::routeCount)).setter(setter(Builder::routeCount))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("RouteCount").build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(DATA_SOURCE_FIELD,
DISTANCE_UNIT_FIELD, ERROR_COUNT_FIELD, ROUTE_COUNT_FIELD));
private static final long serialVersionUID = 1L;
private final String dataSource;
private final String distanceUnit;
private final Integer errorCount;
private final Integer routeCount;
private CalculateRouteMatrixSummary(BuilderImpl builder) {
this.dataSource = builder.dataSource;
this.distanceUnit = builder.distanceUnit;
this.errorCount = builder.errorCount;
this.routeCount = builder.routeCount;
}
/**
*
* The data provider of traffic and road network data used to calculate the routes. Indicates one of the available
* providers:
*
*
* -
*
* Esri
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon Location
* Service data providers.
*
*
* @return The data provider of traffic and road network data used to calculate the routes. Indicates one of the
* available providers:
*
* -
*
* Esri
*
*
* -
*
* Here
*
*
*
*
* For more information about data providers, see Amazon
* Location Service data providers.
*/
public final String dataSource() {
return dataSource;
}
/**
*
* The unit of measurement for route distances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #distanceUnit} will
* return {@link DistanceUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #distanceUnitAsString}.
*
*
* @return The unit of measurement for route distances.
* @see DistanceUnit
*/
public final DistanceUnit distanceUnit() {
return DistanceUnit.fromValue(distanceUnit);
}
/**
*
* The unit of measurement for route distances.
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #distanceUnit} will
* return {@link DistanceUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #distanceUnitAsString}.
*
*
* @return The unit of measurement for route distances.
* @see DistanceUnit
*/
public final String distanceUnitAsString() {
return distanceUnit;
}
/**
*
* The count of error results in the route matrix. If this number is 0, all routes were calculated successfully.
*
*
* @return The count of error results in the route matrix. If this number is 0, all routes were calculated
* successfully.
*/
public final Integer errorCount() {
return errorCount;
}
/**
*
* The count of cells in the route matrix. Equal to the number of DeparturePositions
multiplied by the
* number of DestinationPositions
.
*
*
* @return The count of cells in the route matrix. Equal to the number of DeparturePositions
multiplied
* by the number of DestinationPositions
.
*/
public final Integer routeCount() {
return routeCount;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + Objects.hashCode(dataSource());
hashCode = 31 * hashCode + Objects.hashCode(distanceUnitAsString());
hashCode = 31 * hashCode + Objects.hashCode(errorCount());
hashCode = 31 * hashCode + Objects.hashCode(routeCount());
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CalculateRouteMatrixSummary)) {
return false;
}
CalculateRouteMatrixSummary other = (CalculateRouteMatrixSummary) obj;
return Objects.equals(dataSource(), other.dataSource())
&& Objects.equals(distanceUnitAsString(), other.distanceUnitAsString())
&& Objects.equals(errorCount(), other.errorCount()) && Objects.equals(routeCount(), other.routeCount());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CalculateRouteMatrixSummary").add("DataSource", dataSource())
.add("DistanceUnit", distanceUnitAsString()).add("ErrorCount", errorCount()).add("RouteCount", routeCount())
.build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "DataSource":
return Optional.ofNullable(clazz.cast(dataSource()));
case "DistanceUnit":
return Optional.ofNullable(clazz.cast(distanceUnitAsString()));
case "ErrorCount":
return Optional.ofNullable(clazz.cast(errorCount()));
case "RouteCount":
return Optional.ofNullable(clazz.cast(routeCount()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function