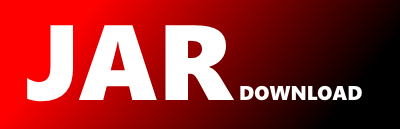
software.amazon.awssdk.services.location.model.CalculateRouteRequest Maven / Gradle / Ivy
Show all versions of location Show documentation
/*
* Copyright Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package software.amazon.awssdk.services.location.model;
import java.time.Instant;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Function;
import software.amazon.awssdk.annotations.Generated;
import software.amazon.awssdk.awscore.AwsRequestOverrideConfiguration;
import software.amazon.awssdk.core.SdkField;
import software.amazon.awssdk.core.SdkPojo;
import software.amazon.awssdk.core.protocol.MarshallLocation;
import software.amazon.awssdk.core.protocol.MarshallingType;
import software.amazon.awssdk.core.traits.ListTrait;
import software.amazon.awssdk.core.traits.LocationTrait;
import software.amazon.awssdk.core.traits.TimestampFormatTrait;
import software.amazon.awssdk.core.util.DefaultSdkAutoConstructList;
import software.amazon.awssdk.core.util.SdkAutoConstructList;
import software.amazon.awssdk.utils.ToString;
import software.amazon.awssdk.utils.builder.CopyableBuilder;
import software.amazon.awssdk.utils.builder.ToCopyableBuilder;
/**
*/
@Generated("software.amazon.awssdk:codegen")
public final class CalculateRouteRequest extends LocationRequest implements
ToCopyableBuilder {
private static final SdkField CALCULATOR_NAME_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("CalculatorName").getter(getter(CalculateRouteRequest::calculatorName))
.setter(setter(Builder::calculatorName))
.traits(LocationTrait.builder().location(MarshallLocation.PATH).locationName("CalculatorName").build()).build();
private static final SdkField CAR_MODE_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("CarModeOptions")
.getter(getter(CalculateRouteRequest::carModeOptions)).setter(setter(Builder::carModeOptions))
.constructor(CalculateRouteCarModeOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("CarModeOptions").build()).build();
private static final SdkField DEPART_NOW_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("DepartNow").getter(getter(CalculateRouteRequest::departNow)).setter(setter(Builder::departNow))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DepartNow").build()).build();
private static final SdkField> DEPARTURE_POSITION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DeparturePosition")
.getter(getter(CalculateRouteRequest::departurePosition))
.setter(setter(Builder::departurePosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DeparturePosition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DEPARTURE_TIME_FIELD = SdkField
. builder(MarshallingType.INSTANT)
.memberName("DepartureTime")
.getter(getter(CalculateRouteRequest::departureTime))
.setter(setter(Builder::departureTime))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DepartureTime").build(),
TimestampFormatTrait.create(TimestampFormatTrait.Format.ISO_8601)).build();
private static final SdkField> DESTINATION_POSITION_FIELD = SdkField
.> builder(MarshallingType.LIST)
.memberName("DestinationPosition")
.getter(getter(CalculateRouteRequest::destinationPosition))
.setter(setter(Builder::destinationPosition))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DestinationPosition").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build()).build()).build();
private static final SdkField DISTANCE_UNIT_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("DistanceUnit").getter(getter(CalculateRouteRequest::distanceUnitAsString))
.setter(setter(Builder::distanceUnit))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("DistanceUnit").build()).build();
private static final SdkField INCLUDE_LEG_GEOMETRY_FIELD = SdkField. builder(MarshallingType.BOOLEAN)
.memberName("IncludeLegGeometry").getter(getter(CalculateRouteRequest::includeLegGeometry))
.setter(setter(Builder::includeLegGeometry))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("IncludeLegGeometry").build())
.build();
private static final SdkField TRAVEL_MODE_FIELD = SdkField. builder(MarshallingType.STRING)
.memberName("TravelMode").getter(getter(CalculateRouteRequest::travelModeAsString))
.setter(setter(Builder::travelMode))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TravelMode").build()).build();
private static final SdkField TRUCK_MODE_OPTIONS_FIELD = SdkField
. builder(MarshallingType.SDK_POJO).memberName("TruckModeOptions")
.getter(getter(CalculateRouteRequest::truckModeOptions)).setter(setter(Builder::truckModeOptions))
.constructor(CalculateRouteTruckModeOptions::builder)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("TruckModeOptions").build()).build();
private static final SdkField>> WAYPOINT_POSITIONS_FIELD = SdkField
.>> builder(MarshallingType.LIST)
.memberName("WaypointPositions")
.getter(getter(CalculateRouteRequest::waypointPositions))
.setter(setter(Builder::waypointPositions))
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD).locationName("WaypointPositions").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField.> builder(MarshallingType.LIST)
.traits(LocationTrait.builder().location(MarshallLocation.PAYLOAD)
.locationName("member").build(),
ListTrait
.builder()
.memberLocationName(null)
.memberFieldInfo(
SdkField. builder(MarshallingType.DOUBLE)
.traits(LocationTrait.builder()
.location(MarshallLocation.PAYLOAD)
.locationName("member").build()).build())
.build()).build()).build()).build();
private static final List> SDK_FIELDS = Collections.unmodifiableList(Arrays.asList(CALCULATOR_NAME_FIELD,
CAR_MODE_OPTIONS_FIELD, DEPART_NOW_FIELD, DEPARTURE_POSITION_FIELD, DEPARTURE_TIME_FIELD, DESTINATION_POSITION_FIELD,
DISTANCE_UNIT_FIELD, INCLUDE_LEG_GEOMETRY_FIELD, TRAVEL_MODE_FIELD, TRUCK_MODE_OPTIONS_FIELD,
WAYPOINT_POSITIONS_FIELD));
private final String calculatorName;
private final CalculateRouteCarModeOptions carModeOptions;
private final Boolean departNow;
private final List departurePosition;
private final Instant departureTime;
private final List destinationPosition;
private final String distanceUnit;
private final Boolean includeLegGeometry;
private final String travelMode;
private final CalculateRouteTruckModeOptions truckModeOptions;
private final List> waypointPositions;
private CalculateRouteRequest(BuilderImpl builder) {
super(builder);
this.calculatorName = builder.calculatorName;
this.carModeOptions = builder.carModeOptions;
this.departNow = builder.departNow;
this.departurePosition = builder.departurePosition;
this.departureTime = builder.departureTime;
this.destinationPosition = builder.destinationPosition;
this.distanceUnit = builder.distanceUnit;
this.includeLegGeometry = builder.includeLegGeometry;
this.travelMode = builder.travelMode;
this.truckModeOptions = builder.truckModeOptions;
this.waypointPositions = builder.waypointPositions;
}
/**
*
* The name of the route calculator resource that you want to use to calculate the route.
*
*
* @return The name of the route calculator resource that you want to use to calculate the route.
*/
public final String calculatorName() {
return calculatorName;
}
/**
*
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries or
* tolls.
*
*
* Requirements: TravelMode
must be specified as Car
.
*
*
* @return Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries
* or tolls.
*
* Requirements: TravelMode
must be specified as Car
.
*/
public final CalculateRouteCarModeOptions carModeOptions() {
return carModeOptions;
}
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate a route. Otherwise, the best
* time of day to travel with the best traffic conditions is used to calculate the route.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @return Sets the time of departure as the current time. Uses the current time to calculate a route. Otherwise,
* the best time of day to travel with the best traffic conditions is used to calculate the route.
*
* Default Value: false
*
*
* Valid Values: false
| true
*/
public final Boolean departNow() {
return departNow;
}
/**
* For responses, this returns true if the service returned a value for the DeparturePosition property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDeparturePosition() {
return departurePosition != null && !(departurePosition instanceof SdkAutoConstructList);
}
/**
*
* The start position for the route. Defined in WGS
* 84 format: [longitude, latitude]
.
*
*
* -
*
* For example, [-123.115, 49.285]
*
*
*
*
*
* If you specify a departure that's not located on a road, Amazon Location moves the position to
* the nearest road. If Esri is the provider for your route calculator, specifying a route that is longer than
* 400 km returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDeparturePosition} method.
*
*
* @return The start position for the route. Defined in WGS 84 format:
* [longitude, latitude]
.
*
* -
*
* For example, [-123.115, 49.285]
*
*
*
*
*
* If you specify a departure that's not located on a road, Amazon Location moves the
* position to the nearest road. If Esri is the provider for your route calculator, specifying a route
* that is longer than 400 km returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public final List departurePosition() {
return departurePosition;
}
/**
*
* Specifies the desired time of departure. Uses the given time to calculate the route. Otherwise, the best time of
* day to travel with the best traffic conditions is used to calculate the route.
*
*
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*
*
* @return Specifies the desired time of departure. Uses the given time to calculate the route. Otherwise, the best
* time of day to travel with the best traffic conditions is used to calculate the route.
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*/
public final Instant departureTime() {
return departureTime;
}
/**
* For responses, this returns true if the service returned a value for the DestinationPosition property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasDestinationPosition() {
return destinationPosition != null && !(destinationPosition instanceof SdkAutoConstructList);
}
/**
*
* The finish position for the route. Defined in WGS
* 84 format: [longitude, latitude]
.
*
*
* -
*
* For example, [-122.339, 47.615]
*
*
*
*
*
* If you specify a destination that's not located on a road, Amazon Location moves the position to
* the nearest road.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasDestinationPosition} method.
*
*
* @return The finish position for the route. Defined in WGS 84 format:
* [longitude, latitude]
.
*
* -
*
* For example, [-122.339, 47.615]
*
*
*
*
*
* If you specify a destination that's not located on a road, Amazon Location moves the
* position to the nearest road.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public final List destinationPosition() {
return destinationPosition;
}
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #distanceUnit} will
* return {@link DistanceUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #distanceUnitAsString}.
*
*
* @return Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @see DistanceUnit
*/
public final DistanceUnit distanceUnit() {
return DistanceUnit.fromValue(distanceUnit);
}
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #distanceUnit} will
* return {@link DistanceUnit#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #distanceUnitAsString}.
*
*
* @return Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @see DistanceUnit
*/
public final String distanceUnitAsString() {
return distanceUnit;
}
/**
*
* Set to include the geometry details in the result for each path between a pair of positions.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @return Set to include the geometry details in the result for each path between a pair of positions.
*
* Default Value: false
*
*
* Valid Values: false
| true
*/
public final Boolean includeLegGeometry() {
return includeLegGeometry;
}
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #travelMode} will
* return {@link TravelMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #travelModeAsString}.
*
*
* @return Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
* @see TravelMode
*/
public final TravelMode travelMode() {
return TravelMode.fromValue(travelMode);
}
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
*
*
* If the service returns an enum value that is not available in the current SDK version, {@link #travelMode} will
* return {@link TravelMode#UNKNOWN_TO_SDK_VERSION}. The raw value returned by the service is available from
* {@link #travelModeAsString}.
*
*
* @return Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
* @see TravelMode
*/
public final String travelModeAsString() {
return travelMode;
}
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
*
* @return Specifies route preferences when traveling by Truck
, such as avoiding routes that use
* ferries or tolls, and truck specifications to consider when choosing an optimal road.
*
* Requirements: TravelMode
must be specified as Truck
.
*/
public final CalculateRouteTruckModeOptions truckModeOptions() {
return truckModeOptions;
}
/**
* For responses, this returns true if the service returned a value for the WaypointPositions property. This DOES
* NOT check that the value is non-empty (for which, you should check the {@code isEmpty()} method on the property).
* This is useful because the SDK will never return a null collection or map, but you may need to differentiate
* between the service returning nothing (or null) and the service returning an empty collection or map. For
* requests, this returns true if a value for the property was specified in the request builder, and false if a
* value was not specified.
*/
public final boolean hasWaypointPositions() {
return waypointPositions != null && !(waypointPositions instanceof SdkAutoConstructList);
}
/**
*
* Specifies an ordered list of up to 23 intermediate positions to include along a route between the departure
* position and destination position.
*
*
* -
*
* For example, from the DeparturePosition
[-123.115, 49.285]
, the route follows the order
* that the waypoint positions are given [[-122.757, 49.0021],[-122.349, 47.620]]
*
*
*
*
*
* If you specify a waypoint position that's not located on a road, Amazon Location moves the position to
* the nearest road.
*
*
* Specifying more than 23 waypoints returns a 400 ValidationException
error.
*
*
* If Esri is the provider for your route calculator, specifying a route that is longer than 400 km returns a
* 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* Attempts to modify the collection returned by this method will result in an UnsupportedOperationException.
*
*
* This method will never return null. If you would like to know whether the service returned this field (so that
* you can differentiate between null and empty), you can use the {@link #hasWaypointPositions} method.
*
*
* @return Specifies an ordered list of up to 23 intermediate positions to include along a route between the
* departure position and destination position.
*
* -
*
* For example, from the DeparturePosition
[-123.115, 49.285]
, the route follows
* the order that the waypoint positions are given [[-122.757, 49.0021],[-122.349, 47.620]]
*
*
*
*
*
* If you specify a waypoint position that's not located on a road, Amazon Location moves the
* position to the nearest road.
*
*
* Specifying more than 23 waypoints returns a 400 ValidationException
error.
*
*
* If Esri is the provider for your route calculator, specifying a route that is longer than 400 km returns
* a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*/
public final List> waypointPositions() {
return waypointPositions;
}
@Override
public Builder toBuilder() {
return new BuilderImpl(this);
}
public static Builder builder() {
return new BuilderImpl();
}
public static Class extends Builder> serializableBuilderClass() {
return BuilderImpl.class;
}
@Override
public final int hashCode() {
int hashCode = 1;
hashCode = 31 * hashCode + super.hashCode();
hashCode = 31 * hashCode + Objects.hashCode(calculatorName());
hashCode = 31 * hashCode + Objects.hashCode(carModeOptions());
hashCode = 31 * hashCode + Objects.hashCode(departNow());
hashCode = 31 * hashCode + Objects.hashCode(hasDeparturePosition() ? departurePosition() : null);
hashCode = 31 * hashCode + Objects.hashCode(departureTime());
hashCode = 31 * hashCode + Objects.hashCode(hasDestinationPosition() ? destinationPosition() : null);
hashCode = 31 * hashCode + Objects.hashCode(distanceUnitAsString());
hashCode = 31 * hashCode + Objects.hashCode(includeLegGeometry());
hashCode = 31 * hashCode + Objects.hashCode(travelModeAsString());
hashCode = 31 * hashCode + Objects.hashCode(truckModeOptions());
hashCode = 31 * hashCode + Objects.hashCode(hasWaypointPositions() ? waypointPositions() : null);
return hashCode;
}
@Override
public final boolean equals(Object obj) {
return super.equals(obj) && equalsBySdkFields(obj);
}
@Override
public final boolean equalsBySdkFields(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (!(obj instanceof CalculateRouteRequest)) {
return false;
}
CalculateRouteRequest other = (CalculateRouteRequest) obj;
return Objects.equals(calculatorName(), other.calculatorName())
&& Objects.equals(carModeOptions(), other.carModeOptions()) && Objects.equals(departNow(), other.departNow())
&& hasDeparturePosition() == other.hasDeparturePosition()
&& Objects.equals(departurePosition(), other.departurePosition())
&& Objects.equals(departureTime(), other.departureTime())
&& hasDestinationPosition() == other.hasDestinationPosition()
&& Objects.equals(destinationPosition(), other.destinationPosition())
&& Objects.equals(distanceUnitAsString(), other.distanceUnitAsString())
&& Objects.equals(includeLegGeometry(), other.includeLegGeometry())
&& Objects.equals(travelModeAsString(), other.travelModeAsString())
&& Objects.equals(truckModeOptions(), other.truckModeOptions())
&& hasWaypointPositions() == other.hasWaypointPositions()
&& Objects.equals(waypointPositions(), other.waypointPositions());
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*/
@Override
public final String toString() {
return ToString.builder("CalculateRouteRequest").add("CalculatorName", calculatorName())
.add("CarModeOptions", carModeOptions()).add("DepartNow", departNow())
.add("DeparturePosition", departurePosition() == null ? null : "*** Sensitive Data Redacted ***")
.add("DepartureTime", departureTime())
.add("DestinationPosition", destinationPosition() == null ? null : "*** Sensitive Data Redacted ***")
.add("DistanceUnit", distanceUnitAsString()).add("IncludeLegGeometry", includeLegGeometry())
.add("TravelMode", travelModeAsString()).add("TruckModeOptions", truckModeOptions())
.add("WaypointPositions", waypointPositions() == null ? null : "*** Sensitive Data Redacted ***").build();
}
public final Optional getValueForField(String fieldName, Class clazz) {
switch (fieldName) {
case "CalculatorName":
return Optional.ofNullable(clazz.cast(calculatorName()));
case "CarModeOptions":
return Optional.ofNullable(clazz.cast(carModeOptions()));
case "DepartNow":
return Optional.ofNullable(clazz.cast(departNow()));
case "DeparturePosition":
return Optional.ofNullable(clazz.cast(departurePosition()));
case "DepartureTime":
return Optional.ofNullable(clazz.cast(departureTime()));
case "DestinationPosition":
return Optional.ofNullable(clazz.cast(destinationPosition()));
case "DistanceUnit":
return Optional.ofNullable(clazz.cast(distanceUnitAsString()));
case "IncludeLegGeometry":
return Optional.ofNullable(clazz.cast(includeLegGeometry()));
case "TravelMode":
return Optional.ofNullable(clazz.cast(travelModeAsString()));
case "TruckModeOptions":
return Optional.ofNullable(clazz.cast(truckModeOptions()));
case "WaypointPositions":
return Optional.ofNullable(clazz.cast(waypointPositions()));
default:
return Optional.empty();
}
}
@Override
public final List> sdkFields() {
return SDK_FIELDS;
}
private static Function
*
* Requirements: TravelMode
must be specified as Car
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder carModeOptions(CalculateRouteCarModeOptions carModeOptions);
/**
*
* Specifies route preferences when traveling by Car
, such as avoiding routes that use ferries or
* tolls.
*
*
* Requirements: TravelMode
must be specified as Car
.
*
* This is a convenience method that creates an instance of the {@link CalculateRouteCarModeOptions.Builder}
* avoiding the need to create one manually via {@link CalculateRouteCarModeOptions#builder()}.
*
* When the {@link Consumer} completes, {@link CalculateRouteCarModeOptions.Builder#build()} is called
* immediately and its result is passed to {@link #carModeOptions(CalculateRouteCarModeOptions)}.
*
* @param carModeOptions
* a consumer that will call methods on {@link CalculateRouteCarModeOptions.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #carModeOptions(CalculateRouteCarModeOptions)
*/
default Builder carModeOptions(Consumer carModeOptions) {
return carModeOptions(CalculateRouteCarModeOptions.builder().applyMutation(carModeOptions).build());
}
/**
*
* Sets the time of departure as the current time. Uses the current time to calculate a route. Otherwise, the
* best time of day to travel with the best traffic conditions is used to calculate the route.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @param departNow
* Sets the time of departure as the current time. Uses the current time to calculate a route. Otherwise,
* the best time of day to travel with the best traffic conditions is used to calculate the route.
*
* Default Value: false
*
*
* Valid Values: false
| true
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder departNow(Boolean departNow);
/**
*
* The start position for the route. Defined in WGS
* 84 format: [longitude, latitude]
.
*
*
* -
*
* For example, [-123.115, 49.285]
*
*
*
*
*
* If you specify a departure that's not located on a road, Amazon Location moves the position
* to the nearest road. If Esri is the provider for your route calculator, specifying a route that is longer
* than 400 km returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param departurePosition
* The start position for the route. Defined in WGS 84 format:
* [longitude, latitude]
.
*
* -
*
* For example, [-123.115, 49.285]
*
*
*
*
*
* If you specify a departure that's not located on a road, Amazon Location moves the
* position to the nearest road. If Esri is the provider for your route calculator, specifying a
* route that is longer than 400 km returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder departurePosition(Collection departurePosition);
/**
*
* The start position for the route. Defined in WGS
* 84 format: [longitude, latitude]
.
*
*
* -
*
* For example, [-123.115, 49.285]
*
*
*
*
*
* If you specify a departure that's not located on a road, Amazon Location moves the position
* to the nearest road. If Esri is the provider for your route calculator, specifying a route that is longer
* than 400 km returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param departurePosition
* The start position for the route. Defined in WGS 84 format:
* [longitude, latitude]
.
*
* -
*
* For example, [-123.115, 49.285]
*
*
*
*
*
* If you specify a departure that's not located on a road, Amazon Location moves the
* position to the nearest road. If Esri is the provider for your route calculator, specifying a
* route that is longer than 400 km returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder departurePosition(Double... departurePosition);
/**
*
* Specifies the desired time of departure. Uses the given time to calculate the route. Otherwise, the best time
* of day to travel with the best traffic conditions is used to calculate the route.
*
*
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
*
*
* @param departureTime
* Specifies the desired time of departure. Uses the given time to calculate the route. Otherwise, the
* best time of day to travel with the best traffic conditions is used to calculate the route.
*
* Setting a departure time in the past returns a 400 ValidationException
error.
*
*
*
* -
*
* In ISO 8601 format:
* YYYY-MM-DDThh:mm:ss.sssZ
. For example, 2020–07-2T12:15:20.000Z+01:00
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder departureTime(Instant departureTime);
/**
*
* The finish position for the route. Defined in WGS
* 84 format: [longitude, latitude]
.
*
*
* -
*
* For example, [-122.339, 47.615]
*
*
*
*
*
* If you specify a destination that's not located on a road, Amazon Location moves the position
* to the nearest road.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param destinationPosition
* The finish position for the route. Defined in WGS 84 format:
* [longitude, latitude]
.
*
* -
*
* For example, [-122.339, 47.615]
*
*
*
*
*
* If you specify a destination that's not located on a road, Amazon Location moves the
* position to the nearest road.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder destinationPosition(Collection destinationPosition);
/**
*
* The finish position for the route. Defined in WGS
* 84 format: [longitude, latitude]
.
*
*
* -
*
* For example, [-122.339, 47.615]
*
*
*
*
*
* If you specify a destination that's not located on a road, Amazon Location moves the position
* to the nearest road.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param destinationPosition
* The finish position for the route. Defined in WGS 84 format:
* [longitude, latitude]
.
*
* -
*
* For example, [-122.339, 47.615]
*
*
*
*
*
* If you specify a destination that's not located on a road, Amazon Location moves the
* position to the nearest road.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder destinationPosition(Double... destinationPosition);
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* @param distanceUnit
* Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @see DistanceUnit
* @return Returns a reference to this object so that method calls can be chained together.
* @see DistanceUnit
*/
Builder distanceUnit(String distanceUnit);
/**
*
* Set the unit system to specify the distance.
*
*
* Default Value: Kilometers
*
*
* @param distanceUnit
* Set the unit system to specify the distance.
*
* Default Value: Kilometers
* @see DistanceUnit
* @return Returns a reference to this object so that method calls can be chained together.
* @see DistanceUnit
*/
Builder distanceUnit(DistanceUnit distanceUnit);
/**
*
* Set to include the geometry details in the result for each path between a pair of positions.
*
*
* Default Value: false
*
*
* Valid Values: false
| true
*
*
* @param includeLegGeometry
* Set to include the geometry details in the result for each path between a pair of positions.
*
* Default Value: false
*
*
* Valid Values: false
| true
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder includeLegGeometry(Boolean includeLegGeometry);
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
*
*
* @param travelMode
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and
* road compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
* @see TravelMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see TravelMode
*/
Builder travelMode(String travelMode);
/**
*
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and road
* compatibility.
*
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
*
*
* @param travelMode
* Specifies the mode of transport when calculating a route. Used in estimating the speed of travel and
* road compatibility.
*
* The TravelMode
you specify also determines how you specify route preferences:
*
*
* -
*
* If traveling by Car
use the CarModeOptions
parameter.
*
*
* -
*
* If traveling by Truck
use the TruckModeOptions
parameter.
*
*
*
*
* Default Value: Car
* @see TravelMode
* @return Returns a reference to this object so that method calls can be chained together.
* @see TravelMode
*/
Builder travelMode(TravelMode travelMode);
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
*
* @param truckModeOptions
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use
* ferries or tolls, and truck specifications to consider when choosing an optimal road.
*
* Requirements: TravelMode
must be specified as Truck
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder truckModeOptions(CalculateRouteTruckModeOptions truckModeOptions);
/**
*
* Specifies route preferences when traveling by Truck
, such as avoiding routes that use ferries or
* tolls, and truck specifications to consider when choosing an optimal road.
*
*
* Requirements: TravelMode
must be specified as Truck
.
*
* This is a convenience method that creates an instance of the {@link CalculateRouteTruckModeOptions.Builder}
* avoiding the need to create one manually via {@link CalculateRouteTruckModeOptions#builder()}.
*
* When the {@link Consumer} completes, {@link CalculateRouteTruckModeOptions.Builder#build()} is called
* immediately and its result is passed to {@link #truckModeOptions(CalculateRouteTruckModeOptions)}.
*
* @param truckModeOptions
* a consumer that will call methods on {@link CalculateRouteTruckModeOptions.Builder}
* @return Returns a reference to this object so that method calls can be chained together.
* @see #truckModeOptions(CalculateRouteTruckModeOptions)
*/
default Builder truckModeOptions(Consumer truckModeOptions) {
return truckModeOptions(CalculateRouteTruckModeOptions.builder().applyMutation(truckModeOptions).build());
}
/**
*
* Specifies an ordered list of up to 23 intermediate positions to include along a route between the departure
* position and destination position.
*
*
* -
*
* For example, from the DeparturePosition
[-123.115, 49.285]
, the route follows the
* order that the waypoint positions are given [[-122.757, 49.0021],[-122.349, 47.620]]
*
*
*
*
*
* If you specify a waypoint position that's not located on a road, Amazon Location moves the position
* to the nearest road.
*
*
* Specifying more than 23 waypoints returns a 400 ValidationException
error.
*
*
* If Esri is the provider for your route calculator, specifying a route that is longer than 400 km returns a
* 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param waypointPositions
* Specifies an ordered list of up to 23 intermediate positions to include along a route between the
* departure position and destination position.
*
* -
*
* For example, from the DeparturePosition
[-123.115, 49.285]
, the route
* follows the order that the waypoint positions are given
* [[-122.757, 49.0021],[-122.349, 47.620]]
*
*
*
*
*
* If you specify a waypoint position that's not located on a road, Amazon Location moves the
* position to the nearest road.
*
*
* Specifying more than 23 waypoints returns a 400 ValidationException
error.
*
*
* If Esri is the provider for your route calculator, specifying a route that is longer than 400 km
* returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder waypointPositions(Collection extends Collection> waypointPositions);
/**
*
* Specifies an ordered list of up to 23 intermediate positions to include along a route between the departure
* position and destination position.
*
*
* -
*
* For example, from the DeparturePosition
[-123.115, 49.285]
, the route follows the
* order that the waypoint positions are given [[-122.757, 49.0021],[-122.349, 47.620]]
*
*
*
*
*
* If you specify a waypoint position that's not located on a road, Amazon Location moves the position
* to the nearest road.
*
*
* Specifying more than 23 waypoints returns a 400 ValidationException
error.
*
*
* If Esri is the provider for your route calculator, specifying a route that is longer than 400 km returns a
* 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
*
*
* @param waypointPositions
* Specifies an ordered list of up to 23 intermediate positions to include along a route between the
* departure position and destination position.
*
* -
*
* For example, from the DeparturePosition
[-123.115, 49.285]
, the route
* follows the order that the waypoint positions are given
* [[-122.757, 49.0021],[-122.349, 47.620]]
*
*
*
*
*
* If you specify a waypoint position that's not located on a road, Amazon Location moves the
* position to the nearest road.
*
*
* Specifying more than 23 waypoints returns a 400 ValidationException
error.
*
*
* If Esri is the provider for your route calculator, specifying a route that is longer than 400 km
* returns a 400 RoutesValidationException
error.
*
*
*
* Valid Values: [-180 to 180,-90 to 90]
* @return Returns a reference to this object so that method calls can be chained together.
*/
Builder waypointPositions(Collection... waypointPositions);
@Override
Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration);
@Override
Builder overrideConfiguration(Consumer builderConsumer);
}
static final class BuilderImpl extends LocationRequest.BuilderImpl implements Builder {
private String calculatorName;
private CalculateRouteCarModeOptions carModeOptions;
private Boolean departNow;
private List departurePosition = DefaultSdkAutoConstructList.getInstance();
private Instant departureTime;
private List destinationPosition = DefaultSdkAutoConstructList.getInstance();
private String distanceUnit;
private Boolean includeLegGeometry;
private String travelMode;
private CalculateRouteTruckModeOptions truckModeOptions;
private List> waypointPositions = DefaultSdkAutoConstructList.getInstance();
private BuilderImpl() {
}
private BuilderImpl(CalculateRouteRequest model) {
super(model);
calculatorName(model.calculatorName);
carModeOptions(model.carModeOptions);
departNow(model.departNow);
departurePosition(model.departurePosition);
departureTime(model.departureTime);
destinationPosition(model.destinationPosition);
distanceUnit(model.distanceUnit);
includeLegGeometry(model.includeLegGeometry);
travelMode(model.travelMode);
truckModeOptions(model.truckModeOptions);
waypointPositions(model.waypointPositions);
}
public final String getCalculatorName() {
return calculatorName;
}
public final void setCalculatorName(String calculatorName) {
this.calculatorName = calculatorName;
}
@Override
public final Builder calculatorName(String calculatorName) {
this.calculatorName = calculatorName;
return this;
}
public final CalculateRouteCarModeOptions.Builder getCarModeOptions() {
return carModeOptions != null ? carModeOptions.toBuilder() : null;
}
public final void setCarModeOptions(CalculateRouteCarModeOptions.BuilderImpl carModeOptions) {
this.carModeOptions = carModeOptions != null ? carModeOptions.build() : null;
}
@Override
public final Builder carModeOptions(CalculateRouteCarModeOptions carModeOptions) {
this.carModeOptions = carModeOptions;
return this;
}
public final Boolean getDepartNow() {
return departNow;
}
public final void setDepartNow(Boolean departNow) {
this.departNow = departNow;
}
@Override
public final Builder departNow(Boolean departNow) {
this.departNow = departNow;
return this;
}
public final Collection getDeparturePosition() {
if (departurePosition instanceof SdkAutoConstructList) {
return null;
}
return departurePosition;
}
public final void setDeparturePosition(Collection departurePosition) {
this.departurePosition = PositionCopier.copy(departurePosition);
}
@Override
public final Builder departurePosition(Collection departurePosition) {
this.departurePosition = PositionCopier.copy(departurePosition);
return this;
}
@Override
@SafeVarargs
public final Builder departurePosition(Double... departurePosition) {
departurePosition(Arrays.asList(departurePosition));
return this;
}
public final Instant getDepartureTime() {
return departureTime;
}
public final void setDepartureTime(Instant departureTime) {
this.departureTime = departureTime;
}
@Override
public final Builder departureTime(Instant departureTime) {
this.departureTime = departureTime;
return this;
}
public final Collection getDestinationPosition() {
if (destinationPosition instanceof SdkAutoConstructList) {
return null;
}
return destinationPosition;
}
public final void setDestinationPosition(Collection destinationPosition) {
this.destinationPosition = PositionCopier.copy(destinationPosition);
}
@Override
public final Builder destinationPosition(Collection destinationPosition) {
this.destinationPosition = PositionCopier.copy(destinationPosition);
return this;
}
@Override
@SafeVarargs
public final Builder destinationPosition(Double... destinationPosition) {
destinationPosition(Arrays.asList(destinationPosition));
return this;
}
public final String getDistanceUnit() {
return distanceUnit;
}
public final void setDistanceUnit(String distanceUnit) {
this.distanceUnit = distanceUnit;
}
@Override
public final Builder distanceUnit(String distanceUnit) {
this.distanceUnit = distanceUnit;
return this;
}
@Override
public final Builder distanceUnit(DistanceUnit distanceUnit) {
this.distanceUnit(distanceUnit == null ? null : distanceUnit.toString());
return this;
}
public final Boolean getIncludeLegGeometry() {
return includeLegGeometry;
}
public final void setIncludeLegGeometry(Boolean includeLegGeometry) {
this.includeLegGeometry = includeLegGeometry;
}
@Override
public final Builder includeLegGeometry(Boolean includeLegGeometry) {
this.includeLegGeometry = includeLegGeometry;
return this;
}
public final String getTravelMode() {
return travelMode;
}
public final void setTravelMode(String travelMode) {
this.travelMode = travelMode;
}
@Override
public final Builder travelMode(String travelMode) {
this.travelMode = travelMode;
return this;
}
@Override
public final Builder travelMode(TravelMode travelMode) {
this.travelMode(travelMode == null ? null : travelMode.toString());
return this;
}
public final CalculateRouteTruckModeOptions.Builder getTruckModeOptions() {
return truckModeOptions != null ? truckModeOptions.toBuilder() : null;
}
public final void setTruckModeOptions(CalculateRouteTruckModeOptions.BuilderImpl truckModeOptions) {
this.truckModeOptions = truckModeOptions != null ? truckModeOptions.build() : null;
}
@Override
public final Builder truckModeOptions(CalculateRouteTruckModeOptions truckModeOptions) {
this.truckModeOptions = truckModeOptions;
return this;
}
public final Collection extends Collection> getWaypointPositions() {
if (waypointPositions instanceof SdkAutoConstructList) {
return null;
}
return waypointPositions;
}
public final void setWaypointPositions(Collection extends Collection> waypointPositions) {
this.waypointPositions = CalculateRouteRequestWaypointPositionsListCopier.copy(waypointPositions);
}
@Override
public final Builder waypointPositions(Collection extends Collection> waypointPositions) {
this.waypointPositions = CalculateRouteRequestWaypointPositionsListCopier.copy(waypointPositions);
return this;
}
@Override
@SafeVarargs
public final Builder waypointPositions(Collection... waypointPositions) {
waypointPositions(Arrays.asList(waypointPositions));
return this;
}
@Override
public Builder overrideConfiguration(AwsRequestOverrideConfiguration overrideConfiguration) {
super.overrideConfiguration(overrideConfiguration);
return this;
}
@Override
public Builder overrideConfiguration(Consumer builderConsumer) {
super.overrideConfiguration(builderConsumer);
return this;
}
@Override
public CalculateRouteRequest build() {
return new CalculateRouteRequest(this);
}
@Override
public List> sdkFields() {
return SDK_FIELDS;
}
}
}